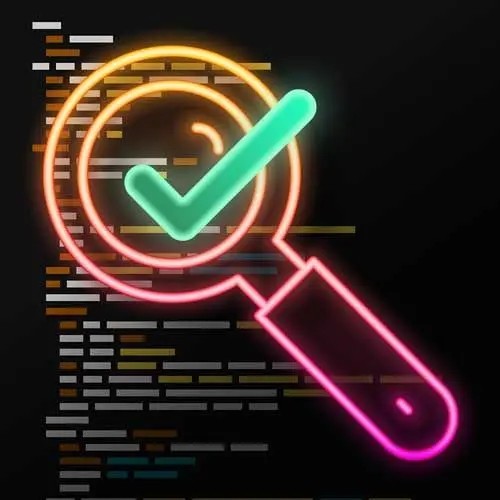
Lesson Description
The "Using Testing Library Utilities" Lesson is part of the full, Testing Fundamentals course featured in this preview video. Here's what you'd learn in this lesson:
Steve introduces Testing Library which provides additional utility methods for testing DOM elements and events. An added benefit of Testing Library is the built-in selectors find elements the way users and screen readers do, so you are encouraged to write more accessible code.
Transcript from the "Using Testing Library Utilities" Lesson
[00:00:00]
>> Steve Kinney: And so, there is this thing called testing library, right, which you kinda saw hiding in that V test config. And you can even see, if you need any more convincing on how close these two testing libraries are, that should tell you everything you really need to know.
[00:00:20]
There is this library called testing library. So, testing library is a library that allows us to kind of have a bunch of helpers around working in the DOM. We have turned on the DOM, right? But things like querying for various things in the DOM with an expect helper can be a little tricky.
[00:00:40]
Some of those user events that we saw before as well, can become tricky as well, but this test works, but let's see if we can make it a little bit better. So for instance, one thing we could do is we could import,
>> Steve Kinney: Actually, there's no render for this one.
[00:01:13]
>> Steve Kinney: We can import this screen from testing library DOM. To the question I got earlier which inspired this little live decision that I made, which is, if you were just using regular DOM nodes, you use testing-library/dom. If you would like to work with React components.
>> Steve Kinney: If you would like to work with Svelte components.
[00:01:42]
If you would like to work with Vue components, right? Beyond that, there is very little difference between the bunch of them, which we'll see momentarily. But we'll start out with this one right here. So we can start out with the screen. And so, what screen does as effectively as you can imagine is the browser window, right?
[00:02:09]
And one of the things that testing library does is it's got a bunch of selectors for finding DOM nodes, right? If you've ever used jQuery before, you know this like the back of your hand. If you've used something like document.querySelector, you've done this as well. The interesting part about testing library that I think is super cool is that it forces you to use selectors that are based on how a screen reader would find things.
[00:02:36]
Which means, it forces you to both write your test and thereby your markup in a way that is accessible, right? And so, it allows you to kinda like query stuff along those lines, and allows you to have a version of the screen that you can kinda see. So this is less about a DOM node and more about what is on the page, how would a screen reader find it, and finding the elements like that as well.
[00:03:02]
And so, what we could do here is we could have our button and we could do something like, we can put it on the page.
>> Steve Kinney: So in this case, let me just do, I'm using replaceChild() instead of a penChild(). So if I use an additional test, it's gonna swap everything out with a fresh button.
[00:03:25]
Otherwise, you could end up with three buttons on the page, because life's hard. Button, and now what I could say is something like,
>> Steve Kinney: Let's actually just only use that variable name.
>> Steve Kinney: So I could say something like, we're gonna put that on the body, and this is something I might choose to do.
[00:03:52]
If I was gonna use the same button in every test, I could choose to put this in a before each, right? But in this case, I'm just gonna put it in line with this one. And then I could say something like, const_button = screen getByRoll. In this case, we want a button.
[00:04:14]
And I could say one that has,
>> Steve Kinney: Right, what are you angry about?
>> Steve Kinney: I'll do that for now. So we've got the button, the nice part too is, theoretically, this is a reasonable test. Because if it couldn't find a button with click me on the page, then this test would fail, right?
[00:04:52]
So I said one with,
>> Steve Kinney: The act of find, this is where that like thing we're talking about before helps us, if it can't find one on the page, that'll throw an error. So by definition, this is theoretically a reasonable test. And so at this point, we can do, or you can even do something like expect screen or expect button.
[00:05:30]
>> Steve Kinney: Can't remember what I started this with.
>> Steve Kinney: Cool, and so, here we've got one where it's kind of using it from the screen reader version, right? Where we say, hey, put this thing on the body, right? And then, from the perspective of the screen, go find that button.
[00:05:54]
And then in this case, it's in the document. And this test is now doing effectively the same thing, so we can get rid of it. Where this can become more interesting is this idea of click, right? There's a few ways that we can kind of improve upon this.
[00:06:12]
And we'll kinda take it slowly and do it in a few other examples. As you can see, there are plenty of examples that we have to play around with here. So we'll get our muscle memory behind us. But what we can do here is the same basic idea, what I might do after every test.
[00:06:35]
>> Steve Kinney: Is just say,
>> Steve Kinney: Document.
>> Steve Kinney: I don't know if it works for the DOM1, but for React and stuff, it will clear out the DOM in between every test, I just don't remember if it does it for this one. So what we'll do is, we'll do the same thing we said before here.
[00:07:03]
Now, instead of calling .click, what we can do is pull in this, fire an event, which will actually, instead of calling the click method, basically, take a a little event and shoot it at the button, right? And so, what we can do is,
>> Steve Kinney: GetByRoll, in this case, Button,
>> Steve Kinney: And we'll go with the name.
[00:07:47]
>> Steve Kinney: Click here, where is it lowercase? Yep, and what we can do at this point is say,
>> Steve Kinney: FireEvent at the button.
>> Steve Kinney: It's gotta be,
>> Steve Kinney: Did end up with two on the screen.
>> Steve Kinney: The nice part is you can see this is the DOM I'm working with, I gotta deal with this in a second, but I ended up with two buttons on the screen.
[00:08:38]
I'll deal with that one moment, as I get my major point across here.
>> Steve Kinney: So we've made this a little bit better, right? We now fire an event at it, which is, again, from the perspective of the browser. There's another library along with testing library called user-event.
>> Steve Kinney: Which will then do what we were saying before, where it will simulate everything that would go on with a user actually doing it.
[00:09:11]
And so this is just an abstraction. It'll do, kinda the mouse enter and the click, it'll do all of the things that a user would normally do to interact with an element like this, and simulates that a little bit better. So in this case, it does need to be async function because,
>> Steve Kinney: And so now, this is basically simulating everything that would happen in an actual user event.
[00:09:44]
The end result is the same. Now, cuz this is a very, very, very simple test, but the idea here is now we are mounting it on the page, like a real page, right? We are querying that it is in the DOM with the right roles, and we're getting it.
[00:09:58]
We are doing a real click, and then we are, in fact, verifying, we could even, this is the same button in memory, we could also grab it again, and so on and so forth, right? But again, we thought about the arrange, act, and assert. By arranging, we're rendering it on the page, we will go ahead find it on the page to make sure it was mounted correctly as we expected.
[00:10:29]
Click on it, and then verify that thing changed the way that we wanted it to.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops