Codesmith
Course Description
Go under the hood of some of the most important aspects of JavaScript! You'll learn what you need to know to become a sought-after, versatile, problem-solving developer. Combining mental models of JavaScript's inner workings and hands-on programming challenges, this course will give you a solid understanding of callbacks and higher-order functions, closure, asynchronous JavaScript, and object-oriented JavaScript! This course is for developers with a basic to intermediate knowledge of JavaScript who wants to deepen their understanding of the fundamentals to the next level.
This course and others like it are available as part of our Frontend Masters video subscription.
Preview
CloseWhat They're Saying
I just completed "JavaScript: The Hard Parts, v2" by Will Sentance on Frontend Masters! Will is such a insightful and funny guy, making this whole learning experience quite entertaining. Can recommend. 100%.
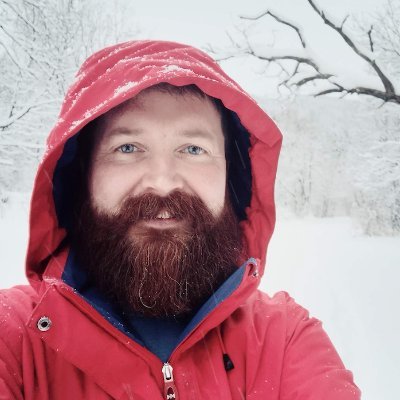
Markus August
sobergklyver
The Hard Parts of JavaScript and all the related hard parts series by Will is totally worth it.
Will Sentance is the goat.
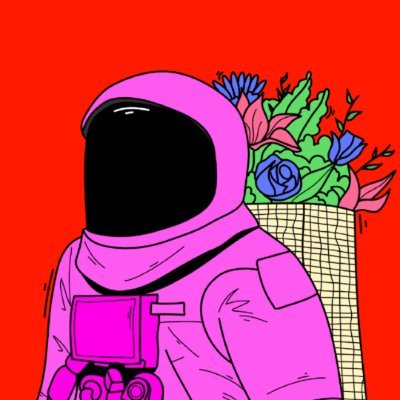
Abhinav
sehgxl
Will Sentance has the best way to describe closures in JavaScript, a rather advanced concept. Definitely recommend his course "JavaScript: The Hard Parts" on Frontend Masters.
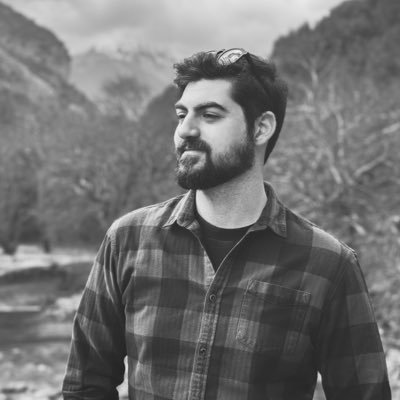
Andreas Karabetian
adreaskar
Course Details
Published: January 7, 2020
Learning Paths
Topics
Learn Straight from the Experts Who Shape the Modern Web
Your Path to Senior Developer and Beyond
- 200+ In-depth courses
- 18 Learning Paths
- Industry Leading Experts
- Live Interactive Workshops
Table of Contents
Introduction
Section Duration: 3 minutes
- Will Sentance introduces the agenda for the course, defines the purpose of the course that differs for mid level and senior level engineers, then explains how this version of the course is an improvement to version one.
JavaScript Principles
Section Duration: 17 minutes
- Will reviews how JavaScript traverses code with an example while using precise language.
- Will reviews how functions scope the thread of execution and memory.
- Will introduces the call stack to explain how JavaScript keeps track of the thread of execution.
Functions & Callbacks
Section Duration: 58 minutes
- Will uses a trivial example to demonstrate how parameters can be used to extend functionality.
- Will diagrams an function that uses an array as a parameter. Students are then directed to pair with a partner and verbalize the same diagram with one character changed to provide context a case where a generalized function might be useful.
- Will diagrams the function with one piece of functionality changed to pursue the point that this function and the previous could be restructured to accommodate both functionality cases by changing it to accept both data and instructions in the form of a function of how to manipulate the data.
- Will diagrams a proposed solution that generalizes a function to accept an argument and a function as parameters.
- Will answers a question from the audience about execution context within a for loop, and whether the function arguments are pass by value or pass by reference.
- Will defines which function in the previous example is the higher order function, and which is the callback. The previous material is reviewed by outlining how this pattern keeps the code DRY, readable, and is the backbone of asynchronous JavaScript.
- Will diagrams how anonymous functions are used under the hood when an arrow function is instantiated. The drawbacks of using arrow functions on overall understanding and readability is then discussed, and a question is asked about whether there are memory considerations when using an arrow function is asked.
- Will explains why pair programming is the most effective way to grow as a software engineer. It's outlined how another person helps to tackle blocks, stay focused, refine technical communication, and improve collaboration skills.
Closure
Section Duration: 1 hour, 17 minutes
- Will explains the many areas where closure is utilized within the JavaScript language, then goes on to foreshadow the next section by explaining how a function eliminates the state after it's executed, but that it could be useful if it could keep a live cache of data.
- Will diagrams what is happening when a function returns another function, and drives home the point that by assigning a variable to the result of a function that returns a function, that it's possible to then call the internal function by using the named variable.
- Will diagrams a function that is both defined and called within a function to pose the question of whether its where it's defined or called as to what scope it has access to.
- Will diagrams what is happening when a function is called outside of the function in which it was defined. This new function retains it's original local memory from where it was created.
- Will reveals the key functionality of closure that allows a function to have a permanent stored data cache. The caveats of the functionality are discussed, as well as the hidden property of [[scope]] afforded to functions.
- Will fields questions from the audience regarding whether there can be a wrapper such that there is another execution context returning out the first closure, function decoration, and whether variables that aren't referenced by the returned function are accessible by the backpack. Clarifications are also made on a private variables, and error handling within closure.
- Will discusses the concept of lexical or static scope as the key as to why closure works, and defines in technical terms what is occuring when closure is used. A clarification is also taken regarding reassigning variables with the backpack attached.
- Will diagrams what is happening when another instance of the original closure is instantiated and called. Emphasis is placed on whether the original instance of the closure affects the secondary instance.
- Will provides examples of where closure is used, including helper functions, iterators, generators, modules, and asynchronous JavaScript. Real use cases are discussed for clean, professional code.
- Students are then instructed to continue pair programming with closure exercises.
Asynchronous JavaScript
Section Duration: 52 minutes
- Will introduces what will be covered in the next few sections, then goes on to review how JavaScript executes code through a single thread.
- Will uses the previous example to explain what issues could be posed by having only a single thread of execution when calling to an API. The additional features on top of JavaScript that have to be introduced to explain the functionality of a setTimeOut function are defined as web browser or Node background APIs, promises, and the event loop, callback/task queue, and microtask queue.
- Will discusses some of the key features that the browser provides. To interact with the features that the browser offers, JavaScript offers "facade functions" that look like JavaScript, but are actually part of the browser. Examples of these functions include console, fetch, document, and setTimeout.
- Will diagrams what is happening when setTimeout is called, and demonstrates why it doesn't break the rule of JavaScript being single-threaded.
- Will fields questions from the audience about how the function that is used by the "facade functions" keep state, and how the call stack works when asynchronicity is introduced. These questions are used to preface the point that there needs to be clearly defined rules for how this functionality works.
- Will defines the concept of a callback queue, then diagrams an example that demonstrates the functionality of the event loop, which ties the call stack and callback queue together.
- Will fields questions from the audience about what would happen if the one of the functions were to return an augmented variable, a clarification on the previous example, and what would happen if the function's order was augmented.
- Will reviews the problems and benefits of using web browser APIs with callback functions. Students are then instructed to continue pair programming with asynchronous exercises.
Promises
Section Duration: 1 hour, 12 minutes
- Will gives context to what JavaScript was like before ES6, and explains why promises are an extremely powerful feature today. The two-pronged "facade" functions that were introduced to initiate background web browser work, then return a placeholder object to JavaScript are introduced.
- Will diagrams what is happening behind the scenes when a call to a Twitter API is made using fetch. The two pronged functionality stemming from the fetch promise is explored.
- Will diagrams the built-in method that calls methods that depend on the returned data. The rest of the example is diagrammed in full to demonstrate how the combination of fetch and then can pull data from an external API, and halt execution of methods that depend on the data until the data is received.
- Will diagrams a more complex example involving both web browser APIs and promises. The problem begins by instantiating functions into the global memory, then a setTimeout function is called, and a fetch call is made to a Twitter API. It's demonstrated how each of these things end up in the context of the web browser, callback queue, or call stack.
- Will continues diagramming the example involving both web browser APIs and promises. The promise is returned by the fetch call, and the "then" statement is reached and the function call is placed on the callback queue. The thread of execution is then blocked by a function, and a log statement is reached.
- Will continues diagramming the example involving both web browser APIs and promises. The event loop reports back that the call stack is clear, so the callback (or task) queue is addressed. The concept of a microtask queue is introduced.
- Will answers several questions from the audience regarding the returned data from the fetch function, whether functions are passed by reference or by value when passed to a web API, arguments within the function passed into the web API, what precedence objects take within the queues, and which functions go into the microtask queue and which go in the callback queue.
- Will discusses the problems of promises, including that most developers don't understand them. The benefits introduced include error handling. The features of Promises, web APIs, and the callback, microtask queues, and event loop are reviewed. A final question is asked regarding whether the async await has the same functionality.
Classes & Prototypes
Section Duration: 1 hour, 55 minutes
- Will introduces why classes and prototypes are important to learn as a developer, and what will be learned in the upcoming sections. The core idea of development is covered to understand how code should be structured, and how developers write code to be easy to reason about, easy to add new functionality, and efficient. The argument is made that object oriented programming is all of these things.
- Will provides a scenario in order to make the argument for storing data and functionality together in one place. Dot notation is introduced as a way to allow users to assign and access elements in an object, including functions.
- The built-in function Object.create() is introduced as a way to create null objects that provide more control on the object. The concept of the generalized function to produce objects is introduced.
- Will diagrams an examples of a function that is generalized to accept properties that are assigned to a new object and returned. After demonstrating how easy it is to reason about and utilize in a codebase, Will makes the argument that the approach is unusable.
- Will introduces the prototype chain, or `__proto__` as a way to access functions that were set when Object.create() is used to instantiate an object.
- Will diagrams an example of a factory function that utilizes Object.create() with the argument of a function with desired functionality to create an object with a prototypal link to the functionality.
- Will answers questions from the audience regarding whether only functions can be referenced on the prototypal reference chain, and a clarification as to whether an Object.create() argument is always the `__proto__` property. How to visually see the `__proto__` property in the console is briefly covered. The execution context created when a function called on the prototypal reference chain is diagrammed, and it's demonstrated how the implicit parameter set in the execution context allows the function work with individual objects despite being shared.
- Will diagrams the hasOwnProperty method to determine whether an object has a specific property to clarify where the property is stored.
- Will introduces the use case where a function (or several) is created on a function store, and the question is posed as to what the `this` keyword gets assigned to when nested. The call and apply method are introduced as a way to take control of what `this` gets assigned to, which is different than more antiquated methods.
- Will diagrams an example that demonstrates that the normal `this` keyword rules are overridden when used with arrow functions, because of when arrow function contents are evaluated. A question is asked about what would happen if a method on an object is defined as an arrow function.
- Will reviews the last few sections, and how they relate to the standard way of object creation in the proceeding sections.
- Will introduces the new keyword that takes advantage of JavaScript's object-oriented system to automate a factory function.
- Will diagrams what the new keyword is doing under the hood when it's utilized to instantiate a new object. It's demonstrated how, through accessing the prototype object, it can automate much of the process that was demonstrated previously in the course.
- Will introduces the idiomatic way to define when the new keyword needs to be used to instantiate an object. In addition, the syntactic sugar of the class keyword is discussed, and how it really is no different under the hood than what was demonstrated in previous sections.
Wrapping Up
Section Duration: 3 minutes
- Will concludes the course by summarizing what was covered in the course, as well as suggesting further resources and points of interest to study.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops