Immutable Array Operations Exercise
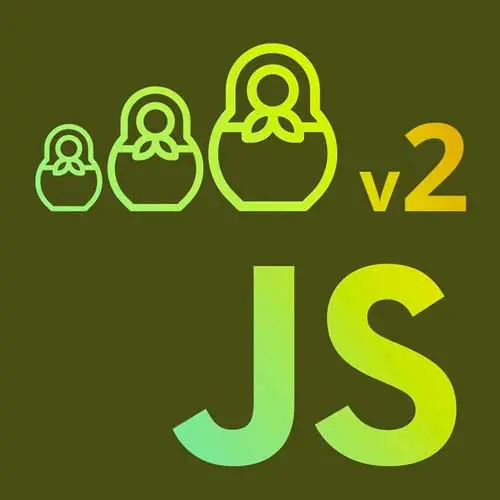
Lesson Description
The "Immutable Array Operations Exercise" Lesson is part of the full, Functional JavaScript First Steps, v2 course featured in this preview video. Here's what you'd learn in this lesson:
Students are instructed to implement an immutable version of the push and pop array methods.
Transcript from the "Immutable Array Operations Exercise" Lesson
[00:00:00]
>> Anjana Vakil: What we're gonna do in our next exercise is think about how we would create non-mutating alternatives to some of the mutating utilities that we're used to working with all the time. So for example, we're used to pushing elements to an array that mutates the original array. We're used to maybe popping something or updating, assigning a new value to a given index.
[00:00:34]
What would it feel like if we couldn't do all that mutation, what would we do differently? How would we return instead of a original object that's been changed in place, how would we return a new value that we can use to replace the original version? So what we're gonna do is in arrays.js, we're gonna implement these,
>> Anjana Vakil: Functions that we are used to using as mutating array methods in JavaScript like array.push, array index = value, array.pop.
[00:01:08]
And we're gonna create kind of functional equivalent, not all exactly the same, like pop doesn't return and it doesn't. Anyway, but we're going to create the same functionality without the mutation.
>> Anjana Vakil: And then, to do this we're just gonna use regular data structures, then we're just gonna take a look at how we could.
[00:01:31]
>> Anjana Vakil: Make sure we're still not doing that mutation on these operations, but still use our old APIs. So first step, we're gonna take in this arrays.js, these little stubbed functions, and we will implement them such that these tests pass. So for example, if my original array was 1, 2, 3, and I call push4 on the original, pushed should be 1, 2, 3, 4, but original should still be 1, 2, 3.
[00:02:05]
Unlike if I were to call original.push4, in which case, original now has a 4 in it, sucks to be you if you were expecting it to only have three elements. So, if we can get these tests to pass with our non-mutating versions of these. We will have satisfied a little tiny part of our functional programming hearts that really feels uncomfortable when we're mutating data.
[00:02:39]
>> Anjana Vakil: Let's start with push, shall we? So again, we wanna take an array with a bunch of stuff and a new element and return an array with all the original stuff, but also the new element. Anyone care to walk me through what they would do for this?
>> Speaker 2: You can just concat them together.
[00:03:00]
>> Anjana Vakil: I could concat,
>> Speaker 2: The array and the element.
>> Anjana Vakil: I'm not sure if I have access to this right now, but yes, I could concat them together, the array, and then,
>> Speaker 2: The element.
>> Anjana Vakil: The element.
>> Speaker 2: But if we using our other function, the element has to be an array too.
[00:03:23]
>> Anjana Vakil: Concat operates on arrays, right, and this would be the same, I could also use the built-in array.concat, but I don't wanna concatenate it with an element. That's not gonna work, I wanna concatenate it with an array that has that element in it. This is just the built-in array.concat which, again, our little helper was a wrapper around to just make it feel less object-oriented of an API.
[00:03:47]
But that is totally one way that we could do it, using concat, is there a way that we could do it without concat?
>> Speaker 3: Return a new array, first spreading the array, and then comma the element.
>> Anjana Vakil: So we also have our spread operator, which we said kind of spreads out all the elements of an array into the context where it's used.
[00:04:08]
And so here, dot, dot, dot, array is sort of all of those things, plus element.
>> Anjana Vakil: Yeah, so this is gonna be each element of the original array and my new element spread out into a new array.How about pop? So in the JavaScript method, it's gonna return the last element here, but here, what we wanna do is if I call pop on an array, I don't care about the last element that's just gone.
[00:04:43]
I want an array that has all the same stuff from the original, except the last element. So kind of the inverse, yeah, of the push, pop, push, okay. How about this one?
>> Speaker 2: Slice it.
>> Anjana Vakil: We could slice it, we saw before we had that array.slice, right?
>> Anjana Vakil: And then, we can look up the documentation of array slice, it's like zero.
[00:05:11]
And so, we're gonna start at zero and we're gonna slice the whole rest of the array, but then, the second argument, if we give it a negative one. It will stop before it gets all the way through the array, that's just the documentation here. But we could also imagine a way to do this with our little helpers from before theoretically, I'll leave that as an exercise for the reader.
[00:05:40]
Instead of using slice, for example, use head and tail to figure this out? I don't know, let's find out, but this is a totally legit implementation because if we hop over to our friend mdnrslice, we will be able to look up whether or not this actually works. Actually mutates the array that I'm calling it on to see if I can rely on it or not.
[00:06:08]
And so we have a sort of gotcha here where it does give me a new array. I just have to be a little careful if I'm dealing with deeply nested, complex data with these simple values like numbers and things, we are probably okay, but array slice is a shallow copy.
[00:06:30]
It's not like a deep copy, which would make sure that, if like, let's say the array includes an array, are we just pointing to that same array, or are we making a copy of that inner array? Anyway, so when in doubt, check MDN, see if the operation you're trying to do is a mutating operation or not.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops