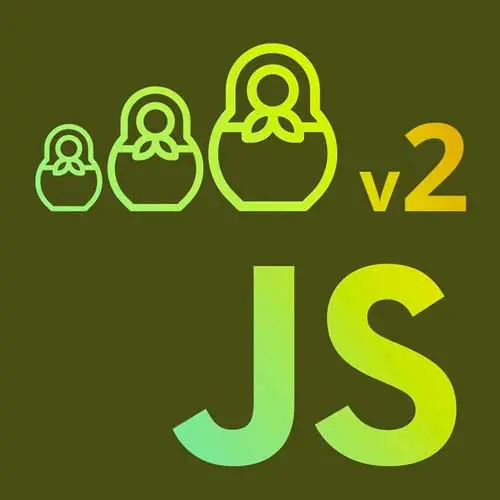
Lesson Description
The "Immutable Data with Immer.js" Lesson is part of the full, Functional JavaScript First Steps, v2 course featured in this preview video. Here's what you'd learn in this lesson:
Anjana introduces Immer.js, a library that works with immutable data in JavaScript. Immer provides APIs for working with Arrays and Objects using familiar JavaScript methods, but has reimplemented them to be immutable.
Transcript from the "Immutable Data with Immer.js" Lesson
[00:00:00]
>> Anjana Vakil: Now let's come back to that,
>> Anjana Vakil: Question of immutable data.
>> Anjana Vakil: As I mentioned, arrays in JavaScript are mutable, right? We know that we can mess with the contents of an array, and that's not great if we want the guarantees, and the confidence that comes with knowing that once I have created some data, nobody else can mess with it.
[00:00:27]
And so there are solutions for doing that in JavaScript libraries that provide these type of data structures. And there's a bunch of different choices. There are more links in the end of the course, but one really popular one is called immer, which Immer in German means always. And so this gets into the persistence, which is another word you hear used a lot with these immutable data structures, persistent data structures, they never change.
[00:00:56]
Once you have created it, it's like a const declaration. It's like assigning const to a string. Once that has been declared, no one else shall change it. So Immer is one choice. There's a bunch of others. I'll let you read through the docs and everything on your own.
[00:01:16]
But how does this work? Okay, so a cool thing about Immer is that you don't have to do all these mental gymnastics of like spreading the slice and the mapping the thing and whatever. You can actually still just use array.push. But Anjana, didn't you just say that push is a mutating method and therefore is super dangerous?
[00:01:39]
Yes, if I was calling it in a regular JavaScript land, but here I'm calling it inside of a callback function, first class function. There is a produce function exported by Immer.
>> Anjana Vakil: That works kind of similar to a lot of our functional utilities. What it does is it takes in an array and a function that describes but does not yet do whatever the transformations are that you would like to make to this array.
[00:02:14]
By convention, the input is sometimes called draft, or usually it's called draft. It's like a draft of what you're gonna return, and it starts out being identical to the input. And then you can mess with it inside this function body, but under the hood, MR is managing the immutability for you using some fancy data structures and what not, and you can read how it all works on their documentation site.
[00:02:40]
There's a whole blog about it, it's pretty cool. That allows us to write code as if we're mutating stuff, but because it is isolated, that mutation, what we think is a mutation, under the hood is maybe not a mutation, is isolated in this function. That then gets applied to the older array to produce a new array, for example.
[00:03:04]
So, real quick, let's rewrite these in an Immerized way [LAUGH]. So, instead of, in push, instead of, returning the stuff we just had. Well, we have this example already right here, basically. [LAUGH] So we can,
>> Anjana Vakil: Turn, yeah. Well, we don't wanna push always four, we wanna push the element to the draft inside of the callback.
[00:03:39]
Now, I'm just gonna walk us through this just as an example of how one implementation here of persistent data structures and of immutable data in JavaScript lets us do this. But you get the idea, it's gonna be pretty similar for the rest of these, right? So if I have return, oops, I'm supposed to be returning this and I am going to be returning here we are updating so instead of push I want to do an assignmen, right?
[00:04:16]
So draft index =new value. All the same stuff that we're used to doing in JavaScript magically gets immutablised under the hood. Now for pop, we just need to think a little bit more carefully about how we would like the equivalent to pop in yeah. Here, we're not really doing, like, myArray.pop.
[00:04:42]
We want to return, again, the array with everything except the popped thing, if that makes sense. So we could do something like.
>> Student: In both cases, you want array instead of oldArray.
>> Anjana Vakil: Oops, thank you. Okay, so instead of assigning here, we are going to maybe just pop it.
[00:05:10]
And then we can return draft. But actually, Immer is returning draft for us. So that's why we didn't need to have returns statements and the other things. Immer is taking that draft and returning it for us. All right, so we now have, yeah, and you can look through the test, but it's just it's just a demonstration of this API.
[00:05:30]
This is just one way of doing it, but a lot of people really like Immer. There's some other solutions that have totally different APIs, like for example, immutable JS is a library that the artists formerly known as Facebook put out that, Does the same underlying thing, but the way that you would work with it is a lot more different and you have to remember to always make your arrays, special immutable arrays.
[00:05:58]
>> Anjana Vakil: Immer is cool and a lot of people like it because it lets you just pretend everything is a regular JavaScript array, and it just does the magic for you of making sure nothing gets mutated. All right, so this is all to say if you wanna work with immutable data, which you probably will if you end up doing a lot of functional programming and seeing how slow it is to copy everything all the time and avoid mutation that way.
[00:06:19]
There are solutions in the JavaScript ecosystem for that.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops