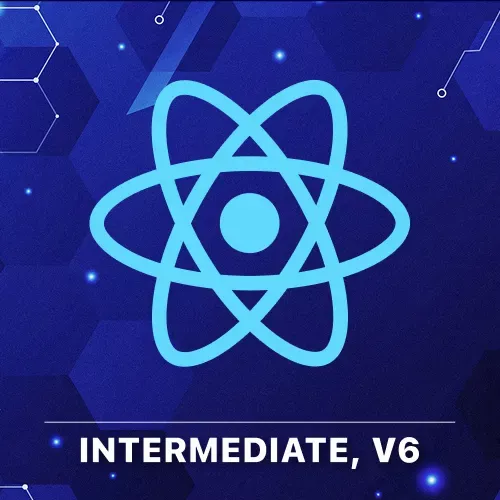
Lesson Description
The "Performance Issues in React" Lesson is part of the full, Intermediate React, v6 course featured in this preview video. Here's what you'd learn in this lesson:
Brian introduces the concept of optimizing React components by preventing unnecessary re-renders using techniques such as memoization. He cautions against overusing these techniques and highlights that the default behavior of React is usually sufficient for most applications.
Transcript from the "Performance Issues in React" Lesson
[00:00:00]
>> Brian Holt: All right, so we just did RSCs with Next.js. Hopefully RSCs were much easier with Next.js and you understand why next is the way it is, because parts of this are not fun to write, right? Yeah.
>> Brian Holt: Okay, so let's talk a bit about the next couple of sections here.
[00:00:22]
One, they're gonna be way lighter weight than what we just did, these are gonna go much faster. And it's just fixing potential React problems. So let's hop into performance optimizations here, so where React can be slow. I'm just gonna say out of the box, if you're writing React, like I've taught you here, it's fast enough, almost always, right?
[00:00:47]
Many, many, many apps I've written I've never had to hack around the performance. Just because the model that they've built for React generally just works fast enough, even on fairly slow devices. I'm sure you've read all of the thought leader pieces or tweets or something like that. It's like React doesn't do this fast enough and that kinda stuff, even on slow devices it typically works just fine.
[00:01:11]
We measured that a decade ago at Netflix and that was generally fairly true. And Netflix deeply cares about that because that's their bottom line, right? It's pretty hard to make non performing code. In fact, writing these examples, I wasn't even really able to do it. We're gonna have to artificially slow down our code so that you can actually see the difference here, I couldn't get things slow enough.
[00:01:36]
So that should tell you a whole lot about what I'm about to teach you is like, this is very, I won't say niche, but it's gotta be contextual and maybe stated differently. Please don't preemptively do these things. Don't say like, this could be slow, I'm just gonna write a memo.
[00:01:54]
No, bad, stop it [LAUGH]. As your potential future coworker, I'm just mortified that I was like, I learned this from you, and I'll be like, I have failed humanity. So this is another thing is like please measure, please have a problem before you solve a problem. Again, React is normally fast enough for almost everything.
[00:02:17]
So I'm gonna show you several different ways. Some of them are about perceived performance, which we talked about earlier. And some of them are about, like, actual performance, of like, how to make react actually run faster. Some of these concepts aren't really that important until they're very important, right?
[00:02:31]
You're going to hit some slow down. You're gonna realize, like, my gosh, I need these right now, which is why it's important for you to still have these filed with, I could do these if I needed to. Yeah, strong advice here, do not preemptively use these. And I'm harping on this because it's gonna be a temptation.
[00:02:47]
The code is actually not that hard to write, so you're thinking that maybe I could do this all the time.
>> Brian Holt: No [LAUGH], okay, I think I've said my piece enough times, I might still say it again, I can't make any promises. So we're gonna talk about the most useful way, probably for actual React performance.
[00:03:09]
Let's say you have app here. Let's just make this a bit smaller, for a second, you have app, and your app has, what is that? Four child components you have content which is its own island, and then you have profile and profile pic and profile name. So let's imagine for a second that you have something happen in profile and nothing else happens.
[00:03:33]
Well, content won't run it's React is actually smart to say like it only happened in this component, so I'm not gonna render app again, I'm not gonna render content again, I'm only going to re-render profile. Imagine this is like a use state hook that changed, right? So someone called like, set count, and it changes.
[00:03:53]
Does profile pic run again? Yeah, it does, so does profile name right both of these will run again. Because whenever you dirty a tree, right? What React does is, cool, re-render everything in this tree. Now some people might be horrified, but how much inefficiency is that generating? Some, minuscule, almost unmeasurable amounts, right?
[00:04:19]
It's not enough for you to worry about, I should prevent these things from me running because it generally just doesn't matter. Cuz you can imagine this might have some sort of side effect that would cause these to be also stale. And so rather than try and nitpick or fine grain is actually the term, right?
[00:04:39]
Rather than having a fine grain rendering of those things that just re-renders everything. Again, generally, this pattern is almost always fast enough. I'm sure some of you use the Netflix app, right? It's all done with React and most of it doesn't touch any of these React tools and it's just fine, right?
[00:04:58]
It renders plenty fast. 99,999 times out of 100,000, this is just fine. However, what happens if you update something in profile? We know nothing's gonna change in profile pic, and this is also very expensive to re-render. Let's say, this is doing interpolation of images and running like image magic in the browser or something wild and silly like that.
[00:05:24]
And we want to make sure that please don't re-render this. That is what I'm gonna show you right here. It's, okay, profile needs to change and we cannot have profile pick re-render unless it absolutely needs to.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops