Codesmith
Course Description
In this course you’ll go under the hood of Node.js in two contrasting ways - by understanding how to develop servers from intuitive first-principles (HTTP, TCP/IP, Ports, Loopback, SSH) and by understanding the JavaScript features that make up Node (the event loop, streams, buffers, asynchronicity, prototypes). Taking these two approaches together you will develop a deeper understanding of servers, Node, and JavaScript itself!
This course and others like it are available as part of our Frontend Masters video subscription.
Preview
CloseWhat They're Saying
Will Sentance courses on Frontend Masters are the best I've taken. Great use of graphical representations for explaining core basic concepts that we usually don’t understand clearly.
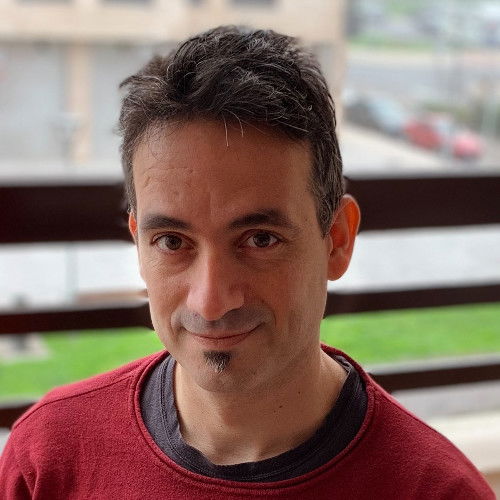
Ricardo Crespo
Ricardo53365192
I finished Frontend Masters "The Hard Parts of Servers & Node.js" taught by Will Sentance! It was a comprehensive dive into server-side concepts, equipping me with a deeper Node.js understanding in just the right detail.
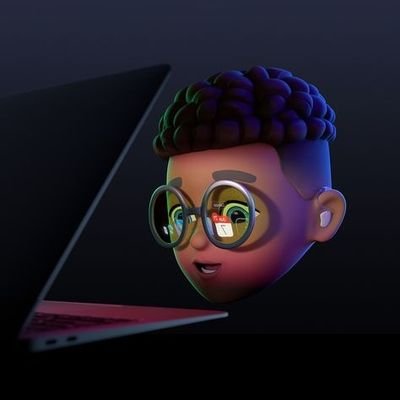
Hareesh Bhittam
hareesh_bhittam
Course Details
Published: May 24, 2019
Learning Paths
Learn Straight from the Experts Who Shape the Modern Web
Your Path to Senior Developer and Beyond
- 200+ In-depth courses
- 18 Learning Paths
- Industry Leading Experts
- Live Interactive Workshops
Table of Contents
Introduction
Section Duration: 38 minutes
- Will Sentance introduces the course on Servers and Node.js by explaining why Node is such a powerful piece of technology.
- Will diagrams client-server interactions in a web application to highlight the area where Node operates.
- Will explains the role of JavaScript and Node within a server and differentiates between the two roles.
- Will walks line by line through the execution of code in JavaScript to demonstrate what is going on under the hood.
- Will discusses how Node is able to talk with the inner features of the server computer to enable network communication.
Using Node APIs
Section Duration: 53 minutes
- Will explains what is occurring within the server when using Node to open a network channel that listens for incoming requests.
- Will explores the inner process of using Node's createServer method to auto-run a specified JavaScript function.
- Will analyzes Node's role in running a function, including auto-running the function at the right time and inserting arguments.
- Will discusses what occurs when HTTP messages arrive in Node and how Node sets up two JavaScript objects automatically, one for the received information and one to send back to the client.
- Will summarizes the timeline of events in JavaScript and Node from previous lessons and reviews each part of the system's role in creating a server.
- Will fields questions about semicolon usage, port numbers, errors, the end function, and the timing of auto-running functions.
- After summarizing the functionality created by the server creation code, Will gives a real-world example of how Node is being used in relation to server requests and responses.
- Will discusses the goals of the course and then explains what the job of Express and other middleware is within Node.
Node with HTTP
Section Duration: 1 hour, 2 minutes
- Will walks through the execution of JavaScript code that sets up the server to receive HTTP requests.
- Will demonstrates how the HTTP message arrives in Node and is then parsed to acquire needed information from the request message.
- Will runs through the process of filling in the auto-run function's parameters and executing it, and then using end to trigger Node to send the response.
- Will fields questions about data handling, where to declare get/post methods, errors during JavaScript execution, and the request method.
- Will discusses the headers for the response in HTTP format, and mentions a few additional items the response can include.
- Will explains the value of using the built-in require method to access certain features in Node.
- Will describes how to write code in JavaScript, load the code into Node, and then run the code. Nodemon is introduced as well.
- Will explains how cloud development allows developers to run Node on external computers. Will also explains the role of devops professionals.
- Will describes how to run JavaScript code and Node apps locally for the purposes of testing, using the localhost domain.
- Will gives instructions for running Node with JavaScript code locally and instructs students to complete the exercises using pair programing.
Events & Error Handling
Section Duration: 33 minutes
- Will explains how Node's background HTTP features determine which function to auto-run, specifically for errors instead of requests.
- Will discusses the event system in Node by going over how to edit a server after setup to respond to certain events differently or change ports.
- Will walks through lines of JavaScript code to modify the server by declaring functions to run on request and client error events.
- Will demonstrates how the server reacts to a client error and how Node auto-runs the function that was specified to be run.
File System
Section Duration: 40 minutes
- Will plans out a way to use the file system API, fs, to save the tweets into the file system and then apply modifications using JavaScript.
- Will explains location in the terminal, the benefits of using json to store data, and then diagrams the process of storing and using json data.
- Will highlights the process of using fs to read from the computer's file system to modify the data, explaining how JavaScript is used to trigger Node functionality. Threads are also discussed.
- While going through each line of execution for modifying the data acquired through fs.readFile, Will describes how JavaScript's call stack works along with what data is stored in local memory.
- Will fields questions about the call stack, the buffer data format, imports, the auto-created error object, and parsing file contents.
Streams
Section Duration: 43 minutes
- Will introduces Node streams by presenting a use case where a group of data is broken up and worked on in parallel.
- Will creates a diagram of a Node stream and explains how streams processes large-scale data in a more efficient method.
- Will walks through the execution of code that uses readStream to set up a stream to a JSON file and set a function for Node to auto-run on each batch of data.
- Will demonstrates how Node processes data in batches, which involves auto-running the specified function on a batch and adding calls for subsequent batches to a queue.
- Will runs through Node's event loop process, where the callback queue is checked and a call to the auto-run function is made on each batch at the correct time.
- After discussing the callback queue and event loop, Will fields questions about sharing the queue, the final batch of data, buffers, queue timing, and handling incomplete data.
Asynchronicity in Node
Section Duration: 41 minutes
- Will emphasises the importance of knowing in what order Node is executing queued operations and why, and sets up the section to examine how Node's event loop, stacks, and queues work.
- Will introduces the timer queue by beginning to walk through code that demonstrates how the event loop prioritizes certain queues over others.
- Will introduces the IO queue by further explaining the event loop's prioritization system, diagramming the addition of calls to the timer and IO queues.
- Will introduces the check queue and demonstrates how setImmediate adds function calls to the check queue, as well as in what order queued elements will be run.
- Will completes the event loop's processing of the various queues to demonstrate the order in which each queue is checked.
- Will introduces the microtask and close queues, and explains when the items within will run compared to those in other queues.
- Will summarizes Node's event loop's priority rules for automatic execution of JavaScript code within the queue system.
- Will fields student questions about require, the structure of the code that makes up the event loop, and the queue data structure.
Wrapping Up
Section Duration: 2 minutes
- Will concludes the course by summarizing Node's process and offering ideas about what to learn next.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops