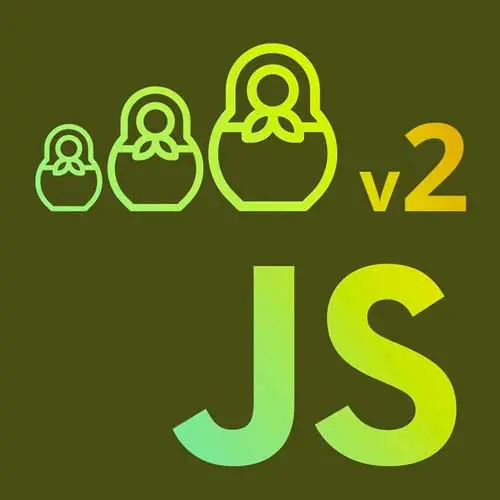
Lesson Description
The "Pure Functions Exercise" Lesson is part of the full, Functional JavaScript First Steps, v2 course featured in this preview video. Here's what you'd learn in this lesson:
Students are instructed to determine if a function is pure or impure. The solve button in the Stackblitz exercise can be clicked to show the correct answers.
Transcript from the "Pure Functions Exercise" Lesson
[00:00:00]
>> Anjana Vakil: If we go through, and we have just a note on this tutorial kit set up here, there's a kind of drop-down at the top where you can see all of the course. And then you'll have these little left and right buttons to go through the sections. So we discussed pure functions, and just to recap, there are essentially two defining characteristics of a pure function.
[00:00:23]
It does not do anything besides return its return value, meaning it has no side effects, like logging to the console, or maybe writing to a file, or posting something to an HTTP endpoint. What have you. It also is deterministic, which is another way of saying it only depends on its input arguments.
[00:00:50]
So if it depends on some other state of the world, like the weather outside the time of day, it is what happens to be the value of a certain variable at this point in the program. Then that means, like we said before in our impure greet function, I can call the function multiple times with the same inputs and I might get different outputs.
[00:01:10]
That means it's a non deterministic function. In other words, I can't predict what the output of the function is gonna be just from its inputs. So that is not a pure function, and what we want in pure functions is for them to be 100% deterministic. If I call this function a million times on a million days, is that even possible in a human lifetime?
[00:01:32]
[LAUGH] with the same input, I will always get the same output, okay? So we are going to take these considerations. And use them to work through a little reading exercise, essentially. So in this next section, pure or not, you've got a little function file here, pure or not JS, which has a bunch of little and some of them.
[00:02:00]
Different types of functions in here. And your mission should you choose to accept it is to read through these and then in the comments, think through and decide whether each function is pure or not, and just write for yourself, mostly a few words about why that is.
>> Anjana Vakil: All righty, so I am going to do to commit a sin of teaching right now where I am going to do something that I want you to know is possible but I do not want you to do right now.
[00:02:36]
Okay, you will notice that the little solve button up in the right corner. Don't click it cuz it will replace what you've written in your editor with my reference solution. So this is gonna become a little bit more useful as we work with actually executing code samples. But right now I'm gonna click it so you can see my answers and then we will discuss and compare.
[00:02:58]
Sound good? So there are some spoilers, but let's go through these functions one at a time, so get time. Hopefully everyone is on the same page, so this function is not pure, yeah, is this what folks have said? Okay, can anybody tell me why they thought so yeah.
[00:03:17]
>> Speaker 2: It's not deterministic.
>> Anjana Vakil: Non deterministic if I call it a different times, I will get a different time because it's the time. Okay, how about compare date strings? What about this one? Do we agree that it is, as I labeled it, pure? Okay, now in this case, is there anything?
[00:03:42]
Did anybody have a different answer? Did anybody have any question marks around, wonderings about this one? Because there is one thing that's a little eyebrow raising in this function,
>> Speaker 3: I assume you have to rely on the fact that day would be purely functional as well.
>> Anjana Vakil: So date, this date function, which here, if we notice, is not passed in as an argument.
[00:04:07]
So that is something outside of the function. But this is a built-in in the language. So we can be pretty reasonably sure it's not gonna change between lines of code execution. And we have to know whether or not. We have to know its behavior. We have to know whether or not this is going to always give me the same result if I pass in the same string.
[00:04:27]
And in this case, it will. So there are some asterisks on the never look at anything else in the rest of the world that I said before, for example, built ins are something that we'll be using, and there's some more in the rest of this, right, as we'll go through.
[00:04:46]
The link on the on the side indicates MDN is a web developer's best friend. And so if we wanna find out what a particular built in does, we can go to MDN to check out if it's going to be a deterministic pure operation or not. Cool, all righty how about string to JSON?
[00:05:10]
Are we all on the same page? This one is similarly pure. Again, we have that JSON built in, but we can rely on it being predictable. So, sweet, bow about file to JSON I'm seeing thumbs down. I am seeing disgusted faces. [LAUGH] can anybody explain what is not pure about this?
[00:05:36]
>> Anjana Vakil: File to JSON?
>> Speaker 4: It depends on a file that's outside?
>> Anjana Vakil: It depends on something outside of the function. This file that I'm looking up by file name, anything could happen to that between runs to this function. So if I run it today, and then tonight my coworker edits the file.
[00:05:58]
Cool, and then tomorrow I run it again, expecting the same output, I will be very upset. So this is what we want to avoid by not doing things like this. And we'll talk about momentarily how we actually do anything [LAUGH] when we can't do this stuff. Okay, add pretty pure, pretty simple sum question marks.
[00:06:24]
So I'm going to put an asterisk on this one. Did anybody have a different answer to some here? Now, I am saying this is impure, but there is an asterisk on that because we're gonnna talk a little bit later about how to work with data that we don't want to ever change.
[00:06:44]
But in JavaScript, by default,
>> Anjana Vakil: Even though I'm taking in this num array as the argument, this function is still not pure. Because it's not necessarily deterministic. Can anybody help me explain why? Why might this not be deterministic? What could happen that could ruin our functional purity here?
[00:07:13]
The thing is, this array, even though it's being passed in, and I'm looking at it, arrays are something that my coworker over on the other team can mess with, right? Arrays are what we call mutable in JavaScript. So if I'm expecting this function to return the same result tomorrow as it does today.
[00:07:34]
But then it turns out that my coworker pushed a whole bunch of new values to that array, even though I'm passing in the same array object, I might get a different value. Now that's a gotcha. So there's a little tricky one. I'm asking you, but I think that if we can force ourselves to work with arrays that we know will never change, which we will talk about how to do later in the course.
[00:08:04]
Then we could consider this a pure function. Logical XOR, this is a logical operator exclusive or we got a little arrow fat arrow syntax here going on. But that doesn't change the purity. And as we can see, it's a pretty simple series of Boolean evaluations. So does everybody agree with me that this is a pure one?
[00:08:28]
Cool, truth table? Anybody see anything weird going on in here that I just said was a bad idea? [LAUGH]
>> Speaker 5: Arrays.
>> Anjana Vakil: Yeah, so we got arrays going on and we got this push operation, right? Which is changes the actual array. We're pushing new values to it. However, all of that is happening inside of the function.
[00:08:55]
So this isn't something that's gonna be giving us different results at different times. So we can have in the JavaScript case, not in languages that enforce functional purity, but in JavaScript which does not force you to write pure functions, you can have a pure function that is deterministic.
[00:09:15]
And has no side effects, that contains less functional things like mutation, but as long as we're wrapping it up in the purity of the functional definition, we can still be productive functional programmers. So this is what I think is awesome about doing functional programming in JavaScript. We don't have to go 100%, it's not all or nothing.
[00:09:38]
We can start functionalizing our code little by little, wrapping things up to make sure that if we're doing any impure things, they're at least happening in the safe, little sandbox inside of the function. And we'll be talking more about that in one moment. Okay, now what about this one, the XOR truth table that uses our XOR and our truth table to do something.
[00:10:07]
Why is this not a pure function?
>> Speaker 6: Log into the console.
>> Anjana Vakil: 110 into the console. We already saw it side effects. Now, if I were returning a string representing the value of the truth table itself, that would be a different matter. But logging to the console, even though this is gonna do the exact same thing every time I run it, unless somebody replaces, redefines console.
[00:10:30]
Or whatever, which, again, JavaScript doesn't force us to do functional things, we are going to be getting the same output every time but it is an effect on the world, and that is not pure cool. Okay, now we're getting into a little bit more realistic code. So some of this is is actual, like real world code from GitHub.
[00:10:55]
And again, we're using some built ins, or some things that are. Kind of not passed into the functions, like encode URI component. So, we might want to refer to our good friend MDN to check on any of that, but when we look at this encode string, and feel free to check my facts.
[00:11:19]
But encodeuri component is a pure deterministic function that's always going to return the same encoded.
>> Anjana Vakil: String based on the same input string or based on its given input string. So in this case, we are using a pure function inside of our function and doing nothing else, so we have purity.
[00:11:46]
>> Anjana Vakil: Now we have encodeArray, which with that same asterisk similar to before, where we're passing in an array value, and then we're doing something with it. We're not changing it, But my coworker might be in some other code. That's part of this. So that's the gotcha here. And then we come to the last one in code value.
[00:12:13]
So did folks think this was pure, impure? I thought it was a zoom here, perhaps you can help me understand your reasoning. If you got the same answer, yep,
>> Speaker 7: It's calling encode array which is above it,
>> Anjana Vakil: Bingo, so encode array we just said with that, gotcha of arrays being mutable in JavaScript and we could consider an impure function.
[00:12:42]
And so when we use an impure function, it kind of infects whatever function we're using it in. So this encode value is infected by the impurity of ENCODE array, and therefore also becomes impure Here. So one little impurity is enough to ruin the impurity of an entire function or program even.
[00:13:08]
And that's a challenge, right? How do we do real things when we can't log to the console, [LAUGH] we can't write the files, we can't read files, [LAUGH] we can. Can't make fetch requests like, what are we supposed to do? And that's perhaps one of the major like?
[00:13:28]
We need to wrap our heads around as functional programmers.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops