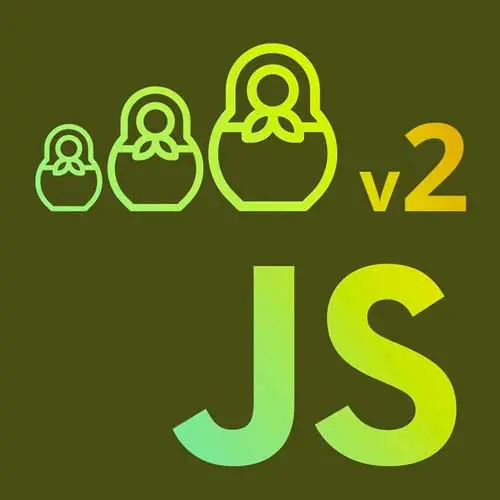
Lesson Description
The "Introduction" Lesson is part of the full, Functional JavaScript First Steps, v2 course featured in this preview video. Here's what you'd learn in this lesson:
Anjana Vakil begins the course with an outline of the functional programming topics that will be discussed. The notes and exercises for the course are available in the browser. A repo is provided to allow the exercises to be completed locally.
Transcript from the "Introduction" Lesson
[00:00:00]
>> Anjana Vakil: My name is Anjana Vakil. My name is Anjana Vakil. The first version came out in 2020, like eight lifetimes ago, right? And so very excited to be back and to be re-upping this course on functional programming principles. Now I will say functional programming principles haven't changed a lot since, well, the 1930s, let alone 2020.
[00:00:30]
My name is Anjana Vakil. And the hope is that after taking this course, you'll be really well set up to go on and continue the entire rest of the functional programming learning path on the other Frontend masters courses that go a lot more in depth into some of these topics and also some of the tooling around it.
[00:01:01]
So today, what we're gonna be focusing on is using vanilla JavaScript, just the JavaScript we know and love, mostly, to get into our head the conceptual framework, if you will, not in the React sense, but in the programming paradigm sense. That we're gonna need to start using functional principles in our JavaScript code basis.
[00:01:25]
So a little a note on the materials. You can find all of the materials for this course at functional-first-steps.netlify.app. This is a course website built in tutorial kit which is a a product put out by a stack blitz, so we're gonna be working through the whole course right in the browser.
[00:01:46]
You're not gonna need to download anything or install anything. But if you would like to there some instructions at the bottom of the the main page here on where you can find the repository and download the source code and why not if you would like to but right now we're just gonna all be working through these exercises in the browser.
[00:02:02]
So we'll see how that goes as we move on. So you might be wondering why I'm I here? What I'm I doing in this course? What are the life choices that have led me to this moment? And perhaps those life choices also include things like becoming a developer, starting to get your kind of feet under you.
[00:02:24]
But realizing that as your programs get more and more complex, that complexity is increasingly difficult to handle, and wondering if there might be better ways of structuring your programs and of structuring your code. So that you can be more productive, you can write more maintainable code, code that's easier to predict, to test, to debug, etc.
[00:02:44]
So you might be wondering, how can I just get better at JavaScript? And I think that functional programming is one of the tools that we as JavaScript programmers can use to make sure that our JavaScript is the best it can be. So, you might have been hearing about terms from Functional Programming World, or hearing about Functional Programming is sort of the only way real programmers work.
[00:03:10]
That's not what we're gonna be doing today, we're gonna be exploring it as a way. So, what we're gonna be looking through is kind of these questions like what is functional programming. I love the high level big picture. What does it all mean, ma'am? So we're gonna be talking about that, and then we're gonna also be talking about how code actually looks in functional programming, and how it feels to be writing functional code.
[00:03:34]
We are gonna be doing everything in JavaScript because you can actually do functional programming in JavaScript without having to learn a whole new language like Haskell or Clojure, or one of these, what are more typically considered functional programming languages. So if you've been wondering like, what is this functional programming thing, why are so many of my dev heroes so obsessed with it?
[00:03:55]
What does it all mean? Can it even happen in JavaScript? That's what we're gonna be looking at today. And the answer to the JavaScript question is heck, yeah, it can. So what we're gonna be doing is we're gonna be exploring again the fundamentals. They're really the ground floor, the first steps is what this course is taking.
[00:04:15]
And as I mentioned, there's a whole bunch of awesome deep dive courses on the platform that you can continue your learning journey with. These are really just the first steps, they are not all of the steps. So what we'll be doing is we will be looking at concepts like pure functions, higher order functions, first class functions, recursion.
[00:04:38]
And function composition and all these other jargony words that you might've heard about. So we're gonna be exploring them as we go. So as I mentioned, everything here is in the browser. You don't need to check out any code locally, but if you'd like to, there are instructions here.
[00:04:54]
So let's take a look at the plan for this course. We're gonna start out by talking about the single most important concept, if you take nothing else away from this course, all I would like you to go home with, if there's only one thing you get to go home with, hopefully it's many.
[00:05:16]
The most important idea for functional programming is the idea of a pure function. So we're gonna spend a lot of time talking about that. And then we're gonna move on to some more kind of techniques that we use in functional programming that are all based around this concept of a pure function.
[00:05:29]
We're gonna talk about what purity is and what it is not, and how we manage the real world in a pure functional program. So we can actually do useful things, and we'll talk about why that is a challenge for functional programming. Then we'll look at those techniques like recursion, for example, as an alternative to iteration for doing an operation multiple times.
[00:05:54]
So we're gonna be writing some recursive functions and having some fun bending our minds on that front. We're also gonna talk about higher order functions. What they are? There are a bunch that you already know and love, and if you're a JavaScript programmer, chances are you've already used, or probably even written a higher order function.
[00:06:15]
You just might not know it yet. So, we'll talk about that. We'll also talk about some of the functional programmers best friends, which are functions like reduce, map and filter. So no, we're not gonna just be relying on JavaScript to give us these methods. We're gonna re implement them ourselves so we understand how they work in the functional world.
[00:06:34]
We're also gonna talk about techniques such as writing closures. So what that is a certain type of function and how it lets us kind of remember data throughout our program. And we'll talk about composing functions together to build pipelines through which our data flows, making our programs essentially like one long string of data transformations.
[00:06:57]
So we'll talk about functional composition and how we put together pipelines. And we will also spend a little bit of time talking about how important immutable data is for functional programming and why. And we'll look at a couple of ways, or at least one library that we can use to help us get that functionality into JavaScript, which doesn't natively support the kind of immutable data that functional programmers like to work with.
[00:07:25]
Now the question is, why? Why would we go through all of this pain of learning jargon terms and perhaps even different languages? And why do programmers do this? Why do we burden our minds with so many different paradigms?
>> Anjana Vakil: Well, the thing is, if a function is not looking at anything about the world other than its inputs, and it's not doing anything to the world other than returning its output, that function becomes a lot more predictable.
[00:07:52]
It becomes a lot easier to know exactly what's gonna happen when I call this function, becomes a lot easier to test that, and it also means that I'm gonna have less surprises, when I am working in complex applications. And somebody, my co-worker just changed out the value of the name variable from under me and I didn't know that and I was relying on it being the original value, bla, bla, bla, why do I have this bug?
[00:08:18]
This are the types of headaches we wanna avoid by making sure all of our functions are pure, and this helps us write more fault tolerant and more predictable, more testable, more debuggable programs. Is the hope and the goal. And yes, like I said, that has implications for our day to day as developers.
[00:08:40]
So the hope is that if we work in a pure functional world, it helps us kind of move faster. Because we don't have to be doing things like figuring out what the state of the program must be at this particular line in its execution and how that might be affected by the weather outside or the time of day or whatever.
[00:08:59]
We just know this function is gonna do exactly one thing, and I can verify that it's doing what I thought it was gonna do.
>> Anjana Vakil: Some reasons that some other people use for doing functional programming is to feel smart and put down other programmers by saying that the way that we functional programmers do it is the best way, or sometimes even the only way.
[00:09:25]
None of that. We don't need any of that. We can be perfectly productive programmers without using functional programming. What we're interested in are the concrete benefits for our life as developers, the headaches it can save us, the years of life that it can give us back by not stressing us out over mutable variables, things like that.
[00:09:48]
So this why I think we should use functional JavaScript, is because of those concrete benefits. Like I mentioned, one of the motivations that I have for learning functional programming was I was starting to learn JavaScript about 10 years ago, geez. And I was getting a lot of headaches, I was like used to a different object oriented language at the time, I was a Python developer, and I was trying to map those principles to JavaScript.
[00:10:20]
And I was like, what is happening? We have prototypes. There's this keyword everywhere, and I got to memorize a bunch of rules for it and what's going on. So I personally think that doing object oriented programming in JavaScript gets pretty tricky, pretty fast. And I found that doing things on a functional style, or at least a more functional style, helped me worry less and program more, [LAUGH] let's put it that way.
[00:10:51]
So I think that functional JavaScript makes a ton of sense. There's also a lot of people doing functional programming in JavaScript, even if they might not advertise it as such. So there is a ton of community resources, libraries, tools, frameworks, for example, React. Any React users out there?
[00:11:14]
A couple hands. Is heavily inspired atleast in its hook-based instance from principles of functional programming. And the entire way that React apps work with this reactive updates and the declarative way that we say, I want the element to look like this is very much in line with functional programming.
[00:11:37]
So functional programming has had a pretty big influence on the web community, especially in the last 10 years or so. So it's great to be doing a style of programming in a setting where you have other people around you, other community resources, other courses, other entire learning paths on Frontend Masters to help support you through that journey.
[00:11:59]
So that's, I think another great reason that JavaScript makes sense as a first step for functional programming, even if you go on to get super into the category theory and the monoids and the functors and the haskells and the closures. Even if you go on to do all of that, I think JavaScript is a great place to explore the fundamentals because it has everything we need, it's right in the browser, and we have this whole community to help us learn.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops