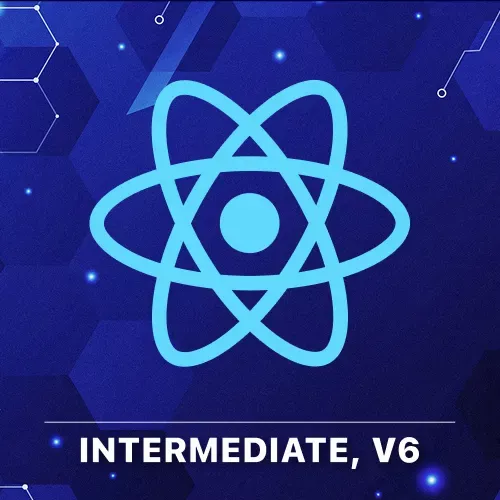
Lesson Description
The "Creating a Next.js Application" Lesson is part of the full, Intermediate React, v6 course featured in this preview video. Here's what you'd learn in this lesson:
Brian demonstrates how to create a new Next.js app using JavaScript instead of TypeScript. He explains the app router in Next.js and shows how to create routes using the Link component. He also discusses the layout component and how it is used in conjunction with the page component.
Transcript from the "Creating a Next.js Application" Lesson
[00:00:00]
>> Brian Holt: Okay, so next step, should we build the next step? Sounds kind of fun, right? Let's do it. All right, so you can copy and paste this, that's what I'm gonna do. I'm gonna build a new one here and we're gonna call this one mkdir next, cd next and then I'm gonna run npx create-next-app@15.1.7.
[00:00:23]
We're doing js today, I'm sure some of you are wondering why I'm not doing this in TypeScript. I'm just trying to remove barriers from you and learning React. I love TypeScript. I technically was part of the TypeScript team when I was at Microsoft, cuz that VS code team, TypeScript team is kind of all the same team.
[00:00:39]
So I love it, but I just want you to have the most access to the information possible, and I don't want TypeScript to get in the way of that. We're doing the app router, we're gonna do a source directory, and we're gonna use turbopack, which is like the rust powered version of webpack.
[00:00:59]
And probably oversimplifying that, but it's the Vercel official build product for Next.js. Okay, what do you wanna call it? My-super-cool-note-app. Do you want to use ESLint? Sure, yes, no, I don't know, no. We're not doing Tailwind today, I'm not gonna do the import alias, you could if you want to.
[00:01:30]
>> Brian Holt: And we should get a whole app, all right.
>> Brian Holt: So, yeah, there we go, code..
>> Brian Holt: Maybe, okay, cd my-super-cool-notes-app.
>> Brian Holt: Okay, so couple more things to install here, we need our doodle.css, npm install. Doodle.css@0.0.2, promised-sqlite3@2.1.0 and sqlite3@5.1.7.
>> Brian Holt: Again, feel free to copy and paste those directly on my notes.
[00:02:34]
>> Brian Holt: Do I care about any those? Nope, sure don't care. No one cares about warnings. And then I'm gonna copy that same notes.db file that we had in our other project. Okay, so same file we're gonna work with the exact same notes.db. Yeah, it looks like I put a seed file there as well.
[00:02:53]
I thought some of you might wanna use Postgres or something like that, feel free to.
>> Brian Holt: There's also a very good Postgres course on Frontend Masters. Again, taught by just a stunningly handsome young man. [LAUGH] I don't know why, when I'm speaking of myself in the third person, I have to be steadily handsome.
[00:03:13]
Aspirational, I guess, vanity, who knows? No one can know. Okay, so let's get going on this. This is a Next.js project, there's a config file here. We don't have anything in it, that's totally fine. Jsconfig, this is for Typescript reading and Javascript project, that's what that's for. There's a bunch of stuff in here, you can leave it in here if you want to, that's totally fine with me.
[00:03:48]
And then we're gonna go to source/page.js, so this is the app router, and there's a page router, which is different. Not talking at all about the page router today, this is just the app router today. Just two different ways of creating routes in Next.js is essentially what it is.
[00:04:08]
I have my /directory, and in that /directory I have the page, and that's going to be the page that's rendered whenever that is it. So if I make, I don't know, just a blah, right, and I put a page.js in blah, then whenever I go to /blah, it'll render the page inside of that.
[00:04:26]
I also have a layout and anything that's a child of that will be rendered inside of that layout. So you'll notice here that it has children, the page is going to be rendered inside of the children there as well, so that's what layout is. And this is what I was talking about, I'm pretty sure this would qualify as static site generation.
[00:04:44]
So this part of it's actually generated beforehand, totally on the server, and then that's filled with the page.js is like a child component or a child React app, essentially. Okay, not that you ever really have to care about that they don't make the distinction for you, so you don't have to make the distinction.
[00:05:03]
Okay, so page.js delete all that, just total nonsense. Just kidding, I'm sure it's fine, but I don't care. Import Link from "next/link";.
>> Brian Holt: And then we're just gonna make a homepage here, of all the essentially lessons that you and I are gonna do together. default function Home(), return and then just a pretty basic little ul here unordered list.
[00:05:42]
>> Brian Holt: li and then we're just gonna do a Link and href = My Notes. So links here are basically like a tags, right? Anchor tags for Next.js apps and we're just gonna make four of these, so you might as well just copy and paste like me. And My Notes is going to be going to /my the next one here is going to be going to write, so we're gonna do Write a Note.
[00:06:18]
>> Brian Holt: And then we're gonna do /teacher, which is gonna be the super Secret Teacher Feed. And then the last one is for all my existential friends out there, we're gonna do a "who-am-i" route. We're gonna peer into our own souls and wonder who we are.
>> Brian Holt: You don't need the explanation point and question might but it felt appropriate, so I put it there.
[00:06:53]
Okay, let's go ahead and go into to layout.js now. Delete that and import Link from "next/link". Import "doodle.css/doodle.css" and import "./global.css", which reminds me, let's go ahead and go grab that. I have it here, where do I have it? Looks like I forgot to put it in there, that's something to fix their destined.
[00:07:39]
Github/btholt/Irv6-project, and let's just grab it from completed here, cuz I know this one works. Next.js src/app, this globals.css, that's really the one that I care about here. Raw, copy and paste and then we're gonna come back over here and not this one, nobody likes that one anymore, we're done with that one.
[00:08:10]
>> Brian Holt: Here we're going to put in globals.css, we're just gonna replace all of that with much superior css that I wrote. Much better than the people of Vercel, and you can delete the page.modules at css, cuz we don't need that one anymore.
>> Brian Holt: Okay, and then we're going back to layout.js, export const metadata = title:.
[00:08:42]
"Note Passer", this is all the stuff that's gonna be put into the head, essentially, right? If you've ever used React helmet, this produce that as well. React 19 now has better ways of putting stuff into the head implicitly. If you render a link, it knows that that never goes in the body, so it can put stuff in the head for you, so it's pretty neat.
[00:09:05]
description: "Example app for Frontend Masters", and then export default async function RootLayout. It's gonna take in children, and we're just gonna return html lang="en". body className, we need the doodle one, so that the css shows up, and then nav, h1, we'll have a Link.
>> Brian Holt: href="/", we'll call this Note Passer and then under nav, we're gonna have children.
[00:10:08]
Pretty simple so far, root layout here. So again, layout will be the most outer one and then inside of layout will have page.js. These are significant names to Next.js, right? Next.js is going to be looking for these specific file names, which is how everything gets put together. I don't like this pattern to be super frank, cuz I could see a new developer coming into this code base being like, why is this put together, right?
[00:10:33]
How is layout and page linked together? There's no explicit way that those are, you just have to know that Next.js does that for you. When I learned Rails for the first time, when I was a much younger developer and Rails was super hot, I'm dating myself again. That was hard for me, that was really hard for me because I didn't understand how things got built.
[00:10:55]
But here we are, more than a decade later.
>> Speaker 2: Do you have to name the components root layout and home as well as that also [INAUDIBLE]?
>> Brian Holt: No, that's just for what I was talking about before which is the debug ability of it all, you could totally leave this out.
[00:11:11]
It does have to be the default, that's important that it has to be the default. Export and the metadata that has to be called metadata, the variable names, the exports matter, but this is just for making it more readable. It's good question, though. Okay, this should run. Probably runs, I don't know, YOLO, YOLO.
[00:11:37]
We're gonna do npm, is it just dev? Lucky guess and I'm gonna open ths up in 3000. Globals.css, let's go find that.
>> Speaker 3: Can you call it global in the import?
>> Brian Holt: Like a noob, right? Here we go, okay. So there we go. Note Passer, we can always click to go here.
[00:12:03]
None of these are gonna work, right? So you're gonna get 404s on each one of these. But now we have a place for all the components that we're about to build.
>> Brian Holt: But hopefully you're already seeing, Next.js is a pretty productive framework, right? We didn't have to do a whole lot, and this just kinda worked pretty quickly.
[00:12:23]
Static route, neat, I had not seen that before.
>> Brian Holt: Anyway.
>> Speaker 4: Is that just the next thing?
>> Brian Holt: Yeah, so it'll forward server failures onto your client so you see them right away. There's a lot of good thinking going, it's their core business hosting next apps is their core business.
[00:12:44]
And so they need everyone to use Next.js, and then they need to be the best place to host Next.js, so that you use for sale. It's great business, to be honest to you, it works for me. I know exactly what's happening to me, but I'm still gonna do it.
[00:12:57]
By the way, I like server component, right? You didn't even know you're writing one and these are server components. So take that for what you will, it is very cool.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops