Master Modern React to Build High-Performance Apps
Get hands-on with the latest React 19+ features: Server Components, Suspense, and Transitions to build high-performance apps using modern patterns.
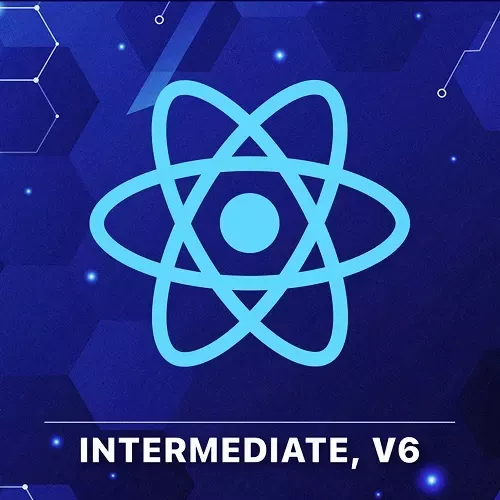
Why Intermediate React
Build Scalable, Modern React Apps

Go Beyond the Basics

Build Real-World Apps with New React Features

Optimize Performance

Stay Ahead with Production-Ready Skills
What You'll Learn
Dive Deep into the Latest React 19 Features and Advanced Topics
This course takes your React skills to the next level by diving into more advanced concepts and modern best practices. You'll learn how to build faster, cleaner, and more maintainable React applications.
- Client-side React vs. Server-Side Rendering (SSR) and Static Generation (SSG)
- Use React Server Components (RSCs) from scratch and in Next.js
- Build hybrid server/client apps with server actions and form handling
- Advanced UI techniques like optimistic updates and low-priority transitions
- Best practices for scaling React codebases and maintaining fast user experiences
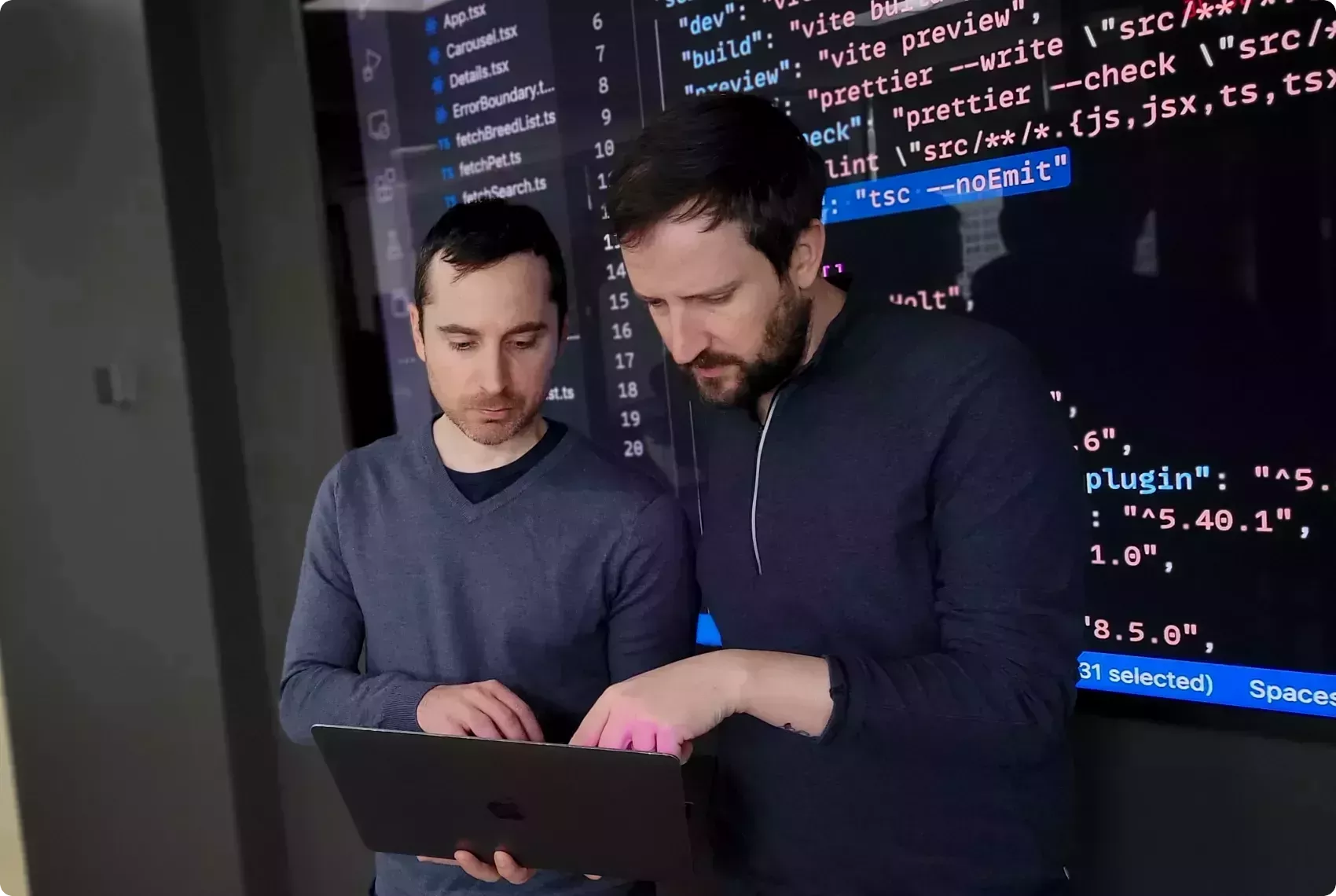
Your (Awesome) Instructor
An Engineer, Leader, & Teacher Who Builds for Top Companies
With deep experience building developer tools at Microsoft, Stripe, Netflix, and Reddit, Brian Holt brings a passion for engineering, teaching, and creating world-class developer experiences to every project.
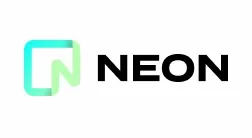
Staff Product Manager
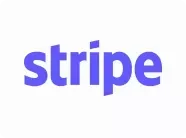
Product Manager
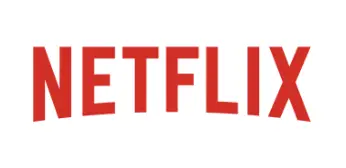
Senior UI Engineer
Coursework
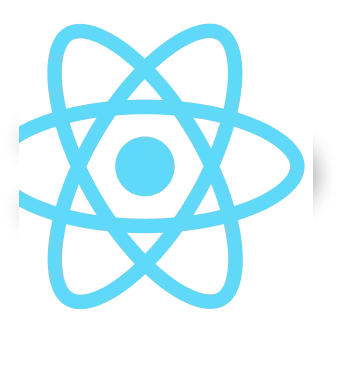
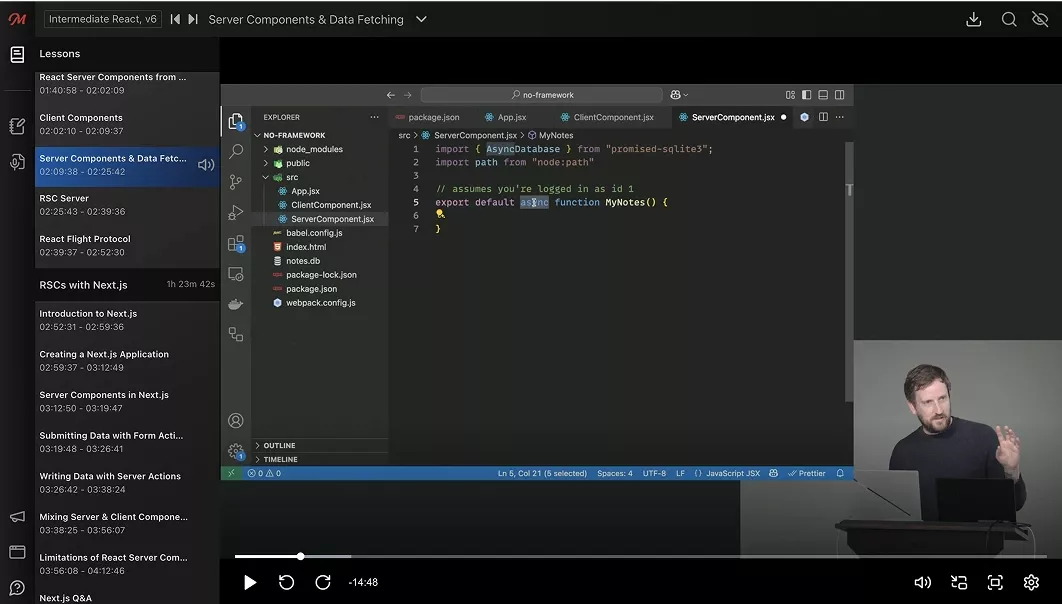
Best in Class Course Player
Your React Learning Adventure Begins Here
- Course Progress: Learn at your own pace and pick up right where you left off.
- Robust Note-Taking: Take notes alongside transcripts to easily reference information while learning.
- Q&A and Code Corrections: Submit and view questions and answers, as well as code corrections.
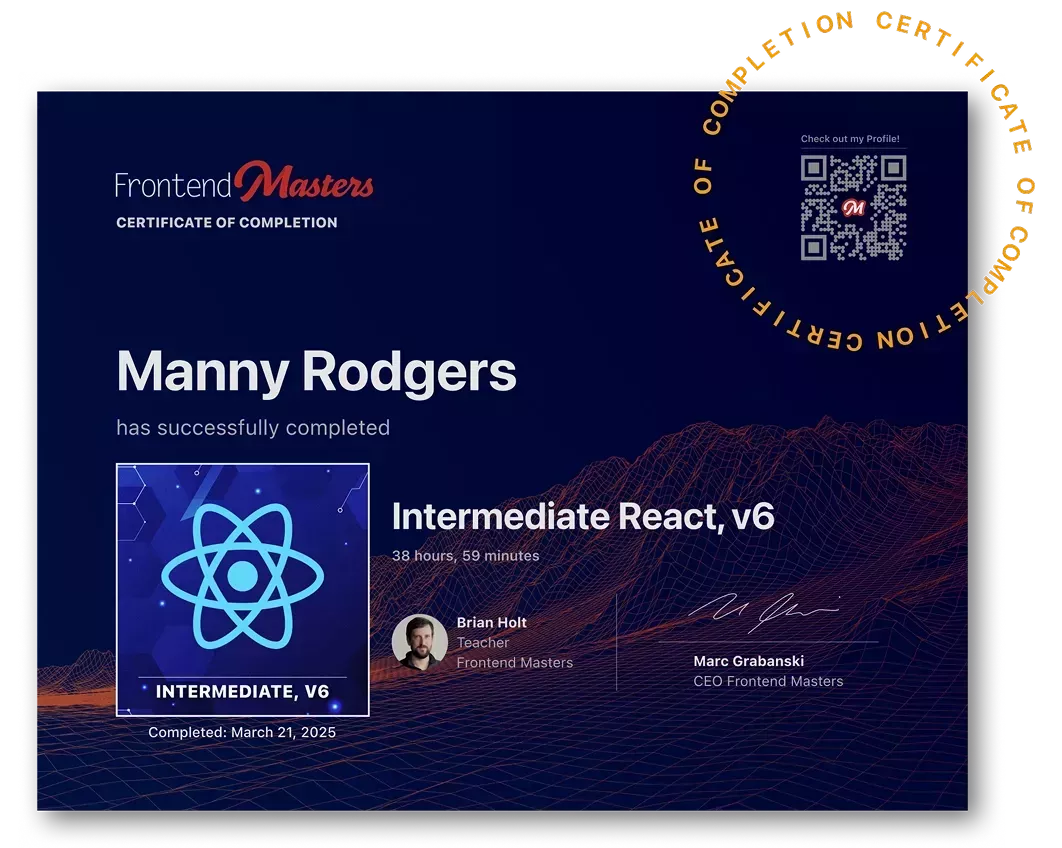
Earn a Completion Certificate
After completing this course, you'll receive a certificate of completion that serves as proof of your achievement, showcasing your expertise, and commitment to professional development. You can easily share this certificate on your LinkedIn profile to highlight your new skills and demonstrate continuous learning to potential employers and professional connections.
Get Started with Intermediate React, v6 and Much More
- 200+ In-depth Courses
- 21 Learning Paths
- Industry Leading Experts
- Live Interactive Workshops
What They're Saying about Brian Holt
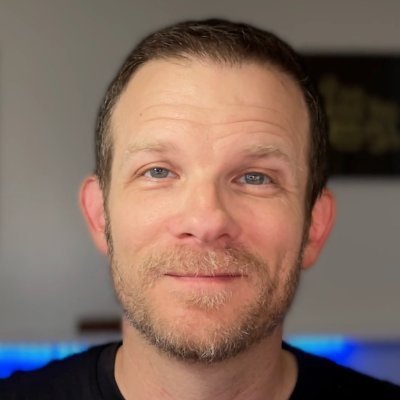
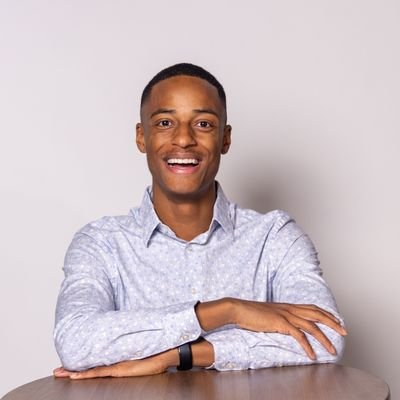
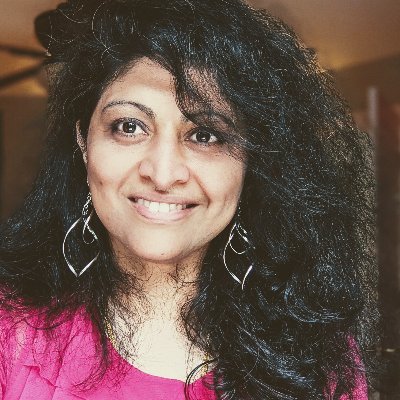
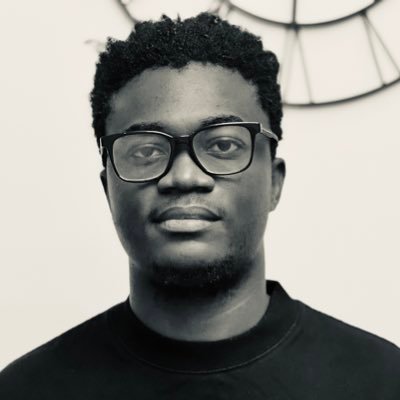
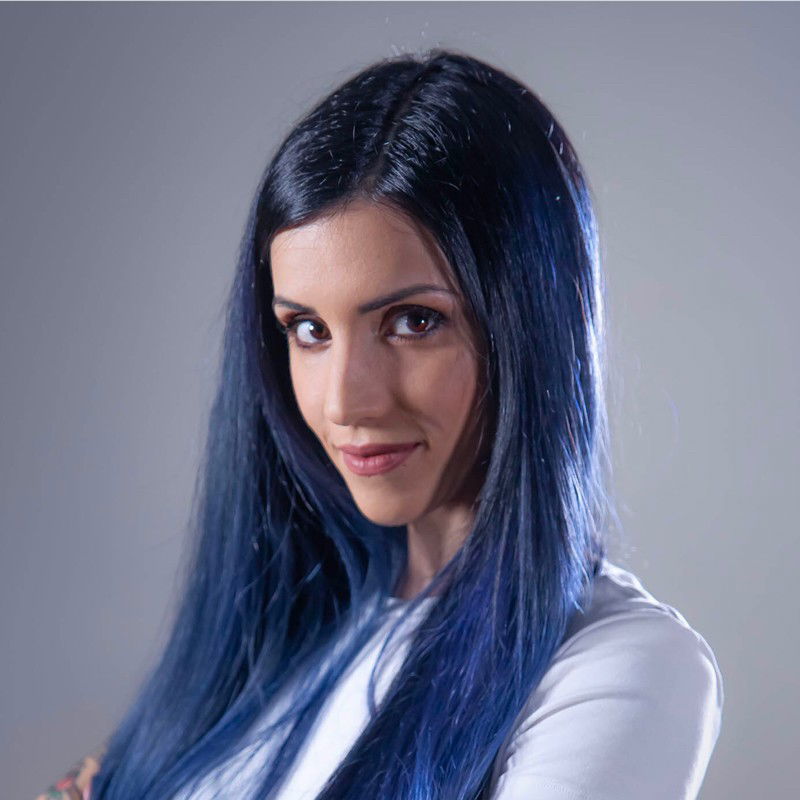
It was extremely interesting to see what Next.js actually does under the hood. I learned a lot. Thanks for this course!