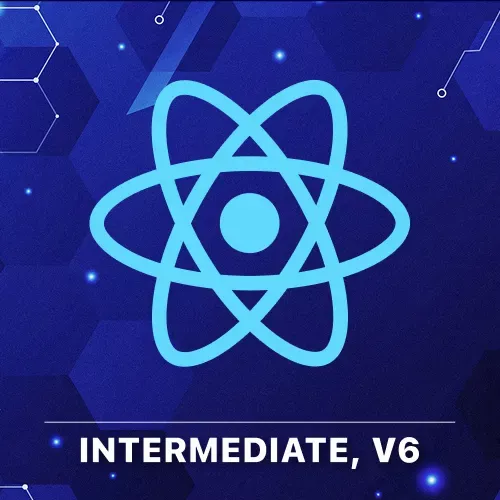
Lesson Description
The "Client Components" Lesson is part of the full, Intermediate React, v6 course featured in this preview video. Here's what you'd learn in this lesson:
Brian begins by setting up the project structure, creating a src directory, an App.jsx file, and builds the client component. The client component uses useState to manage a counter and includes a button that increments the counter when clicked.
Transcript from the "Client Components" Lesson
[00:00:00]
>> Brian Holt: So we're going to create a source directory. This is gonna be like a more serious app. There's gonna be more to it, which is fun, cuz we've now bothered to set up the entire server, make an app.jsxfile here in your source directory, and we're gonna import suspense from react.
[00:00:19]
We talk about suspense and the complete intro to react, but it basically allows you to have boundaries around, hey, if stuff inside of here isn't finished loading yet, show this loading screen. And then allows you to write all of your React components as if everything was loaded all the time.
[00:00:32]
Which makes the authoring of React components much more simple, which I appreciate. Okay, and we're gonna write two components here, a server component so that you can see what it looks like from the server side. And then we're gonna write a client component so you can see how to opt into client components.
[00:00:52]
So we're gonna import server component from ./ server component, which we'll write here momentarily. And we're going to import client component from ./client component. Okay, and then export default function app. Okay, export default. Okay, what did I do? Form that's what it was. I was like, why is this not syntaxing correctly?
[00:01:29]
>> Brian Holt: Okay, I'm gonna put a bunch of console logs in here, because I think this actually is, it kind of shows you where everything is rendering, which I think ends up being kind of cool. So console.log rendering app server component.
>> Brian Holt: Okay, and then we're just gonna say return.
[00:01:50]
Suspense, fallback equals whatever you wanna put here. Normally you'd have something a bit nicer, we're just gonna say loading.
>> Brian Holt: Okay, and we're gonna put h1, we're gonna make this a notes app. Then we'll have our server component. We'll have our client component. And that will be our app.
[00:02:24]
>> Brian Holt: So I kind of spoiled you a little bit on this. But is this a server component, or is this a client component? It's a server component.
>> Brian Holt: Again, by default, they wanna make everything in here a server component, so you only opt into client when you need to.
[00:02:45]
And so, especially, typically the top level of your app is always going to be a server component. I'll show you how to hack around this a little bit, but you can't make server components children of client components, only the other. So if you wanna have a server component, it has to be the child of another server component.
[00:03:08]
So by virtue of that, if your top level component is a client component, that means all of its children will be client components.
>> Speaker 2: That's just because of the dependency inheritance of you would be waiting for the client component anyways?
>> Brian Holt: Yeah, well, server components are always gonna run first, yeah, so it's gonna run the entire tree first, right?
[00:03:27]
And that you can't opt out of that cycle. So let's do our client component, I think that makes sense. Let's do that one first. So just the big thing to file in your brain here is, hooks don't work on the server. If you want any sort of hook, you need to opt into a client component.
[00:03:48]
And that's the part where I was saying it's a departure from writing normal JavaScript because or normal react because normally you're just like, cool. I need to use state right now, use state, and you have to have a frame of mind of like, wait, do I have children that are server components?
[00:04:06]
Do I need, you have to start having these concerns around, where am I in my app?
>> Brian Holt: And you didn't have to before.
>> Brian Holt: Clientcomponent.jsx, so, how do we make it a client component? What do you do? This is rhetorical, cuz I have not told you [LAUGH]. You put this, what do they call them?
[00:04:32]
Directives? Forgot, pragmas?
>> Brian Holt: But this is what you do, you put at the very top, the first thing must be a string and it says use client.
>> Brian Holt: Right there playing off of like use strict here. Do you remember writing use strict everywhere? If you don't again, bless you, we used to have to do that back in my day, back in ES5 days.
[00:04:59]
We had to tell we're in strict mode, or we're not in strict mode, they kind of use that same pattern here for use client. Now, you might ask yourself, why didn't we put use server here? Use, it's just assumed to be server, so you don't have to put that.
[00:05:14]
You can put it there, but it's the same as putting any string at the beginning of your thing. It just doesn't make any sense to so UseClient, that's what this is for. We will use useServer later, but it's not for the same thing. Okay, so now that we've done this, this is just a normal React component here.
[00:05:35]
So import useState from React, export, default,
>> Brian Holt: Function, ClientComponent,
>> Brian Holt: Console.log.
>> Brian Holt: Rendering, client component, God, I was redundant, wasn't I? Rendering, client component, client component. It sounds more dumb when you have to say it out loud, but that's what my notes say. And yeah, again, we're just gonna say const, counter, set counter, enter equals, use state zero, and return.
[00:06:28]
I have this using this again, this doodle.css, and so, they use this field set everywhere. So that's why we're gonna use that, legend. Client component, P, and then we'll have counter, counter, okay and button, and we'll have an on click here. This is going to set counter of counter +1.
[00:07:09]
>> Brian Holt: And then we'll have increment, or whatever you wanna call it there. So again, very the normal React component here, just to demonstrate that, like all the interactivity of it still works. Yeah, directives, that's what they call these. And yeah, useServer is assumed, so you don't need to put it.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops