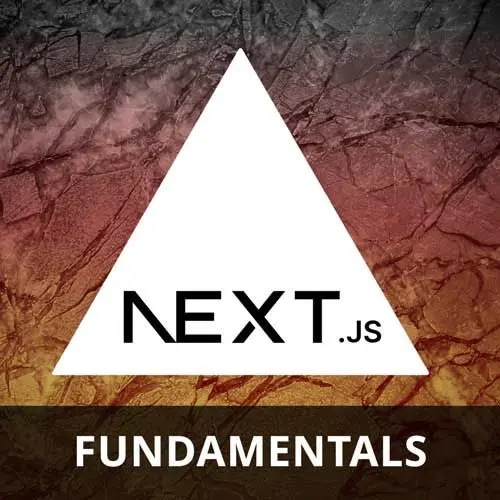
Lesson Description
The "Dashboard UI & Layout" Lesson is part of the full, Next.js Fundamentals, v4 course featured in this preview video. Here's what you'd learn in this lesson:
Scott explains the concept of suspense and how to use it to control the loading behavior of components. He also discusses the benefits of pushing data fetching calls down to the component level and using caching for static data. Additionally, Scott shows an alternative way to wrap a route in suspense using a loading file in Next.js.
Transcript from the "Dashboard UI & Layout" Lesson
[00:00:00]
>> Scott Moss: Okay, so let's get our dashboard stuff in here so we can have a nice-looking dashboard. Here we go. I just gonna grab this stuff and just paste it in, boom. Now that I have that, we should have a dashboard. If I didn't break anything that looks like this, it's still not perfectly looking cuz we still have to go fix the layout.
[00:00:30]
The layout has no styling and no components in it and stuff like that. But there we go. And then for the layout we'll do the same thing. Should be down here. There we go. We'll grab the layout. It still has our suspense right there, as you can see.
[00:00:48]
But now we're gonna get rid of that loading and we're gonna add this that I made that makes the dashboard look kinda cool when it loads in. So go to our layout. We're gonna paste that in there. Still have our suspense. And actually might even wanna comment. Well, this navigation might cause issues for now.
[00:01:07]
We'll see, but actually you might wanna comment that out for now. Actually, we didn't get there yet. So we'll do that. We'll go back, we'll refresh. It's happening so fast that you can't see a skeleton. So this is why I introduced those mock delays. So what you could do, you can go to actions, you can go to issues, I'm sorry, not actions.
[00:01:29]
Look, see, I almost fell for it. Go to right here, get issues, and you can add a mock delay in here. Wait, mock delay for like a second or something like that and you'll see the skeleton. And this is never cached. That's why we see the skeleton every time cuz it's calling that function every single time.
[00:01:51]
It is hitting that mock delay every single time. This was not the previous behavior on NextJS. If I were to refresh this previously without that flag, it would just show up instantly cuz it was cached. I had to do a hard refresh on the browser to clear it, which is great, but I wanna be in control of that.
[00:02:14]
Cool, okay, now let's see why I commented out the navigation cuz it's gonna be busted. I'm pretty sure it is or maybe we fix it, I don't know, let's see. Maybe, okay, maybe we did fix it. I thought it was gonna show an error for something, but maybe I didn't add that yet.
[00:02:36]
Did I add that? No, we already had, never mind, I did handle it, sorry. We are good to go. Cool, so this is a great use case to show you something cool here. So if you look at the navigation, it shows the user email here. After you sign in, it's happening so fast that you can't really see it.
[00:02:56]
But if I put a mock delay in here. Let's do one right here inside of Git current user and then inside of navigation. What do I have for a fallback? That's a shitty fallback [LAUGH]. Wish I had a better one, but you'll see what I'm talking about. Actually, that's a shitty fallback.
[00:03:24]
Let me show you one that looks better. If I had a skeleton, this would look better. I just don't feel like making one. Let's just do loading for now. This is where suspense comes in. So this part of the app is dynamic and then only this part is dynamic.
[00:03:38]
And I wanna walk you through how that is. But this isn't. So this loads up instantly cuz it's static, right? This page is dynamic cuz the whole page is blocked by getting issues, so it does not load up. Although we could push that down even more and load up this and this and only have this be dynamic.
[00:03:55]
We could push it, we can keep pushing it down, which is what I did here. Instead of blocking the whole navigation from loading until this came back, I pushed it all the way down just to this component is blocked, right? So you can see it says loading right there.
[00:04:11]
Just that one part, but everything else rendered. And this is where it starts to get really powerful. And this is what Next js recommends. It's like push it down as far as you can. So like before we would like if I needed to show the user in here, somewhere in this component is where I would fetch the user on the client, and I'll be fine with that.
[00:04:28]
But now as we're getting things being done on the server, you can just have just this component right here do it, right, and that's exactly what I did. And so if you go look at the navigation component, it itself is a server component and it calls suspense on this user email component.
[00:04:49]
If I go look at this user email component, it is a server component that awaits get current user. So it's being suspended until that comes back. And once it gets it, then it shows the email. So I pushed the call to get the current user all the way down to the component that actually uses it.
[00:05:09]
Whereas I could have called getuser here, but that would have suspended this whole navigation component and it would have been blocked from rendering until the user came back. So the user would not have seen that panel on the left until it fetched the user. It's not a good experience.
[00:05:25]
Even worse, I could have called getuser inside of the layout and passed it as a prop to the navigation. Now this whole page will be blocked. The user won't see anything until the user comes back. And that's what happens now in today's apps. You just see a full page loading screen until they get the data they want, then they show you the whole thing.
[00:05:46]
Whereas now you can just go piece by piece loading in the things that you need to load in. I think it's very powerful. So push down your calls to get data as close to where they're gonna be rendered as possible, suspend them if they're dynamic, use cache if they're not.
[00:06:10]
And yeah, you're gonna have a way better performing app, I think. Well, I know for sure. Before we move on, just wanted to show you an alternative NextJS specific way to wrap a route in a suspense. So I talked about two ways. So if we go look at our layout for a dashboard, we literally wrapped the children in a suspense.
[00:06:36]
I said the second way is we could have just made a component out here that basically did this and then called that component here and suspended it in here. The other way is you could just make a file in the directory called loading and that will wrap the page in a suspense as well.
[00:06:57]
So that should work actually. So what we can do is we can get rid of this. I'm just gonna take this dashboard skeleton. We get rid of this, I'm gonna put children back here. We'll probably get our error back. Well, turn it on. Here, refresh that. We'll get our error back saying like hey, you gotta pick.
[00:07:25]
So what we can do is should be able to add a loading here. So loading definitely adds a suspense to the component if it should directly wrap the page. So I'm just gonna say consts, dashboard loading. I guess I'm running out of names at this point and I'm just gonna return the skeleton and import this skeleton.
[00:08:01]
I feel like I should have auto imported but it doesn't love me. So components, that's where it's skeleton. Tripping. Okay, just, how about you do that? There we go. Okay, cool. So we got that. Now, if we go back, same thing. So loading is probably the cleaner way but just showing you how to do it without NextJS with just using React.
[00:08:47]
But if you just want NextJS to handle it for you, just add a loading file.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops