Independent Consultant
Course Description
Gain speed and simplicity in writing web apps by taking a "vanilla" approach! See how you can build rich web apps and websites without depending on libraries or frameworks, using only the core JavaScript language. You'll learn fundamental concepts like the DOM API, finding and modifying elements in the DOM, and event handling. Build a real web app, "Coffee Masters," to demonstrate advanced topics like SPA routing, web components and shadow DOM, and reactive programming with proxies.
This course and others like it are available as part of our Frontend Masters video subscription.
Preview
CloseWhat They're Saying
This course has been paradigm shifting for me as an Angular/React dev for the last 6 years. Awesome content and great way of teaching
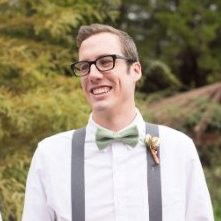
Kev Harvell
I just completed "Vanilla JS: You Might Not Need a Framework" by Max Firtman on Frontend Masters! Vanilla is a good flavor that stands the test of time.
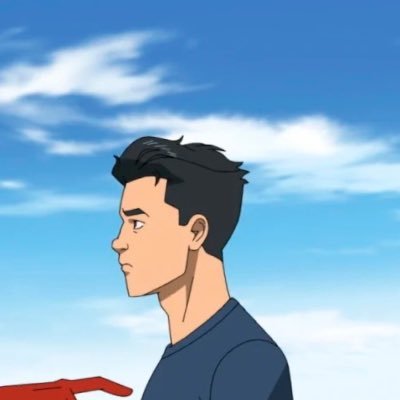
noah
noahh_ts
Just completed 'Vanilla JS: You Might Not Need a Framework' by Max Firtman on Frontend Masters! 🚀 Delighted to gain insights into how libraries like React work and what's happening under the hood. 💡💻
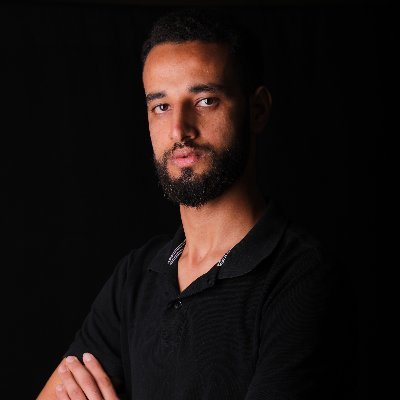
Azedine Ouhadou
OuhadouAzedine
Course Details
Published: July 6, 2023
Learning Paths
Learn Straight from the Experts Who Shape the Modern Web
Your Path to Senior Developer and Beyond
- 200+ In-depth courses
- 18 Learning Paths
- Industry Leading Experts
- Live Interactive Workshops
Table of Contents
Introduction
Section Duration: 8 minutes
- Maximiliano Firtman introduces the course by providing some personal and professional background. An overview of the material to be covered in this course is also provided in this segment.
Vanilla JavaScript
Section Duration: 1 hour, 6 minutes
- Maximiliano discusses the disadvantages of relying on only frameworks and the meaning of "Vanilla JavaScript." Vanilla JavaScript is the core language and browser APIs to create web apps without any additional libraries or frameworks added on top.
- Maximiliano discusses some reasoning for learning and use Vanilla JavaScript, including understanding library functions and changes, extending libraries with plugins, and mixing with other libraries. The main advantages and common fears of using Vanilla JavaScript is also covered in this segment.
- Maximiliano discusses the DOM API, where it's available, and walks through parts of a simple DOM example. Referencing DOM elements and actions that can be performed on elements are also discussed in this segment.
- Maximiliano discusses selecting elements from the DOM, types of returns when selecting elements, functions that return a single element, and functions that return multiple elements. Common pain points when returning elements are also discussed in this segment.
- Maximiliano uses JavaScript dot syntax to access properties mapped from HTML attributes and demonstrates reading or changing attributes of a DOM element. Listening to events and working with innerHTML are also covered in this segment.
- Maximiliano answers student questions regarding the appendChild() method and different types of Vanilla JS architecture patterns.
The DOM
Section Duration: 1 hour, 21 minutes
- Maximiliano discusses an overview of the contents of the course project, Coffee Masters, and demonstrates how to install and run the app as a PWA. A demonstration of what will be rendered on the DOM and what is considered valid HTML is also provided in this segment.
- Maximiliano demonstrates the difference between querySelector and querySelectorAll, including what data is returned. The DOM API is available in every DOM element, which allows more fine-tuned scoping of the querySelector and querySelectorAll methods.
- Maximiliano discusses adding scripts with the async or defer attributes to the course project. The defer attribute will defer the script execution until the parsing is finished while the async attribute will execute the script in tandem with the parsing operation.
- Maximiliano walks through setting up the main application script and discusses the difference between the Load event and DOMContentLoaded. The DOMContentLoaded event uses the DOM API, which is widely supported across browsers.
- Maximiliano discusses some possible DOM events that can be listened to, event naming patterns, and binding functions to events. Using onevent properties will only allow one function to be bound, while addEventListener allows binding multiple event handlers.
- Maximiliano discusses attaching multiple event handlers per event/object and dispatching custom events. Student questions regarding removing event listeners with the parent element, if events with multiple functions fire synchronously, and if there is a limit to the number of event listeners are also covered in this segment.
- Maximiliano provides some shorthand methods to help reduce the amount of code needed by creating aliases.
- Maximiliano walks through creating the initial services for the coffee masters application to access the menu eventually. Updating the script to a module to allow imports is also covered in this segment.
- Maximiliano demonstrates importing and utilizing the application's previously created Store and API modules. Calling the API to load the menu data is also covered in this segment.
Routing
Section Duration: 46 minutes
- Maximiliano discusses navigating between pages using the DOM for single and multi-page applications. The History API will be used to push new entries to the navigation history.
- Maximiliano walks through creating a router, managing which navigations get stored in History, and handling deep linking. The created router will be specific to the course application but can be made reusable by adding abstractions.
- Maximiliano answers student questions regarding using a function for loadData and not Router, how arrow functions handle bindings, and if the menu isn't modular because it uses app as a dependency.
- Maximiliano demonstrates updating the DOM content based on the URL by utilizing a switch case and createElement. The page content is targeted using children instead of childNodes to avoid selecting empty text and new text lines.
- Maximiliano demonstrates creating a dynamic page route to handle navigating to individual product detail pages. Handling URL changes by adding an event listener for the popstate is also covered in this segment.
Web Components
Section Duration: 50 minutes
- Maximiliano briefly discusses an overview of web components and their associated set of standards, including, custom elements, HTML templates, shadow DOM, and declarative shadow DOM. Custom element attributes, lifecycle, slots, and custom builtins are covered in this segment.
- Maximiliano discusses creating markup containing HTML content that can be reused, rendered, and dynamically modified. CSS style declarations made in templates will apply to the entire document by default.
- Maximiliano discusses utilizing the shadow DOM for more control over the styling and functionality of custom elements. If a template element is on a shadow DOM, the style declarations will only apply to the template.
- Maximiliano briefly discusses the declarative shadow DOM which allows the shadow DOM to be defined directly in the HTML markup. Alternatives for where to define custom element CSS including CSSOM APIs, script tags, link tags, and external CSS files are also discussed in this segment.
- Maximiliano creates the Menu, Details, and Order web components and exports them to app.js so the browser can recognize and execute the modules. Applying the web components to the DOM is also covered in this segment.
- Maximiliano walks through loading the previously created templates and rendering them to the DOM. Templates must be cloned before they can be injected into the DOM.
- Maximiliano demonstrates creating, attaching, and defining the mode of a shadow DOM. A student's question regarding why the template isn't placed in a JavaScript file and then rendered is also covered in this segment.
- Maximiliano walks through loading and applying stylesheets to web components.
Reactive Programming with Proxies
Section Duration: 1 hour, 25 minutes
- Maximiliano discusses creating a proxy to allow modifications to operations performed on the wrapped object. A proxy can be used for validation, data binding, and reactive programming. Proxy handlers and traps are also covered in this segment.
- Maximiliano walks through creating an event listener that listens for and renders changes to the coffee master's menu. A student's question regarding if the shadow DOM allows the use of local IDs is also covered in this segment.
- Maximiliano implements the ProductItem component and demonstrates an alternate method of passing in the product as an attribute. Updating the event listener to not link the add to cart button to the details page is also covered in this segment.
- Maximiliano walks through the code for the DetailsPage component and updates the product ID to the correct variable name.
- Maximiliano demonstrates implementing a new Order.js service to allow users to add products to the cart. Handling adding multiple of the same item is also covered in this segment.
- Maximiliano walks through and implements the code for the CartItem component to display the items that have been added to the cart. A student's question regarding why importing the custom element renders the content is also covered in this segment.
- Maximiliano demonstrates binding the order form's input data to a user object. Clearing out the form when the order is placed and updating the home page to render when navigated to are also covered in this segment.
- Maximiliano answers students' questions regarding the order page not loading when directly navigated to and how to decide when to use vanilla JavaScript or a library. Some helpful resources are also provided in this segment.
Wrapping Up
Section Duration: 14 minutes
- Maximiliano wraps up the course by reviewing the covered material and common fears of using vanilla JavaScript. The CEO of Frontend Masters, Marc Gabanski, also shares some thoughts on coding in Vanilla JavaScript.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops