Software Engineer & Educator
Course Description
A friendly, practical introduction to functional programming fundamentals in JavaScript. Learn the power of the pure function while exploring functional programming paradigms. Compare iterative and recursive code and create more complex programs with higher-order functions and functional composition. Free yourself from mutations and write more reliable and predictable code with functional programming!
This course and others like it are available as part of our Frontend Masters video subscription.
Preview
CloseWhat They're Saying
Thank you very much for your excellent delivery and your joy in presenting the course so clearly and methodically! Bravo.
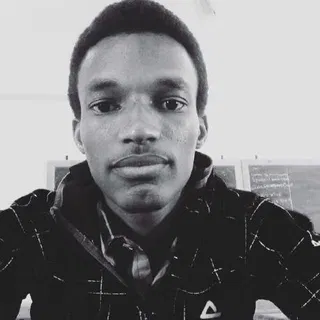
Béchir Soualhi
Anjana's explanations were so clear and fun!
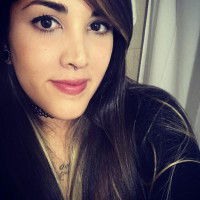
Flor Gomez
Course Details
Published: February 14, 2025
Rating
Learning Paths
Topics
Learn Straight from the Experts Who Shape the Modern Web
Your Path to Senior Developer and Beyond
- 200+ In-depth courses
- 18 Learning Paths
- Industry Leading Experts
- Live Interactive Workshops
Table of Contents
Introduction
Section Duration: 22 minutes
- Anjana Vakil begins the course with an outline of the functional programming topics that will be discussed. The notes and exercises for the course are available in the browser. A repo is provided to allow the exercises to be completed locally.
- Anjana shares some of the terms associated with functional programming, like higher-order, monoid, and functor. These terms can be confusing to developers new to functional programming. Along with functional programming, other programming styles exist, like imperative, declarative, and object-oriented. This course aims to outline the differences between these styles and dive into the functional programming concepts.
Programming with Pure Functions
Section Duration: 30 minutes
- Anjana introduces pure functions. A function is considered pure if the return values are identical for identical arguments and the function has no side effects like mutation of local static variables, non-local variables, or references to outside dependencies.
- Students are instructed to determine if a function is pure or impure. The solve button in the Stackblitz exercise can be clicked to show the correct answers.
- Students are instructed to refactor the code so the pureGetSVG function returns an SVG while remaining a pure function. Any impure operations are moved to the impureRenderApp function, allowing the tests to pass. In the Stackblitz example, the tests will rerun as the code is edited.
- Anjana discusses the solution to the Managing Impurity exercise.
Recursion
Section Duration: 43 minutes
- Anjana explains the difference between iterative and recursive code and provides an example using a function that sums the values in an array. The iterative approach uses a for loop, while in the recursive approach, the sum function calls itself until a base case is reached.
- Anjana shares two factorial function implementations. The interative implementation uses a while loop to complete the factorial operation. The recursive example can be implemented in only a couple of lines but can be difficult for developers to grasp conceptually.
- Students are instructed to implement both an iterative and recursive function that generates a Fibonacci number.
- Anjana spends a few minutes discussing the performance implications of recursion. Since recursive operations continue to add to the call stack, eventually a stack overflow error can occur. Features like proper tail calls can help eliminate "too much recursion".
- Students are instructed to create an SVG design by recursively drawing a square with alternating colors and rotation. The SVG group element can be used to nest the recursive calls and apply the rotation using CSS transforms.
Higher-Order Functions
Section Duration: 33 minutes
- Anjana introduces higher-order functions, which take one or more functions as arguments. Examples of higher-order functions are the array methods filter, map, and reduce. These methods accept a function as an argument, and that function performs an operation on each value in an array. For example, the predicate function passed to the filter will return true or false for whether an item in the array should be included in the filtered results.
- Students are instructed to implement a filter function that filters values out of an array. Utility methods for concatenating arrays and returning the length, head, and tail are provided. Recursion should be used rather than iteration.
- Students are instructed to implement the map function. This function transforms an array by passing each element to a function passed as an argument. This recursive operation continually calls map while concatenating the head of the array with each subsequent recursive call.
- Anjana explains the functional implementation of the reduce function. Students are then instructed to implement reducer functions for sum and max operations.
Scope & Closure
Section Duration: 16 minutes
- Anjana introduces closure, which is the combination of a function bundled together with references to its surrounding scope. Closures are created every time a function is created, and the function "remembers" its outer scope. This lesson also explains additional terms like partially applied functions and currying.
- Students are instructed to create higher-order functions that close over the diamondPattern function. These higher-order functions will "lock in" values with closure to create more specific SVG-generating functions.
Function Composition
Section Duration: 21 minutes
- Anjana demonstrates functional composition, which leverages closure to combine functions into pipeline-like systems. The result of one function call will be passed as an argument to a subsequent function. Function composition is the basis for all functional programs.
- Students are instructed to create a pipe function that takes an array of functions as input and calls the functions in order.
Immutability
Section Duration: 30 minutes
- Anjana introduces immutability and explains why avoiding mutation is a core principle of functional programming. With immutable data, when something needs to be updated, a new copy is returned rather than changing the original object. This eliminates the need for state in the function.
- Students are instructed to implement an immutable version of the push and pop array methods.
- Anjana continues walking through the solution to the Immutable Array Operations exercise by implementing the update method.
- Anjana introduces Immer.js, a library that works with immutable data in JavaScript. Immer provides APIs for working with Arrays and Objects using familiar JavaScript methods, but has reimplemented them to be immutable.
Wrapping Up
Section Duration: 7 minutes
- Anjana wraps up the course by asking students to share thoughts about functional-style programming. Additional resources are provided and students are encouraged to explore other functional languages.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops