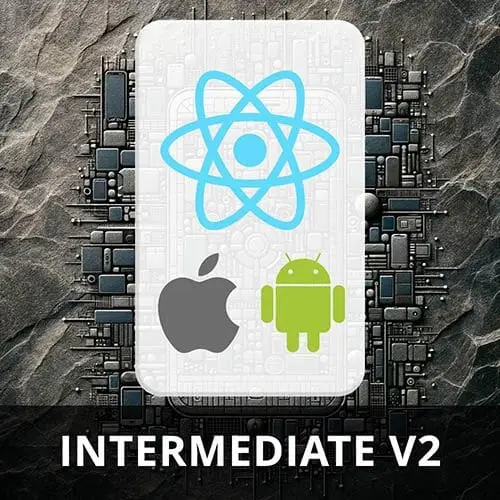
Check out a free preview of the full Intermediate React Native, v2 course
The "State Management with Zustand" Lesson is part of the full, Intermediate React Native, v2 course featured in this preview video. Here's what you'd learn in this lesson:
Kadi introduces Zustand, a Redux-like state management library. A userStore component is created to manage the user's state. A hasFinishedOnboarding property is added to the store
Transcript from the "State Management with Zustand" Lesson
[00:00:00]
>> Kadi Kraman: So right now, we have hard coded our state for whether the user has on board or not. This is obviously not what we're going to do in our real application, we need to have some kind of state management. Even at the intercourse, you saw us using async storage to persist some data across app launches.
[00:00:19]
And we're pretty much going to do the same thing, but we're going to use a state management library. And in pretty much all production, I would say in most production applications, you are going to use a state management library instead of using async storage manually. But most state management libraries will use async storage under the hood, to actually persist your data across app launches.
[00:00:43]
So which state management library use, I'm not really advocating for any particular one. There's a couple of popular examples here. So we have Redux, of course, with Redux Toolkit, which is pretty lightweight these days. We have Zustand, which I think a lot of people who were old school Redux users have migrated to Zustand because it is quite an easy and natural migration.
[00:01:13]
And we have Yotai, which is more lightweight and hooks -based. So as I said, I'm not advocating for any particular one, I recommend that if you're choosing a state management library for your own project, you just try out a couple of these. Or at least try out all three and choose one that you and your team like, yes.
[00:01:30]
>> Speaker 1: Do you automatically go to a state management library when starting an app, or do you rely on maybe optimizing second and maybe use I don't know if context is popular in the react native world?
>> Kadi Kraman: Yeah, for sure. So context is really good, really useful. If I need to persist data across app launches, I would use a state management library just because I don't want to handle the async storage, get from storage and save from storage.
[00:01:58]
And I don't want to handle the sinking because a state management library will do it for you and it will do it in a more optimized way as well, so we're not saving all the time.
>> Speaker 1: You said between app launches, Is that what you said between?
>> Kadi Kraman: Yes.
[00:02:10]
>> Speaker 1: Okay.
>> Kadi Kraman: So basically in our case we have this has finished onboarding boolean. And every time I close and reopen in my app, this will be reset to true because it's just a constant that I've defined here. Or in case I'm not even updating it, it's just a constant.
[00:02:28]
But what we want to do is that once the user has finished onboarding, we will set this to true and save it into our device storage. So that the next time the user opens the app they don't have to do onboarding again. All right, so let's install the Zustand library.
[00:02:49]
>> Kadi Kraman: I'll try to keep it in my VS Code. So I stop my server, install Zustand.
>> Kadi Kraman: Okay, so let's create a new folder in the top level. And we'll call it our store, and we'll put all our Zustands stores in here, and let's create a new file called User Store.Ts.
[00:03:29]
Now we want to, so we're using TypeScript, so let's type our user store so what we're going to implement. So a user store is going to have a Boolean as finished onboarding. This will be a Boolean that is mandatory, and it's gonna have a function, to toggle this onboarding to true and false.
[00:03:58]
So toggle has onboarded,
>> Kadi Kraman: And this will be a function, and return nothing. So onboarded. Installing a spell check on my VS Code, code has been incredibly handy, I make a lot of spelling errors. All right, so this is what we're going to implement. So let's import create from Zustand.
[00:04:39]
>> Kadi Kraman: And we want to create our userStore. So we understand what you actually export is a hook to your store. So export const useUserStore.
>> Kadi Kraman: And we're going to create,
>> Kadi Kraman: So this will be a function. And we're going to, with TypeScript, we're gonna pass our userStore in here.
[00:05:08]
So just to define what we're going to create. And then inside this, we're going to have a function. So this will have set to update the store, and we'll have some initial, there we go. So this needs to return the object, I think this is right. And we're gonna have our initial state.
[00:05:35]
So has finished onboarding. Let's start with, false. And we'll do toggle has onboarded, which will be a function.
>> Kadi Kraman: We're gonna use this set, so, this is how you update the state of your zest and store. And then we can use set, so, this will get the state.
[00:06:04]
>> Kadi Kraman: And we're going to return the new state of the store. So return, let's spread the existing state, this is why it's like Redux. Feels right at home. hasFinishedOnboarding, and this is toggle so we're just going to go to state.hasFinishedOnboarding and then flip the Boolean, okay. So this is a little store where we have our hasFinishedOnboarding, which is a Boolean, and then a function to toggle it from true to false.
[00:06:41]
>> Kadi Kraman: Now, in our layout file, instead of having this hard-coded value, let's do hasFinishedOnboarding, and this will be useUserStore. So the hook that we've just created and this takes a function, where you get the state and then you pick the things you want to include from the state.
[00:07:15]
So we'll do a state hasFinishedOnboarding. So you could conceivably create a hook here that just returns all of the values in your state. But, I think the convention is to do one value at a time just because to make it a bit optimizing for updating. So every time this store updates all the functions that listen to this change would obviously, all the components would get re-rendered.
[00:07:46]
So if you're listening to something that you're not using, it would get re-rendered unnecessarily. So that's where we're specifically using this constant and picking out this hasFinishedOnboarding.
>> Kadi Kraman: Okay, so let me,
>> Kadi Kraman: Start my bundle again.
>> Kadi Kraman: Okay, so let's go to our onboarding screen.
>> Kadi Kraman: And in the screen, so in the intercourse, it said that we almost never used the native button element from but this is the moment.
[00:08:41]
It is really useful for having some quick buttons if you need to test things. So, let's replace this with a button, and title will be ''Let me in!''. And onPress, we need to add this handlepress function.
>> Kadi Kraman: So onPress, we're going to call handlePress and in this function, we need to also hook into our start store, but we want to get our toggle has on boarded function so we could toggle it from false to true.
[00:09:24]
So const
>> Kadi Kraman: ToggleHasOnboarded,
>> Kadi Kraman: So be useUserStore from our store then we get the state and we pick out our toggle has unboarded function. Right, so we press, we want to toggleHasOnboarding state from true to false. Now if you do this, you notice that nothing happens. And the reason is that this layout file where we're doing the switch isn't being rendered.
[00:10:09]
So if we work backwards, so right now we're in this onboarding screen, this is what's being rendered. So if we work backwards in order of rendering, we're getting this onboarding screen and then the layout screen that's in the same folder so that's rendered first. So we're getting a layout on boarding so none of this path is rendered at all.
[00:10:29]
So for that reason we have to manually navigate into our app as well. So for this we can use programmatic navigation, so let's use the use router hook. So we'll do const router and we'll useRouter This is from Expo router. And after the toggle, we'll do router and you have a couple of navigation application options here.
[00:10:58]
The important ones, I guess, is back, so you can go to back to a previous screen on your stack. You can do navigate, so this will be navigating to a screen. You can do push, which pushes the next screen onto a stack. And in our case, we're actually going to use replace.
[00:11:18]
So this will replace the current between the stack or current stack with the next root. The reason we're doing replace, is that we don't want the users to be able to swipe and go back to onboarding, that doesn't make sense. So we want to actually replace our stack with the home.
[00:11:40]
>> Kadi Kraman: So now if I do let me in, it actually opens our home screen and again for testing, I've used toggle here on purpose so we could go back to onBoarding which is handy for testing. So in our profile screen, let's add a button here as well. So we'll use the same button from React Native.
[00:12:06]
>> Kadi Kraman: And let's do Button title, say Back to onboarding. And on press we will do const.
>> Kadi Kraman: ToggleHasOnboarded, this will be from our store again. So useUserStore and we'll go to the store, and we'll pick out the toggleHasOnboarded function, and then on press, we will toggle the onboarded state.
[00:12:53]
Get rid of some styles we're not using, this is why I installed that ESLint plugin. Here it is, lovely. And if I go to profile, now if I go back to onboarding, this will open my onboarding state again. And finally, you'll notice that our navigation here is maybe a little bit sub Optimal.
[00:13:17]
We are navigating by sliding in the screen and we can change the animation of the navigation. So by default, we will, because we're entering in a stack, it will just float in from the right. So if we go to the root layout file, let's open the, let's go to the onboarding screen and pass in some options.
[00:13:43]
So firstly, cool. Well, that was silly of me. So firstly let's hide the onboarding header. So we'll do a header shown,
>> Kadi Kraman: And for the animation, let's do animation fade. Let's do animation fade for the tabs there as well. So let me go back to onboarding. So now, when we toggle between them, the screens actually fade into from one to the other, which this animation makes a lot more sense when it comes to onboarding.
[00:14:29]
But you can use a different animation here and you can also configure custom animations.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops