Codesmith
Course Description
Keeping what the user sees in sync with the app's data can be tricky, particularly in browsers and big apps. UI tools like React, Angular, and Vue help, but they can be hard to use if you don't know what problems they solve. Develop an under-the-hood knowledge of UI dev by learning techniques such as data binding, UI composition, templating, virtual DOM and its reconciliation, and hooks, all from scratch! You'll learn how JavaScript interacts with browser features like the DOM and HTML parser. By the end, you'll have a complete mental model of UI dev that you can apply to any framework!
This course and others like it are available as part of our Frontend Masters video subscription.
Preview
CloseWhat They're Saying
I just completed "The Hard Parts of UI Development" by Will Sentance on Frontend Masters! Gosh, my head is full of diagrams and execution contexts.
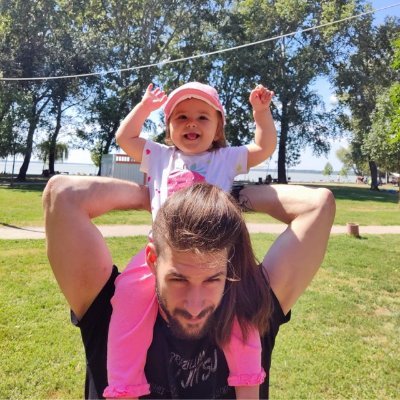
Bence Fekete
fekete965
I just completed "The Hard Parts of UI Development" by Will Sentance on Frontend Masters! This is mind blowing 🤯 , thank you, Will. 🤍🫡
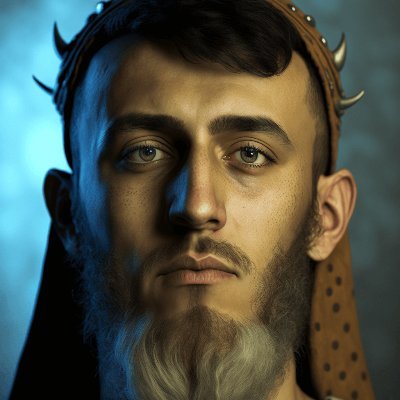
Yassine El Hajjami
yassine_el01
Learn Straight from the Experts Who Shape the Modern Web
Your Path to Senior Developer and Beyond
- 200+ In-depth courses
- 18 Learning Paths
- Industry Leading Experts
- Live Interactive Workshops
Table of Contents
Introduction
Section Duration: 7 minutes
- Will Sentance begins by discussing the goals of the course, which include understanding how user interfaces display content, manage data-binding, are represented in the virtual DOM, and can be optimized. Core principles of engineering, including problems solving, technical/non-technical communication, and programming experience, are also discussed in this lesson.
State & View
Section Duration: 1 hour
- Will explains that almost every piece of technology needs a visual user interface. Content is output from the computer and displayed on the screen. The UI enables users to interact with or change the content and the underlying data.
- Will describes the process of using a programming language to model how content show be displayed on a screen. The model is stored in an object and rendered in the application or browser. This representation is referred to as the DOM: Document Object Model.
- Will walks through the fundamentals of rendering content in the browser. The markup written in HTML is represented as an object in C++ and then rendered to the document.
- Will introduces the CSS Object Model (CSSOM), which represents the styling instructions in a similar way as the Document Object Model.
- Will describes how the UI represents the underlying data in the application. When a user interacts with the page, a change in data is triggered. The change in data subsequently causes the rendering engine to update the UI.
JavaScript, DOM & Events
Section Duration: 1 hour, 36 minutes
- Will diagrams the relationship between the HTML parser, browser, and JavaScript engine. Once the HTML content is parsed, it cannot be changed without using JavaScript. The list of DOM elements is stored in a C++ data structure, and any new data or changes reside in JavaScript.
- Will explains that WebCore is the C++ representation of the DOM and gives JavaScript access to the pixels to display data. WebIDL is a standardized way for browser features to interact with the JavaScript engine.
- Will walks through the flow of data when JavaScript is used to update the content on the page. JavaScript APIs pass data to the C++ DOM list through the WebIDL abstraction layer.
- Will summarizes the process of displaying content and data. JavaScript creates and stores data and accesses DOM elements through the document API. Getters and setters can access and change the DOM element values.
- Will shares a new code snippet that selects elements in the DOM and creates an event handler for responding to user interaction. The HTML code is interpreted and stored in the C++ DOM list. The document API is used to store references to these elements in JavaScript.
- Will diagrams how memory is allocated for the handleInput function. Since the function is a callback and not immediately invoked, the function's implementation is stored as an object in C++ and will be called once the corresponding user event is triggered.
- Will explains how DOM updates are performed as users interact with the elements on the page. As a user types in the input field, the callback stored in C++ is triggered, and the JavaScript function is invoked. The value in C++ is accessed and passed to the textContent setter.
- Will provides a summary of the data flow as users interact with the DOM elements on the screen. Questions about the relationship between C++ and JavaScript and the microtask queue are also discussed in this lesson.
Data-Binding in the UI
Section Duration: 1 hour, 32 minutes
- Will introduces one-way data binding. It's a popular paradigm for handling the UI engineering challenge of maintaining consistency between the UI and underlying data.
- Will walks through how the view is updated when a user interaction triggers a change in data. JavaScript accessor methods mutate the underlying data in the C++ DOM. When the underlying data is changed, the pixels in the display are redrawn.
- Will revisits user interaction by diagraming the data flow as the next user interaction is triggered. In this case, handleInput function is triggered by a user typing text into the HTML input element.
- Will explains the benefit of decoupling data changes and view updates. Isolating view updates to a single function reduces duplication and makes the code more maintainable. As changes in data occur, the dataToView function will be called.
- Will begins diagramming the application with the new dataToView function, which will handle all view updates regardless of the user interaction.
- Will continues the application schematic that uses the new dataToView function. The function runs initially to update the UI with default data creating a one-way data binding relationship between updates to the JavaScript data and the view.
- Will completes the application schematic for one-way data binding by adding user interactions. The handleClick and handleInput functions are triggered by the user. The C++ DOM calls the callback function, which updates the data and triggers the dataToView function to rerun.
- Will summarizes the predictable flow of data and view updates created by this design pattern. The code is then refactored to run the dataToView function as a callback to setInterval so the function can be run continuously.
Virtual DOM
Section Duration: 2 hours, 36 minutes
- Will introduces the Virtual DOM, which represents the visual elements displayed on the screen. By storing this representation in JavaScript, previous versions of the display can be compared to the current representation allowing only the updated elements to be re-rendered. This would increase the performance of the display engine by reducing unnecessary display updates.
- Will begins diagramming the UI elements and underlying data structures for the next code example. An input element will receive input from the user. The event handler will update the JavaScript data.
- Will continues the auto-updating view example. The setInterval method will repeatedly call the dataToView function allowing the UI to be automatically updated rather than requiring the function to be called manually.
- Will introduces the concept of a UI Component, a function that fully creates elements and the logic to bind the data and view layers. The dataToView function will include this behavior by creating the input and div elements if they do not exist, then updating their displayed values.
- Will diagrams the initial setup of the UI Component code example. Like the previous code versions, global variables are declared to store data in JavaScript memory. Since the dataToView function handles the creation of the elements, the only initial element stored in C++ is the body element.
- Will walks through the execution context of the first call to the dataToView function. The ternary operation determines no input is present, so the DIV element is yet created. The onInput handler is also established.
- Will completes the UI Component example by walking through the effects of user interaction. The setInterval function calls dataToView repeatedly. As a user interacts with the input element, both the input and DIV elements are updated.
- Will demonstrates how string interpolation will be used to create more visual code. The closer code is to its visual representation on screen, the better the developer experience.
- Will explains a Virtual DOM consists of blocks of code representing each piece of view. These blocks are stored in a JavaScript array, and a function is created to accept an array of data and produce DOM elements.
- Will incorporates user input into the virtual DOM example. The handle callback function responds to the user interacting with the input element and updates the data in JavaScript. When the updateDOM function is called, the DOM is reconstructed with the updated data.
- Will summarizes the paradigm of declarative user interfaces, which store visual representations of the elements on screen in data.
Composition & Functional Components
Section Duration: 1 hour, 6 minutes
- Will discusses the value of using lists when constructing user interfaces. Lists allow the execution of code to be decoupled from the number of elements displayed. The Array.map method will iterate through however many elements are present.
- Will begins diagramming a more flexible virtual DOM. The flexibility comes from the Array.map method, which iterates through the data returned from the createDOM function. Each element in the list is passed to the convert function to generate a DOM element.
- Will introduces the Event API, which provides getters for accessing properties like the x and y of the mouse or if a key is pressed during the event. The most important property for the handle method will be the target property because it references the input element triggering the event. This creates element-flexible code with fewer dependencies.
- Will reviews the Event API usage and the flexibility it adds to the application. Leveraging an Array for storing the list of elements on the screen allows for semi-visual coding and more composable user interfaces.
- Will refactors the createDOM function further by creating a Post function which generates DIV elements from an Array of posts. This technique is a more concise way to create multiple elements of a similar type.
Performance Improvements
Section Duration: 1 hour, 16 minutes
- Will discusses how the code can be refactored to allow for automatic UI updates without relying on a loop or iterating through the list of VDOM elements. The task of updating data is abstracted into a function where the updateDOM function can be called after the data is changed. This ensures the visual representation of the data will always be in sync with the underlying data.
- Will introduces a hook, which is a function added to the application to tap into and modify the behavior of an existing system or component. Hooks are commonly used in frameworks and libraries to provide extensibility and customization options.
- Will explains the performance gains achieved by leveraging diffing. Rather than recreate the entire DOM when data changes, a findDiff function compares the new version of the DOM to the previous version and will only update the elements that changed.
- Will creates the schematic for the DOM diffing code example. Like previous examples, the HTML is loaded in the browser, and the JS is executed. The DOM will be represented in the C++ DOM and links from JavaScript to the C++ DOM are established.
- Will walks through the refactored updateDOM function, which conditionally updates the DOM based on if the elements Array exists. The first time the function is called, the Array is undefined. The elements are mapped over and passed to the convert function before being appended to the DOM.
- Will demonstrates how the code example handles user interaction. The handle function updates the JavaScript data as the user inputs values into the field. The setInterval timer calls updateDOM to re-create the virtual DOM and prepare the diffing algorithm
- Will integrates the diffing algorithm, increasing the application's performance by only re-rendering the changed elements. The UI is more composable and is built through a semi-visual process.
Wrapping Up
Section Duration: 3 minutes
- Will concludes the course with a final summary of the UI approaches achieved throughout the course. They create a single source of truth for data, UI composition, and VDOM diffing for increased efficiency.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops