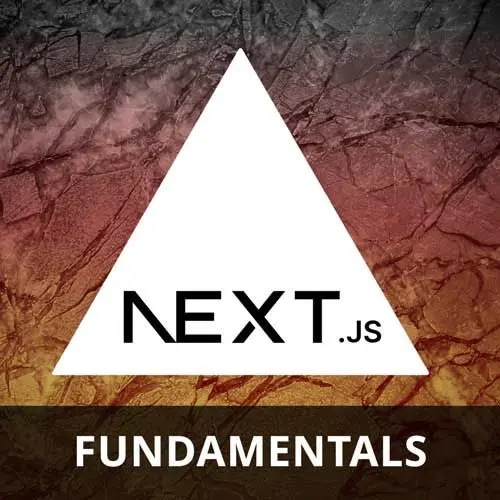
Lesson Description
The "Data Access Layer" Lesson is part of the full, Next.js Fundamentals, v4 course featured in this preview video. Here's what you'd learn in this lesson:
Scott explains that Data Access Layers (DAL) functions are utility functions that run on the server side before a route renders. They have access to the request and are used for fetching data dynamically for the page. Scott also explains how to access the request and cookies within a DAL function using the `next headers` package.
Transcript from the "Data Access Layer" Lesson
[00:00:00]
>> Scott Moss: Let's actually get this working by implementing those functions. So I need to go into, Where that's happening. So in this case it's DAL. So what does dall stand for? It stands for data access layer. You can think about it as utility functions that get used on the server side to fetch data for server components.
[00:00:24]
Not to be confused with server functions or server actions. These are just regular functions that happen before a route renders. They don't cross the network barrier, they aren't on the client, they aren't responding to interactions on the client. They happen before the browser even knows what's going on.
[00:00:44]
They're the first thing that gets executed when someone goes to a route that is a server component. So that's the difference. They have access to the same thing a server action does. They have access to the request because they're happening on a request. But they are not server actions.
[00:01:00]
Server actions are API routes that respond to some interaction on the client. There's no official name for what I'm describing to you right here, but these functions that just run on React Server Components are just functions that run before the component renders on the server. They're not responding to a button click or form submission.
[00:01:20]
I did a request to this route, before that route loads, run this function on the server. That's what these functions are. They're mostly just used for getting data. You almost wouldn't do anything mutative here, maybe outside of analytics, I guess. I don't know if you did that but this is mostly just for fetching data for the page that the person is trying to see dynamically.
[00:01:46]
That's it, so don't confuse the two. If you try to use a server action in the place of one of these, you could have potential bugs, errors, things like that. So this is why I like to keep the file separated, because in those server actions, I put useServer at the top so everything in there is a server action.
[00:02:09]
If I did that here, it would make this a server action. I don't want this to be a server action. I just want it to be a regular function that I can use on the server [LAUGH]. Not a server action that gets executed on the server, but triggered from a client.
[00:02:22]
They should come up with a name for these, but I'm calling them data access layers. So that's why it's called DAL. Okay, enough about that, and I think I go into a lot more detail in the notes actually about that, but let's just get to it. Yeah, so I kinda already talked about those things, the network boundary.
[00:02:49]
These functions run on the server, they don't cross the network boundary. They're not API routes being triggered from clients, so that's the difference. And again, they are bundled separately. This code will never ever be bundled on the client, even though we're importing them into components. But remember, the components that we import them into are server components.
[00:03:09]
You cannot import these functions that we're about to make into a component that has useClient. It won't work, you'll get an error. You can only import these functions to components that don't have useClient. If you wanna import a function that runs on a server to a component that does useClient, then that function needs to be a server action.
[00:03:30]
So you have to put useServer at the top, then you can import it. I don't have useServer at the top of this, so it's not a server action, therefore it's just a regular function that runs on Node. So I try to import that into the browser and run it, it's not gonna go well.
[00:03:46]
And anything that is useClient is gonna run on the browser. All righty, so getUserByEmail, what do we do here? Pretty simple, it actually doesn't take an email because like I said, although it's not a server action, it has access to the request, it has access to the cookies.
[00:04:08]
So we can use this function called getSession that I made, you can go look at it, but all this is doing is grabbing the JSON web token from the cookie. So you might be asking yourself, well, where is that being passed in? Next.js exposes the current request through its headers package.
[00:04:24]
So let me show you what that looks like. Let's go to getSession. So we go look at getSession, these cookies right here comes from next/headers. You can use this whenever you are inside of a function that has access to the request. So this would be a server action, this would be a server component.
[00:04:47]
This would be a route handler, this would be these data access layer functions that we're making now. All these have access to the request, therefore they can use this package. It's pretty confusing, but at the same time, that's how it works [LAUGH].
>> Student 1: One question.
>> Scott Moss: Yes?
>> Student 1: Does Next.js have some kind of middleware implemented that is managing all that exposure of data that come from the request?
[00:05:19]
>> Scott Moss: Yeah, the question was, does Next.js have some middleware that's managing the exposure to the request? Yeah, it's a combination of, it's inferred by the use of directives, right? So it all comes down to all of this stuff. It's either this,
>> Scott Moss: Or this, and this right here tells Next.js what the hell is going on, essentially.
[00:05:44]
So through the use of inferring those directives, it knows which environment something is gonna run in. And then two, in the case of directing that towards a route, that depends on where you put the code. So, as you'll see, this function right here is only gonna have access to a route, because we're gonna import it into a server component that is in itself a route.
[00:06:13]
But if I were to call this function outside of the case of a server component, it wouldn't have access to a route. You can only use those header functions if you're in the context of a call. And in this case, I will be calling this, as you'll see soon, inside of a React Server Component.
[00:06:33]
So anything that is being executed in that context has access to cookies, headers, whatever. Because you can think of a React Server Component essentially as a route handler that sends back JSX. It's the same thing, that's essentially what it is. So if you think about it from that perspective, this is just like an API route handler that sends back JSX, I can do whatever the hell I want here.
[00:06:51]
I have access to the requests, the headers, everything. So that's why this has access to it.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops