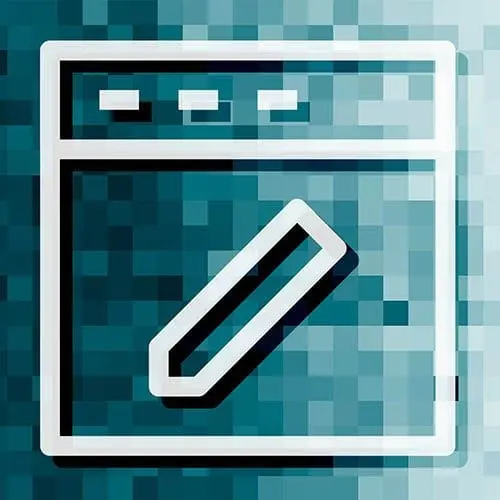
Lesson Description
The "Filtering with JavaScript" Lesson is part of the full, Professional CSS: Build a Website from Scratch course featured in this preview video. Here's what you'd learn in this lesson:
Kevin demonstrates how to create a filtering functionality for a set of cards using JavaScript. Kevin also discusses the event listener for the change event and shows how to retrieve the selected value from the event target.
Transcript from the "Filtering with JavaScript" Lesson
[00:00:00]
>> Kevin Powell: So to create the filtering, we could include this in our main file here. So if you wanna do that, that's completely fine. I'm just gonna do it in a separate file 'cuz that way, for our own purposes, it keeps it more organized. We won't get mixed up with the two different bits of JavaScript that are going on.
[00:00:14]
Plus, we only need it on this page anyway. So we can just do mushroom-filtering.js, or maybe mushroom-filter.js. And [LAUGH] as usual, never forget when you do this, that you wanna go back and make sure you add the link to that file. So, we can come all the way back up.
[00:00:35]
I have this script here. Let's just close that for a second. And I can come in with another script, src="/mushroom-filter, and I can defer it. Now, in here, we're gonna need three different things that we're gonna need to get. The first one is we need to get all of the cards, cuz we're gonna need to look at those cards to be able to filter them.
[00:00:56]
So we need to say const cards and document.querySelectorAll. In the last time we did it, it was a query selector cuz we only needed one element. This time, I need a lot of elements, so I'm gonna get all. And on this page, we have more than one set of cards.
[00:01:15]
I have these cards here, but our Bento, we also use cards as well. But this is always just a CSS selector, and we have that mushroom guide parent. So I can say '.mushroom.guide space .card', and it scopes that selector or the variable there. So we're only getting the cards that are inside of our mushroom guide.
[00:01:34]
The other thing we need to get is we need to know what's selected from our two selects here. So to be able to do that, we can come in with a const of, we'll do a seasonalFilter is going to be equal to document.querySelector. And for that, I just recently put that id on there, so we can do the id of #season.
[00:01:59]
And const edibleFilter = document.querySelector and the id of #edible on that one right there. Before we do anything else, we need to keep track of which filters are actually selected at any given time with JavaScript. So the easiest thing to do, in my opinion, is to create an object at the beginning that will update to be able to keep track of those.
[00:02:26]
So we can call that our current filter currentFilters, cuz we have two different filters. And this looks a bit different, but we're gonna do it like that with the curly braces. So we're creating an object that we can store different information inside of. And season by default will be all, right, cuz we have all of our seasons.
[00:02:44]
That's the first one that will always be on. Don't put a semicolon, put a comma here. I always put semicolons, and it gets me in trouble. And then we can say, whoops, edible is also all, cuz that's the default filters that we're starting with. So we have our cards, we're selecting all of them.
[00:03:01]
We have each one of the two filters just so we have them, that we can be able to access the information in there. And then we're saying, currently, when the page first loads and the JavaScript fires, we have a season of all and an edible of all. So our filters are currently showing us everything.
[00:03:16]
To look quickly at what we can do with this, let's grab that seasonalFilter. And we saw this earlier, we can add an event listener. Last time we looked at the addEventListener, we did a click and then we did the function here. So we're not gonna keep the click, but I'll set it up like this.
[00:03:34]
The reason we don't wanna do it as a click is cuz that means when I click on it, it's gonna do this function that we're putting in there. And if I do that every time I click, it means it's firing when I'm opening it as well. And the click, just so you know, if I'm using space bar to open it, the click is still firing there, and that just doesn't seem like the right type of way to approach it.
[00:03:53]
So another event listener that we can put here is change. And what change is doing is it's looking for when, if I click on Seasons and then I just select the same one or I click away, nothing's changed, so the event listener doesn't do anything. If I come here and I actually change what's selected, now, we're gonna do whatever we're doing within this function.
[00:04:13]
Again, an easy way to be able to adjust this. What we can do is we can look at this current filter. So we could come here and we could say that the currentFilters, and we're doing our seasonalFilter, so I can say the season is going to be equal to something.
[00:04:28]
And what I wanted to get is the value of whatever I've clicked in here. Cuz if you remember when we set this up in the select, let's just do it, select, we gave each one of these a value, and we can get that value when it's selected. To be able to do that, when we have our function that's going here, I'm gonna put an event, just so we're able to use the event.
[00:04:52]
So whenever it changes, and we'll see what this event is in a second, we're gonna use that event and get where we're getting from it. Or actually, let's see what the event is before we do this next step, just so it makes a bit of sense if you haven't done this before.
[00:05:04]
So we can console.log the event itself that's happening. We'll inspect on there. Let me just move this down to the bottom.
>> Kevin Powell: We'll jump on over to my console and we'll go find my select. And if I do spring, you can see the event fires. So it's saying here's the event, and it's giving me all this information that I can get from it.
[00:05:30]
The type was changed, cuz that's what we were looking for. So that makes sense that that's what we're getting here. And there's lots of different stuff that we can grab. And one of those things is the actual trusted, type, target, and there's all this information. But one thing we can get from it, it's with the target there.
[00:05:46]
So we can see there's a target, is our season. So if we say event.target and I go and I choose Spring, you see I'm getting the id of season. And there's all this other stuff that's in there. And we can get the one that was selected by coming on here and saying .value.
[00:06:07]
And if I have that, when I click Spring, you can see it's grabbing the spring. It's not grabbing it because of the text here, it's grabbing it because that was the value that we had on the option. So if I go to Summer, we get summer coming through here.
[00:06:19]
So what I wanna do is use that event.target filter. We don't need any console.log anymore. We wanna say that the current filter, the season, instead of being all, is now equal to that value that we had right there. So if I do that, and then we do a console.log again, but we do it for our currentFilters, if I change to Spring, you can see now the season is 'spring', edible: 'all'.
[00:06:50]
If I change the edible to toxic, we should see, I didn't set one on that one yet,. We can change the season though, Summer, and we can change to the fall, and it's coming up to the fall. So we're updating the current filter every time that we choose something there.
[00:07:04]
Now, one thing we could do is, as we just realized, we don't have anything on this side for that filter. So the option that we have here is actually to take all of this, copy it, paste it, and then do the exact same thing for the other filter.
[00:07:20]
Whenever you do that, especially in JavaScript, same thing in CSS, though, if we can make something more generic, that's usually a good idea. Just cuz if then ever you need to update it, you're only updating it once. So anytime you need to do the same thing twice, it's probably a good idea to see if you can find a way to make something more generic that can work for either one of them.
[00:07:37]
So that's what we're gonna do next.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops