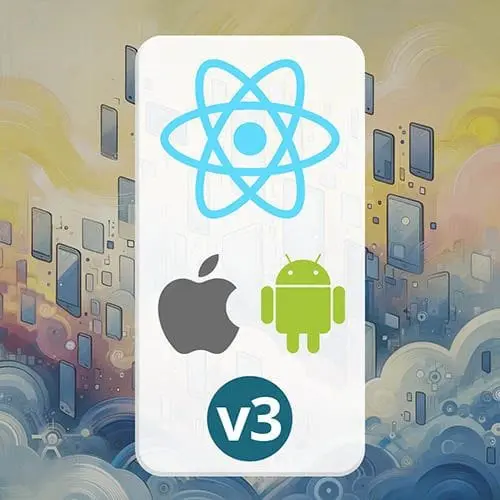
Check out a free preview of the full React Native, v3 course
The "Your First View Component with Styles" Lesson is part of the full, React Native, v3 course featured in this preview video. Here's what you'd learn in this lesson:
Kadi introduces the basic components in React Native, such as View and Text, and explains their differences from web development. She also covers styling in React Native, including how to use inline styles and create a global theme file.
Transcript from the "Your First View Component with Styles" Lesson
[00:00:00]
>> Kadi Kraman: So we're going to build a shopping list. So, if you scroll to the end of the screen, have an example of what we're going to start with. So, we're going to start with a shopping list item. So, it's gonna have some text and it's gonna have this cerulean line underneath.
[00:00:14]
I looked at this color from a color website because I liked it, and I tried to find out what its name is cuz I know it was blue, but it's not it's cerulean. So, now we all get to learn this color. All right, this is what a React Native app looks like.
[00:00:27]
So, if you're used to web development, you would expect this to be maybe a div. And, well, this wouldn't even have anything.
>> Kadi Kraman: So, this is what would look like on the Web. So, unlike the web in React Native, everything is wrapped in a component. So, you see that you replace this view with a div, is because a view is basically a React Native equivalent of a div.
[00:00:56]
We use it the same way, I know it sounds like when I first started. The name View sounded really confusing to me cuz it sounds like this big thing, like model view controller, you only have one that is not true. You have many, many, many, same as divs.
[00:01:10]
It's Views all the way down. And the second thing is a text. So, this is something that you don't have on the web and on the web, you can just put text anywhere and it will render. If I actually do this in React Native, I'm gonna get an error, because in React Native, all text must always be rendered inside a text component.
[00:01:31]
And the reason for this is that, React Native renders this as underlying native UI elements for each platforms. So, it will be the iOS text and the Android text and web text and macOS text and tvOS text. So, it needs to be wrapped in a text component, there we go.
[00:01:51]
And another thing to watch out for is something that you might be used to doing on the web, which is using this double ampersand to conditionally render things. So, you might do things like true and like render this thing, which is really common. And this actually works in React Native, that's fine.
[00:02:11]
And false would also work and it will not render it. The problem is, if you render something falsy, that actually renders on the screen. So, if you accidentally do if, like array.length, and the array is zero, and you render a zero here, you actually get this error. Because it's trying to render this zero, and then you get this error saying the text strings must not be rendered outside of a text component.
[00:02:34]
It used to be that empty strings had the same effect, thankfully that was resolved. But if you do not a number, this will actually also error. So, to play it safe, generally, in the React Native Codebase, you would do something like if not a number, and you do like, a full statement.
[00:02:56]
All right, and then the last thing we need before we can actually build our, which I'll bring this component, is to understand how styling works. So, styling in React Native, the built-in styles, are actually very similar to how styles work in the web. So, if you can do CSS for the web, you'll pretty much understand the styling here.
[00:03:18]
So, let's do a View. I'm gonna wrap my text here in a View, and then we can pass in a style prop. And then this takes an object. I'm going to just write these styles in line. So, I'm gonna start with just a background color. So I'm gonna make a background color, pink, same as we would on the web, just to see what's happening.
[00:03:43]
So, this is the view that's being rendered, and it's rendered then outside of our text. And we can use margins, paddings, and such. Actually the things you notice here, so on the Web, the CSS would look like this, I think. And obviously, this won't work because it's not a valid JavaScript object, but you can naturally figure out how to convert this into a JavaScript object, and that will probably work in React Native.
[00:04:17]
And let's add some padding.
>> Kadi Kraman: So the handy thing, I think CSS still doesn't have that.
>> Kadi Kraman: Let's do padding horizontal, and let's do padding vertical 16.
>> Kadi Kraman: There we go. So, instead of writing padding left and padding right, and padding down and padding bottom, we have these handy utility styles.
[00:04:53]
Where you can do horizontal and vertical padding, because it's just something that you end up using quite a lot. Wanted to remove this align item center here, so that this would float to the start of the screen. And you notice that this is using flex, so all styling in React Native is using FlexWorks.
[00:05:16]
So, do React Native for a couple of months, you're gonna get really, really good at flex. And if it would be the flex in React Native is really similar to flex on the Web as well, but some of the defaults are different, like just enough different that when you're moving from Web to React Native, you're like, hold on, that's a bit twisted.
[00:05:35]
I think I listed them here as well. Yeah, there we go. So, all elements have display flex by default, you never have to write display flex. And the main thing that catches you out is that the default flex direction is column instead of row on the web. And there's a couple of other differences as well but these are the main two.
[00:06:01]
>> Kadi Kraman: All right, let's add this bottom border, which is our pretty cerulean color.
>> Kadi Kraman: That is the background color. Border, bottom,
>> Kadi Kraman: Color, there you go, and the color doesn't show up because they haven't set a width. So, let's do your border, bottom, width, and let's do just a one.
[00:06:29]
You might think this is one pixel, but it's not. Notice that there's no units at all here. So, we have an 80 and 60 and a one, and in your head you might be thinking pixels, but they are not pixels. They're actually Display points. So, a display point is how actually differs by device.
[00:06:49]
So, d is pixel density, that's what it was or pixel ratio there we go. So, let me just show you if I print this out, so picture ratio get, there we go. So, it's a little bit small here we can see that on my iOS simulator the pixel ratio is three.
[00:07:13]
And this means that it's three by three square to render this, so one display point on this iOS device is actually three times three. And you might have pixel density of 2 or 3.5, etc. So this is what this unit is.
>> Kadi Kraman: And this also means that, if you're displaying an image on this on your React Native app, then on a high end iOS device, you'll need a larger image for it to look crisp than on a older Android device.
[00:07:51]
And actually, some Android devices have like, I'm very sure this phone has a pixel density of 3.5, so which can go the other way round. All right, and last, let's add styles to our text as well. So, let's do a font size of 18, make it a little bit bigger, let's call it something else, it's a shopping list.
[00:08:21]
Let's start with coffee, of course there we go. And I thought I'd change the font weight, so make the text a little bit thinner and Interesting, so let's do a font weight of 200. Now, the thing that I like to do if I'm using the built-in styles instead of a styling library is to have a global theme file that I use to pull all the commonly used styles.
[00:08:51]
So, in your root directory create a new file and let's call it theme.ts and let's export const theme. Export const theme, and let's move our color that we have here, the cerulian color into our theme file. So let's do color, cerulian.
>> Kadi Kraman: I can't even spell that, cerulean, there we go.
[00:09:29]
I think we used a white as well. So, let's move this background color here as well, and color white here. And this is kind of handy to do because you can't have global styles in React Native. So, unlike the Web where you have CSS and you have you just apply a style to all the H1 elements, for example, that's not possible.
[00:09:52]
So, an easy way to just share styles between multiple components is to have a shared theme file. So, that's just a pattern that I've used for ages. In production projects, I would put spacing here as well, so font sizes, spacing, any kind of units I would put here.
[00:10:10]
So, I would have like spacing 12, spacing 16. But we're not gonna do it for this workshop because I think that's forcing my preferences onto you. Yes.
>> Speaker 1: So in React Native, it's all styling with JavaScript objects. There isn't a thing like CSS modules, CSS variables, or Sass?
[00:10:29]
>> Kadi Kraman: Yeah, nothing like that is built in. There are styling libraries. So, NativeWind is a popular one, where you basically can use Tailwind styles in React Native in a similar way. And it's actually very popular, especially when you're coming from the Web, then you can use your existing Tailwind knowledge for that.
[00:10:48]
I think I've listed some, I think I haven't. I think I listed them in the intermediate course. Yeah, there's quite a few sign libraries, NativeWind is the big one. That you can use that opt out of the style sheet. But for the built-in, this is basically what you get, just a style object.
[00:11:15]
And finally, let's do a bit of tidying as well. So, you noticed that we have this style sheet create here? And here we pass the styles in line, and you can do both, it's obviously tidier to have this one style object at the bottom. And this is the common pattern actually.
[00:11:33]
So, every file that's a UI file where you render something, you will have your styles object at the end of the file where you do style sheet and then you have the styles. So if you do get these files, I go styles.itemContainer, item container.
>> Kadi Kraman: Here we go, and I'll move my font as well.
[00:12:01]
So this will be, styles.itemText. Item text,
>> Kadi Kraman: There we go. And the stylesheet create, it doesn't actually do anything special under the hood It mostly just does checking to make sure styles are valid that your types are valid. This is a standard reactive component.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops