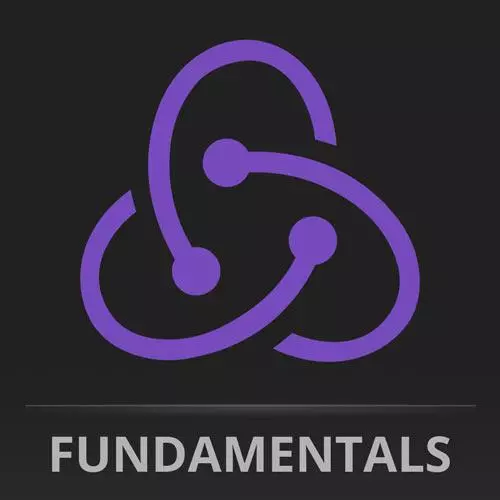
Check out a free preview of the full Redux Fundamentals (feat. React) course
The "Slice Actions & Creating Actions" Lesson is part of the full, Redux Fundamentals (feat. React) course featured in this preview video. Here's what you'd learn in this lesson:
Steve demonstrates how to add actions and actionCreators to state slices using hooks. The ReduxToolkit actions use a different syntax from Redux and automatically generates the action type based on the name field and the reducer action.
Transcript from the "Slice Actions & Creating Actions" Lesson
[00:00:00]
>> Now let's get some actions working and we'll go back to using hooks cuz we just use Connect for a little while. And our actions are hooked onto that slice ready for us to use as well. So we'll go in here and we'll take our create human task.
[00:00:19]
And now we've got dispatch, so I have this one that says like, implement me. Let's go ahead we'll just say humanSlice or I guess a call it a singular human, let's stick with it for now, .s.actions where it's got ad, right? And as expecting a name as we saw before, cuz it's gonna add it on there, but it automatically created the action crater on our behalf based on the reducer action.
[00:00:47]
So it's like cool, if you've got something in your reducer that knows about adding humans, you probably need an action for adding the humans. And the slice basically automatically creates that on our behalf, and we go ahead and we use it. Let's make sure that this works. You can see we can add more people.
[00:01:08]
And they're on there as well. If you look, this is something we talked about the very beginning of our time together. Which is it uses a different syntax than the all capital letters because it's abstracting it with a object key, it's actually gonna use human/ad when we wire up the task is gonna be task/ad.
[00:01:29]
It's gonna automatically generate the action types on our behalf based on the name field that we gave that slice and then the reducer action. So is those two things put together creates the action type automatically for us in this case. And so doing the same thing for tasks is somewhat similar.
[00:01:51]
So we'll go to create task, and we've got that other implement me here. And this time we're just gonna say taskSlice.actions.add, we're dispatching that with this case with the title. And so now we can go ahead and we can say what are the plans? File the TPS reports.
[00:02:16]
Let's see actions blowing up. It's not a function. I didn't put it in the reducers key when I was typing before. So we have reducers, now we can go ahead and we can use it. Yeah, and we have both kinda in place. You can see task that ad, we have everything we need right there.
[00:02:49]
So it's making a lot of the boiler plate a lot easier for us. Now here's the problem. What happens if we wanna have an action that isn't immediately generated by this for us, so we have a way to go about doing that as well. Let's start with the one that's automatically generated and then we'll see if we want to be able to change it on our behalf as well.
[00:03:19]
So, we can say we've got this, Let's add the ability to toggle one of them on and off. And so, we'll go to the tasks, and we'll say toggle, which is gonna be that done or undone check box that we had. Toggle is gonna take the state in the action as well.
[00:03:39]
And by default, it simply gives you whatever was passed in as the one argument as the payload, right? And so if you wanna create a little bit of a different syntax for that you get to generate your own actions. But let's go ahead and let's just say, a given task is gonna be state.find, task is gonna based on this as we saw before taskid equals, equals, equals action.payload.taskid.
[00:04:08]
But then we also maybe wanna get whether the box is checked or unchecked. So we're gonna need that as well in here. So once we find that task we'll say task.completed equals action.payload completed. So now we can go in to our individual tasks and find other implement me.
[00:04:33]
And we could dispatch taskid which we're getting passed into the prop and then completed is gonna be event.target.checked which will either be true or false. So, make sure that works. Now we can check them, we gotta refresh it. Let's see misspell constant. I gotta actually use the action creator.
[00:05:05]
So we'll say tasksSlice.actions.toggle. We'll pass it in like that and that's feels verbose, right? It feels like I would love to just give the taskid as the first argument and whether or not it's completed as the second argument. Do you feel that is that something that you're spiritually thinking coz if you are, we can make that happen.
[00:05:30]
We're just gonna have to create an action that's not auto generated for us. And we can absolutely do that. But let's just make sure our initial version works. All right now it works and you can see that the correct actions are getting toggled. Let's say that we wanted to just create our own action creator.
[00:05:48]
Listen, I like that you're doing this for us Redux Toolkit, but I still have opinions on how some of these should be used and I know what I'm doing. I would like to go ahead and create an action that works with Redux Toolkit follows all the right moves, but I can use to kinda format things differently.
[00:06:04]
We can totally do that as well. And so in this case, we'll go back to our tasksSlice, and we'll go ahead and use this create action, functionality. And so I'm just gonna name this one something slightly different just to highlight the fact that this is the one that I made.
[00:06:26]
I'll call it export const toggleTask. And instead of creating Slice I'm gonna create action. And create action takes, what do you want the type of the action that you're creating to be called cuz we do you want to task/toggle. If you really wanted to you could do tasks.action.toggle.string.
[00:06:48]
But we can figure it out based on the name and the key what we want it to be to trigger that reducer. And we can go here and this is basically, how do you want the action created work? We said before, we just wanna pass in a taskid, we just wanna pass in completed, and I want the payload, To be formatted with the taskid and the completed.
[00:07:18]
So we're taking that complexity we're using our component and putting it all in one place so we don't have to worry about it anymore. It's simply gonna take two arguments and it's gonna create that object for us. I gotta put in curly braces there as well, and that should do the tricks and now I can swap this one out.
[00:07:36]
Instead of having all this for both logic, I can actually say, ToggleTask, taskid, event.target.checked. Great, and let's make sure that that works. Everything works as we expected it to, I'm just gonna refresh to confirm one more time, yep, everything works. So you can create your own actions as well, if there's something about the syntax that the one that Redux Toolskit gives to you.
[00:08:11]
You can go ahead and pass in what the type of the action is, how you wanna format that payload yourself, and change a little bit of the API for that action as well. Great. There is one other problem that we need to solve for which is in our previous examples, all of the actions flew through all of the reducers.
[00:08:39]
And that wasn't a big deal. I mean, it was good in a lot of ways. Because we were just writing JavaScript. We're saying action.type, which means since every action we're doing it was we had the full power of JavaScript in front of us. We could just do whatever we wanted programmatically here, because we have so much obstruction if it becomes a little trickier, right?
[00:09:00]
If I wanted to change who a task is assigned to in the tasksSlice, that's not hard, but what if I wanna listen to a task action in the humans reducer, right? That was easy before, it's not that hard now, but it does involve learning an extra thing or two.
[00:09:18]
So let's actually do it in the tasksSlice first. And so we'll go into tasksSlice. And we're just gonna have one more which is, AssignedToUser. Okay, so what does assigned to user need? It needs a state in the action obviously. What needs to be on the action? If I want to assign a task for the user, what are the two things that I need to know?
[00:09:42]
>> Which stalls can what users use.
>> Yeah, I need to are those two things. And eventually that seems useful on that same action, right? To go through the user reducer. And also those are the same two pieces of things that I wanna use. I don't wanna fire two actions just cuz Redux Tools here try to do me a favor.
[00:09:59]
What I wanna figure out how to do is wiring this up in the taskSlice is not a big deal. But then I also want to tell the userSlice or the humanSlice, you also care about this too. And I wanna provide you with additional functionality to work with this and care about this.
[00:10:15]
So that's what we're gonna need to figure out now. All right, so we got assignToUser, we'll do state.find with the task again. I mean I can just copy that, let's grab that. And then we'll just do task.assignedTo action.payload.humanid. All right. And then, how do we deal with that in the other one?
[00:10:54]
Well, we'll go into our humanSlice. And we've got the reducers that are dedicated, that are actions created, that are for working with the humans in this case, but then I have this other one called extraReducers. And I guess that really good abstractions usually give you the ability to do the easy stuff easily and then drop down into a lower level when you need to do more complicated things.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops