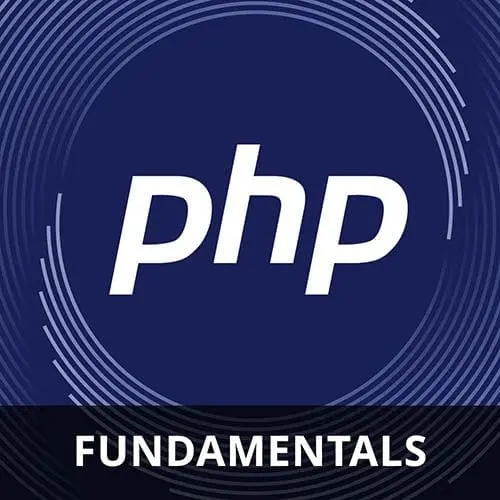
Check out a free preview of the full PHP Basics course
The "Loading Data from API & Parsing JSON" Lesson is part of the full, PHP Basics course featured in this preview video. Here's what you'd learn in this lesson:
Maximiliano uses the file_get_contents method to load data from an external API. After receiving the data, the JSON is decoded, and the converted currency value is returned from the class to the converter.php file.
Transcript from the "Loading Data from API & Parsing JSON" Lesson
[00:00:00]
>> Maximiliano Firtman: Now let's try to do something within the converter because yeah it's not really converting okay so it's doing nothing actually after we have the converter we can get the result and we can say how to call the method convert thin arrow. So, I'm going to convert this amount.
[00:00:25]
>> Maximiliano Firtman: Okay, so I'm going to convert the amount, and actually I should do an echo with the result, and you have US dollars, and then result.
>> Maximiliano Firtman: That's my goal, okay? When I convert, I should check errors, and there are a lot of other things that they should verify here.
[00:00:52]
But that's kind of the idea. So I create a converter object, an instance of my class, and then I call the convert function. The convert function or method is this one, it's public, so I can call it, it receives a value, and it will try to do something with that value.
[00:01:10]
So what do I need to do? I need to go to the network, okay? That sounds like a lot of work, right? So, I don't know how to do that. Well, actually PHP is too simple, but really too simple. So what I need is I get the the JSON object as text first.
[00:01:33]
Remember we are using an API. The API is here, and this is text, okay? So we wanna get this. So for that, we are going to call a lower function that is called file get contents that receives the file name. And you are thinking, hey, Max, this is not a file.
[00:01:57]
We wanna go to the network. It doesn't matter. If the find name starts with a protocol, it will use the handler for the protocol. If it start with HTTPS, it will just go and download the file from the web as simple as that. Where is my URL? I have it in this file, URL- It's PHP.
[00:02:20]
So should I copy and paste? I mean I can. But also I can include, that's fine global variables here, okay? So, you can do that or it's just one line. So in this case I can just copy that. But remember you can include. This is currently the URL.
[00:02:40]
And instead of crypto, I need a code. Let's call it code, and I will say that the code is actually my currency code. So this currency code. I'm sure why it's adding me the dollar sign there, that shouldn't be there, okay? And file get contents will get that URL.
[00:03:07]
>> Maximiliano Firtman: Does it make sense? Kind of, the code. Well, let's try it. So what, can I echo the JSON? I can, I'm not saying it's gonna look nice. But that means we can see if something is happening. So I will say that I have two Bitcoins convert. I'm getting an error.
[00:03:26]
It says culture undefined methods converter convert in blah, blah, blah. So let's see. You can see here the double column that I mentioned before. That means it's the method of the class. Okay? Yeah, that's it.
>> Student: Is your class called Crypto Converter?
>> Maximiliano Firtman: Exactly. My class is Crypto Converter, not Converter.
[00:03:47]
But you say, but the filename doesn't matter.
>> Student: Yeah, right.
>> Maximiliano Firtman: Okay? The filename, it's not like Java. In Java, the class must match the file name. Here, do whatever you want. So maybe here we enter the field of best practices and maybe to avoid these kind of problems, it's a good idea to maybe match the name of the class In the name of the file.
[00:04:20]
Okay, we are not forced to, but maybe it's a good idea to avoid this problem. Make sense? Yeah? But, Java, for example, will force you, will keep you under the tracks. But here, now it's up to you. So it's up to you if you know. Now I need to go here and change my required ones, by the way.
[00:04:43]
Now require once, let's try now it should complain. So, if I require once, it's not working, okay? So, you can see it's not working because it's required, but if it's include, it will just continue. And of course, then the class will not exist, so it will give me error at some point.
[00:04:59]
Okay, so anyway now it's crypto, or I can use what I said before, I can just import classes PHP, do whatever you want. So I can import classes, and then you can go back to classes, and say that this is a PHP that for now it's including ./classes/CryptoConverter.php.
[00:05:24]
So then I say class, every time I create a new class, okay? I need to remember to add it here. This is one design pattern. I've used that pattern a lot, by the way. There were other design patterns that are more they feel more magical. In fact, they were called Magic Methods, but also they may lead to some other problems.
[00:05:49]
So that's why, yeah, you should use that those magical methods with care. Okay, so let's go back now to convert PHP so. Now it's crypto converter, and now it's complaining the converter converter converter, let's see why it's comparing here sometimes the it depends the linter you're using here in bs code sometimes, it's not the it says assigning boil from a function, so what it's saying it's a warning saying hey you know what convert that function.
[00:06:22]
Convert this one we have here is not returning any value, which, yeah, you are right, because I don't have any return. So this the linter is an static analyzer, so it's really your code and see, hey, you're executing a function and assigning a value, but that function is not returning a value, okay, so warning.
[00:06:43]
Yeah, I didn't finish, that's why, okay, it's not working. But for now, we can just say return zero, so. And also you can say that convert will return float. Remember, in that case, we use colon. In function declarations, we use colon with the type. Okay, so now it shouldn't complain anymore.
[00:07:04]
Now it's not complaining anymore. Does that make sense? Yep. Cool. Well, now refresh, continue. Now, well, look at that. What is that? The JSON from the other website, from the API. So PHP is downloading the string, so that part is working. Make sense? The next step will be to parse the JSON.
[00:07:32]
Let's see how to work with JSON in PHP. Now we have good news. It's actually pretty simple. It's much simpler than any other language. I could say. So once you have the JSON, so let's open the CryptoConverter.php, to actually convert that into an object, an actual object, actually it's going to be converted by default into an array.
[00:08:00]
Because remember in PHP, a map or a dictionary, that structure is actually an array, and we use string keys for the array. So we just say JSON, underscore, and we have two functions, decode and encode. Which one do we need to use? Do we wanna decode the JSON or encode the JSON?
[00:08:25]
>> Students: Decode.
>> Maximiliano Firtman: Decode, so we need to decode that JSON and we will get the results in another variable that we can call that data, something like that. JSON decode, we should check errors, right? Because, what happens if the URL doesn't exist? Well, we should check the documentation of file get contents.
[00:08:47]
What happens with that when it couldn't read the file or the URL? The documentation will give you the answer at some point, but it says false. On failure, it will return false. So maybe I can check if JSON is not false or if it's true if it's truthy this can do this, this is gonna be so see and you say well but I need if I put the return here within the if also if you want.
[00:09:21]
You say not false. I know it's the same, but sometimes it's more straightforward to read that there. So now my function is complaining. Do you know why? It's complaining about the return. I don't even need to see the error message. Its It's about that.
>> Maximiliano Firtman: What happens with the else of this if?
[00:09:48]
I'm not returning any value, so I still need to return something on else, okay, or after the if, I shouldn't have any path. That returns no values. So I don't know. We need to specify can I return false and saying I can do that. I can because it can treat it as a float to zero.
[00:10:16]
But this is an advanced part of PHP, don't tell anyone I'm explaining to you in fundamentals, but you can use union types in PHP. So you can also say, you know what, it can be float or bull. That's it. TypeScript has something like that as well. So then you can say, well, you know what, it can be a float or it can be an integer, a float or a bull.
[00:10:40]
You use, or, okay? But if not we can talk about exceptions later and you can throw an exception if you want. We have try catch and all that stuff. But I don't wanna add too much stuff here at the same time. So let's finish this. So we have the JSON and now we have the JSON parsed, so I can do echo of data to see if, what is that.
[00:11:07]
So instead of the string, I should see something parse. And now I say, oof, I can convert STD class into string. You say, where is the error? The error is on line 21 the error is actually in my echo. I cannot do an echo of an object, because it's too complex.
[00:11:27]
It doesn't know what to do. Do you know any other way to render the contents of a variable and see its content? I mean, you remember, maybe, because it's var_dump. So we can do a var_dump and see what's inside that data.
>> Maximiliano Firtman: And this is what we have an object that the object is find associative array with all the properties.
[00:11:56]
Is that the JSON? Well, actually it has the same data, but it's not JSON.
>> Maximiliano Firtman: So actually, it's a parsed JSON, it's the result of parsing the JSON. Make sense? So now I need to extract some part of that data. I said that I won the last. So I will say, data last, like an array.
[00:12:20]
And I'm going to return. It should be the value multiply by last, right? Because we are parsing the amount of Bitcoins, we are going to multiply by the rate. Does that make sense? Yep? So, If I refresh now, cannot use option of type class as an array. Okay, so because it's an object.
[00:12:48]
Let's try using it as an object then, not as an array. I think, let me see, yeah, so if you have two Bitcoins, you have a lot of money, actually, right? So now let's go back and try a different thing. I have ten ethers convert. You have less money, okay?
[00:13:12]
But it's still. It's a good amount of money. That is k.
>> Maximiliano Firtman: Will it work with decimals? I have. Bitcoin, yep. Remember, this is no client-side. This is actually working everything is in the HML server-side render.
>> Maximiliano Firtman: So that's how that looks like. So, in this case, we mix a couple of stuff.
[00:13:42]
We created an object. We use OOP. We downloaded something from the network. It was just really weird file get context. It just works. Then we are parsing JSON to an object. We can also parse into an array, and then I'm using the object. Remember. For objects, we use the thin arrow syntax.
[00:14:05]
We also see how to specify types, return types, argument types, and so on. Do we need a class for this? No, I wish I were forcing it an option for this, you can just copy this inside a function you don't need a class, okay? But just to play with the class here, we are receiving the currency call.
[00:14:26]
Remember this is a shortcut. This is creating a property, even if I don't see it's a property, okay? Just remember we have a property there and we are receiving arguments.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops