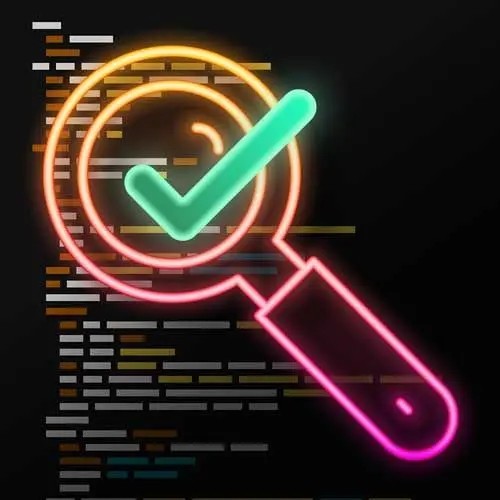
Lesson Description
The "Introduction" Lesson is part of the full, Testing Fundamentals course featured in this preview video. Here's what you'd learn in this lesson:
Steve Kinney begins the course by sharing the benefits of testing and providing the code for a basic test. Prerequisites are also discussed. Students do not need any previous testing experience but should be comfortable with JavaScript and NPM.
Transcript from the "Introduction" Lesson
[00:00:00]
>> Steve Kinney: Hi my name is Steve, and today we're gonna talk about testing software. I've got a few basic tenants around that, but I kind of wanna start with of the kind of four major beliefs that I have about testing, one that I kind of want to call it in the very beginning.
[00:00:16]
Don't worry we'll talk about all four. One is that writing tests isn't all that hard despite what you believe, right? Writing tests is super easy that said, not all code is easy to test, right? And so we are gonna talk about testing software, right? And one of things I will kinda mention a few times, which is you're always testing software one way or the other, right?
[00:00:39]
Either you are testing it or your users are testing it, right? In production, paging you at night or whatever, filing bug reports and making your life hard, or you're testing it and you're either doing it manually, or you've got some kind of automated system to make sure everything works, right?
[00:00:55]
And so, our goal is to get to that automated system that makes stuff work right? And some of that involves the actual writing of tests. Some of that involves how do we think about our code in a way that makes it easier to test? Because sometimes if a piece of code is hard to test, it's not necessarily that you need to get better at testing.
[00:01:15]
It is maybe that you need to start pulling apart the code. So there are two pieces of this and I kind of want to make sure we think about both of them equally, cuz the answer to making easy to test code is somewhere kind of balancing those two different pieces as well.
[00:01:30]
So in this course, we're going to definitely write tests, of course. We're going to look at how to make some code that already exists a little bit easier to test right? In some cases, we will write some tests and then we'll go back, change the code and see ways that we could have made our life simpler after the fact, which is always very validating.
[00:01:48]
And then sometimes we're gonna look at how to test code that's hard to test. Because in a lot of cases, yes, you could learn how to write the most testable code in the world, but you're not always testing your code, right? There's sometimes where it's just code you inherited sometimes it's a library.
[00:02:01]
Sometimes it's the worst developer that we all despise the most, which is us three months ago, right? Not that any of us would ever do that, but yeah, so as we kind of jump into this, what are the prerequisites and what do you need to know? I am not going to assume that you've ever written a test before in your life, right?
[00:02:20]
If you have that's probably helpful. I'm not going to assume that you are familiar with any particular framework, just to make a point, there are some react components and there are some Svelte components. But we are mostly focusing on the testing piece. We'll look at how to test those things, because, generally speaking, it is not.
[00:02:40]
Uncommon that you might find one of these frameworks in your day-to-day life yes, Mark?
>> Mark: At what point in your journey of learning to program would you say now is the time to focus on testing?
>> Steve Kinney: I would say like about the time that you can kind of get one or two functions up and running, right?
[00:02:59]
Like the longer you put it off, the larger mess you have to go clean up, right? So I think there is nothing particularly difficult about testing. That means if you can JavaScript, if you can get a script and you see something in the terminal or on the page, we're probably at a good enough place to start talking about the testing piece, right?
[00:03:20]
There is always depending on how big the thing you're working on is, right? If it's just one little script, sometimes, you know what? Staring at those console logs is probably good enough, right? But generally speaking, the point where you know that you need tests, and by that point, knowing how to test is probably, you probably need to learn that beforehand.
[00:03:38]
The moment you know you need to test is when you get that piece of existential dread in your heart. When you go to change a piece of code and you do not know what else you are going to break or what regressions you're introducing, right? The sole goal of testing is I'll make the point on another slide, and then I'll act like it's the first time I've ever seen it is to take that fear when you change existing code.
[00:04:01]
And try to minimize it as much as possible to the point where you feel confident as much as you can in software. I'm always surprised by edge cases sometimes. But to get to the point where you can go ahead, change code, and feel mostly confident that you didn't mess something else up as you tried to fix the initial thing.
[00:04:16]
That is like the bar that we seek to reach. And we'll make some jokes about that in a little bit. But that is effectively the kind of why do we test so that we can sleep at night, right? Why do we test so that we can open up a PR and not worry that all of our co-workers are gonna hate us in a week cuz we took down production, right?
[00:04:34]
That is the ultimate goal. Every other perfectionist tendency in your body needs to chill out for a second. That is where we seek to go. And so to answer Mark's question from earlier, or I assume the chat's question, the moment that you feel anxiety that there are too many things going on for me to hold them all in my head.
[00:04:54]
And I'd like something to automatically kick all of the tires on this application, make sure I didn't break anything that's the moment that testing becomes super useful right? And we will talk a little bit about the idea of test-driven development right? This idea that you write the tests first.
[00:05:11]
And that kind of goes to the previous slide we talked about before, which is that writing tests isn't hard. Testing hard-to-test code is hard. The idea when we talk about test development when we look at it is that, if you write the test first and make it pass, it is very hard to have written code that is hard to test, right?
[00:05:27]
And sometimes in that, trying to figure out a solution, saying, hey, I would like this very simple thing to be true. Cool, move on to the next step. Like we were talking about in the introduction, that little dopamine hit as you go kinda along of red, now it's green.
[00:05:43]
Now, I do the next thing and it's not working, now it's working. Those things I think help as we even try to think about and rationalize our code. So I would say the moment that you can do some basic JavaScript, it is probably a good time to learn how to test because all you're doing is automatically validating that your code works the way you think it works.
[00:06:04]
And the moment that it's taking more time to constantly check over and over and over again than it's worth, it's time to start testing. Cool and we'll talk about what we're going to do today, all that other stuff. But first, I want to take a moment and just look at the world's simplest test to kind of make my point.
[00:06:21]
This is a test this has all the components of a test in it, right? Which at the end of the day, our tests are just JavaScript, right? To go to that previous point of if you know JavaScript, you can probably tackle testing, which is we've got this function, it's called it.
[00:06:37]
You can also call it test it takes a string that describes what it is. And then there's a function where you just make some statements about the world, right? This one will almost always pass can't think of a world where it won't. But that is the core function of a test now, obviously, this isn't doing much.
[00:06:59]
And so slowly we begin to build it up, right? Which is, we could write a function, we could hope that the results of that function are what we expect. And this is a super simple test, but those super simple things are sometimes the things that we miss, right?
[00:07:12]
And so we can validate that if we're changing the way that addition works for some reason, right? Or, let's say we had a multiplication function. At first it's just using the multiplication symbol, but then we wanna get really fancy and just recursively call, add that number of times.
[00:07:25]
We can verify that all these things work. And that's all we're really doing as a test, is we have got a test run that goes through, takes all these statements, runs all of them. And makes sure that everything the world works the way we think everything the world should work, right?
[00:07:39]
And that is all our tests are doing at the end of the day. And so, like I said before, the end goal of testing is to get rid of that fear. It's not about a 100 percent test coverage. It's not about any kind of badge of honor. It is simply to feel confident with your own code and the fact that you can change it and know that you probably didn't break something.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops