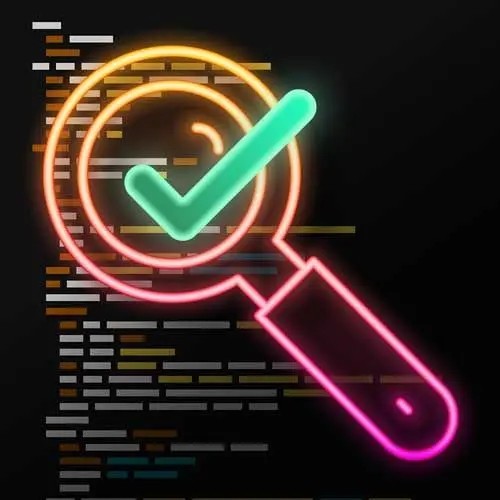
Lesson Description
The "Mock Service Worker" Lesson is part of the full, Testing Fundamentals course featured in this preview video. Here's what you'd learn in this lesson:
Steve introduces Mock Service Worker. The JavaScript Service Worker API can intercept network requests. The Mock Service Worker library leverages this capability, allowing mock network requests to be created and used in place of real backend APIs.
Transcript from the "Mock Service Worker" Lesson
[00:00:00]
>> Steve Kinney: We talked a lot about mocking and stubbing and spying, and even in this case there is the what about the backend, right? And there is a bunch of really great tooling to help you solve for this issue. One of them, and I'll kinda bring up the website and I'll show you some example code is this App called Mock Service Worker.
[00:00:23]
And all this really does is you can use it in development in the browser. You can also use it in your tests. I'll show you an example in a second of the app that I have it working with where basically a service worker is something for progressive web apps.
[00:00:39]
But like It allows offline use of a web app and so on and so forth. What a service worker does in real production practice is the stands between you and the network. So if you're offline, it can intercept a network request and fill it in and then when you're online sync back up, a service worker intercepts a network request.
[00:00:59]
That's interesting, right? So what you can do with a service worker, and what mock service worker will do for you, is you can basically say, hey, here's a bunch of endpoints where I don't want you to hit the server, right? What I want you to do is just return this object back to me, right?
[00:01:19]
So you can basically mock the backend, right, and have it just respond with known good objects so you can continue your testing and totally separate yourself from the backend. This is useful for a few ways. One, you test one super fast because it's not hitting the real network.
[00:01:36]
Two, they pass because it's not hitting the real network, right. Three is that you can start working on APIs before they're done, right? Cuz you can mock out just one or two given APIs, right? So if we look at that task list that we had before, so check it out.
[00:01:52]
This is like, here we can just basically put in a bunch of handlers. In this case, we can do something like,
>> Steve Kinney: Or something like this, and then we can have it basically,
>> Steve Kinney: You can say HTTP response and then that takes an async request, like a callback.
[00:02:32]
It can say like, hey,
>> Steve Kinney: I have two fake tasks in this JSON file. So okay, whenever somebody goes to hit / does a get request /api/tasks, block it hitting the network. Ignore that, right? And instead, what I want you to do is like,
>> Steve Kinney: Right? I guess I don't need the request on that one, per se.
[00:03:11]
And what this will do, and this will work in your unit tests. You can actually spin it up in the browser. As well so it'll work in Playwright too, but there's actually something even better for Playwright. We'll talk about it in a second. But now in your unit test, you can basically pull in this server and have it basically mock out all network requests.
[00:03:31]
So now instead of hitting the real API, you can have it hit just some standard responses, right? And you can do a whole bunch of stuff with like put and patch and get and stuff like that. But what's really cool is you can use different responses for different tests, right?
[00:03:48]
And so like cool, have like an admin account set of responses, right. And so instead of hitting the real network you can, kind of like, fill in again the tasks is just two fake tasks, right? And so now it's never gonna hit the real network. But you're not, like, stubbing weird stuff in your code.
[00:04:07]
You're only the boundaries of your app is it was leaving your app to go hit the server, right? This is stopping it at the very like, borders of your app and saying, like, yeah, here's what you could have expected to get back from the server, right? Wicked fast, right?
[00:04:23]
Able to change based on, like, what you want to test at that given moment. Maybe I want bad data. Maybe I want a 404, right? You can kinda do all of those things and just like, if you've ever written an Express App, or even if you haven't, like, going back into this handlers, right?
[00:04:39]
Like, it's a get request to this route. Other than this, like, thing that was just gonna do the rest of the, like, HTTP response stuff, we're just responding back with JSON of what we wish that API would have given us. Great for endpoints you don't have yet, too.
[00:04:52]
The nice part is you're not doing weird stuff to your code, either. You're just saying cuz you never controlled what the server did in your code, unless you are also testing the server, at which point you do own that as well. But also great for developing things that maybe you don't like the APIs that aren't ready yet.
[00:05:13]
My least favorite thing as a front end engineer Is every other team takes a little longer than expected, but the deadline didn't change [LAUGH]. So if I wait for the API to be done, I ain't got enough time to build the thing. So for instance, we could do like a post as well.
[00:05:31]
Like in this one we want the request object. So that will have been the request from the quote unquote client. At this point, yeah, we're just doing like, that one, I just want the title,
>> Steve Kinney: Here as well and then we'll just say, I've got this fake create task function that does, I wrote the server, so I happen to know what the server does too.
[00:06:01]
Granted, I don't use a real database or anything. So then we'll say,
>> Steve Kinney: Title and I could push that onto the array, too, if I wanted to. I can make it work as close to a real server as possible. Now, the problem is, generally speaking, you do need to like, at what point are you simulating the server so much that you've just rewritten the server, unclear, right?
[00:06:33]
And so that's why I'm not pushing it out. I'm not pretending like it's a real server, too much. But now you can theoretically post a new task to it, and this will then send back the response that I would have gotten from the server, right? And you just kinda get that.
[00:06:48]
So now you can use that in your unit tests, right? Cuz now it'll intercept those requests too. From fetch and we'll just respond back with it immediately. You can use it in development. The one thing that we're using a little bit in Playwright, which is,
>> Steve Kinney: If you've ever gone to the network tab in Chrome, a lot of times for an API request, it would be like copy HAR.
[00:07:19]
You can copy like the full request and response. And basically, you can tell Playwright like, hey, just like when you hit this endpoint, respond with this HAR file. But what's even cooler Is right here where you can say, hey, give me the request that I would've gotten for this endpoint.
[00:07:45]
But if you are able to hit the network, record it. So now what you can do is you can fire up Playwright, you can start writing the test against real production server or staging or whatever, record all the network requests, have it store them and then play everything back.
[00:08:03]
And so if, even if you did like a, Hey, get me all the tasks, post a task, get all the tasks again, it keeps the order right? And so you will get like a recording of all the network requests. So now, when we think about what we saw before, you can basically turn on this recording mode.
[00:08:18]
And so what we're doing with it is we, there's a few bad things that I, mistakes that I made early days. I forgot to think about dark mode ever. That was a three months of my life I'll never get back. I wasn't as great as I should have been on responsive design.
[00:08:34]
Do you know how much it stresses me out to retrofit in a mobile view? And so what we're basically doing is we have it set up in our cloud thing, we're setting up the open source side, which is fire up a Playwright browser at all the different viewpoints, record all the network requests for a bunch of different cases, and then take screenshots, right?
[00:08:56]
And that way if we accidentally regress the tablet view while working on the mobile view, I've got visual evidence, right? And we can kind of see. And this is like, again, right, it's about how do we do this big refactor without like breaking stuff, which is to first surround it in tests, right, to the point where we feel confident, right?
[00:09:21]
Yeah, we might break something, but the ideas that we see before our customers do.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops