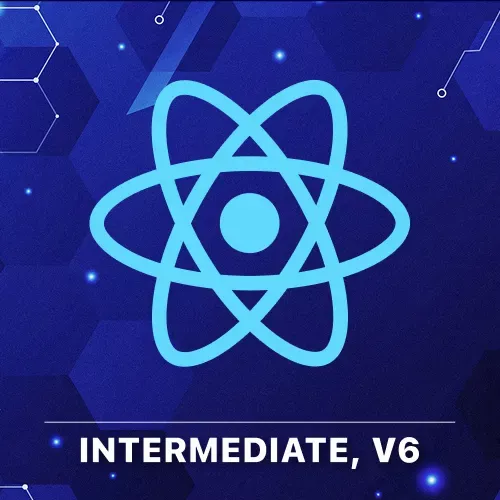
Lesson Description
The "Submitting Data with Form Actions" Lesson is part of the full, Intermediate React, v6 course featured in this preview video. Here's what you'd learn in this lesson:
Brian demonstrates how to use a form action attribute to send data from the client to the server, without the need for additional wiring or state management. He walks through an example of creating a form with select options, a text area, and a submit button.
Transcript from the "Submitting Data with Form Actions" Lesson
[00:00:00]
>> Brian Holt: So let's go make it so that we can write a note. It's interesting that we can look at notes, but how do we take data back from the client to the server? We could have totally done this with our no framework version, but we would have ended up just wiring up a bunch more stuff, when in reality Next can just do all this stuff for you.
[00:00:17]
So I kind of spared you another type fest. So let's go into our app folder, we're gonna make another directory and we're gonna call this one write. You can delete the blah one, we don't need that. And we're going to make a page.js.
>> Brian Holt: And a lot of this will look really familiar as well.
[00:00:49]
This is gonna have some measure of interactivity, but we're just going to rely on the browser to do it, cuz we're gonna do this through untracked forms, right? So we're just gonna allow the browser to manage all the states. So we don't need to use useState, and then we're going to accept that back onto our server component through what's called a form action and then saved to the database.
[00:01:09]
So let's go ahead and do that. We're in page.js on the right, we're gonna import AsyncDatabase, and we're going to import something called postNote, which we'll do here in just a second, from ./postnote. And yeah, we'll write that momentarily, export default async function Write or WritePage or whatever you wanna call it, again, just for debugging purposes.
[00:01:44]
And we're gonna say async function getUsers. And we're just gonna say const db = await AsyncDatabase.open (./notes.db). And then we're just gonna say db.all(SELECT * FROM users).
>> Brian Holt: And then const users = await getUsers.
>> Brian Holt: Again, this is so nice. For everything that I say, all the caveats I throw at Next.js and server components, you can't deny, this is just really nice to be able to do this in React components.
[00:02:41]
>> Brian Holt: Even if you feel a little dirty inside, that makes it better, right?
>> Brian Holt: [LAUGH] You're the bad guy or the bad girl of coding, that's how I feel all the time. fieldset className = note-fieldset.
>> Brian Holt: legend>Write a new note. form, and here's the magic, action=(postNote) className= note-form.
[00:03:26]
Now, postNote is really just gonna be a function. But what's cool about this is that, essentially, you're explicitly, well, kind of explicitly, wiring up this form call an API endpoint, and it's just going to pass that data directly along, right? So you don't actually have to write the API endpoint, you're just gonna say, whenever this form gets called, just invoke this function with whatever was in the form.
[00:03:52]
Normally, we would have to pull it out, we would have to make sure all the data gets into the API call. We'd have to send that API we call fetch, right? That would end up on a API endpoint, and this just mushes it all together. It's like, no, when the form gets called, invoke this on the server with whatever was in the form.
[00:04:09]
I think that's really cool.
>> Brian Holt: Okay, we're gonna do just a couple, we're gonna write a form really quick. So label,
>> Brian Holt: And label's gonna be From, and it's gonna be a select name= from_user. And then we're gonna say users.map (user).
>> Brian Holt: And we're gonna give back an option for each one of them.
[00:04:54]
So you need a key, and that's gonna be user.id, and the value will also be user.id, and then this will just be the user.name.
>> Brian Holt: Okay, that's a good select, the select, label, and then we need another label underneath that one, which is gonna be To,
>> Brian Holt: And same sort of thing.
[00:05:26]
In fact, you probably could just copy and paste this and just modify it manually, don't tell anyone that's exactly what I'm going to do.
>> Brian Holt: Actually, it's the same, cuz in theory, you should be able to validate this, but validation is for people with less time, or more time rather.
[00:05:45]
>> Student 1: So to change the name, that write to to user, or is it [INAUDIBLE]?
>> Brian Holt: Yeah, good point, exactly. So yeah, so this can actually end up being the correct thing on the server side. And then we're just gonna put a default value here as well, default. The default value, at least, is not to and from the same person, so I'm just gonna make it to number 2.
[00:06:13]
>> Brian Holt: Okay, that's good for that. Under that, we'll have another label, and we'll just put the Note and textarea name = note. And cuz this is React, we'll make it self-closing cuz that makes sense. And then under that, we'll have a button to submit the whole thing, button type= submit.
[00:06:43]
Actually, don't need that, that's implied, but let's be explicit and safe. Okay, just a form, right?
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops