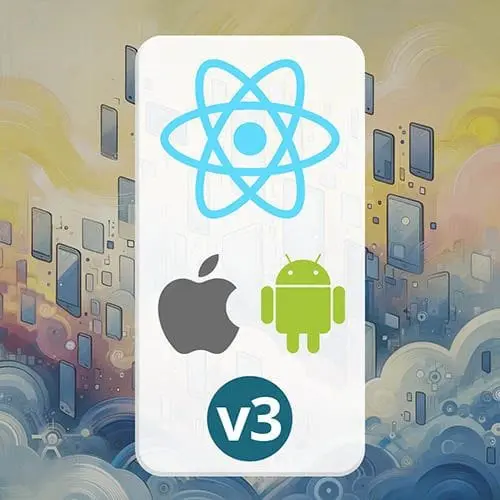
Lesson Description
The "Data Persistence" Lesson is part of the full, React Native, v3 course featured in this preview video. Here's what you'd learn in this lesson:
Kadi explains how to use async storage in React Native to persist data across app launches. She also demonstrates how to use the useEffect hook to retrieve data from async storage when the app loads, and how to update async storage when adding, deleting, or modifying data.
Transcript from the "Data Persistence" Lesson
[00:00:00]
>> Kadi Kraman: So far, we've created our shopping list, but the problem is that every time we refresh our app, so if you shake your phone and refresh, or you close [INAUDIBLE], you go and reopens, or in my case, if I just reload this, the shopping list is empty. And that's because we are rendering our shopping list in memory In our new state.
[00:00:23]
So what we really want to do is you want to persist this shopping list when we save it, so that when we close our app and reopen it, it would still be there. On the web you might use local storage for this and the native equivalent of this Is asyncstorage.
[00:00:42]
It's a key-value store, so it's an unsecured key-value store for native mobile applications. And you can use it pretty much the same as local storage. The only difference is, well, the clue's in the name. It's asynchronous, so all the calls to async storage are asynchronous. So let's install Async Storage.
[00:01:06]
>> Kadi Kraman: So we'll do NPX expo install, React Native Async Storage, Async Storage. And actually, here's a fun thing about Async Storage. So this actually used to be part of the core React Native package, but it's an example of how the React team at Meta really wanted to draw the line between what the community should handle and what the core React Native package should handle.
[00:01:32]
So this is actually a great example of something that used to be part of React Native Core, and it was explicitly moved out and turned into a community library so it could be Built, developed and managed independently of the core React native package. So let's write a, let's create a UILs folder so new folder UILs and.
[00:02:08]
>> Kadi Kraman: And let's call a function called storage.ts. So we'll put our async storage kind of utility functions now. And the two things you wanna do with async storage Because you wanna get, and you wanna set. So let's do export async function getFromStorage.
>> Kadi Kraman: And for this, we need a key which is a string.
[00:02:43]
So this will be the key for your asing storage on your device, and it'll be scoped to the application you're using. So one application can't access things from another application's asing storage, unless you, well jade break your device and then all things kind of things can. Can happen, but generally they're scoped to the application that you're using.
[00:03:06]
>> Kadi Kraman: So what I want to do is we'll do const data.
>> Kadi Kraman: And this will be an asynchronous function, so await asyncstorage. So this is the first The default export from the acing storage package, and we'll do the item with the key.
>> Kadi Kraman: And everything in Async storage is a string So we're going to save a JavaScript object, and so adjacent object, so we will pass it so.
[00:03:59]
>> Kadi Kraman: [COUGH] Actually, the best way to do this is to use the The way to do this is, usually, if you're parsing anything or you want to wrap it into a try-catch, so let's do a try and a catch over this, and then default to returning null. Whenever you JSON parse everything, make sure you do a try-catch in React Native, because otherwise, things might crash if the thing that you're passing is not a valid JSON object.
[00:04:27]
So we want to return if there is data. So it's not the initial time we'll do JSON pass data and otherwise let's return a null. And I probably should have done a save from storage first but let's do an export async function save to storage and here we need to do a key again so this is the key the string address so, Where we're saving to and then our data.
[00:05:05]
Let's just accept any old object in this case.
>> Kadi Kraman: And let's also do a try catch around this just in case and we'll just do an await.
>> Kadi Kraman: AsyncStorage.set Item and we'll have two properties so the first one will be the key so the address of where you're saving it to and the second one will be what you're saving so this has to be a string so we call it jsonStringify On this data object.
[00:05:45]
So the reason I say that probably should have done this, save the storage first, is actually the way that you get your item depends on what you're saving. So in this case, I'm saying that I'm always going to be saving an object. And that's why I'm calling json.stringify on it.
[00:06:03]
And then therefore, when we get the item, we can call jsonparse on it. But you could set just like any value value here. So it could just be a string. So as long as it's a string, it can be anything. And one thing to note is because you can only store strings here, then everything that you store in these storage has to be serializable.
[00:06:31]
So you can't have date objects, for example.
>> Kadi Kraman: All right, so now it's time to use it. So, let's create our storage key in our in our index screen. So let's do const storageKey.
>> Kadi Kraman: And this can be anything I'm just gonna call it shopping list, but this will be unique.
[00:06:58]
So whenever you go to asyncstorage on this app, using this key- You will get that data. And we're going to use a useEffect. So useEffect is a React hook and using it like this, so we're going to pass in just an arrow function and an empty array. So two arguments, an arrow function and empty array.
[00:07:22]
And then what this does is that it lets you execute a thing once when the component is loaded. So in this case, it's very common to use this to fetch some initial data. One thing about use effects is that they are synchronous, so you can't do anything Asynchronous in them.
[00:07:43]
So, as you might have recalled, our async storage is asynchronous. So how do you execute an asynchronous function from a synchronous useeffect. And the really common workaround for this is that you define your asynchronous function inside your useEffect. So I'm going to do const fetch initial and this will be an asynchronous arrow function and it's going to do const data so this will be await get From storage to the sketch from storage is the utility function which is created, and we'll pass in the storage key
>> Kadi Kraman: So if data we will setShoppingList data, so yeah so this will be an array of items so I know I'll call this an object but an array is an object in JavaScript, but okay so the way we call it Is we literally just call fetch initial here immediately underneath it.
[00:09:05]
So this is, we could have defined this like, like somewhere else, but this is like the the way that you would execute an asynchronous function inside a useEffect. So we're not waiting, so we can't do a wait, so this will. This will tell us that this is asynchronous and if we try to make this asynchronous, it would fail, so we're just fire and forgetting it.
[00:09:29]
And the last thing we need to do is we need to sync all of our updates. So whenever we are creating a new item or we are toggling the completion of an item, we also update our a sync storage. So here, in HandleSubmit, where we're setting our shopping list, let's also do Save to Storage and we have our key and our shopping list.
[00:09:58]
>> Kadi Kraman: I'm going to call this our storage key. And when we are deleting it we are doing the same, so we are doing save to storage, storage key and shopping list and save for an alter we complete. So save to storage key storage key, and the new shopping list.
[00:10:33]
Lovely, so now when I do coffee, tea, milk, sugar, I'm just going to mark one of them as complete, so now, hopefully, if I reload my app, all of them are still here, and my status persisted. So that's how you use asyncstorage in React Native. To persist data across app launches,
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops