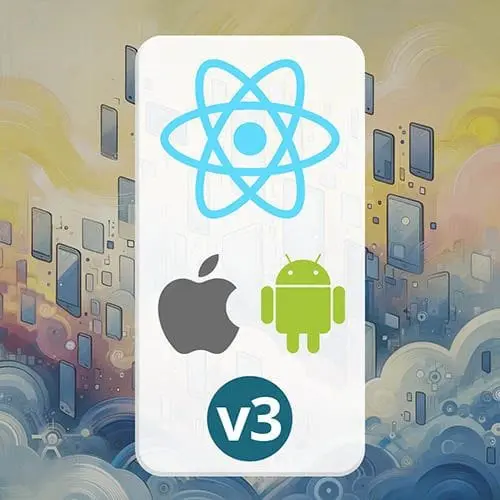
Check out a free preview of the full React Native, v3 course
The "Confetti & Haptics" Lesson is part of the full, React Native, v3 course featured in this preview video. Here's what you'd learn in this lesson:
Kadi adds haptic feedback using the Expo Haptics library and confetti using the Confetti Cannon library to add a fun and celebratory effect when a certain action is triggered.
Transcript from the "Confetti & Haptics" Lesson
[00:00:00]
>> Kadi Kraman: So functionally, our app is now ready. We have a shopping list, where we can add things to our list. We can delete them, we can mark them as completed, we have some haptics, we have ASIC storage. And we have our counter or timeout scheduler, whatever you wanna call it, where we count down from a time and we can schedule notifications to remind us to do something.
[00:00:24]
And the last thing, I just wanted to add some joy to this counter application. So we're going to add some confetti, who doesn't love confetti when good things happen? And of course, add a good success haptic feedback when you press this button. [COUGH]
>> Kadi Kraman: So for the haptics, should be an old hand at this, but let's install, let's import haptics.
[00:00:57]
So import star as Haptics from expo-haptics
>> Kadi Kraman: And just before we schedule a notification in here, so when we press the scheduleNotification button, let's do Haptics.notificationAsync. And let's do Haptics.,
>> Kadi Kraman: NotificationFeedbackStyle.Success
>> Kadi Kraman: So there should be a big old heavy haptic feedback. So let me try it on my Android phone.
[00:01:53]
>> Kadi Kraman: So if I hit this, that was a nice satisfying feeling haptic feedback.
>> Kadi Kraman: And lastly, let's add some confetti. So this is just a little JavaScript library to add some confetti into your React Native app. So it was a bit cute and fun. So let's install the ConfettiCannon.
[00:02:31]
>> Kadi Kraman: All right, so the way this works, it looks like I need to. Whenever you see refreshing or the app doesn't load, then the standard thing to try, by the way, I think I haven't mentioned this before, is you stop your bundler. So wherever your metro process is, you stop it and then you start it again.
[00:02:55]
If it still doesn't work, if you think something is cached, you can actually start with --reset-cache. So just an FYI. In this case, I will just start it and reload.
>> Kadi Kraman: There we go.
>> Kadi Kraman: Turn it off and on again. [COUGH] All right, so ConfettiCannon. So we're gonna place the ConfettiCannon at the end of our screen.
[00:03:36]
So Confetti, it should be probably imported. So import ConfettiCannon from,
>> Kadi Kraman: react-native-confetti-cannon. And now, we're going to render it at the very bottom of our file.
>> Kadi Kraman: Right, so we need to trigger this ConfettiCannon programmatically. So it's kind of here, but we don't want it to fire when you just open the page.
[00:04:19]
>> Kadi Kraman: So the way we fire it is using a ref. So a ref is something we haven't used before, but it is another React hook, and it basically contains a reference to an element on the screen. So you can call functions on it, for example, which is what we're going to use it for.
[00:04:39]
So confettiRef, so we'll do a useRef, and we're gonna start with nothing, which is fine.
>> Kadi Kraman: [COUGH] I'm just gonna type it as any because I think the ConfettiCannon didn't have types.
>> Kadi Kraman: And so what we'll do is we'll pass this ref into the ConfettiCannon component. So ref = confettiRef.
[00:05:21]
>> Kadi Kraman: And then we have some properties as well that we can do. So let's do a count of 50. I think I found the default count was a bit intense on Android phones. And,
>> Kadi Kraman: Okay, we also need to set where we want it to be displayed. So let's do an origin.
[00:05:48]
And okay, so by default, I'm going to just do x0, y0 and see what happens.
>> Kadi Kraman: Okay, so we go on the screen and there's a media of confetti from the left of our screen, which is the x0, y0 position on your screen. And this is great, but not exactly what we want.
[00:06:11]
So we wanted to, firstly, not stay here, so we wanted to fade out. fadeOut = true. I think we can just pass in fadeOut because it's a Boolean. There you go. So now it will launch and it will sprinkle down and it will disappear, which is better. And we also want it to actually be in the center of the page instead of in the corner.
[00:06:42]
And we want it to be in the middle of our screen, but the problem is that screens are a different size. So if we just do x, let's say 100,
>> Kadi Kraman: Then this might be here on my screen, but the phone screens aren't always the same distance. So we can use the dimensions from React Native to get the dimensions of the current device to position its center for that device.
[00:07:11]
So this is Dimensions from here, from React Native.
>> Kadi Kraman: And we can do Dimensions,
>> Kadi Kraman: get(window), and we do width/2. So now, it's actually quite handy for testing when it fires every time I open the page. There we go. And now, it's fired from the center. In fact, now that I look at it, I think I would like it to be just off the screen when it fires rather than having this little pile of confetti.
[00:08:00]
So I can make it use the y value to put it slightly off the screen.
>> Kadi Kraman: I think that looks better. And actually, we use this Dimensions value from React Native. It's worth knowing that this actually comes with a hook as well. So instead of this, I could use,
>> Kadi Kraman: useWindowDimensions.
[00:08:30]
So useWindowDimensions is also an export from React Native. And then this gives you a width, the window width. So instead of doing Dimensions.get, I could actually use this width here instead. And the good thing about that is if you're building a mobile app, which is responsive, so you could set it to landscape or portrait, then this would adapt.
[00:08:59]
Otherwise, with the initial implementation, where we import dimensions from React Native, then it is set once. And then it won't get recalculated if you changed your device size. So that is handy. Let's do autoStart = false. And we can use our confettiRef instead to start it programmatically. So when we go to this scheduleNotification, so we kind of kept it as scheduleNotification, although it's kinda like I've completed the thing, but let's keep it.
[00:09:37]
So let's also do our confetti. So confettiRef?.current, so refs always have ref.current, and start.
>> Kadi Kraman: Now, this confetti should confetti when we press the button, which is awesome. Everything is so much more satisfying with confetti.
>> Kadi Kraman: And lastly, it's worth updating this to something, I kinda call this thing, because it really depends on something that you need to do.
[00:10:13]
But a good example for me is washing the car. So something you need to do every two weeks, and it tends to take longer. So this is what the initial thing that I had in mind when I was building this. So I'm gonna change this to two weeks.
[00:10:26]
Who knows what two weeks is in milliseconds? So two weeks in milliseconds is, right, so we've got 60 seconds. And then this will be one hour, and this would be a day. So this would then be 14 days, which is two weeks.
>> Kadi Kraman: Okay, so it's a thing that I want to do every two weeks.
[00:11:01]
And I'm gonna just update this name as well, so I've washed the car.
>> Kadi Kraman: There we go. And,
>> Kadi Kraman: Car wash due in, and Car wash overdue by. And in my notification, oops, where's the notification?
>> Kadi Kraman: Let's say Time to wash the car.
>> Kadi Kraman: Let's add a car emoji.
[00:11:55]
>> Kadi Kraman: There we go. So now it's updated to 14 days. So I didn't use this value for testing because looking at this countdown is a little bit more boring than looking at ten seconds. But the idea is you could tweak this history to also see how frequently you've done it.
[00:12:10]
So let's say that you need to wash the car every two weeks, but maybe it took three weeks and maybe it took one week. So you can have some additional handling here that will tell you the distance between the last two history items. Let me just, for the last time, double-check this on my Android phone.
[00:12:43]
>> Kadi Kraman: There we go. So car washed due in 13 days, but I'm very eager, so washing the car now. And I get some confetti and the item is added to my history, which is great.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops