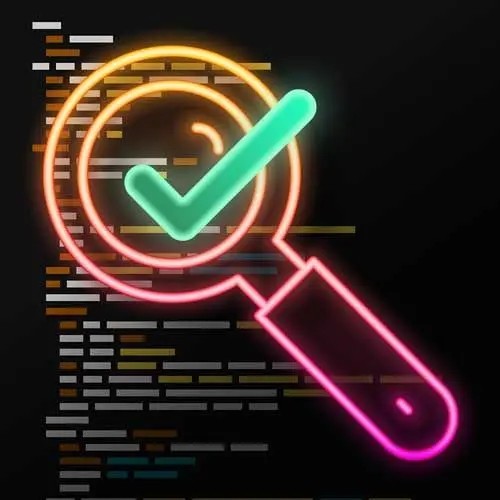
Check out a free preview of the full Testing Fundamentals course
The "Course Overview" Lesson is part of the full, Testing Fundamentals course featured in this preview video. Here's what you'd learn in this lesson:
Steve provides links to the course website and the GitHub repository containing examples used throughout the course. Students should clone the repo and run `npm install` in the root directory. Vitest, the primary testing tool used in this course, is introduced, and some additional testing tools are discussed.
Transcript from the "Course Overview" Lesson
[00:00:00]
>> Steve Kinney: First one is we're going to write some super simple tests, right? Some of them you'll be like, why am I testing this? Because we're writing tests. We're getting used to, we're getting the blood flowing, right? You will not be surprised to learn that addition works the way that you think it does, right?
[00:00:14]
But it is mostly to get us comfortable and get us the momentum of writing tests and then I will slowly turn up the heat on you. And you won't even notice it. It'll be great. Things will escalate, it'll be great. But then it is one thing to test that things work the way that we think it's gonna work in a known situation where they should work.
[00:00:31]
Yeah, you don't wanna break that. But then also understanding of dude, we handle all of the weird ways that are code can change. We know the problem we're trying to solve. We sit there, we write some code. Maybe we didn't write any tests, but maybe we pulled it up in the browser, clicked all the buttons.
[00:00:50]
Everything did things the way that we expected, right? Until we put it into production and a user comes along, and they decide that their first name has an emoji in it, right? Or what have you. Or all of the weird ways that things can kind of happen where, that value we thought we would get is actually undefined, right?
[00:01:11]
And thinking about okay, so how do we use our test to help us? Sometimes it's not necessarily the test is simply gonna throw those edge cases in there. But the thinking about our test and using the test to make sure that we've got all those cases covered, right?
[00:01:24]
That's kind of where we balance the how do we write code and how do we write tests and how to relate with each other. We'll play around with it there as well. There are some little tricks that will make your life easier. They are the tricks that, are you going to use them every single day?
[00:01:39]
You're probably not going to use them every single day. Do I expect you to memorize them? I don't. Do I want you to just kind of put like a landmark in your brain, in your neural pathways, so that like when you find yourself struggling with a problem, you at least remember that there can be a better way and go and look it up, right?
[00:01:56]
That is the kind of goal there as well. Because like I said, they are things that make my life very easy when I use them once every six months. But they're probably not everyday things, Mark.
>> Speaker 1: CJ online just said something I've noticed with Tess is when there's too much mocking, there can be a false positive, meaning things pass that shouldn't.
[00:02:18]
>> Steve Kinney: Yes.
>> Speaker 1: And then someone plus one'd.
>> Steve Kinney: Yeah, so we'll talk about this. There's this idea of mocking, let's say you're hitting an API, right? You probably don't want to hit that API in every single test, especially if you're running a test multiple times a day. Also, it's really hard to get the external server to be exactly the way you want it.
[00:02:38]
So we might use this thing called a mock which is like, pretend this is the real world, right? And it kind of like the intellectual exercise kind of works here, which is if you start making more and more of the real world make believe, you get to a point where you can make the entire world make believe, and your test will totally pass, and they're totally worthless at the same time, right?
[00:03:00]
So, we will look at how to do that when it's appropriate, right? We'll do it for a code that maybe needs it. And we'll talk a little bit of how to refactor some of that code so that you don't need it as well, right? Because if you try to make too much of the real-world imaginary, you end up living in a fantasy land.
[00:03:17]
And yeah, your test pass but again, if you have not, lower that existential dread of making changes to your code. It does not matter if you have 3,000 tests and 100% test coverage. It is possible to get to 100% test coverage and not remove any of that existential dread, right?
[00:03:35]
You can game that number real easily and still have no value. And that's why we're not aiming for the sheer number of tests, the sheer amount of test coverage. It is, do you feel comfortable refactoring the code? And if you've mapped out way too much and you don't feel comfortable refactoring your code, then we know that we haven't been successful.
[00:03:52]
Yeah, cool. And most of us are JavaScript engineers. And this is a website called frontendmasters.com. So I can make some assumptions that maybe not all of you, but some of you have once in a time dabbled in the browser. And so just being able to say two plus two equals four is probably you hate when you leave something like this and you go to a moment like, DOM node shows up, you're like, I don't know what to do here.
[00:04:20]
So, we will make sure that we are ready and able to deal with things in the DOM. And we'll do it from multiple angles, from one just grabbing DOM nodes and playing with them, and then it also can we spin up a browser and point it at our app and poke buttons for us, right?
[00:04:35]
Cuz it's all about that. Automating the kicking the tires on things so that we can make changes and feel good about it. And then we'll talk about the stuff that we don't control, right? Whether it's external network with hitting an API, nobody likes the world where all of a sudden your tests fail because a backend engineer introduced a bug, right?
[00:04:56]
Never happens. Or because you got rate limited by an external API or something along those lines. So we'll learn how to make sure you're not testing other people's code, because if other people break other people's code, now your tests fail and now you turn them off. The moment anything is flaky, you know what you're gonna do, you're just gonna delete that test.
[00:05:14]
So we'll deal with that kind of stuff as well. And like I said, we will drive this from the browser as well. That's probably a larger topic, its own right, but let's do the initial versions of getting our heads wrapped around it as well. So we have this website, and it has all of my notes, which I did a word count was like tens of thousands of words of notes.
[00:05:37]
That is mostly if I took the time to write them, might have them up there for you. So there are things where it's like, I wanna see that kind of code step by step. Or a lot of times there's a lot of stuff that we're not including that are kind of further reading or stuff along those lines.
[00:05:51]
That is all included there as well. Even ancillary topics that I might, in our time together only get a chance to Kind of briefly touch upon. Sometimes there is more depth in there as well. And linked from that site, we've got a repository with a bunch of little examples that I can kind of kick the tires on and play around with.
[00:06:11]
And like I said, if we need to whip up something new for a particular question you have, we'll do it. But just to make sure that we spend more time testing code than watching me write very simple code. So we've got a bunch of examples to inspire us for tests and stuff along those lines, cool.
[00:06:30]
Lastly, just in terms of tooling, there are a lot of test runners out there. There's Jest, there's Mocha, and Chai, which are related, as you can guess. Jasmine, there are a whole bunch of them. Most of them are actually the same under the hood, right? Or at least we could write the same test in about three different frameworks, and the COVID run across the altar with maybe just where we're importing it from, or one little change.
[00:06:57]
So we're gonna use this one called Vitest. It is a companion to V, which is also works with react and angular and spelt and view. That said, if you use jest at work, all of this stuff applies. Occasionally, I'll point out the minor difference between the two, but in most cases it doesn't really matter, right?
[00:07:16]
There might be a tiny little syntax change between one or the other, but most of them are based on the same tooling. The wrappers around the same common libraries anyway.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops