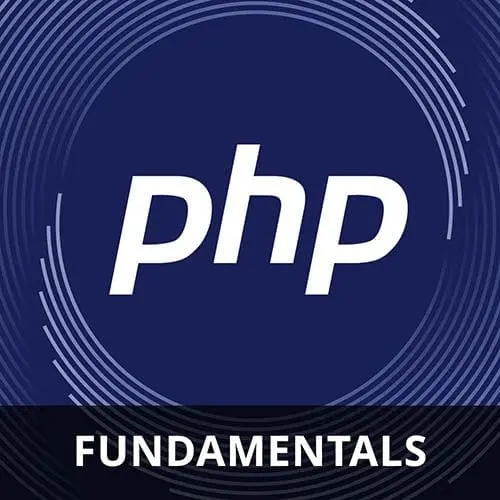
Lesson Description
The "include & require" Lesson is part of the full, PHP Basics course featured in this preview video. Here's what you'd learn in this lesson:
Maximiliano demonstrates how to use the include method to import a class into another PHP file so the class can be instantiated. This lesson also discusses the difference between include, include_once, require, and require_once.
Transcript from the "include & require" Lesson
[00:00:00]
>> Maximiliano Firtman: We have now a class, our class converter. We've seen then constructors. Distractors, we mentioned they are there. A class can also extend from another class. For example, I can extend from Converter. That doesn't exist. So I can create another class here called Converter, that can include some properties or methods, and this one is extending from the other one.
[00:00:24]
With everything you know about OOP, so in this case, it's following Java rules, so you can call your parent. Here it's not called super, it's parent. So when you're in a class and you wanna call something from the super class, you use parent, the same thing. You can also implement interfaces.
[00:00:45]
So again, if you are coming from Z-Sharp or Java or languages with interfaces, you can implement interfaces that are like protocols, where you say, well, I have an interface that will, no, no, we can call interface CanConvert, for example. The interface CanConvert will have a function, convert. So then, without code, right, something like that.
[00:01:12]
So now, we can have a class that will implement that interface, which in that case, the interpreter will force me to get code for that function, okay? That's the same. I mean, if you're coming from JavaScript, maybe you haven't seen the interfaces, but if you're coming from the standard OOP, probably you know what I'm talking about.
[00:01:35]
It can be called protocol in other languages, such as Swift. It's a protocol in Swift and so on. But for now, let's say that we are good with that for now. So what's the idea? I wanna go back to convert.php, and somehow, after we had the amount of the crypto, I wanna create an object of my converter class, okay?
[00:01:57]
Make sense? So we did an object, so we can use it. So then we say, well, I'm going to create the converter, new Converter, and it was receiving as an argument the crypto code that we have in crypto. But we have the first problem, we are encountering our first problem, I have an error there.
[00:02:15]
The error is because convert.php doesn't know about converter. It doesn't know what it is. So if I go to my problems, remember problems in VS Code are here at the bottom. It says,'Class Converter' does not have any constructor and shall blah, blah, blah, blah. So actually, you say, yeah, it has a constructor.
[00:02:38]
Yeah, look at it, it's there. My constructor is there. But the problem is that it doesn't know about the other file, because each file is an individual module. It's not reading the rest of the PHP files, even if it's in the same folder, which is just a difference compared with Java.
[00:02:57]
Maybe if you're coming from JavaScript and ECMAScript modules, yeah, maybe you understand what's going on, because, yeah, maybe I need to import that class somehow, okay? Well, I have good news and bad news. Not sure which one do you prefer first, but the bad news is that we don't have modules here, so we cannot export and import elements.
[00:03:21]
Okay, that's a bad news. So you cannot pick what to export, and from the other side, you cannot pick what to import. Okay, that's the modules. Design pattern is not here. The good news is, yeah, we're gonna still do something. Of course, we don't need to write spaghetti code in one file.
[00:03:38]
Of course, I can copy and paste the class declaration, okay? But, yeah, doesn't seem like a good idea. Also, so far I'm putting everything in the same folder in the root, maybe I could like to create a new folder. We'll talk about that later anyway, but I can create this in a new folder called classes.
[00:03:56]
So then I put my converter there, because I feel it's better, right, to organize my code. Can I? Yes, but also, because this php, it has the same type as this php. So can I access this one directly from the browser? But it just a class declaration, I wasn't expecting that to happen.
[00:04:20]
But actually, it can. Will it do anything? Well, actually, it's just finding a class and nothing else, so actually, no. But later in your web server, that's not a PHP problem, in your web server, you can say, no, the classes folder is not visible to the web, okay?
[00:04:37]
But that's a web server problem, not the PHP problem. But anyway, we are here, and I need to import or do something to get the other part. Well, to do that, you need to call anywhere in your code. I will probably do it in a different place, but let me do that here now.
[00:04:58]
There are many ways to do that. I will show you all of them. There is one called Include. Include is like echo. It's a function that you can call with or without parenthesis, okay? So it's up to you. So I can include classes/converter.php, with or with app parenthesis, up to you.
[00:05:22]
What include is going to do, well, include is going to go and grab that file, and it's like a copy and paste, to be honest. It will grab everything that is in the other file, and it will include it here. And most of the time, we put all these Include at the top, even sometimes here before the HML.
[00:05:48]
So we add all the includes that we are going to use later, okay? Make sense? So that pattern is pretty common. So, well, include is copy and pasting. And what's the problem? That if you include more than 1, we have a problem, because it's copy and pasting the file twice.
[00:06:13]
And that means it's going to try to redeclare the same variables, the same classes, and you will get errors. And you say, well, who is going to do that? Well, maybe, you have an index,php that is including services.php and it's also including a component that will render a list of countries.php.
[00:06:34]
services,php is including also a database.php. But you know what, countries.php is also including. So there is an inclusion tree here. Everything goes to index.php at the end. And maybe you're not including in index.php twice the same file, but at the end, you will end up with two dbs, so you have a problem.
[00:06:57]
Does that make sense?
>> Speaker 2: Is db.php, that's the same in both of those, or?
>> Maximiliano Firtman: It's the same, yeah, I'm talking about because they're accessing the same database. Let's say it's just a pseudocode here. It's just an idea, right? But I'm just explaining that include may have a problem, okay?
[00:07:16]
That if it's a complex website that you're importing something and dependency, dependency, dependencies, if the same dependency appears twice in the tree, you will get errors. So to solve the problem, there is another option. That's why I'm showing you this. The other option is include once. So you can go to convert.php, and instead of include this, you can include_once.
[00:07:47]
So include_once will not include that file again if it was already loaded for the current script. And maybe you're thinking, why not using include_once always. Performance reasons, because including, that is faster, it doesn't need to check anything.
>> Maximiliano Firtman: Okay, make sense? By the way, here, we are talking about relative folder.
[00:08:14]
So you can also do this if you want. So you can go from here to classes to converter, or you can go up one level. From the current folder, you go up, okay? Remember, you can include here. There's no need to include it at the top. You can include at any time, so maybe here at the top of the script.
[00:08:34]
That means that you can start using your thing. Okay, so another way, what happens if you don't have the file? If you don't have the file, it will generate a warning. But in production, typically, warnings are never shipped. So users won't see any error, nothing, okay, in production.
[00:08:59]
In development mode, typically by default, warnings are enabled. But you say, no, I really want that. So I wanna make sure, I don't want to continue executing code if I cannot load that file. So instead of include, we have require. The only difference, a require will trigger an error, runtime error, runtime exception, instead of a warning, if it cannot load the file.
[00:09:28]
And because we have require, we also have require_once that is doing the same thing as include, but enforcing that the file is there. Does it make sense?
>> Speaker 2: Is it possible to include just specific components from there?
>> Maximiliano Firtman: Unfortunately, no. That was the bad news.
>> Speaker 2: Yeah.
>> Maximiliano Firtman: You cannot just include a part of that file.
[00:09:52]
If you need that, you need to split that file into separate parts, okay? So we don't have the modularization design path that are implemented here in PHP. So you just include the whole file. And by the way, if the other file also has HTML, it will include the HTML as well.
[00:10:17]
Like, hey, it will also be included and it will be in the output of your HTML. Because remember, PHP file is not just PHP code, it can also contain other code. Does it make sense?
>> Speaker 2: Yeah.
>> Maximiliano Firtman: Yeah? Okay, so that's how you use the content of other five PHP.
[00:10:47]
That's how you separate content, okay? In fact, it's pretty common when you have a large application. Let's say you have an application with 15 PHPs, so 15 pages, different pages. But then you have created 40 different classes. You're using OOP, 40 different classes, and you're using one PHP per class.
[00:11:14]
Think about that, then you need to import 40 files every time you need all the classes. Does it make sense, what I'm saying? Later, before the end of the of today's workshop, I will show you an advanced solution to that. But a typical trick is to create in the home, an include,php file that will include everything you need, and then you include the include file, okay?
[00:11:46]
Because you can do that. It's a tree. So then you create one, or you say classes.php, where here, you include all your classes. So then you just say, I wanna include the classes, and that will indirectly include all of them.
>> Maximiliano Firtman: Okay, makes sense? There is another solution also for that.
[00:12:07]
It's called auto load. We will talk about that later.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops