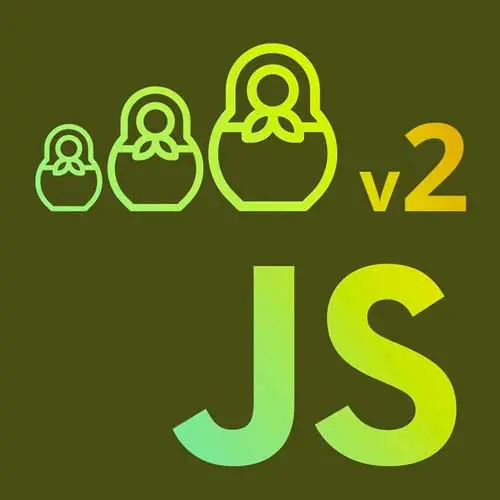
Lesson Description
The "Higher-Order Functions" Lesson is part of the full, Functional JavaScript First Steps, v2 course featured in this preview video. Here's what you'd learn in this lesson:
Anjana introduces higher-order functions, which take one or more functions as arguments. Examples of higher-order functions are the array methods filter, map, and reduce. These methods accept a function as an argument, and that function performs an operation on each value in an array. For example, the predicate function passed to the filter will return true or false for whether an item in the array should be included in the filtered results.
Transcript from the "Higher-Order Functions" Lesson
[00:00:00]
>> Anjana Vakil: The next tool that we're gonna start adding to our toolbox is higher-order functions. And the concept of higher-order functions goes hand in hand with the concept of first-class functions, and JavaScript has both. And so what we're gonna be doing is using some higher-order functions like, filter map and reduce to make some delicious sandwiches.
[00:00:23]
We'll talk about this in a moment. And understand how their illustrations of what a higher-order function is, and what first class functions are, and why they're useful for functional programming.
>> Anjana Vakil: Okay, so first-class and higher-order functions. We say a language has first-class functions if it supports us taking a function that's been defined, and sending it around like a regular value.
[00:00:57]
The same as a string or a number or what have you. So if I can pass a function as an input argument to another function, for example, that means that the language I'm working in has first-class functions. Cuz I can use a function just like any other value, and guess what?
[00:01:15]
JavaScript has first-class functions that you all have already taken advantage of I think, for example, by writing a callback function. So when we pass a callback function to, let's say, set up an event listener on an element in the DOM. We pass in a function that every time somebody clicks on that button or what have you, JavaScript will run that function for us.
[00:01:42]
So it's taking in the function as a value, it's not calling the function yet, it's not handling a click yet when I attach a listener. It's just going to remember that function, put it in its pocket, save it for later, and then do whatever it needs to do with it.
[00:01:59]
Just like a regular value, like a string or a number. So you all have already taken advantage of the fact that JavaScript is a language with first-class functions. And when we have a function that operates on functions that either takes them as input or returns them as output, we call that a higher-order function.
[00:02:20]
It's a function that's like one level above the function it takes in, or returns or multiple functions or what have you. So higher-order functions are something that we spend a lot of time working with in functional programming, because everything is a function in pure functional programming. And so we're kinda passing around our functions here and there.
[00:02:40]
Just like the way that we passed in a logical operator to our truth table function earlier, that sort of thing. Or the same way that you could define a function like handle click and pass it into a bunch of different calls to add event listener on click. Okay, so now in functional programming land, for example, when we wanna manipulate an array of values, do something with it instead of using iteration, which we said is taboo in the functional programming world.
[00:03:15]
Because it has state, and state is dangerous, unpredictable, gives me headaches, etc. We are going to exercise our newly found skills with recursion to use functions like map, reduce and filter. Which you might be familiar with because they're also built in methods on JavaScript arrays. But instead of using those built in methods, we're gonna re-implement them functionally.
[00:03:45]
Okay, so map, reduce and filter, have folks worked with JavaScript array.map.filter, yeah. Okay, great, so my favorite way of thinking about filter, map, and reduce and what they do. And illustrating this is a graphic that someone else, I can't take credit for this, I just adapted it, made called the MapReduce sandwich and I just added the filter.
[00:04:07]
So filter, map, reduce is these three functions all take in, they're all higher order functions, they all receive a function as input. And they all apply that function to elements in an array, but they do different things. So filter takes in a function that we call a predicate function.
[00:04:31]
Which means, the predicate function is going to receive a single element from the array, and it's going to return a Boolean value, either true or false. So for example, let's say I have an array of possible sandwich ingredients here, and I give a filter a predicate function like Anjana wants in her sandwich, this thing, right?
[00:05:01]
Or Anjana thinks this is tasty, or Anjana has this at home and doesn't need to go to the grocery store, that sort of thing, right? So for each element in the array, filter is then gonna call that function on the element and find out true or false. And then for the elements that return true, they pass the filter and for the elements that return false, they are filtered out.
[00:05:25]
So, for example, I can give it this array of ingredients and say Anjana likes X and it will filter out, let's say cucumbers and onions, even though that's not factual. I do both of those things, but whatever, for the sake of example. And it's going to give us a shorter array, essentially, now it might be the same length, but it's certainly not gonna have anything new in it, at most, can have everything in the original array.
[00:05:53]
Then, if we take that array of carefully filtered ingredients, and pass it to the map function, we are also going to get a new array out, but this time, we're going to transform each of the elements in the array. So map is gonna take in a function, but unlike filter, it's not gonna be a predicate function that takes a value and always returns true or false.
[00:06:19]
Instead, map is gonna take something more like a transform function that takes in a value and returns a different value. It might be the same as the original, it might not, depends on the function. So, for example, I could pass in the value of chop up to map, as a function that takes a thing and chops it up and returns a bunch of slices.
[00:06:39]
And map is gonna call that on every element in my array, and it's gonna return an array of all the chopped things. So bread becomes chopped bread, tomato becomes chopped tomato, etc. Okay, so far, hopefully this is a sort of review, cuz these are often a regular part of our day to day JavaScript lives, calling array.filter, array.map, etc.
[00:07:03]
Reduce is also in there, have folks work with reduce also?
>> Speaker 2: Yeah.
>> Anjana Vakil: A little bit, yeah. Okay, some folks are big fans, others I'm seeing a little more hesitation, but it's a little bit different because instead of filter and map, they take an array and return an array.
[00:07:23]
And reduce takes an array, but it returns a single value, essentially, it reduces an array of a bunch of stuff, reduces it to one thing. And so the type of function that reduce takes in is called a reducer, cleverly. And this is kind of a special type of function that basically is gonna get two things, sort of the reduced value I already know.
[00:07:55]
And the new element I wanna add to that reduction, and it's going to apply the reducer to return a single thing. So for example, let's say I have a reducer function called stack or layer, layer these things. And what that's gonna do is, if I give it bread and peppers, it's gonna layer the peppers into the bread.
[00:08:17]
And so what this is gonna do is, it's gonna keep applying to each of these elements successively in the array, and it's gonna keep layering them one on top of the other on top of the other until we've finished. And we have one delicious multi-layer sandwich to chow down on, after all of our hard brain work.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops