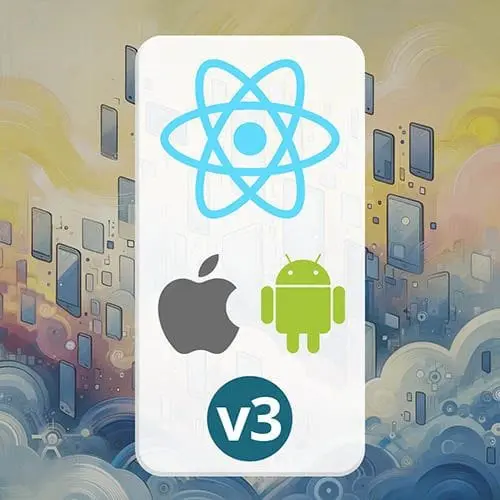
Check out a free preview of the full React Native, v3 course
The "Create a Button with Pressable" Lesson is part of the full, React Native, v3 course featured in this preview video. Here's what you'd learn in this lesson:
Kadi demonstrates how to create a delete button using touchable components in React Native. She explains that the built-in button element in React Native is not commonly used because it cannot be customized. She also shows how to add styling to the button, handle the onPress event, and display an alert confirmation with options for the user to delete or cancel.
Transcript from the "Create a Button with Pressable" Lesson
[00:00:00]
>> Kadi Kraman: Next up, we're going to create a delete button. So we're gonna look at some touchables. So if you scroll to the bottom, you see what we're going to build. So we're going to have a DELETE button to the right of our item. And we're going to have this confirmation alert if you press on it.
[00:00:17]
So, react-native comes with a Button element and it's almost never used. I'll show you why, cuz if I render this Button title,
>> Kadi Kraman: It looks like this. It looks slightly different on Android, it basically renders a native-looking button for each platform. And you can't customize it at all, you can't give it any kind of styles.
[00:00:46]
And this is why we only tend to use this Button element if we're just testing out something locally. But generally, for production apps, you don't use it. But the way that you actually make something into a button in react-native is you wrap it in a Touchable or a Pressable components.
[00:01:07]
So, the most commonly used ones are a TouchableOpacity and a Pressable.
>> Kadi Kraman: So let me create some text here,
>> Kadi Kraman: Delete,
>> Kadi Kraman: And let's wrap this in a Pressable. So Pressable is kind of the new generation of Touchable components. Let me do an onPress,
>> Kadi Kraman: And this takes a function, and we can console.log, just console.log something.
[00:01:57]
>> Kadi Kraman: So now when I press it, actually, I wonder if I can open my terminal here. I don't really use the VS Code terminal. Terminal > New Terminal, there we go.
>> Kadi Kraman: So let's do yarn start. All right, so I have my terminal running here. Yeah, there we go.
[00:02:16]
So this console.log gets executed. And this is kinda the new generation of Pressable components. It has some additional props that you can use.
>> Kadi Kraman: There you go. You can have onHoverIn, HoverOut, onPress, PressIn, PressOut. You can also have passing custom styles based on whether the button is pressed or not.
[00:02:42]
But let's keep it simple and use a TouchableOpacity. And this is very handy and very popular, because when you press something, then it automatically applies an opacity. I always find the default opacity to be a bit intense. So you can actually change this with the activeOpacity prop. So if we do, let's do 0.8, so 80%.
[00:03:08]
Yeah, there we go. So there's still opacity, but it's less intense.
>> Kadi Kraman: So we're gonna create a button with a black background, so let's add a colorBlack.
>> Kadi Kraman: So this is 000.
>> Kadi Kraman: And let's put this button inside our item. So it will be here. There we go.
[00:03:46]
But they're rendered underneath each other, which is inconvenient. So, how do we render them in a row? Well, we are in the world of flex, so in our itemContainer, let's do a flexDirection of row. There you go. Then they get rendered next to each other, but we want the Delete button to be at the end of the screen and the copy to be on the other side.
[00:04:10]
So let's do a justifyContent, and you want space between. So this renders them with a space between.
>> Kadi Kraman: And also, to make sure that these are centered, actually, you can't see it that much because they're not next to each other now, but they're not actually centered. So if we do alignItems center, then they're actually centered on the same line.
[00:04:38]
And now, let's do our space between.
>> Kadi Kraman: All right, so this TouchableOpacity, we want to give this a black background. So let's do a style={styles.button} and add a button style here.
>> Kadi Kraman: And let's give this a backgroundColor of theme.colorBlack.
>> Kadi Kraman: Obviously, our text isn't showing up now, so we'll need to make the text, right?
[00:05:17]
So for the text, let's do a style={styles.buttonText}. Let's create this buttonText style to have the color be theme.colorWhite. Beautiful, and back to our button, let's add some spacing. So let's do a padding of 8. And there you go. And borderRadiuses tend to look nice on buttons, so let's do a borderRadius of 6.
[00:06:00]
So these numbers might look random, but I tend to have a convention, I guess, for myself, where I only use even numbers. So I tend to use 2, 4, 8, 12, 16, 24, 42. And I think it's just conventions from styling guides. And then for the borderRadius, I found that borderRadius 6 just tends to look nice [LAUGH] in most cases, so I tend to default for that.
[00:06:30]
So lastly, for our buttonText, let's make it bold, so let's do a fontWeight of bold.
>> Kadi Kraman: And let's make it uppercase, so we can use textTransform.
>> Kadi Kraman: Uppercase to make it an uppercase button. And finally, I find that the letters are a bit cramped. So we can use letterSpacing to actually add some spacing between the letters.
[00:07:03]
So if we do letterSpacing: 1, you can see that it kind of breaks them apart a bit. It looks a bit pretty.
>> Kadi Kraman: All right, so we've created this button now, let's also add this alert confirmation. So alert is a built-in component in React Native, so you can import alert from React Native.
[00:07:35]
>> Kadi Kraman: And let's create a function that is inside our React component, so let's do const handleDelete. So let's do an arrow function. And again, whether you use arrow functions or function functions, that is up to you. But I'll just tell you the convention that I am currently favoring, which is that if you have components, they will be function functions.
[00:07:57]
And then things inside the components, like low utility functions, I tend to use arrow functions. So just my preference, but there's no particular reason you have to follow it. All right, so let's do an Alert.alert.
>> Kadi Kraman: Are you sure you want to delete this? And in our TouchableOpacity, when we onPress, we can call this alert.
[00:08:29]
And this is a React thing, but if you have a function that doesn't take any arguments, all the arguments are the same as what I passed in from the component. You can actually omit this arrow function, nonsense, and just put handleDelete here and it will call itself. And now, if I do DELETE, I'll get a little alert.
[00:08:54]
>> Kadi Kraman: So this alert just has one text, but we wanna give some subtext as well. So we can pass in a second argument for some subtext, so let's do, it will be gone for good.
>> Kadi Kraman: There you go. Now, we have some additional text here, but we don't have the confirmation buttons.
[00:09:21]
So we can pass in a third argument, and this will be an array of options for the buttons that you want to display. So this will be an array of objects. So the first one, let's say, text: Yes. So yes, I do want to delete it. Then we can give it an onPress, so if the user chooses to delete it, what do we do?
[00:09:44]
Let's pass in an arrow function. And then for now, let's just do a console.log of Ok, deleting, and we're gonna fill this in later. And we can also give it a style to make it red, so we can say that it is destructive. And the second button we want is a Cancel button, so let's do a text: Cancel,
>> Kadi Kraman: style: cancel.
[00:10:16]
>> Kadi Kraman: There we go. So now if I choose DELETE, we actually get this Cancel and Yes. So if I do Cancel, I don't get any logs. And if I do Yes, it executes the function that was passed in with the onPress. So now, if I run this on a Android and I do DELETE, you actually notice that this alert looks different than my iOS one.
[00:10:37]
And that's because it's recognitive, it's using a platform-specific alert, which is cool.
>> Speaker 2: I want to customize the alert, would I have to build a custom dialogue?
>> Kadi Kraman: Yes, yeah, if you want to customize the alert, you would build a custom dialogue. It's not that tricky to do that, mostly, you would do a full screen modal.
[00:10:58]
Do we do modals here? We do actually go through what a modal looks like. You would do a full screen modal with a transparent background, and then you can do a small alert with whatever styling that you want. It's actually a good idea to commit when you get to a point that things are working.
[00:11:13]
Because then when you inevitably try things that make things not to work, you can always go back in time to start again.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops