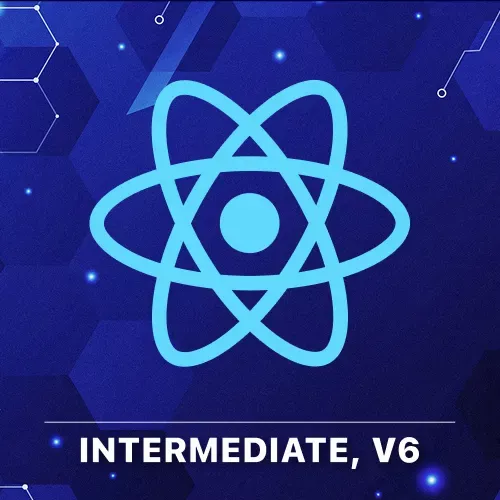
Lesson Description
The "What are Deferred Values" Lesson is part of the full, Intermediate React, v6 course featured in this preview video. Here's what you'd learn in this lesson:
Brian introduces the concept of deferred values in React and explains how they can be used to prevent jank (jerky animations or slow rendering) when dealing with computationally expensive tasks that require frequent re-rendering. He demonstrates the use of the `useDeferredValue` hook, which allows developers to specify that a value should only be re-rendered once it has settled, rather than on every intermediary change.
Transcript from the "What are Deferred Values" Lesson
[00:00:00]
>> Brian Holt: Let's move on to deferred values.
>> Brian Holt: So I'm going to get out of here, I'm gonna get into deferred, and I'm gonna run npm install, and I'm going to code, and then I think that'll just reuse the one, yeah, instead of making a new one. You would think I used to work there.
[00:00:28]
Okay, so what if you have computationally expensive things that need to re-render really, really frequently? If you don't do something about it, you're gonna get something called jank. We've talked quite a bit about jank in the course of this course. I'm gonna give you another tool called UseDeferredValue that allows you to say, this is going to re-render a lot, and then it'll settle for a little bit.
[00:00:58]
Don't try and re-render every single intermediary, wait until this, basically settles, and then re-render it. This is called UseDeferredValue. So, I find that this one is really useful for when you give users sliders, right? So you give them a slider to move up and down and it changes something.
[00:01:18]
If you're trying to re-render every single time that the value changes on a slider, those change several times a millisecond if a user is just sliding it all the way across. And if it's expensive to react to those sliders changing, then you don't wanna do that. So the idea that came to me was like an image editor, right?
[00:01:39]
So if I'm sliding like this, something across, and then I'm changing the blur, or the brightness of it, I don't wanna be trying to re-render every single time, because on an old browser, that would be really bad, even on new browsers, that could be pretty bad. Despite that fact, I actually still had to introduce artificial jank because my browser was still fast enough to keep up with it.
[00:01:58]
It's frustrating how good these things are, right? It makes my job hard, so thanks Mozilla. Still if you're on an older computer, this actually still might be janky enough for you. But if you were doing something like real image interpolation with like image magic through WebAssembly, I imagine that probably would be janky enough for you.
[00:02:19]
So let's go and get this done. The other thing that's really cool about UseDeferredValue, you could do something like debouncing or throttling, it can never keep straight, which is which. The problem with trying to do with that approach is you are now throttling the entire experience for everybody, be it slow device or fast device.
[00:02:41]
What's really cool about UseDeferredValue, it's using the React scheduler underneath it. And so it's just basically saying, if the React scheduler hasn't finished yet, then just like, cancel this re-render cycle and then go to the next one. But if it does finish, then it does do the re-render.
[00:02:58]
And so what's cool about that is slow devices will be very throttled, which is good. And fast devices will still see a lot of intermediary frames, which is good cuz it makes it feel responsive.
>> Brian Holt: Or like another example, this is like, if you put your phone on low battery mode, it throttles the CPU, I don't know if everyone knows that.
[00:03:17]
But like, if you're on low battery mode, your browser will render significantly less frequently. And so, by using UseDeferredValue, instead of trying to build this yourself, you get to take advantage of all these different things that would be super hard to contextually take advantage. But because of how React has structured this, I find it actually quite ingenious.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops