Java Champion
Course Description
Java is one of the most popular programming languages in the world, especially for enterprise applications. Quickly learn object-oriented programming (OOP) principles, functional programming techniques, and how to use conditionals, loops, and data structures. Inspired by Java's capacity to model real-world systems, the course offers hands-on coding challenges to reinforce everything you learn along the way!
This course and others like it are available as part of our Frontend Masters video subscription.
Preview
CloseWhat They're Saying
Definitely enjoyed this refresher of Java, definitely recommended for getting lots of examples of using the language.
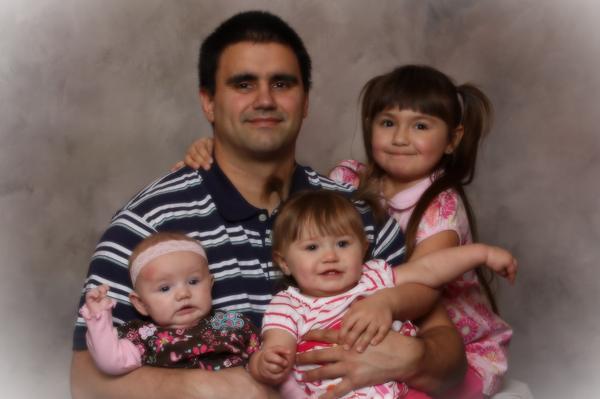
Chad Elofson
chadelofson
I just completed "Java Fundamentals" by Angie Jones on Frontend Masters! So many aha moments. Thank you Angie for this exceptional course.
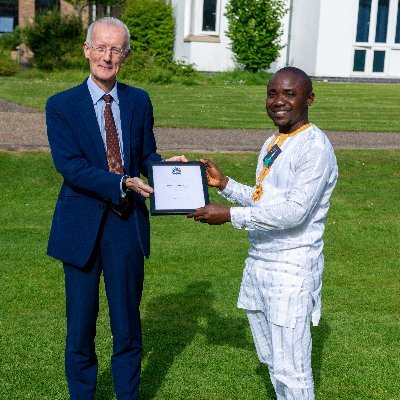
Ajayi Adegbemi
Gbemi_ajayi
Course Details
Published: May 9, 2023
Learning Paths
Learn Straight from the Experts Who Shape the Modern Web
Your Path to Senior Developer and Beyond
- 200+ In-depth courses
- 18 Learning Paths
- Industry Leading Experts
- Live Interactive Workshops
Table of Contents
Introduction
Section Duration: 3 minutes
- Angie Jones introduces the course by providing some professional background and course set-up instructions. Installing IntelliJ and Java is also covered in this segment.
Conditions & Loops
Section Duration: 1 hour, 28 minutes
- Angie walks through creating a gross pay calculator, files, packages, main methods, and Java naming conventions. The built-in text scanner class Scanner, defining variables, setting variable types, and how to make comments are also covered in this segment.
- Angie discusses the function of an if statement and walks through coding an example if statement. If statements must evaluate to true or false and will apply if the defined condition is met.
- Angie adds an else statement to the previously created if statement which will be applied if the condition is not met.
- Angie demonstrates nesting multiple logic paths in an if statement to check for a condition, and if that condition is met, then check for another condition. If-else-if statements can also be used in decisions with more than one path.
- Angie discusses using switch statements instead of if-else-if to check conditionals. Unlike if-else-if statements, switch statements check for equality.
- Angie demonstrates switch expressions, which are similar to switch statements, but allow values to be directly assigned when a case is matched.
- Angie discusses relational operators in Java, including > greater than, < less than, greater than or equal to >=, less than or equal to <=, equal to ==, and not equal to !=. The logical operators && AND, || OR, and ! NOT are also covered in this segment.
- Angie walks through using a while loop to create a program with input validation that runs until the defined condition is met. Adding extra input restrictions using the logical operator || OR is also covered in this segment.
- Angie demonstrates repeatedly running a program while a condition is being met using a do while loop. Student questions regarding valid boolean responses and if data from the loop can be used the next time it runs are also covered in this segment.
- Angie walks through creating a program that will scan a given number of items and keep a running total. A for loop will run a specified number of times and the condition is tested before entering the loop.
- Angie discusses nested loops as a loop inside a loop where the inner loop will execute once for each iteration of the outer loop. A student question regarding how to decide how deeply nested loops should be is also covered in this segment.
Methods, Objects & Data Types
Section Duration: 1 hour, 25 minutes
- Angie discusses a collection of statements that perform a task, known as a method. Parts of the method, including the access modifier, non-access modifier, return type, method name, parameter list, and method body are also discussed in this segment.
- Angie walks through writing two methods to get a user's name and greet that user and discusses the function of the main method. Methods must have a unique parameter list and can be overloaded if they share a signature.
- Angie demonstrates the scope of a variable, where variables can be accessed from, and discusses the scope of variables in reusable methods. How to define global variables is also covered in this segment.
- Angie discusses inferring a local variable's type by declaring it using var. Global variables cannot be inferred using var.
- Angie demonstrates using classes to define the makeup of an object. A discussion regarding one of Java's core principles, encapsulation, is also provided in this segment.
- Angie discusses constructors, which are structures within a class that are used to set an object's initial state. Default constructors and a student's question regarding if static classes have default constructors are also covered in this segment.
- Angie walks through writing a class that creates instances of the Rectangle class to find the total area of two rooms in a house. Student questions regarding how the HomeAreaCalculator knows to reference Rectangle and if objects can contain other types of objects are also covered in this segment.
- Angie demonstrates how to pass objects into methods and how to return objects from methods. Student questions regarding why getRoom() and calculateAreaOfRoom() are not static and if instantiating a class within its own main function is a common practice are also covered in this segment.
- Angie discusses using record classes for simple objects to reduce boilerplate code. Wrapper classes can be used to represent some primitive data types as objects and allow the variable to be operated on by methods.
Arrays & Text Processing
Section Duration: 1 hour, 1 minute
- Angie discusses how to define an array variable. Arrays can hold multiple value, but only if they are the same data type.
- Angie walks through creating a program that generates random numbers and inserts them into an array with a fixed length.
- Angie demonstrates writing the printTicket for loop using i and discusses why a do while loop was used instead of a while loop. Student questions regarding how to print on one line and if the terms mutable and immutable are used in Java are also answered in this segment.
- Angie discusses that strings are objects that contain a sequence of characters and walks through building a word count program. Appending variables in the middle of a string using String.format to allow placeholders is also covered in this segment.
- Angie walks through writing a method that prints a given string backwards. Creating a mutable string that can be modified with StringBuilder is also discussed in this segment.
- Angie demonstrates using the StringBuilder class to create a method that inserts a space before each capital letter in a string.
- Angie discusses working with multi-line strings using text blocks and demonstrates how to define them with three quotation marks. Student questions regarding what data type text blocks are and the return type of the function containing StringBuilder are also covered in this segment.
Inheritance
Section Duration: 52 minutes
- Angie discusses the inheritance of class members when a class becomes an extension of another class. Constructors in superclasses execute before constructors of the subclass.
- Angie demonstrates overriding the behavior of a method inherited by a subclass. Changing the superclass variable's access modifier from private to protected will allow subclasses to access that variable while retaining encapsulation.
- Angie walks through overloading methods that were inherited from a superclass. Student questions regarding whether constructors need to be set to public and whether there are any differences between overloading inherited and non-inherited methods are also covered in this segment.
- Angie discusses restrictions of inheritance between classes, including classes inheriting from only one superclass. A walkthrough of an example chain of classes is also provided in this segment.
- Angie discusses limited member access with inheritance, such as public, protected, and final members being inherited, but final methods cannot be overridden. When overriding an inherited method, the access modifier must be the same or less strict than the superclass.
- Angie demonstrates restricting inheritance to specific classes using sealed classes. The NonSealed keyword is also briefly discussed in this segment.
Polymorphism & Abstraction
Section Duration: 1 hour, 8 minutes
- Angie demonstrates a subclass's ability to define unique behavior while sharing behaviors of their superclass. A polymorphic object will execute its overriding method at runtime.
- Angie discusses converting an object's type into another via type casting and demonstrates how to downcast a type. When casting types, the objects must be related in the inheritance hierarchy tree.
- Angie discusses using the instanceof operator to check if an object is a specified class. A walkthrough of creating a method using instanceof is also covered in this segment.
- Angie discusses using the instanceof operator to perform two actions simultaneously with pattern matching. The scope of the newly created object is restricted to within the if statement's curly brackets.
- Angie discusses one of object-oriented programming's core principles, abstraction, using structures to represent abstract ideas. Abstract classes are designed to be implemented by their subclasses.
- Angie discusses interfaces as stateless constructs with abstract behaviors and demonstrates creating an interface and a class that implements it. By default, methods in interfaces are considered abstract.
- Angie demonstrates how classes achieve multiple inheritance by implementing multiple interfaces. An example of an interface method using the default type is also covered in this segment.
Data Structures
Section Duration: 1 hour, 10 minutes
- Angie briefly overviews data structures and discusses an unordered collection of unique objects called Set. Adding data to a Set using the Set.of method will make the Set immutable.
- Angie demonstrates how to define a collection of ordered elements accessible by position, also known as a List. Replacing items in an array list using .set and removing an item using .remove are also covered in this segment.
- Angie demonstrates how to define a queue, a collection of elements that are processed in the order in which they are added. When a new item is added to a queue, it is added to the end of the array.
- Angie discusses Maps as unordered unique key-value pairs that do not inherit from the collection interface. The put and putIfAbsent operators and how to set immutable objects are also covered in this segment.
- Angie demonstrates looping through a Set. List, or Queue collection using an iterator. Map can be looped through using entrySet() to gain access to iterator.
- Angie demonstrates looping through an array using an enhanced for loop. Looping through a Map using an enhanced loop is also covered in this segment.
- Angie demonstrates using forEach(), a built-in convenience method, to iterate over a collection. The forEach() method can be used with all of the previously mentioned data structures.
Functional Interfaces & Streams
Section Duration: 47 minutes
- Angie discusses functional programming in Java by utilizing the java.util.function package to access several functional interfaces. A functional interface is an interface with a single abstract method which can be implemented by lambda expressions and method references.
- Angie discusses streams, a sequence of elements from a data source, that can be accessed by using the java.util.stream package. An overview of intermediate and terminal stream operations is also covered in this segment.
- Angie demonstrates using the anyMatch, allMatch, and filter operations to return the desired data from an array of strings. The filter operation allows for a more precise return in that it will only return items that match the given condition.
- Angie demonstrates the stream operations map and reduce, which allow manipulation of the data within the stream. Examples using both strings and integers are provided in this segment.
- Angie walks through assigning a stream to a defined collection using the collect operation. A demonstration of combining multiple stream operations is also covered in this segment.
Exceptions
Section Duration: 55 minutes
- Angie discusses handling events that disrupt the normal flow of a program and walks through creating a program with exception handling. Printing out the error message using the getMessage method is also demonstrated in this segment.
- Angie discusses the two categories of exceptions, checked and unchecked, and the differences between the two. Check exceptions are exceptions that are checked by the compiler, while unchecked exceptions are not checked by the compiler.
- Angie demonstrates three ways to handle multiple thrown exceptions, including polymorphism, multiple catch blocks, and catching multiple exceptions in a single block.
- Angie walks through updating the previously created file reader program to close when an exception is thrown.
- Angie demonstrates updating the printer from System to PrintWriter and auto-closing both resources within the try block.
- Angie demonstrates flagging errors within code by manually throwing exceptions. The thrown error messages can be customized or left as default.
- Angie walks through how to rethrow an exception to avoid catching the exception in a try catch block.
Wrapping Up
Section Duration: 2 minutes
- Angie wraps up the course by answering student questions regarding resources for finding bugs and if there is a preference for where the print statement is located in a stream.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops