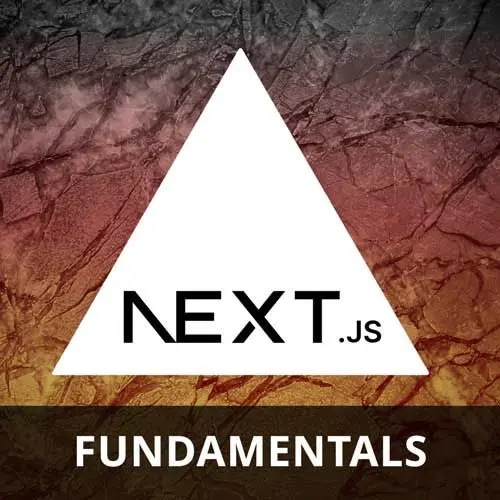
Lesson Description
The "Testing the Dashboard Page" Lesson is part of the full, Next.js Fundamentals, v4 course featured in this preview video. Here's what you'd learn in this lesson:
Scott demonstrates creating tests for the dashboard page and writes test cases using assertions to check if the expected elements are rendered on the page. Vitest is a popular testing framework in the Next.js community, but other frameworks such as Jest can also be used.
Transcript from the "Testing the Dashboard Page" Lesson
[00:00:00]
>> Scott Moss: Let's create some tests, these are pretty boring. We're going to create one that is specific to Next js. All the other ones are just straight up regular tests. So let's create one specific to Next js so you can kind of get the vibe for that. So we'll create a test for the dashboard page, and I think that'll give you a hint on how this works.
[00:00:22]
So what we'll do is we'll go to dashboard, we'll make a new folder in here. I'm going to call it __test__, you don't have to do this you can just literally just make a test file. But I'm going to do that and I'm going to say page.test.tsx or sorry, .ts.
[00:00:54]
And it's important you do page.test for two reasons. One, we only told it to pick up things that have .test in it. Two, if you don't put .test, it's going to make a route [LAUGH] called __test__ and it's going to go here and think that's a page. Okay, so let's get our imports in here and we'll go step by step.
[00:01:25]
So we have some helpers here to help us like render things and then observe the screen. These are the globals that I was talking about, we don't actually have to import this because I did globals too but we're going to import it anyway. Here's the page that we're going to test.
[00:01:39]
We pull this in because this page calls this, we're going to mark it out. It also calls get user so I might just delete that because I don't feel marking that out. And then just some types and stuff to help us. Cool, first thing is let's mark out stuff specific to this test.
[00:01:51]
So we didn't add this to the global because it's specific to this test so we can grab these three.
>> Scott Moss: This thing's freaking out because, why are you freaking out? Did I set that up though, in the config to DTSX though? I did, okay, I did, I did nevermind TSX.
[00:02:17]
All right, so we're marking out the library call to get issues and get current user. Even though we marked out the link here, I have one specific to this test to mark the link out to do this. And then we're marking out the utils call that I was using for like cn and like I was using one of my format a time so we're going to mark all that out.
[00:02:45]
>> Scott Moss: And then from here we can just describe the test that we want to do so in this case, I'm going to say describe dashboard. And then what we want to do is before each test or each assertion block, or in this case, each it, we want to clear all the mocks, as in, like reset them.
[00:03:09]
So before each, we want to v clear all mocks and then we want to start writing some tests. So the first test is going to be it renders the issues list when issues are available. So,
>> Scott Moss: I feel like I spelled, I don't always I feel like I always feel available wrong.
[00:03:38]
It's like such a weird word. Cool and these can be async. So we'll make this async.
>> Scott Moss: And then from there we're going to mark out these issues because remember, we mark out the function, so it's not going to do anything. So let's grab these fake issues here like that.
[00:03:58]
We need to tell the mark that, hey, when you are called, return these fake issues. So we can do that by calling, hey, grab this marked function that we already marked out. And it's asynchronous so when it gets resolved, resolve it with this value, so this array of issues.
[00:04:16]
So we'll do that. This was already marked up. We're now saying when it resolves cuz it's a promise, return this array of issues. And the most Next js specific part in here is just this part right here, which is and I guess it's not even specific to Next js, it's just React Server components, which is just this.
[00:04:40]
So if you want to render the component. Well, technically, React Server component is just a function, it's not like a React component anymore. It's literally just a function, an asynchronous function so you can just call it like a function. This will render the component and then from there we can actually draw it on the screen, which is like this or even shorter you could just do this.
[00:05:09]
You can also wrap this in a suspense and do some stuff there as well but then you'll have to await every single one of these but that's just annoying. Cool, let's copy these assertions and we'll run this test and see if it actually does anything. So I don't know why this thing is saying that to be in the document.
[00:05:34]
I think my typescript stuff's not set up right, but pretty sure that's good we'll see. Yeah so we're checking to see if pretty much does the stuff that we have here that we think is going to be are going to show up on the page. We have an issue with test Issue 1, test Issue 2.
[00:05:52]
So we think that should show up on the page. We think these labels should show up on the page because we gave them the same labels here. All the stuff that we think should show on the page based off this data, it should be there.
>> Student 1: One thing before we run, I think the typo and the vtest setup file name.
[00:06:10]
>> Scott Moss: Say that again.
>> Student 1: The vtest.setup.ts I think it's looking for not vite like V-I-T-E test.
>> Scott Moss: I mistyped it.
>> Student 1: I just think in your code.
>> Scott Moss: Yeah, I did.
>> Student 1: Yeah, I think it's just.
>> Scott Moss: Okay, let's try to test this and see why that thing is messing up and then use the npm test.
[00:06:26]
It did mess up because it said dtest setup ts does not exist import. I may have forgot to install this. Let me try to install this, let me see. Pretty sure I installed that and then vtest.setup.ts that looks about right to me. There we go and this time it passed.
[00:07:00]
So we can make sure this is working and go in here I guess it's working now. And we can say expect that to be on the document and obviously that fails because that's not on the document and here's the actual document.
>> Scott Moss: Cool, any questions on testing?
>> Student 2: Is there a big difference between vVest and Jest or any other test runner?
[00:07:28]
>> Scott Moss: I don't know who started it, if it was Jasmine or Mocha or one of the OG testing frameworks. And well, I guess they all inherited from Ruby at some point. I think they all came from Ruby but as far as Syntax goes, there is no JavaScript testing framework that is gonna deviate from this syntax.
[00:07:48]
It's just not gonna do it. So they all have the same syntax, they all do the same things, they just do them slightly differently. So as far as the differences, sure, there's definitely differences in how you set it up, the things you import. But the feel of it, because they all use the exact same syntax is pretty much the same thing.
[00:08:08]
You just got to find like what is the Jasmine way to do this? What is the Jest way to do this? But Jest is the same way. You set up some describe blocks, you can do some before each, you do some marking, it's all the same thing. I just think the community is backed Vtest.
[00:08:24]
It's faster, it's got more support and it has better development at this point. So yeah, but you can use Jest if you OG, want to use Jest, go ahead. Any questions?
>> Student 2: The testing folder name __test, should that be or can that be plural? Someone's just asking if it's test or tests plural.
[00:08:50]
>> Scott Moss: It could be whatever you want, it's whatever you put in your configuration. So for me, I'm just looking for anything that has a .test or .spec in it. So it doesn't even care about this folder, it doesn't even see it by default, if you don't configure it.
[00:09:04]
I think it does look in this test file for specs. But yeah, you can call this whatever you want. I just put this here because I know that Next js is going to pick up routes and I want someone on my team. When they see this, I don't want them, for instance, let's say we actually had a route called test.
[00:09:25]
Okay, now what? [LAUGH] This is a route, if I see this, I know this is not a route, this is testing. So that's why I do that and plural, sure, you can make a plural if you want.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops