Netflix
Course Description
Build and compile modules from any language to the web in this beginner-friendly introduction to Web Assembly! In this course, you'll learn foundational, low-level programming such as binary, hexadecimal, and all about how memory works within computers. By the end, you'll have dived into array buffers, memory management, and unsigned integers, as well as created Web Assembly modules with AssemblyScript.
This course and others like it are available as part of our Frontend Masters video subscription.
Preview
CloseWhat They're Saying
Fantastic springboard to dive into the deep, deep pool that is WASM and low-level programming. I had dabbled before attempting to learn WASM and got lost. I'm very grateful that so many of my questions were answered in this course. Thank you so much, Jem!
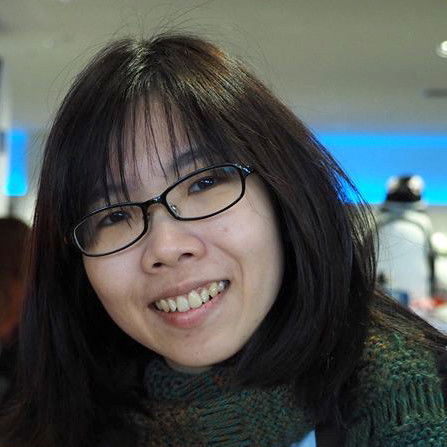
Lee Shu Yun
So excited to learn Web Assembly from one of my favorite people, Jem Young! It includes foundational, low-level programming, as well as array buffers, memory management, and modules. *rubs hands together*
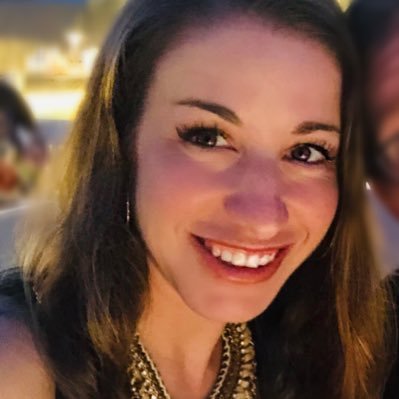
Sarah Drasner
sarah_edo
This "Web Assembly" course by Jem Young on Frontend Masters got me excited. 🔥
Did not know about AssemblyScript. Now I can start playing with WASM using Typescript-liked language. 🍬
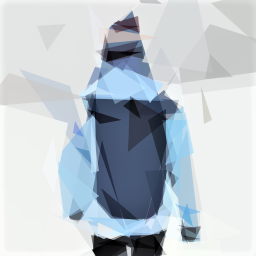
Jason Leung🔺
hangindev
Course Details
Published: April 7, 2021
Rating
Learning Paths
Topics
Learn Straight from the Experts Who Shape the Modern Web
Your Path to Senior Developer and Beyond
- 200+ In-depth courses
- 18 Learning Paths
- Industry Leading Experts
- Live Interactive Workshops
Table of Contents
Introduction
Section Duration: 18 minutes
- Jem Young is a Senior Software Engineer at Netflix and co-host of the Frontend Happy Hour podcast. The Web Assembly course will cover coding topics including binary, assembly, and higher-level languages like JavaScript.
- Jem explains that Web Assembly works in tandem with JavaScript because it allows languages like C and Rust to be compiled into a language the browser can understand. Questions about utilizing JavaScript APIs in Web Assembly and Web Assembly's similarities to other assembly languages are also covered in this segment.
Basics
Section Duration: 41 minutes
- Jem introduces the "bit" which is the smallest unit of information in computing. A group of eight bits is called a byte. Some computer architectures read bytes from right to left, or little endian. Others read bytes from left to right, or big endian.
- Jem explains hexadecimal is often referred to as machine code. Hexadecimal is an intermediate step between high-level programming languages and binary because it's concise enough for machines to interpret but easier than binary for programmers to read. In response to a student question, the process of accessing information from the register by a CPU is also explained in this segment.
- Jem demonstrates how the toString method in JavaScript can be passed an optional parameter called a radix which represents the base value for converting numbers to binary, decimal, or hexadecimal. The prefix "0x" is used to write hexadecimal values.
- Jem describes memory as a giant warehouse full of storage bins. Each bin has an address and can store anything as long as it fits within the bin. The main job of the operating system on a computer is to allocate and deallocate the use of these storage bins.
- Jem explains the two main numeric types in programming are floating point and integer. In JavaScript, all numbers are represented as 64-bit floating point numbers. Web Assembly uses 32-bit memory pointers.
Web Assembly
Section Duration: 33 minutes
- Jem explains Web Assembly runs in its own environment at a near native speed. It can be cached and runs much faster than JavaScript. The two file types in Web Assembly are .wasm, which is the actual assembly code in binary format, and .wat, which is the human readable textual representation of the code.
- Jem describes a module as the fundamental unit of code in Web Assembly. Within a module, functions are created and exported so they can be called by JavaScript. Function parameters are known as locals.
- Jem uses Web Assembly Studio to write a Hello World function. The function will take a 32-bit integer as input and return the input unmodified.
- Jem explains Web Assembly is a stack machine which means all the instructions are either pushed or popped off a stack in a linear fashion. Operation Codes, or OpCodes, are readable computer instructions for representing machine language instructions.
- Students are instructed to create a "minusone" function that takes a 32-bit integer and subtracts one from it.
- Jem demonstrates the solution to the Stack & OpCode exercise.
- Jem shares a more complex JavaScript code example that includes multiple conditions and demonstrates how that code would be written in Web Assembly. Since writing Web Assembly can be very verbose and difficult to visualize what values are on the stack, programmers will use a higher-level language like AssemblyScript which compiles to Web Assembly.
AssemblyScript
Section Duration: 1 hour, 51 minutes
- Jem walks through how to set up an AssemblyScript project with Visual Studio Code. AssemblyScript is a TypeScript-to-Web Assembly compiler. It provides both high-level language features like loops but also allows for low-level memory access.
- Jem writes a minusOne function using AssemblyScript. Function parameters and return values are typed. When the project is built, the wat and wasm files are generated in the build directory.
- Jem imports the minusOne function and calls it from the Node REPL. The AssemblyScript loader automatically imports the AssemblyScipt functions from the build directory.
- Jem codes a utility class that will use the fetch method to load a wasm file in the browser and return an instance for the compiled Web Assembly code. A fallback method is also created to for browsers that do not support Web Assembly's instantiateStreaming method.
- Jem uses the loader class to load the wasm file into the browser. In order for the file to load correctly, a content type of application/wasm must be set in the headers for the file's reponse. An Express.js server is also created to serve the application.
- Jem answers questions about the types shared between TypeScript and AssemblyScript, exposing wasm endpoints on the server, and what happens to the OpCodes in production.
- Jem explains that JavaScript functions can be imported and used in the Web Assembly modules. An imports object is passed as the second argument to the instantiate and instantiateStreaming methods.
- Jem demonstrates how to debug Web Assembly. The AssemblyScript source maps help identify the location of an exception within AssemblyScript code. Importing a custom log function into AssemblyScript is also covered in this segment.
- Jem codes a fizzbuzz example to demonstrate how Strings are handled in WebAssembly. Since WebAssembly only uses integers, AssemblyScript will allocate space for the String values but only return their location in memory. The AssemblyScript loader is imported to the project to add the necessary helper functions to read the String values.
- Jem modifies the WasmLoader class to return all the utility functions provided by the AssemblyScript loader. The __getString function is used to access the String value located at the memory position returned by the fizzbuzz function.
- Jem explains that memory in Web Assembly is a linear representation of 0's and 1's. JavaScript uses an ArrayBuffer to represent this binary data. A TypedArray will coerce the raw binary data in the ArrayBuffer into something useable by the application.
- Jem demonstrates how to use AssemblyScript to write directly to memory and access/update the memory from JavaScript. When the memory buffer is accessed from JavaScript, it is converted to a TypedArray and accessed through the location's index. JavaScript can use this same TypedArray to write values back into memory.
Wrapping Up
Section Duration: 18 minutes
- Jem uses a code example that checks if a number is prime to compare the performance of Web Assembly to JavaScript. In most cases Web Assembly has better performance, however, when dealing with complex data structures, programmers may find better performance in JavaScript or by using the Web Workers API.
- Jem concludes the course by sharing some resources and example applications that use Web Assembly. Questions about file watchers and supported programming languages are also answered in this segment.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops