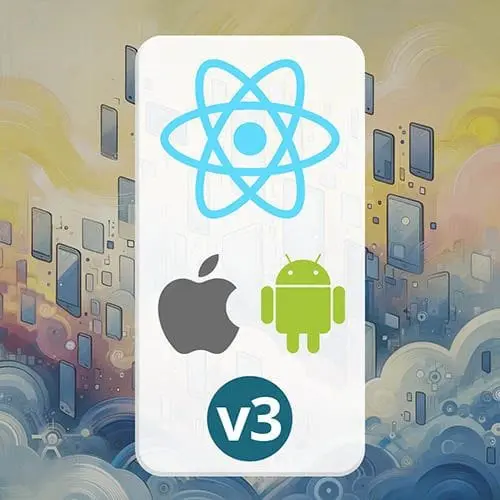
Check out a free preview of the full React Native, v3 course
The "Text Input" Lesson is part of the full, React Native, v3 course featured in this preview video. Here's what you'd learn in this lesson:
Kadi demonstrates how to style text inputs and handle user input using the useState hook. She also explains how to control the keyboard type and behavior, and how to submit the input and update the app's state accordingly.
Transcript from the "Text Input" Lesson
[00:00:00]
>> Kadi Kraman: So where we left off, we have our mobile app with a three-tab layout and our shopping list. We have our counter screen, with a nested stack navigator. And now, we're going to work on finishing up our shopping list app. So, so far, we have three shopping list items that are displayed in a list and they are static.
[00:00:26]
So obviously, we would like to have the user type in ShoppingListItems with a TextInput. So unlike the web, there is no form component in React Native, all the inputs are handled individually. So the input that we use to receive text from the user's keyboard is called a TextInput.
[00:00:51]
So we'll go to our index screen, we can create a TextInput. And you'll notice that nothing whatsoever happened. Well, it looks like, and it's because TextInput, by default, doesn't have any styling at all. So in order for it to be visible, we want to add some styling. So let's add a borderColor, let's say style.
[00:01:19]
Let's pull this into a style component, so let's do styles.textInput.
>> Kadi Kraman: And here, let's give it a borderColor. Let's do theme.colors, it says import theme.color, let's do a LightGray.
>> Kadi Kraman: And again, nothing's happened because we also need the borderWidth. So let's do a borderWidth of 2. There you go.
[00:01:53]
And now, we get our little TextInput. So let's also give it some space. So let's do padding: 12. So padding will give space inside the component. And let's do marginHorizontal: 12,
>> Kadi Kraman: So it's not pressed against the corners. And let's do a marginBottom: 12,
>> Kadi Kraman: So it's not next to this list.
[00:02:31]
And let's do a fontSize of 18.
>> Kadi Kraman: And like a borderRadius, so let's do a borderRadius,
>> Kadi Kraman: borderRadius of 50. Make them really rounded borders. All right, I'm gonna also get rid of this justifyContent and move things to the top of the screen. Now, in a TextInput, we can add a placeholder.
[00:03:08]
So let's do a placeholder, so e.g Coffee.
>> Kadi Kraman: There you go. So the way that the keyboard gets opened is actually if you focus on a TextInput, the keyboard will automatically open. I'm on a simulator here, so I need to do this. But basically, if I now reload, hopefully, there we go.
[00:03:39]
So by focusing on the TextInput, the keyboard opens. So that's an interesting thing. So the keyboard is actually controlled in a lot of ways by the TextInput. And I think we need some padding here so it's not against the screen. So just in my container, I'm gonna add a paddingTop of 12.
[00:04:02]
Lovely, let's do a controlled input, so we're gonna use a useState hook. So this is from React.
>> Kadi Kraman: So it will be a useState, and it's an import from react. And then a useState hook allows you to store little values very conveniently inside your react components. And it returns an array of two items, and you can name these array items whatever you want.
[00:04:35]
The first item will be the value that you are tracking. So in our case, it will be the value that is typed into the TextInput. And then the second array item will be a function that allows you to update this value. So usually, you would call it the thing and then set thing or update thing.
[00:04:55]
So I'm gonna call it value and then setValue.
>> Kadi Kraman: And then let's make it into, I'm gonna say that the default value of an empty string. And then in our TextInput,
>> Kadi Kraman: We'll do value= value. And on the web, you would use onChange where you get an event.
[00:05:23]
So there is an onChange here as well. But actually, more conveniently, you would usually use onChangeText. Which as you can see from here, rather than giving you a native synthetic event, where you have to extract a value, it actually just gives you the text, which is, most of the time, what you need.
[00:05:44]
So let's do onChangeText, and you can call it call setValue. So this will be a value and then recall setValue with the value. But as mentioned earlier, because the argument here and here are the same, we can just use this convenience and pass setValue directly here.
>> Kadi Kraman: So now I can do some typing.
[00:06:13]
So I mentioned that the keyboard is controlled by the TextInput, so there's some props here that guide you what the keyboard should look like. So for example, the keyboardType, so we're going to just use some text. But for example, if you wanted users to enter some numbers, you could have a numeric keyboard.
[00:06:34]
Or if it's an email address, you could have an email as keyboard, so we'll have the at symbol in a convenient place, etc. You can also have autoCapitalize, autoComplete, autoCorrect. So there's all kinds of properties here that you can use to make it more suitable for the thing that you're collecting inputs for.
[00:07:00]
So both text and numbers can be collected using this TextInput.
>> Kadi Kraman: And the other thing we want to do is if you use the returnKeyType.
>> Kadi Kraman: So this defines what this button on the bottom right will look like. So if we do returnKeyType done, it actually changes. So this will be a highlighted button.
[00:07:36]
And you'll notice that I didn't create a submit button next to this TextInput here. And it's because we can submit when the user presses this done button. And the way we do that is we use a callback, it's called onSubmitEditing. So let's do a console.log,
>> Kadi Kraman: Submitted.
>> Kadi Kraman: Oops,
>> Kadi Kraman: So now if I call done, you can see that it actually logs.
[00:08:19]
It calls this callback when the done button is pressed. Okay, and finally, let's hook up this list to use another useState so the shopping list is actually an array in our device memory. So this, let's start by creating a type. So this is a ShoppingListItemType. This is a TypeScript type, it will define what our shopping list will look like.
[00:08:48]
So we want an id, which is a string, and a name, which is also a string.
>> Kadi Kraman: And for now, let's also do an initialList, which you can do a ShoppingListItemType. So this is an array of those.
>> Kadi Kraman: Oops, it's a colon. And this will be an array of items, so I can do id, there's 1, and name is Coffee.
[00:09:35]
>> Kadi Kraman: And we also had some Tea and Milk.
>> Kadi Kraman: So the same way that we store this value, we can store the whole shopping list. So use another useState hook, and we'll do a shoppingList, and setShoppingList, and useState. And this will be an array of these ShoppingListItemTypes. And we're going to give it an initial value of this initialList.
[00:10:13]
>> Kadi Kraman: And now, instead of having these items here defined individually, we can map over them. So we'll do a shoppingList.map.
>> Kadi Kraman: And this will be an item, and for each of these, we will render one of the ShoppingListItems. And the ShoppingListItem name is gonna be item.name. I will also want to do a key, which will just be the item.id.
[00:10:48]
>> Kadi Kraman: And finally, let's hook up this onSubmitEditing. So,
>> Kadi Kraman: Let's do another function here called handleSubmit.
>> Kadi Kraman: And this will be an arrow function, and we'll just check that there is something that the user has entered. So we'll check that the value is not an empty string, so if (value).
[00:11:19]
Then we want to add this new ShoppingListItem at the top of our existing shoppingList. So we can use this setShoppingList function, which was in a useState to update it. So first, we'll create a newShoppingList. So let's do const newShoppingLiist, and this is gonna be an array, and we're going to spread the existing shoppingList.
[00:11:49]
So we'll need to keep whatever we had, and we'll add the new item that the user has just created to the top of the list. So we need two things here, we need the id and the name. So for the id, so if you needed unique ids, there's UUID library or there's NanoID libraries.
[00:12:09]
So there's ways to create unique ids. In our cases, the ids just need to be unique within our phone. So I'm just going to use the timestamp. So let's do new Date and do toISOString. So this will be unique within a millisecond. So unless you're adding shopping items close to the millisecond apart, then this will be unique.
[00:12:34]
And let's do name, will be the value. So now, we have our newShoppingList, and let's set the shoppingList to be our newShoppingList. And finally, we want to also clear the TextInput. So let setValue back to an empty string.
>> Kadi Kraman: And now that we have our handleSubmit function, we want to call it onSubmitEditing.
[00:13:07]
>> Kadi Kraman: So hopefully, what else do I need? Sugar.
>> Kadi Kraman: Lovely, and well, I'm going to type on my keyboard. So as soon as I type, this is just a simulator thing. But as soon as I type, the on-screen keyboard disappears, but it will work as well. So the only thing is that onSubmitEditing, if you're using this on a simulator, onSubmitEditing will trigger when you press Enter.
[00:13:38]
So I can add things as well.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops