Learn JavaScript
Guide for getting started with JavaScript
This guide is not itself the means to learn JavaScript. This guide addresses issues around getting started (obstacles, preliminary knowledge, etc.) and then points you to curated resources to start learning. Consider this material a prologue or an introduction to the learning process itself so, you have the needed background and context before beginning the learning process.
Required Prerequisite Knowledge
The list below entails the knowledge required before attempting to learn JavaScript:
- A necessary step to learning any programming language is first to understand how computer programming languages work in general (i.e., the conceptual mechanics of any programming language). We’ve yet to find a better book for this than “How Computer Programming Works” by Daniel Appleman. The book is old, and the language used isn’t JavaScript, but the mechanics it teaches are relevant to all languages. If you prefer to learn by instruction and want a lot more details, look at the Crash Course on Computer Science (episodes: #11, #12, #13, #14, #16).
- A basic understanding of the internet, web browsers, HTML (i.e. web pages), and CSS.
- A basic understanding of a command line interface (see Crash Course #22) and a graphical user interface (see Crash Course #26).
Helpful Background
- In 1995 a programming language, which we call JavaScript today, was written in ten days and embedded into the Netscape web browser (i.e., a host environment) to validate user inputted data into HTML forms. That is the origin story of JavaScript in a nutshell. Fast forward 27 years, and today it is the most used programming language in the world and is not limited to web browser runtimes. JavaScript, today is used almost anywhere a programmer can prefer it. From the front-end (e.g., React) and back-end (e.g., Express) of a web application, to command CLI tools (e.g., ESlint), to a database (e.g., MongoDB). JavaScript is everywhere, and professionals and as well as novices use it.
- JavaScript is a scripted programming language. It typically is found in a “host environment” where a programing language is required for scripting or programming something using an application programming interface (aka APIs). In the context of web browsers, JavaScript works along with web APIs like the BOM (i.e., Browser Object Model), DOM (i.e., Document Object Model, and CSSOM (i.e., CSS Object Model) to script or program web browsers and web pages. In the context of Node.js, JavaScript is the language used to produce applications and tools using Node.js APIs.
- JavaScript requires an engine to execute JavaScript source code. JavaScript is written using a text/code editor and then fed into an ECMAScript engine to be run/executed in the context of a host environment. The two most common host environments are a web browser and Node.js. The most well-known engine is V8. The V8 engine is the engine used by the Edge and Chrome web browsers as well as Node.js.
- JavaScript has undergone two historically significant language changes. The first occurred in 2009 when ES5 was released, and the second occurred in 2015 when ES6 was released (Note: ES6 today is called “ES2015”). I’m not going to list the language changes because, as of 2022, the details are not as important as they once were. Just beware that ES3 to ES5 and ES5 to ES6 was when the two most significant changes to the language occurred.
- Today small incremental changes to the JavaScript language occur yearly. Before 2015 changes to the language were sparse and often separated by several years. The name/label given to these yearly changes is ES2015, ES2016, ES2017, ES2018, etc. This year, the language will have a set of changes known as ES2022.
Getting Started Obstacles
- Once upon a time, some perceived JavaScript as an inferior programming language for professional endeavors. While this is no longer the case today, you might still encounter this notion. One can mostly ignore this opinion as of today, given JavaScript is used in a wide variety of professional contexts today.
- Newbies and even some veterans of the JavaScript language don’t realize that the word “JavaScript” is interchangeable with “ECMAScript.” JavaScript is the commercial name for ECMAScript and a historical relic. The actual name of the programming language in use today is ECMAScript. Anytime you hear JavaScript, you can mentally replace it with “ECMAScript.” ECMAScript is the programming language used by ECMAScript engines like V8. Consider that the module format used in JavaScript is called “ECMAScript Modules,” also known as “ES Modules” or “ESM.” And, consider that if you want to know which language features are available in a web browser or Node.js, you have to review which ECMAScript specification is supported by the engine (e.g., ES3, ES5, ES6, ES2015 thru ES2023). As you learn JavaScript, be aware that the core language, commonly called “JavaScript,” is technically not “JavaScript”; it is ECMAScript. Knowing this will elevate several layers of indirection as you learn about the language.
- You will be sorely disappointed if you think you can learn programming or JavaScript by reading the ECMAScript specification. The ECMAScript specification does not exist with the intent of being a resource for learning programming or JavaScript. The ECMAScript specification defines the language details for engineers building an ECMAScript engine (e.g., V8). At times, however, advanced JavaScript programmers might read up on the rules of the language by reading the language specification. Regardless, you should know that the document is not written as a learning resource but to help engine authors develop JavaScript engines.
- Focus on the language, alone, at first! Learning JavaScript does not have to come with the overhead of understanding the host environments in which JavaScript runs, regardless of what millions of tutorials on the web directly and indirectly assert. Learning JavaScript is more accessible when first focusing on the language and ignoring the runtimes. If you are new to programming, focus on the language, not web or Node.js APIs (Yes, you’ll need to know enough about a JavaScript runtime to run JavaScript. But learn enough to run JavaScript and then focus on the language).
- JavaScript is not a strongly typed language. It is a loosely typed language. I’m not going to get into what this means because if you are reading this guide, this distinction will either be lost on you or cause noise. TypeScript exists to add a non-native strongly typed syntax to JavaScript. If you are new to programming and coming to JavaScript without programming experience, don’t learn TypeScript before learning JavaScript. Resist the urge to jump on the TypeScript bandwagon until you have an informed and experienced reason, which comes from knowing JavaScript first.
- You’ll bump up against JavaScript compilers sooner than later while learning JavaScript. Babel, Typescript, SWC, and Surcase all take ECMAScript and even TypeScript and compile it to a backward-compatible version of JavaScript (e.g. ES2022 to ES3). Using a compiler before understanding why you need one can be problematic. Don’t compile JavaScript until you know why you are compiling.
- JavaScript had no built-in module mechanism for a long time, and several bolt-on solutions stood up over the years to fill the void (e.g., CJS and AMD). This period is coming to a close. Yippie! We’ve come a long way, and mass adoption of the official ES module format is taking place. But the history of JavaScript modules is fragmented and comes with a long tail. Trying to understand modules before you understand JavaScript will cause indirection. Don’t focus on ES modules before you know the language. When you have a firm grasp of the language, your next learning stop should be ES Modules.
- If you are new to installing and using packages, there is a lot of historical information and a complicated learning curve. JavaScript packages can use different module formats; ESM, CJS, or AMD. Also, the UMD module format combines and abstracts other formats like ESM, CJS, and AMD. Due to the sorted and complicated history of JavaScript module formats, there is also a complex history around the formats found in the npm registry, which is a public registry of JavaScript packages (a package is a set of JavaScript modules). Set all this aside while one learns JavaScript.
- JavaScript Object Notation (aka JSON) is another topic that might not land with you until you understand JavaScript datatypes and some basic programming concepts. Note that the JSON syntax isn’t part of the ECMAScript specification, and the community conventionally drives any standardized usage. However, JavaScript does provide JSON methods to parse and stringify JSON. As a JavaScript neophyte, I would set aside trying to understand JSON until you understand JavaScript datatypes and JavaScript’s implementation of strings, objects, and arrays. If you need a mental model slot to place JSON into today, consider JSON a conventional way of writing data as text, and simultaneously valid JavaScript, in a universally understandable way so that other languages can transport and translate the data.
- You might hear about JavaScript fatigue because the JavaScript development scene is massive and comes with an enormous spectrum of solutions, tools, and churn. It makes sense, given JavaScript is the most used programming language in the world. Set all this churning noise aside until you spend time learning the language. The language is solid.
Why Learn/Use JavaScript?
One should learn JavaScript because:
- JavaScript is the most ubiquitous programming language in the world.
- Ideal for those new to programming.
- To start running JavaScript is accessible and straightforward (i.e., open a web browser and start writing JavaScript immediately in the web developer tools console).
- The job market is vast for developers who know JavaScript.
- A sea of instructional resources (i.e., Traditional Degree Programs, Boot Camps, Independent Learning).
- JavaScript is very unlikely to become unpopular anytime soon.
Top Use Cases of JavaScript
The web platform (i.e., web browsers) and Node.js are the two most common runtimes that use JavaScript as their programming language. We’ll briefly review both runtimes and then discuss the commonality between the two runtimes (i.e., a JavaScript engine).
The Web Platform Runtime:
As you might know, HTML or hypertext markup language is the foundational language used to construct a web page. When opened in a web browser, the following HTML file will render the text “Hello, I’m a webpage.”
<!DOCTYPE html>
<html>
<head>
<title>Title of webpage</title>
</head>
<body>
<h1>
Hello, I'm a webpage.
</h1>
</body>
</html>
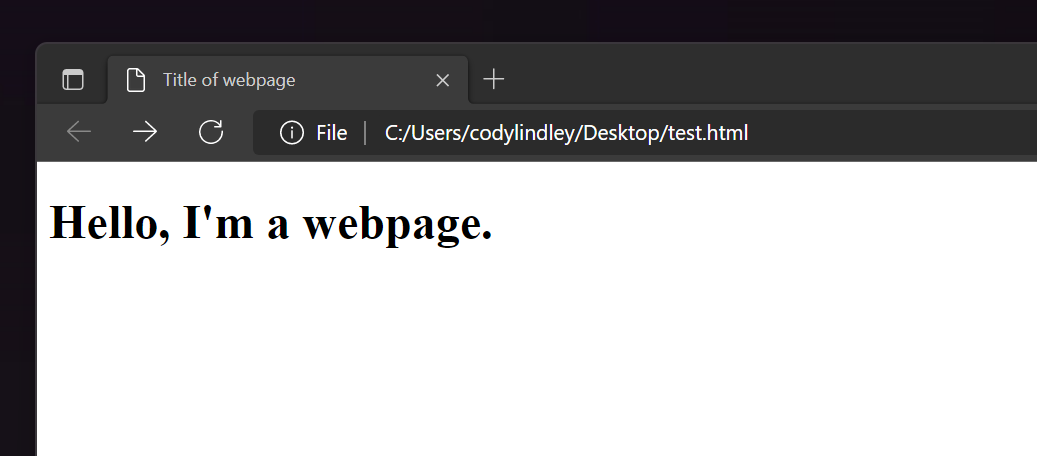
JavaScript is the scripting language used to add programmatic behavior to web browsers and web pages (i.e., UI activities and interactions based on events).
<!DOCTYPE html>
<html>
<head>
<title>Name of webpage</title>
</head>
<body>
<h1>
Hello, I'm a webpage.
<span onclick="callThisFunction()">Click this text.</span>
</h1>
<script>
// e.g. programming aspects of the web page
const callThisFunction = () => {
document
.getElementsByTagName('h1')[0]
.insertAdjacentHTML(
"afterend",
"JavaScript adds behavior to HTML and CSS (i.e. webpages)"
);
// e.g. programming aspects of the browser
setTimeout(() => {
alert("you change the web page");
}, 2000);
};
</script>
</body>
</html>
In the previous HTML code example, we are using JavaScript the language to call the runtime DOM API’s getElementById() and insertAdjacentHTML() as well as the BOM API’s alert() and setTimeout(). Using JavaScript runtime API’s, the webpage and browser window become scriptable (e.g., click the text in the webpage, and the webpage updates with new HTML, and then a couple of seconds later, you get a browser alert that the webpage has changed).
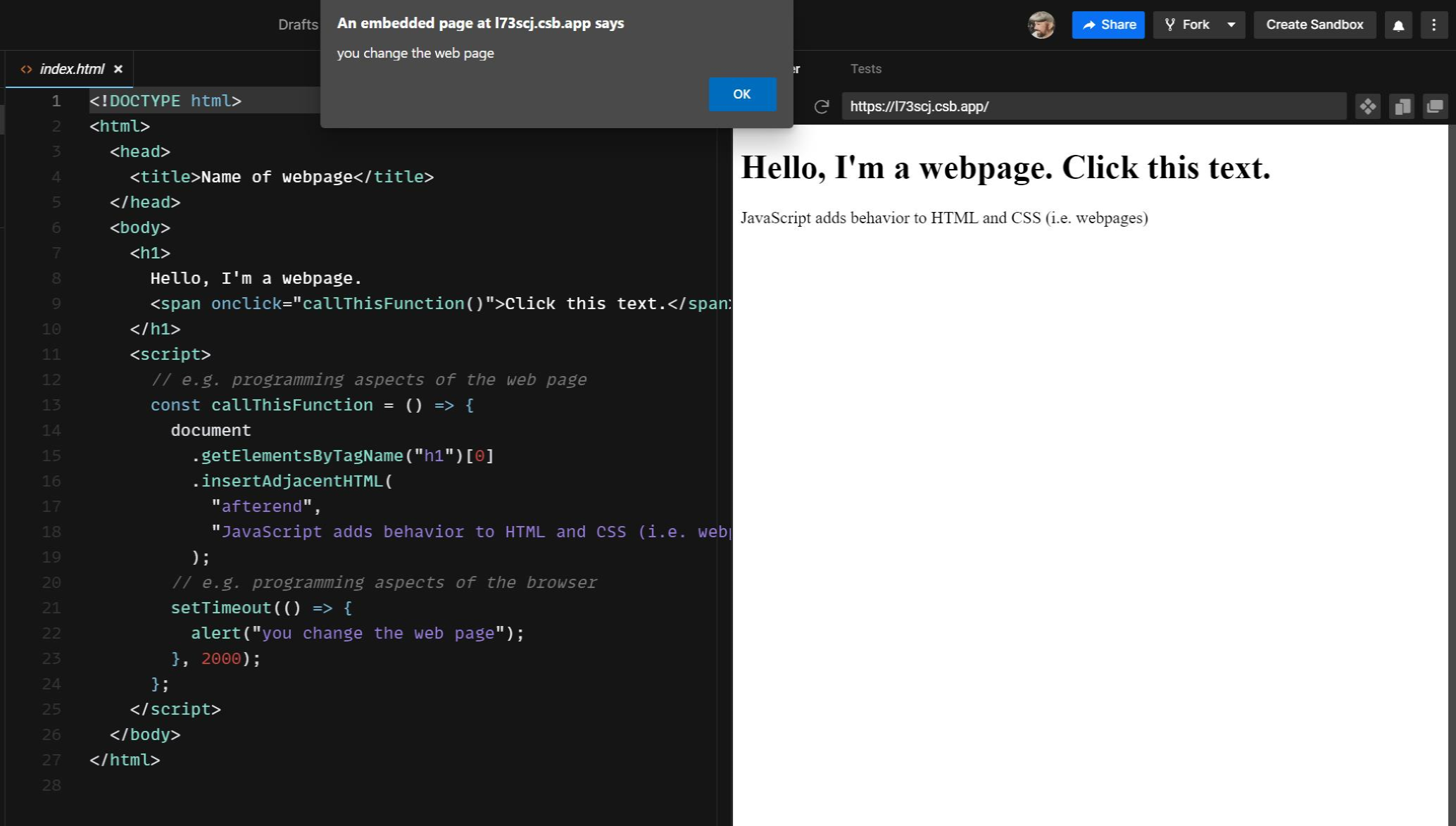
This small webpage is just a tiny glimpse of what can be programmed on the web platform using the JavaScript programming language. Note that JavaScript is the scripting language used to program web browsers and webpages.
Node.js Runtime:
Node.js, loosely speaking, is a command line application that runs a standalone ECMAScript engine that runs Node.js applications. This JavaScript environment’s purpose is to author JavaScript applications like web servers (e.g., express.js) and CLI tools (e.g., ESLint and Prettier) outside of the browser.
The following JavaScript code is a Node.js application that imports an internal http node module to set up a basic web server.
import http from "http";
const hostname = "127.0.0.1";
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader("Content-Type", "text/plain");
res.end("Hello World");
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
Typically, Node gets installed on your local system or a server, but one can run the previous Node.js application online for demonstration purposes.
Visit the link below:
https://stackblitz.com/edit/node-eby9e1?embed=1&file=index.js
When the link is open in a web browser, run the JavaScript source code from the terminal using the command “node index.js.”
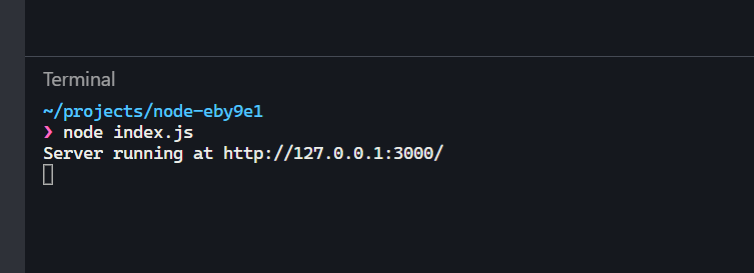
Running the command will start the Node.js application and server the results to a browser view (i.e., “Hello World”).
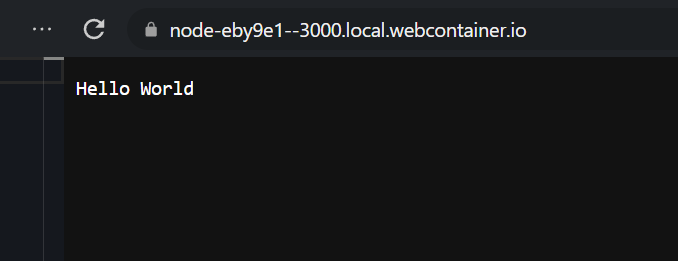
If you see “Hello World,” then you are using the Node.js CLI application to run the Node.js source code (i.e., “node index.js”), and this source code is a small application that servers a plain text document to a web browser from a web server.
Note that in the context of a Node.js application, JavaScript is viewed as a programming language to create Node.js applications.
JavaScript Engines:
Web browsers and Node.js have different runtime details, but both use a JavaScript engine. The context of what you are doing with the language differs, but JavaScript and the engine can be the same. So, for example, whether you open a JavaScript console in the latest version of the Chrome web browser and execute some JavaScript or open a .js file and begin writing a Node.js application executed by the Node REPL, both are being run by a version of the V8 ECMAScript engine.
Before Learning JavaScript
We suggest selecting and becoming comfortable using the following before you begin learning JavaScript:
- A JavaScript authoring tool (i.e., a text or code editor, e.g., VS Code)
- A JavaScript runtime (e.g., web pages, JSFiddle, or Node.js)
- A JavaScript reference (i.e., language and support documentation)
Authoring Tool:
Today most developers use VS Code for authoring JavaScript. We recommend using VS Code but using a simple text editor will get the job done if you prefer not to add on the overhead of learning how to use a code editor. Alternatively, if you want to start ASAP, you can always just open JSFiddle and start typing JavaScript into the JavaScript pane and then press the “Run” button.
Runtime:
One will need to select a runtime in which they plan on learning JavaScript. We recommend starting with a web browser and html (i.e., webpages) instead of Node.js if you are new to programming (e.g., Edge or Chrome will do).
Reference:
There is only one JavaScript reference we recommend using when studying the built-in parts of the language, the Mozilla JavaScript reference (aka MDN). We also recommend purposely avoiding all other reference sources while first learning JavaScript. When searching the web, focus on MDN resources by adding the “mdn” string to all of your searches (e.g., “mdn array foreach") so that MDN results show up first.
Start Learning JavaScript
Here is a three-step topical outline including learning resources to initiate learning JavaScript:
Step 1 - Learn Programming fundamentals Step 2 - Learn Intermediate & Advanced Programming Paradigms Step 3 - Learn ES Modules
You must spend enough time in Step 1 before moving to Step 2. If you are intimidated or struggling with Step 2, return to Step 1 and spend more time with the fundamentals of the language.
Step 1 - Learn Programming fundamentals
1. Programming Basics
1. values
2. operations
3. variables
4. expressions and statements
5. decisions
6. loops
7. functions
2. Types & Coercion
3. Scope & Closure
Video Courses:
- Learn JavaScript [free]
- Getting Started with JavaScript, v2 [free]
- Complete JavaScript Learning Path [$]
Reading Material:
- JavaScript basics [free]
- JavaScript First Steps [free]
- You Don’t Know JS Yet: Get Started - 2nd Edition [free to $]
- MDN A re-introduction to JavaScript (JS tutorial) [free]
- Eloquent JavaScript [free to $] - Chapters 1 - 12
Step 2 - Learn Intermediate & Advanced Programming Paradigms
JavaScript Object Oriented Programming (aka OOP)/Classes
Video Course: The Hard Parts of Object Oriented JavaScript [$]
Reading Material: The Principles of Object-Oriented JavaScript 1st Edition [$]
Functional Programming with JavaScript
Video Course: Functional JavaScript First Steps [$] The Hard Parts of Functional JavaScript [$]
JavaScript Async/Promises
Video Course: JavaScript: The New Hard Parts [$]
Reading Material: Understanding JavaScript Promises [$]
Step 3 - Learn ES Modules
Reading Material:
Chapter 9 : Using ES2015 Modules Today [free] Chapter 10 : Writing ES2015 Module Syntax [free] Modules • JavaScript for impatient programmers [free to $]
Commonly Asked JavaScript Questions
What is JavaScript?
Answer:
Simplistically expressed, JavaScript is a programming language used to program runtimes like web browsers & web pages as well as Node.js applications. If you are new to programming languages, that is about all you can understand at this point. If you’re a seasoned programmer, then what you need to know is:
“JavaScript (often shortened to JS) is a lightweight, interpreted, object-oriented language with first-class functions, and is best known as the scripting language for Web pages, but it’s used in many non-browser environments as well. It is a prototype-based, multi-paradigm scripting language that is dynamic, and supports object-oriented, imperative, and functional programming styles.” - MDN reference: About JavaScript
What is a JavaScript Array?
Answer:
How would one store multiple usernames as a single value called “userNames” in JavaScript?
Doing this with one comma-separated JavaScript string wouldn’t be ideal.
let userNames = "Pat Jones, Bob Riley, Cody Dallas";
As a comma-separated string value, there isn’t an ideal way to operate on each value as a separate value.
A JavaScript Array is a value in JavaScript that holds multiple values that can be stored and accessed using a numeric index starting at zero. (i.e., separate values stored and accessed using a numeric order). A better solution would be to use a JavaScript Array so each value can be stored and accessed as an individual value.
// create an array with three string values
let userNames = ["Pat Jones", "Bob Riley", "Cody Dallas"]; // short hand Array syntax
// access each value in the array
console.log(userNames[0]); // logs 'Pat Jones' at index 0 in the array
console.log(userNames[1]); // logs 'Bob Riley' at index 1 in the array
console.log(userNames[2]); // logs 'Cody Dallas' at index 2 in the array
console.log(userNames.length); // logs 3
Once you have a set of values in an Array, JavaScript provides built-in properties (e.g., .length) and methods (e.g., .forEach()) that are incredibly handy when working on Array values.
What is a JavaScript Loop?
Answer:
To output the numbers between 0 and 50 (i.e., 1 thru 49) using JavaScript we could log out one digit at a time using a console.log() method.
console.log(1);
console.log(2);
console.log(3);
console.log(4);
console.log(5);
console.log(6);
// ... so on, and so on
// logs
// 1
// 2
// 3
// 4
// ... to 49
Logging each digit would be redundant and unnecessary, and given that programming languages offer a mechanism to repeat code, a better solution exists. A JavaScript loop to repeat code without manually writing out each iteration would be a better solution.
The code below uses JavaScript for statement to loop over a console.log() method, calling the .log() method 49 times with the current value of i upon each iteration of the loop.
/* We can read the following JavaScript like so: i is equal to 1, if i is
less than 50 do a loop (i.e. run the code inside the loop) with the current value of i
then increment the value of i by 1 and do another loop
as long as i is less than 50 */
for (let i = 1; i < 50; i = i + 1) {
console.log(i);
}
// stop looping when i is greater than 50
// logs
// 1
// 2
// 3
// 4
// ... to 49
The result is the same as if we had written out 49 sequential console.log()’s, each with a numerical value.
JavaScript loops are simply a mechanism that will call code repeatedly until you tell it to stop (e.g., break).
JavaScript has many different looping mechanisms, some generic and some value specific. Below is a list of these looping mechanisms:
- for statement
- do…while statement
- while statement
- for…in statement
- for…of statement
- Array
every()
filter()
flatMap()
forEach()
map()
reduce()
reduceRight()
How do I use the Array methods .map()
and .forEach()
?
Answer:
The .forEach()
method defines a function that is called for each value in an Array (i.e., all the values from the array are sequentially passed into the function).
The task of looping over an Array of strings is made easy with the .forEach()
method.
Below, the index and value from the animals array is log to the console using .forEach()
.
const animals = ["dog", "cat", "rat", "snake", "bird"];
// loop over animals passing each value to a function, the function runs console.log()
animals.forEach(function (value, valueIndex) {
console.log(`at index ${valueIndex} the value is ${value}`);
});
/* logs
"at index 0 the value is dog"
"at index 1 the value is cat"
"at index 2 the value is rat"
"at index 3 the value is snake"
"at index 4 the value is bird"
*/
The .map()
Array method also loops over an Array of values, but it’s used to return a new augmented Array of values based on the Array the map method was called on.
Below the values from the animals' Array are changed one by one to be a different value in a different Array.
const animals = ["dog", "cat", "rat", "snake", "bird"];
//
const pluralAnimals = animals.map(function (value) {
return value + "s";
});
console.log(pluralAnimals);
// logs ["dogs", "cats", "rats", "snakes", "birds"]
console.log(animals);
// logs ["dog", "cat", "rat", "snake", "bird"]
// animals Array did not change, map() created a new array of values
How do I find a substring in a string?
Answer:
If one means by “find” to verify that a substring is within another string, the String .includes()
method can be used.
const myString = "my favorite animal is a dog";
console.log(myString.includes("dog")); // logs true
If one means by “find” is locating the starting index of a substring within a string, that can be accomplished using the .indexOf()
method.
const myString = "my favorite animal is a dog";
console.log(myString.length); // logs 27,
// which means the string has 27 characters starting at a zero index
const indexOfDog = myString.indexOf("dog"); // find starting index of substring 'dog'
console.log(indexOfDog); // logs 24, meaning that the character "d" is at index 24
console.log(myString.at(24)); // logs "d", can use .at() to verify
// so we know that dog starts at index 24 and ends at 27
// .substring() will pull the string 'dog' from 'my favorite animal is a dog'
console.log(myString.substring(indexOfDog, indexOfDog + 3)); // logs 'dog'
The indexOf()
method searches a string for a substring and returns the first index where the substring begins.
What’s Next?
Make sure to checkout the learning path on JavaScript if you’re interested in learning JavaScript in the browser, or the learning path on Node.js if you want to use JavaScript on the server.
We’re always releasing new JavaScript courses on Frontend Masters. See the full list of related JavaScript courses below!