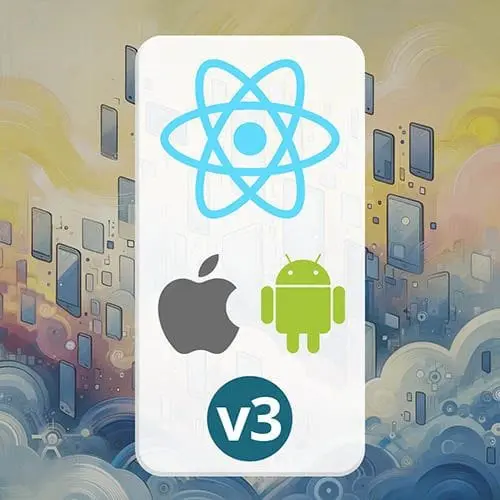
Check out a free preview of the full React Native, v3 course
The "React Components" Lesson is part of the full, React Native, v3 course featured in this preview video. Here's what you'd learn in this lesson:
Kadi explains that components allow for modularizing the app and making it easier to manage. She demonstrates how to create a shopping list item component, import it into the main app file, pass props to components, and use TypeScript to define the types of the props.
Transcript from the "React Components" Lesson
[00:00:00]
>> Kadi Kraman: Next up, let's talk about components. So, components are a React concept, and that's also a React Native concept. But basically, if we were to keep building our app and we put everything inside this app.tsx, this file is going to get massive and very difficult to use. So with React, we can have components to create our app as a modular entity.
[00:00:30]
So the common thing to do is we create a components folder,
>> Kadi Kraman: And you can have components in here. So, let's call this ShoppingListItem,
>> Kadi Kraman: .tsx. [COUGH]
>> Kadi Kraman: So right now, so you need to export a component from here. You could do export default. I think the modern thing to do these days is to do an export, I do export const.
[00:01:02]
But I said I'm using functions for components, so let's do an export function ShoppingListItem.
>> Kadi Kraman: So you name it the same as the file. And let's return, will return null for now. And what we want to do is we want to pull this item that we created into our ShoppingListItem component.
[00:01:28]
So let's copy all of this,
>> Kadi Kraman: And return it from our ShoppingListItem. Obviously, I'm getting lots of ESLint errors. Reason why you want ESLint, it will tell you that things are not right, saying that things aren't imported. So I'm gonna import View from react-native, Text from react-native, TouchableOpacity from react-native.
[00:01:58]
>> Kadi Kraman: There we go. And we also need our styles, so let me just copy this whole styles object here.
>> Kadi Kraman: There we go, we need StyleSheet.
>> Kadi Kraman: We need to move our handleDelete function.
>> Kadi Kraman: And this means we also need the Alert from react-native. There we go. And now, instead of having this item here, we can actually import, ShoppinListItem.
[00:02:46]
There we go.
>> Kadi Kraman: I've forgotten to import theme. There we go. Let's import theme as well. So wait from here, okay? So, now I can have multiple of my ShoppingListItem and this is obviously a little bit useless, because I have all the items say the same thing. So we want from this app.tsx to say what the ShoppingListItems should be called.
[00:03:15]
So to do that, we can pass it props or properties. And props are passed in, well, let's start it from here. So, we'll pass in from here. So let's say this is,
>> Kadi Kraman: Gonna make sure our name is the same thing. The name, let's call it coffee. What else do we need?
[00:03:38]
Some tea and some sugar. Okay, so this gives me a squiggly, because it's saying that there is no proper code name, and that's because we haven't defined them here yet. So these get passed in as an object so we can destructure them as the function arguments, which is really handy.
[00:04:03]
And because we are using Typescript, we can type them. This is again a convention just to do a type called props. And this defines what the properties are, so let's do name. And this name is a string. Those that were maybe an hour in, this is the first sort of Typescript we've done, so this is not TypeScript heavy, don't worry.
[00:04:27]
But I will mention one thing that if you want this to be optional, so right now, if I don't pass this in, I'm getting an error. If I wanted this to be optional, I would do a question mark, so this allows me to not pass it in. In our case, we want to make sure that people always pass in a name, so we're gonna make it a mandatory argument.
[00:04:50]
And in our text, instead of Coffee, let's use this property. So if you want to render any text in line, you put it into these curlies, and then they renders the string that you passed in. And actually now that we have our name, we can update our Alert to say instead of are you sure you want to delete this, you could say the name of the item you want to delete.
[00:05:16]
So, I wanna use backticks. So with backticks, you can embed arbitrary strings or numbers into your text. So we can see, are you sure you want to delete? So do your dollar sign, some squigglies, and name. So I guess they will say, are you sure you want to delete T?
[00:05:36]
>> Kadi Kraman: And the last thing, this is optional. We're going to talk about ESLint again. But we've done some refactoring and we've done some copying of styles. But we have styles here that we're not using, and we have styles here that we're not using and it's really difficult to see which ones we want to keep or not.
[00:05:54]
And I can't be asked to go through all of these. So this is a really handy ESLint plugin. It's a React Native ESLint plugin, and it has a couple of different properties here, but the one that I'm interested about is the no-unused styles. So let's install eslint-plugin-react-native.
>> Kadi Kraman: And this gives us this react-native plugin in our ESLint RC.
[00:06:31]
So let's go to ESLint RC. So whenever you install a plugin, it will show up here. So we installed the react-native plugin, so we'll go into the plugins array, and then this allows us to use the rules from that plugin. ESLint is wild. So, there's a bunch of rules, but I'm just gonna opt into this one.
[00:06:53]
And what this gives me, is now when I go back, it actually redlines all the styles that I'm not using in this file, because I've copied them over. So I can safely delete them,
>> Kadi Kraman: And also delete these unused imports.
>> Kadi Kraman: And the same here, I think I copied the whole styles object, but we don't need the container.
[00:07:20]
The StyleSheet.create, could you cover again what that does? So what StyleSheet.create does, it doesn't do a lot, [LAUGH] actually. So in production, all this does is it creates an object. If you look at the React Native as open source, also if you look at the code for React Native in production, it actually just creates an object of styles, so it doesn't do anything.
[00:07:45]
In Dev, it does some checking to make sure that the things that you pass in are valid. There's more validation for that. I think it came from the earlier days of React Native, where there was more validation needed, because the Styles got passed directly into Native Components and if you passed stars didn't exist, things would crash.
[00:08:02]
Nowadays, there's more production around it, but yeah, it's kind of a leftover, so you could actually just do this kind of, and it would work, although TypeScript would yell at you. So, I think the idea was that eventually StyleSheet.create could have some performance improvements like deduplicating styles or anything like that.
[00:08:27]
But yeah, right now, it doesn't really have anything [LAUGH] mysterious going on under the hood unfortunately. I think it does have some utility methods, so you can flatten styles or compose styles. This is actually handy, like an absolute fill and absolute fill object. There you go. So if you ever want a component to take off the position absolute take off the full width of the screen, there's actually a convenience function where if you do a View Style,
>> Kadi Kraman: Well, actually this would be useless, because it doesn't have a background.
[00:09:13]
Let's give it a background as well.
>> Kadi Kraman: So, backgroundColor pink. There you go. So this is a object that is full width of the screen, so it's rendering underneath here. But if I move it, this actually shows you the order of rendering as well, which is kind of interesting.
[00:09:32]
So when I put it on top of my ShoppingListItem, it renders underneath, and if I put it at the bottom, it renders over the top. So it's kind of a convenience function, or there's also an absolute fill object.
>> Kadi Kraman: StyleSheet, oops,
>> Kadi Kraman: Absolute fill object, which does the same, but it's the styles as an object so you can spread them and add additional styles like I did here, so just utility.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops