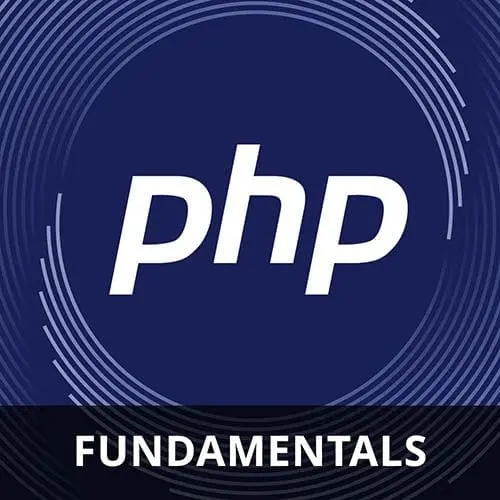
Lesson Description
The "Classes, Methods, & Constructors" Lesson is part of the full, PHP Basics course featured in this preview video. Here's what you'd learn in this lesson:
Maximiliano introduces object-oriented programming concepts like classes, properties, methods, and constructors. A class is created with a public property and a public method. A constructor is used to set the public property when an instance of the class is created.
Transcript from the "Classes, Methods, & Constructors" Lesson
[00:00:00]
>> Maximiliano Firtman: Well, now that we have the data from this side, we wanna go and talk to our API, but we wanna make the conversion, okay? So that will let us add more things on top of what we have already covered. But something that we can do also is trying to start using object oriented programming, just to add something else on top of the same code.
[00:00:28]
So let's talk about that. So let's talk about OOP. So again, what's my goal? My final goal is if you go to urls.php. If you don't have the file, don't worry. It was on the repo. You just need to type this, it's not actually big deal. But actually if you put the BTC in that part, you receive this JSON that we can pretty print here so we can see it better.
[00:00:58]
So this is the current price of bitcoin while shooting this video, 70k, actually. So this is in US dollars because I say so. Because you can also check euro and now it's in euros, okay? So you change the currency code in the URL and you receive that, so you can play with that, with different currencies and so on.
[00:01:22]
So I wanna go to that API and receive this JSON and get one value, okay? We can take last, for example. It doesn't matter. It has several values because it changed over the hour. But it doesn't matter. We can take last. And we can take that last and try to use it in our operation, okay?
[00:01:43]
So, that will need a couple of steps. For example, I need to download something from another web. So I'm a server and I'm going to download a file and I'm going to fetch a request from another server. That's the first step. And then I need to parse a JSON file, okay?
[00:01:57]
We don't know how to do that with PHP, so we will do that. But also, maybe I'm going to do that through a class to an object, okay, just to add a layer of object oriented programming to this story. So let's create a new file. We are going to create a converter PHP file and idea is you create a converter class.
[00:02:29]
It can also be called cryptoconverter. How do you create classes in PHP? This is a good one, as you're expecting, class. For example, I can call this CryptoConverter. So the syntax is pretty similar to others. By the way, I need always the PHP tag, always, okay, always the PHP tag.
[00:02:54]
So I'm able to write the class CryptoConverter. The class can contain properties and methods as you're expecting, okay? And good news, properties can include data types, optional data types. If you want to enforce data type, for example, the CryptoConverter can have the currency code as a string. So I can say currency code.
[00:03:23]
The problem is, this is a variable, so I need dollar sign. Also it's complaining here, and you say, okay, why are you complaining, okay? Syntax error, unexpected token. Because when we are creating properties, by the way, I can just stay there and it's also complaining. So why you're complaining?
[00:03:41]
It's complaining of everything. Properties can actually be, also define as public, private, or protected. It's actually following the Java [INAUDIBLE]. By default, they are public but that's for methods. For properties I need to say, so do I want the public property? That means that anyone from the outside can get it and sell it, or it can be private, and that's private only for this class or protected, that is private for the outside.
[00:04:20]
It's only available for my class and subclasses when you're using OOP inheritance, okay? Makes sense? So you can specify the type or not. And also, we have a method. For example, I will have a method that will be called convert. How to define methods? You just do something like this.
[00:04:42]
So this is going to be convert and I'm going to create a public method, okay? Something like that. I will make some small changes here and there while we are coding this.
>> Maximiliano Firtman: When we are doing methods, we can also specify that the return type with column. So I can come here and say, hey, I want to return, in this case, a float.
[00:05:16]
So I can say, hey, I wanna return a float here. Things like that. Why it's giving me an error? Because something that we are not doing here is using the right keyword, because for variables we don't have keywords. But for functions, we do have a keyword, the keyword function.
[00:05:43]
So I still need to add the function keyword for methods, okay? Now, why do you think I have a yellow underline?
>> Maximiliano Firtman: Does anyone know why I have a yellow underline?
>> Maximiliano Firtman: Because I said that I'm going to return a float and I'm not returning one yet, okay?
[00:06:11]
Remember, this is no mandatory. So adding the return time is all mandatory, but if you add it now, you need to comply with it, okay? So it's up to you. So you define types, data types or no, remember, types are a string, array, bull, int, number. That's kind of all, okay?
[00:06:31]
In terms of basic types. Well, actually this convert needs to go to the network and download the JSON, okay? That's kind of what we're going to do. But before doing that, by the way, convert should receive a value, maybe.
>> Maximiliano Firtman: A value, remember, it's a variable. And I can say it's a float.
[00:06:58]
The code should be a string. So if you want, you can specify that the code is a string, it's up to you. Okay, if you wanna enforce that it's a string, it's not mandatory, okay?
>> Maximiliano Firtman: What are the things we have typically in a class? A constructor, right?
[00:07:16]
A constructor. How do we create constructors in PHP? By the way, PHP has constructors and destructors. Say, wow, that's not common, okay? So unless you're coming from C++, that's actually not common. So the constructor is going to be executed when you create a new instance of that class and the destructor is going to be executed when the garbage collector is about to destroy that object.
[00:07:48]
PHP is using garbage collector. Do you know what a garbage collector is? When you create an object in memory, by the way, JavaScript has garbage collector as well. Java has garbage collector. The C-sharp, not C++. For example, in C++, when you create an object, you are responsible for de-allocating the object, because if not, you may leak that memory.
[00:08:09]
You have a memory leak. So, in this case, we have a constructor and a destructor. That's kind of the idea. How does that work? Well, actually, they are functions, so if you. Let's go just PHP manual, we go to classes and objects, there is a whole section on.
[00:08:31]
There are a lot of things that we won't cover because we can take a whole week to talk about constructor and destructor. The constructor is just __construct. So what? So we just say __construct. So everything that is, oops, I have a typo, it's construct, okay? So we're not going to use the destructor, by the way.
[00:08:58]
Most of the time we don't need it. It needs also the function declaration, okay? That's different from other languages. We create the function__construct, and then specify exactly, for example, we may receive the currency code as an argument, as a string, and we wanna get this into that one, a typical use case.
[00:09:31]
So we're going to say, maybe this, do we have this in PHP? Well, we have dollar this. And how can we access the currency code, .currencyCode, .$currencyCode? Give me a sec. Actually, the way to access a property is not the .syntax, it's the thin arrow syntax. So object, thin arrow, property without the dollar sign, the property without the dollar sign here.
[00:10:07]
And we assign the currency code. This is called the thin arrow. We have the thin arrow and the fat arrow. So we use a thin arrow for accessing properties and methods of objects. Yeah, I'm sorry, okay? That's how it works. Objective C is worse, so there is always a worse solution.
[00:10:29]
Just in case you have experience with Objective C, it's worse there. So if you wanna access properties and methods of different classes. I'm going to do that now here just for testing, but how do you create that object, fortunately? Simple, you create an OC and you say new CryptoConverter as you were expected.
[00:10:51]
And you pass the currency code, okay? And remember because it's a function, you can use position arguments or named arguments. So position argument will be, well, it's BTC, if you want you can also specify the name of the argument. That is currency code. So you can say currencyCode:BTC.
[00:11:15]
Both are exactly the same, okay? That's new on PHP 8. And something that's new that I'm pretty sure that you will like, because that's pretty cool and also it's available in TypeScript and other languages. When you have this pattern of, I have a property, and then I receive that property in the constructor.
[00:11:36]
So I need to assign this. We can skip one step, and I can say public string currency code here, and then I don't need this declaration. So I can remove the property from here, and I don't need the assignment. You don't need to do it, okay, if you don't want to, but let's see if you understand what's going on now.
[00:12:01]
Now, I'm adding the public keyword in the constructor signature itself. This is new in PHP. I think it's 8.2, so it's actually really, really new. So then that means I want to receive a currencyCode deconstructor, and the only thing I want is to save it in the Docker property.
[00:12:23]
I will do that for you.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops