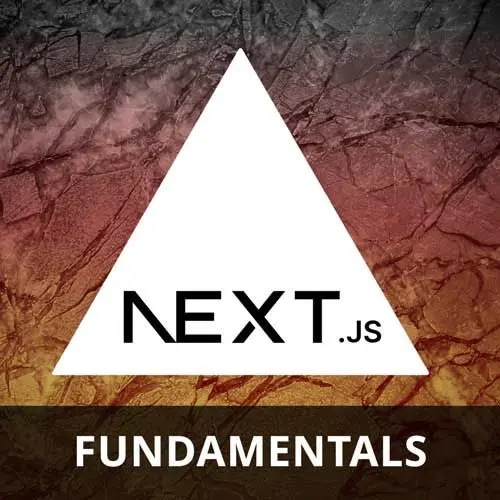
Lesson Description
The "Pages and Routing Exercise" Lesson is part of the full, Next.js Fundamentals, v4 course featured in this preview video. Here's what you'd learn in this lesson:
Scott demonstrates how to use the Link component instead of an anchor tag for client-side routing. Scott emphasizes the importance of returning something in each route component and explains how layouts work by rendering their children.
Transcript from the "Pages and Routing Exercise" Lesson
[00:00:00]
>> Scott Moss: All right, so, what we're gonna do now is we're gonna make all these routes. So, for our app we need, I mean, I pretty much put it all here. We need a root layout, if we don't have it already. We need a auth route group with a slash, sign in and signup.
[00:00:15]
We need a marketing group for the marketing pages. We need a layout inside of there and we need at least the index. There's a couple more pages if you wanna make them, but they're so redundant I didn't feel like putting you guys through it. We need a Dashboard route.
[00:00:31]
It also has its own layup and its layup layout and its own root page and then issues. We have one for new, and then we have this dynamic issues with an id. So, looking at this, go make all these routes. What you should do if you want this to work, is when you go make these routes and we're gonna make it together inside the pages.
[00:00:53]
For each one of these routes, you have to have a component that returns something. It can return null, but it has to return something, and it'll break if you don't. So, let's make these routes. First thing is our root layout that's already made, so, don't have to touch that, gonna keep that here.
[00:01:14]
The second thing is our marketing. Since I just made this, I'm gonna go ahead and play off of it. I'll get rid of this about, we have a layout here, so, I'm going to. Wait, does marketing have layout? Marketing does have layout. So, I'm just going to say, so, we have our layout.
[00:01:33]
And the one rule here is you have to default export your pages with Next.js. So, you can do it like this, you can do a function declaration. There's no wrong way to do it, but it has to be a default export for pages. For components or anything else, it doesn't matter, whatever you want to do.
[00:01:51]
But for something that ends in a layout or a page or one of those special files that I talked about, you have to do a default export. That's how it knows what component you want to be recognized as the route. So, in the case of a layout, like I said, you can return whatever you want, but because it is a layout, you just need to make sure you return.
[00:02:12]
You render the children in here, right? So the children is gonna be props that you get in. So, that's the hello world of a layout. It's just render the children. Cool, and then for the marketing page, just going to hello World. For that, this is literally the roots.
[00:02:32]
This is what you would see on our landing page if you went here. So I'm just gonna call this home. Call whatever you want, and I'll just render home like that. Pretty simple, just got to return something here. So, if we were to start this and try to go here, assuming the rest of the app isn't broken, we should be able to see this.
[00:02:57]
So, I'm going to run this. I keep wanting to run bun so bad. Start that. Cool, and I see home, right? So, I'm in my layout. My marketing routing group has a layout and then that layout gets put in a layout. Okay, so we got that. We need to make a directory for Dashboard.
[00:03:27]
I'm gonna say Dashboard like that. It also has a layout, so I'm gonna say, layout.tsx and it also has a page. So I'm gonna say page.tsx like that. And same thing. I'll just say Dashboard layouts. You can call these whatever you want. I noticed that if you use function declaration in the error stack, you'll see the name of it show up.
[00:04:06]
Whereas I think with this, I don't know if it shows it in error stack, or maybe they fixed it, but it didn't used to. But that might be the only difference I can think of. Again, because it's a layout, we just need to make sure we render our children.
[00:04:18]
If you don't render the children here, what do you think you would see in this layout?
>> Student: Nothing.
>> Scott Moss: Yeah, you see nothing. You just see the layout and not the route. So, the whole point of the layout is just to pass through it's children, the active route.
[00:04:34]
So, you have to do that.
>> Scott Moss: And then for the page, same thing.
>> Scott Moss: Cool, so now we got our Dashboard page. So this should allow me to go to slash Dashboard and I should see Dashboard. Let's see.
>> Scott Moss: Cool, see Dashboard.
>> Scott Moss: All right, what else do we need to make?
[00:05:15]
We need to make the issue stuff. So the issues, one, we need to make a page for slash new and then a page for slash id slash edit and then the auth stuff. So let's do that, I'll start with the off stuff. So, for all stuff we need a route group called auth, and then, inside of here we need two folders, one called signup.
[00:05:35]
So get in a habit of like if you need to make a route, you have to make a folder and then you have to make the file, right? Because in the pages directory, it was different. The pages directory, the name of the file was the route that you were gonna make.
[00:05:47]
So, you didn't have to make a route.tsx or whatever, you would do signup.tsx or sign in.tsx. The name of the file would have been the route. In the app directory, the name of the route is the directory name, and the file is always route. It's always gonna be route.tsx, no matter what the path is.
[00:06:05]
The path is the directory name, not the file name. So in this case, I have signup, I need to also make sign in. So these are two different directories. They both have their own route or I'm sorry, page.
>> Scott Moss: And I'll just say, const signup.
>> Scott Moss: And just return literally whatever you want cuz we're gonna make all this a reality.
[00:06:46]
I'm just gonna copy that, take it into sign in and just replace it all with sign in.
>> Scott Moss: So this should allow me to go to slash. Is it slash auth, slash sign in, or.
>> Student: Just slash sign in?
>> Scott Moss: Exactly, just slash sign in. Because that's a route group, it's not part of the URL, right?
[00:07:17]
If I got rid of these parentheses, it would be auth sign in, and that's just tacky. So, don't do that. Use the route groups, be sophisticated. Elevate yourself and your team. [LAUGH] And then, issues, so, new folder. This one we do want in the URL. So I'm gonna say issues.
[00:07:43]
And then, two new subfolders in here. One's gonna be called new, another one's gonna be called id, so it's dynamic. And then inside the id one is another folder called edit, cuz we wanna be able to edit an issue by the id. And then inside of that one we want a page.
[00:08:04]
And then the same thing inside of new we want a page.
>> Scott Moss: Cool.
>> Scott Moss: And got to put some stuff in here. So, I'll just say, edit issue page.
>> Scott Moss: Cool, got that. And then, same thing with the new page. Just gonna copy and paste, new issue page.
[00:08:44]
No.
>> Scott Moss: All right, and with that, we should have all of our routes set up. Like I said, there are some more in the marketing, I have FAQ pricing, all that stuff. You wanna set that up, you can set that up, it's the same, it's all the same.
[00:09:02]
I didn't wanna force you to do all that. So now, I should be able to go to issues slash new. I see that, and then I should be able to go to slash issues literally anything cuz it's a dynamic variable, slash edit. Then I should see the edit issue page.
[00:09:28]
>> Scott Moss: Cool, notice we didn't have to stop our server to introduce any of those changes Next.js has a really good build system that does hot module replacement. And all the things you've come to expect from a decent tooling setup, you get that for free, so it's pretty good.
[00:09:44]
You don't really have to do stuff, even if you change the environment variable file, it will recognize that and change it. So obviously there's more to it than just this, but I would say this is like 80% of it. You can make a full app just by doing more of what I just showed you.
[00:09:58]
There's really advanced routing stuff, but honestly, I've never used that stuff in production, never had to, so I don't know what people are making [LAUGH] that they needed that. But I'm guessing the Next.js team, the Vercel team, added it for a reason, but I personally have never had to use anything outside of what I'm showing you right now.
[00:10:18]
Maybe I'm doing it wrong, [LAUGH] but this is just my opinion.
>> Scott Moss: Cool.
>> Student: Someone said the red is probably because you have.
>> Student 2: It's TypeScripts.
>> Student: The children has any type.
>> Scott Moss: What red? This?
>> Student: And it needs a type.
>> Scott Moss: Yeah, this needs type, yeah, sorry, you're gonna learn from me that I just ignore typescript errors.
[00:10:45]
So, if you're wondering why there's a red squiggle there and I'm not concerned, it's because I've just ignored typescript errors. And it's just if I want, I mean, I guess I could add, yeah, I forgot the one that they, I think it's component with children or something like that.
[00:11:04]
Component with, I don't even know the type that you would even need from react that has the specific props with children, there it is. Yeah, I could do that and then I could just come here, and I could put it, I could put it here too, actually. Say props of children and then you can add some props here, but, yeah, I just come to ignore typescript errors.
[00:11:31]
But yeah, the reds quickly is just, yeah, it's just typescript doing, it's doing its job, it's doing its job, I don't really care about it.
>> Student: App router organization question.
>> Scott Moss: Yeah.
>> Student: Just in terms of directory structure it's in your editor, it's all alphabetical and you've got actions API components along with the different directories.
[00:11:52]
Would it be possible to organize it so that you just have your routes in one area and then your components folder, for example, somewhere else?
>> Scott Moss: Yep, you don't have to put any of this stuff in the app directory, the app directory is just for routes. Yeah, so if you wanted to for instance, you can see I have database here, lessons, Lib scripts.
[00:12:13]
You could take the components and just move them out of the app directory, which I used to do. It wasn't until two months ago that I started putting in App Directory and I don't have a good reason, it's just I got bored, so, [LAUGH] I moved it into App Directory.
[00:12:27]
>> Student: Does the API directory need to be in there?
>> Scott Moss: The API directory does need to be in the app directory, yeah, anything dealing with routes or pages have to be in the app directory, yeah.
>> Student: And the chat is split, some people really care about the typescript.
[00:12:43]
>> Scott Moss: Clearly, clearly, clearly.
>> Student: Someone said, I thought I was the only one who ignored types.
>> Scott Moss: No, as you see, when we deploy, I'm going to ignore all types, I'm gonna tell our build system to ignore all types. It's just not worth it for me, there are times where I do want the types, but then there are times where it's, I didn't pass this in.
[00:13:09]
React passed this in, I don't really need to type this, I'm never gonna do anything with this, I personally don't care. But if it's something that I'm making, yeah, I wanna type that, I definitely wanna type that because I'm responsible for it. But I'm betting that react's gonna do its job in passing the children here and it's always gonna be the same shape.
[00:13:25]
So, yeah, I don't really care about typing that, I feel that should already be typed, but that's neither here nor there. So, I get it, I think it's kind of if you go to someone's house, it's what level of mess can they tolerate? Some people can only tolerate one sock on the ground, some people can have rotten food on the ground, and they can just function that way.
[00:13:47]
So, it's just for me, it's somewhere in the middle when it comes to typescript, I have my limits, but when it comes to framework specific stuff, I care less, it's not my thing, yeah, yeah.
>> Student: Is there common helper types from next for some of the more next specific typing things.
[00:14:07]
>> Scott Moss: Yeah, Next.js specific typing stuff from my experience is usually server-side things, so next response, next request, a lot of that stuff. Not really a lot on the client side because as you'll see next.js doesn't have any opinions on the client side when it comes to actually writing code.
[00:14:27]
A lot of the client-side stuff is these file structures and opinions here, and then anything else is a thing that you import that's already typed. So, they don't have specific things, they just use react on the client, it's pretty thin, yeah. Yep, Mark.
>> Mark: Does app marketing, in Parentheses, /Layout, TSX, override the app Layout, TSX or how do those interact?
[00:14:53]
>> Scott Moss: I will visually show you how that interacts, so I'm gonna go in here and let me just put something in here that says marketing. And then I'll put the children in here and then I will go into the root one and then I'll say root and then I will go to marketing, I guess, yeah, I'll just go to the root page.
[00:15:18]
Guess we're kind of already here, but so you can see right here, here's the root layout, here's the marketing layout, and then here is the actual homepage. So to answer your question, how does it interfere? It doesn't, it just gets nested, so marketing layout becomes a child of the root layout, and then the home is a child of the marketing layout, so they just become nested.
[00:15:44]
>> Student: So the root groups are still nested inside its parent directory.
>> Scott Moss: Yep, exactly, it's always, there is no way of opting out of that other than, like, trying to split instead of. The only way to really opt out of, not being inherited is to, not have a root layout.
[00:16:04]
And literally every single route that you have is part of some route group, and you don't have look right here. We don't have a page, well, actually, I'll just show you, so look, we have a page here in the marketing, right? What do you think will happen if I just put a page on the root?
[00:16:24]
[INAUDIBLE]
>> Scott Moss: Let's see, cuz now technically we have two of them, we have two home pages because this does not exist in the URL, so this technically is our index page.
>> Scott Moss: If I try to do this and go home, look at that, it decided not to render this one and it rendered this one.
[00:16:50]
So, it's gonna take precedence over whichever is the closest, so it rendered the root layout. It saw that there was an index space, so it rendered that, this just got ignored, so this is a collision, this is a route collision. So, when you start doing these grouped routes, you wanna be careful not to have these collisions, right?
[00:17:10]
So how do you know if you have a collision? Well, you have to think about what the actual route is that's gonna render when this page is routed to in the browser. So, in this case the actual route is just slash cuz this doesn't exist, so if I had another page in here, that would be a third conflict.
[00:17:27]
Had a page inside of Dashboard, that would not be a conflict because Dashboard is actually in the URL, so it'd be Dashboard. So, this is not a conflict because this exists in the URL, so the only other potential conflict could be in here, so you wanna make sure you account for that.
[00:17:41]
But if I were to put every single potential route in its own route group, if I put Dashboard in its own route group, I put issues in its own route group, I wouldn't need this route layout. The route groups would have their own root layouts, but then you'd be writing all this global stuff all over again every single time, which is annoying.
[00:18:04]
So, I think you should always have at least a root layout that reflects both your. If you are making an app that does marketing and an actual app, just have a root layout that's just bare minimums. Hey, it doesn't matter if you're on the signed-out experience or the sign in experience, they're all gonna use this title or this font or something like that.
[00:18:25]
And then from there you break it down to the specific layouts per either route group or route path, like Dashboard.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops