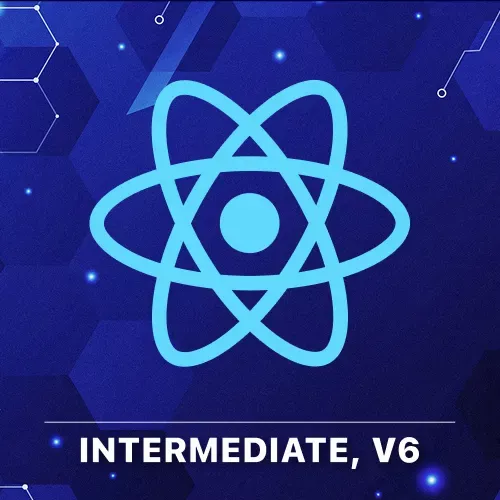
Lesson Description
The "Writing Data with Server Actions" Lesson is part of the full, Intermediate React, v6 course featured in this preview video. Here's what you'd learn in this lesson:
Brian explains how to create a "postNote" server-side function that takes in form data, validates it, and saves it to a database using parameterized queries to prevent SQL injection. He also discusses the use of the "useServer" function to explicitly indicate that the function runs on the server.
Transcript from the "Writing Data with Server Actions" Lesson
[00:00:00]
>> Brian Holt: The next thing we need to do is we need to write post note.
>> Brian Holt: So we're gonna create this post note.js, the name here is not significant, you can call whatever you want. However, this one's important because now we have to say, use server, we have to be explicit that this only runs on the server.
[00:00:27]
>> Brian Holt: So this is the only time that you need to use servers when you're identifying this function that we're doing here only runs on the server. Async database from promise polite, export default async function, postnote, and this is gonna take in form data, okay? We'll just console log here, because I think it's interesting, postnote called and with form data.
[00:01:05]
>> Brian Holt: And then here we're just gonna say const from = form data.get from user.
>> Brian Holt: If this looks familiar, it's because it's the same API for getting stuff out of a form client side and we'll just do the same thing, two, right? And we'll do the same thing here, note.
[00:01:36]
>> Brian Holt: All right, so from to a note, those are what we call them on our form.
>> Brian Holt: And you can do some validation here if you want to, so if no from.
>> Brian Holt: Or no to or no note, you just throw new error.
>> Brian Holt: With very helpful instructions.
[00:02:10]
>> Brian Holt: And then we just saved to the database, right? That's it.
>> Brian Holt: Okay and then await db.run.
>> Brian Holt: Insert into notes from user to user, note values and then just question mark, comma, question mark, comma, question mark. If you're not familiar with parameterized queries, this is gonna do all the SQL scrubbing for you, what escape sequences, right?
[00:03:00]
So that you don't have to worry about SQL injection, all that kind of stuff.
>> Brian Holt: Because all this stuff Is coming from a user, right? From to note, right? And it's the order's important from to a note, okay? And you could send something back here, but we don't need to.
[00:03:24]
>> Learners: Could you put this post note function inside the right page?
>> Brian Holt: Yeah, so you could totally put this directly in here, just like we did here, get users. It would get in the same function, get the form data out and send it, it would work the exact same way.
[00:03:42]
I mostly put these out here because I wanted to write use server.
>> Brian Holt: Right, because this is now being run in a different context. If it's directly in here, it already knows that this is a server component, so you don't have to have it there. But I wanted to show you the occasion where you would write use server.
[00:04:04]
>> Learners: Is that [COUGH] just for functions, or if you had a component, you have to also.
>> Brian Holt: Components are implicitly using server, yeah, so it's only use client that you have to write, yeah, good question.
>> Brian Holt: All right, are we still running? We sure are, so let's go back to our home page and we're gonna write a note and we can't find undefined properties, so let's go figure out what that is.
[00:04:39]
>> Brian Holt: Page here.
>> Brian Holt: Get users await slash notes, I probably messed that up, didn't I? Yep, you got a return.
>> Brian Holt: There we go, so I did not have return there on line seven, It was querying the data base, It just wasn't doing anything with it. So make sure, yeah, line seven, return db.all, select star from users.
[00:05:16]
>> Brian Holt: Okay, so now you can see I have all the people here in the database. I can write a note from Brian, let's write one to Mark since he's not here. What should we write to mark in the database? Because it's like-
>> Learners: Where are you? Where are you?
[00:05:31]
>> Brian Holt: Where the are you?
>> Brian Holt: All right, so now if we go look at my notes, you can see where the F are you. [LAUGH] If I bleep it out, it can go in the course, right, Dustin? That's the rule. [LAUGH]
>> Brian Holt: Okay, questions about that make sense?
[00:05:57]
Form actions, that's what those are called, go ahead.
>> Learners: What's the best way to debug server components in different environments? Do you read the logs or some other method, since debugging in the Chrome Dev Tools is not always an option with server components.
>> Brian Holt: So if you're in the dev environment, it's usually pretty good that it forwards server errors.
[00:06:17]
I mean, let's F around and find out, as they say, as the kids say.
>> Learners: Is there any way to set break points for it?
>> Brian Holt: Everything will work just the way that nothing would apply new here, right? So if you were using the debugger tools here, would work just as well.
[00:06:44]
>> Brian Holt: Oops.
>> Brian Holt: And I put this on the other one, so write a note, so this is on a server component, right? But I got the error here, so they have this actually really well handled. I'm gonna argue it's actually easier to do it this way with next Js cause they forward all of your server errors directly into your browser.
[00:07:11]
And if we look at our, it's the same thing here, right? They actually give you the snippet of what's wrong here.
>> Learners: I'm assuming that's specifically because it's being run in dev mode and it wouldn't do that in production mode.
>> Brian Holt: Correct, yeah, it wouldn't include all of the machinery, I mean, what will actually happen?
[00:07:27]
I suppose it's just gonna crush, right? They're gonna get a 500.
>> Brian Holt: I've never shipped an error, so, I wouldn't know. [LAUGH] Yeah, I'm sure you'd hopefully have some error boundary that would handle it for production.
>> Brian Holt: Other questions?
>> Learners: So, when I click my notes, I'm not seeing the updates I'm sending.
[00:07:57]
Is that because I'm reading a static database or what do you think went wrong there?
>> Brian Holt: So you're inserting to the database, and then you go look at my notes and it's not there?
>> Learners: Yeah, it's coming in just fine on the feed and the server, like the dev server, but I'm just not seeing it in my.
[00:08:14]
>> Brian Holt: Are you sending from Brian?
>> Learners: I'm not, is that would be okay, you can't see everyone's messages.
>> Brian Holt: Yeah, well eventually this secret teacher feed, we'll implement that so you can see it.
>> Learners: Gotcha okay.
>> Brian Holt: If you look at our my page, you can see we're only looking from user one.
[00:08:33]
>> Brian Holt: So it probably is getting written, right? So if you change this to be whatever the correct id was, you probably would find your note. We just did server actions, which are super cool, yeah, actions, server actions is what you would call that little thing that we did here, server actions, that's the term
>> Brian Holt: Yeah, I mean, it's worthwhile seeing that this out, this all works in the context of flight as well, but let's just take a look at that.
[00:09:04]
So I'm gonna write one from Briana Tanner, and I'll just say LOL because I'm basic. And then if we look at the request here, this is actually what it's sending. So this is the server protocol for sending stuff over the wire.
>> Brian Holt: But name from user to user, one note, content disposition at some of these things I don't even know, but that's what actually is getting sent over the wire.
[00:09:35]
That's their API builder that they've kind of built in here, again, you never have to care one iota about this, but that's how it's being implemented, cool, right? It's kind of interesting.
>> Brian Holt: Because, yeah, we called an API right under the hood, it had to get from the client to the server, we just didn't have to write any of that transport.
[00:10:03]
>> Brian Holt: That's kind of the power here, I think that's why they went full in on server like RSCs and next is this is a really good developer experience.
>> Learners: Since that's being somewhat abstracted away, are there any concerns about performance or any time where that being abstracted away becomes kind of a pain point?
[00:10:26]
>> Brian Holt: As long as you're within the bounds of next in React, I can't see why you would do it any other way that being said, it is all still just JavaScript, right? You can still call fetch, you can still have API endpoints, but I'm interpreting your question is like, is there a time where I Could do it and I wouldn't do it.
[00:10:46]
And I haven't encountered that, I haven't encountered where time was like I could write this with React server components, like the implicit wiring up of it, and I have opted to not do it, no performance concerns, right? Because at the end of the day, it's just gathering stuff and sending it over the wire.
[00:11:06]
>> Learners: Actually had a similar question around offline, so would you batch failed calls and then try to retry them in the app logic or how do you handle intermittent?
>> Brian Holt: That's good question, yeah, I mean offline first and RSCs don't go together, I can't really see how they would.
[00:11:25]
You'd have to get real fancy with service workers in a way that I'm uncomfortable with, wouldn't recommend it, right? If offline is important to RSCs in it, right? Because it implies the need for a server.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops