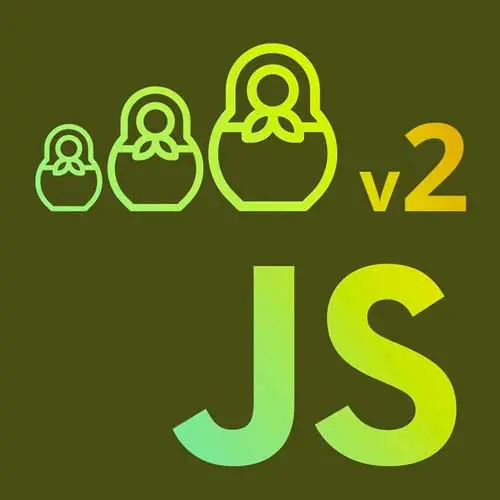
Lesson Description
The "What is Functional Programming" Lesson is part of the full, Functional JavaScript First Steps, v2 course featured in this preview video. Here's what you'd learn in this lesson:
Anjana shares some of the terms associated with functional programming, like higher-order, monoid, and functor. These terms can be confusing to developers new to functional programming. Along with functional programming, other programming styles exist, like imperative, declarative, and object-oriented. This course aims to outline the differences between these styles and dive into the functional programming concepts.
Transcript from the "What is Functional Programming" Lesson
[00:00:00]
>> Anjana Vakil: So as I mentioned, the most important concept here that we're gonna be talking about the whole day or the whole lifetime that we do functional programming is the notion of a pure function. So in some of the course materials here, you'll notice these little slides link, that will bring you to the presentation slides that I'm gonna be walking through as we go.
[00:00:24]
And then we'll jump back to the exercises after we go through each section. We are here because we have heard that it is a thing, [LAUGH] but we wanna understand better what it is. And there's a lot of different ways that I can answer this question. What is functional programming?
[00:00:40]
For one thing, it's certainly a trend, in a sense, it's become a lot more en vogue, if you will, 90s reference to [LAUGH] web development, in particular. And in the last maybe decade or two, it has become a lot more present in kind of the practical day to day world of software development outside of the web, as well as on the web.
[00:01:08]
But it is not a flash in the pan kind of trend. It is something that has been around since before digital computers even existed. It existed as a mathematical construct way back in the 30s when Alonzo Church developed the lambda calculus, which I am happy to dig into after the course, or if folks have questions.
[00:01:31]
But this is something that has been important to theoretical computer science for 100 years. So it's not going anywhere, don't worry, but the buzzwords are real. There is a lot of jargon in functional land. And you'll hear words that sound like they should make sense, like higher order, but what does that mean, or referential transparency.
[00:01:57]
And you'll hear words that you may have never encountered before, like monoid or functor, or maybe you did record some of those in your very advanced math classes. But we're not gonna be focusing too much on this jargon, because what is the jargon important about? What is this lambda symbol anyway, this thing that's in every logo of functional programming languages?
[00:02:20]
What does this all mean? So today, although at some point you will wanna go and Wikipedia these various terms, we're not gonna be focused on the jargon so much as the concepts. Because that is what we want to be taking away as not, I know a bunch of fancy words that I can use to sound smart.
[00:02:39]
But I know a bunch of concepts that I can use to write code that doesn't break on me. [LAUGH] So that's the goal here. We can also define functional programming as a coding style. Some people describe it as a functional style of programming. And that style of programming is supported in certain languages and not supported in others.
[00:02:59]
Now I'm not just talking about style in terms of syntax or naming conventions or what have you, but really a way of kind of thinking about the program, which we will dig into throughout this course. But in terms of the languages that support it, there are a whole bunch of languages out there that are what are typically considered functional languages like Haskell, or Clojure, or the Elm language family, OCaml.
[00:03:24]
There are also some that are specifically developed for the web, like Elm or Elixir, which is based on Erlang, things like that. But we don't need any of those other bajillion languages cuz we have a great functional programming language right in front of us in our browser, and that's JavaScript.
[00:03:42]
Now, was it designed to be a purely functional language like some of these other languages? Not really, has it acquired some less functional characteristics over the years, perhaps. But we can still do totally valid functional programming in JavaScript, because all we need is a function. So that's what we're gonna be digging into.
[00:04:03]
But as I mentioned, if you would like to continue your journey after this course, there are some other cool languages and toolkits and things like that that you can explore. Today, we are in functional JavaScript land exclusively. So I mentioned before that this is more than just a code style in the sense of syntax or linting.
[00:04:25]
This is a way of thinking about our programs. So another word for that is a programming paradigm. A paradigm, in general, is some kind of worldview or kind of meta-level understanding of what this entire world, or in our programming use case, what the entire world of the program is made up of.
[00:04:48]
What are the things inside of a program? How do they interact with each other? And there are a whole bunch of different ways of thinking about computer programs that are called different programming paradigms. So you might have heard of certain paradigms, like functional programming is one. It's often contrasted with object-oriented programming, which perhaps is more familiar to a lot of folks, especially if anyone is coming out of languages like Python or Java or that sort of thing.
[00:05:18]
And we can do both object-oriented and functional programming in JavaScript, arguably. But let us take a moment to step back and consider, what is a paradigm, and how do these paradigms compare? So, a lot of the time, we're used to writing code in JavaScript in an imperative paradigm.
[00:05:39]
So imperative programming is sort of a higher class of paradigm that includes object-oriented programming. It's sort of a special case of imperative programming. In imperative programming, the program is made up of commands. I tell the computer, do this, do that, assign this variable value of this, assign this function the value of that, call this function on this variable, etc.
[00:06:05]
So I'm giving a list of instructions to the computer, and the computer is executing what I told it to do. So I am telling the computer how it should execute step by step. That's in contrast to another kind of higher level or meta programming paradigm that we referred to as declarative programming, which functional programming is a special case of.
[00:06:31]
So in declarative programming, unlike in imperative programming where the work that I do as a developer is to give the computer commands and tell it how I want the program to run. In declarative programming, I just tell the computer kind of the output or the result that I would like, and I let it figure out how it's gonna get to that.
[00:06:56]
So has anybody encountered a declarative programming language before? Spoiler alert, you totally have, things like HTML. It's declarative, it doesn't say, hey, here's how you should cascade all of my style sheets or what have you, or here's how you should load all of my links. It just says, this is the page I wanna see, browser, please, [LAUGH] give it to me.
[00:07:25]
Or what about SQL? Any backend folks around? SQL is another example. SQL is a database querying language that is also a classic example of a declarative language where we don't tell the computer how to execute a lookup or a select or a join. We just tell it what we would like to have, and we let the underlying implementation figure out the details.
[00:07:52]
Now this is all to say that when we're doing functional programming, we're less concerned with thinking about the program as step by step, do this, then do that. We are more concerned with thinking about the program as a single transformation of input into output. So we're gonna spend a lot more time talking about how that works?
[00:08:17]
What it feels like, what tools we need to do it, etc. So functional programming, as I mentioned, is sort of a subset of declarative programming. And in object-oriented programming, we are focused on essentially taking our state, meaning information or values that changes over time throughout the course of the program, and wrapping it up in little objects.
[00:08:49]
And then having those objects do things like call methods on each other so that they can send and receive information. In functional programming, we're gonna be wrapping everything up in the unit of a function. That is really the only thing that we need to do functional programming is one construct, a pure function.
[00:09:09]
So that is what we're gonna spend the entire course digging into the intricacies about. So, I wanna give a shout out to a, it's a bit old now, but an article that kind of inspired this whole course called an introduction to functional programming by Mary Rose Cook. It came on publication from the Recurse Center, which is an amazing programming community in New York and virtual that I was a part of, in about, or I continue to be a part of.
[00:09:42]
But I attended this programming retreat, they call it, in about 2015. And worked with Mary Rose Cook, who happened to be an excellent teacher of the basic principles of functional programming without getting caught up in all of the jargon. Now this article, which you can read through the links, it's Python-based, but the principles are essentially the same.
[00:10:03]
So I just wanna give a huge shout out to Mary Rose Cook and the entire Recurse Center crew, who essentially taught me functional programming in the world of JavaScript using the principles that we're gonna go through in this course. So, there's some fun further reading links at the end.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops