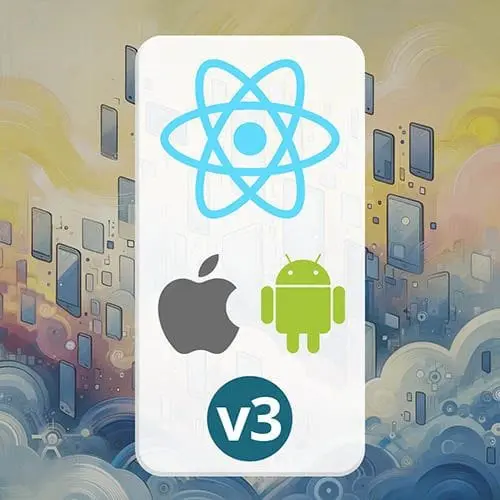
Lesson Description
The "Ordering & Sorting" Lesson is part of the full, React Native, v3 course featured in this preview video. Here's what you'd learn in this lesson:
Kadi demonstrates how to use the Icons component and style it to be rendered in a row with the item name. Kadi also explains how to order the items in the list based on their completion status and last updated timestamp using a sorting function.
Transcript from the "Ordering & Sorting" Lesson
[00:00:00]
>> Kadi Kraman: So it will be a slight detour, we're going back to icon buttons, let's call it revision. We wanted to have a circle if the item is not completed, and then a check if it is completed. So let's go to the shopping list item. And we want to use Entypo, so actually, so let's do expo lectral Icons.
[00:00:34]
And let's do a check.
>> Kadi Kraman: Yeah, that was the one. So import this,
>> Kadi Kraman: And render it, in the component. So what do we want to? So we want to be next to the name, there we go. Okay, so if I render them like this, so this is a layout thing again, because this pressable has a flex direction row, and I think it has a justify content space between, then it renders them in a row with space between.
[00:01:13]
But we would like the name and the icon to be next to each other. So, we'll wrap these in a view,
>> Kadi Kraman: Make sure we import it from react native. There you go, so now they are one is on the one side, one is on the other side, but by default they're rendering underneath each other, so we want this view to be rendered as a row.
[00:01:40]
So let's give it a style as well. So styles, row,
>> Kadi Kraman: Row, we'll just do flex direction row.
>> Kadi Kraman: And I think, can you do a gap? I think you can. If you do a flex direction row, you can use this gap property to add the gap between the items that you are rendering.
[00:02:08]
I think an example I used the margin, and the other hand the UI thing here is how you handle really long text. So what if this shopping list Python was really, really, really super long. Okay, so this is way too long, and it completely messes up our UI.
[00:02:32]
So what we can do here is, I think we give the item text a flex way 1. And we also need to give the row a flex 1, there we go. So now they fill the available container, and also if you want to truncate the text that a overflow certain amount, then in the text, you can actually have number of lines, like if the number of lines 1, and then, [COUGH] it automatically truncates the text it doesn't fit in.
[00:03:12]
So this guarantee stats really long text still works fine. And the last thing I've noticed that, I've made a mistake somewhere where I have some padding on either side. So I need to remove that. So I think in a container, probably just one padding horizontal. Opposite padding vertical, there you go.
[00:03:37]
I think this is what I intended. All right, so we have our check and let's do that, if the item is completed, it's a check, and if it's not completed, it's a different icon. So let's do a circle, if it's not completed. So is completed, we'll do a check, and otherwise we'll do a circle.
[00:04:13]
>> Kadi Kraman: Okay, I think that's good. And we can customize the color as well, so let's do, if it's completed let's do a gray cuz it's grayed out, cuz everything in our completed section is grayed out. And if it's not completed, let's use our cerulean color. Okay lovely. I think this was the UI in the example app.
[00:04:48]
But hey, we've got some extra UI elements, UI patterns that we looked at. So handling overly long text. Generally flex 1 is really helpful, because flex 1 means, fill all the available space. So if things are overflowing, flex 1 is usually what fixes it.
>> Kadi Kraman: All right.
>> Kadi Kraman: And then the last thing that we wanted to do here, is we wanted to order this by last updated.
[00:05:27]
So what I would like to do is that, okay, so everything is uncompleted here. So if I complete this, I would like this to move to the bottom of my list. And then if I uncomplete something, I want it to move to the top of the list. So newest items would be first.
[00:05:54]
And the way we do that is we're going to write a little sorting or like ordering function. And feel free to copy this, but this is the reason that we used our completed at timestamp rather than a number. So this is nothing particularly fancy, it's just a JavaScript sort function.
[00:06:13]
But let's copy that, and paste it into our index file.
>> Kadi Kraman: [COUGH] And then this relies on something that I haven't added yet, which is the last updated timestamp. So in order to order between items that you completed or uncompleted that doesn't [INAUDIBLE] time each of know when they last updated.
[00:06:46]
So let's also act this last updated timestamp here, so this would be a mandotory property. And then whenever we update we create a new item of shopping list or we toggle its completion state, we also update the timestamp when it was last updated. So here in handle submit, let's do last updated, I'll just copy this.
[00:07:14]
Last updated timestamp will be date.now.
>> Kadi Kraman: Love it, and then the same in handle to who will complete. So regardless of whether we're toggling it complete or uncomplete, we're just updating the last updated timestamp. So whenever we're now interacting with our shopping list, we're going to be like updating this last updated timestamp as well.
[00:07:44]
So lemme just talk you through what this ordering function does. Using the JavaScript array .sort method, and the way that works is that you pass in a sorting function, and this gives you item A and item B, so in our case item 1 and item 2. And you return a number which defines whether item A or the first item should be a for the second item or no, so if the number you return is positive, then item 1 needs to be before item 2.
[00:08:25]
And if it's negative, then item 2 should be before item 1, if it's 0 then they are off like equal, they are pretty much equal. And the reason this is actually really cool, and the reason we use timestamps is that, this number can be as big or as small as you want, so it can be minus 1000 or plus 34.
[00:08:51]
Which means that we can subtract timestamps to automatically make one item render before the other. So what we're doing here is, we're doing if both of them are completed, so they'll be at the bottom of the list then we render the last updated item first. If one of them isn't completed, that's pretty obvious, so the first one is completed, the second one isn't, first one gets rendered first.
[00:09:24]
If the second one is completed, first one isn't, second one gets rendered first. And otherwise, if NIDRhythm are completed, then we can again do our fancy subtraction to all them correctly. So if you haven't reload now, just to make sure that all the new items that you create have this last updated timestamp.
[00:09:50]
And lemme just add some my favorites, coffee, tea, milk, sugar sparkling water, or I should write Lacroix, right. La croix there we go. So I've got my shopping list again, and we're going to order it just before we pass it into our flat list. So enough of that list in the data, let's just wrap this shopping list array into order shopping list.
[00:10:28]
So now, if I mark the sparkling water as complete, it will go at the bottom of the list. So marking the sugar as complete, it will go just above the sparkling water etc. And now if I uncomplete it, this will go at the top of the list as if I've just added it.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops