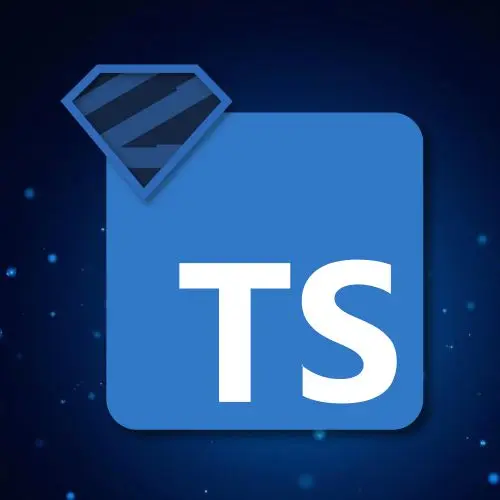
Lesson Description
The "OpenAPI Express Validator & Orval" Lesson is part of the full, Fullstack TypeScript, v2 (feat. Zod) course featured in this preview video. Here's what you'd learn in this lesson:
Steve introduces the openapi-express-validator package that automatically validates API requests and responses. The validator is installed as an Express middleware, allowing it to intercept any requests and responses requiring validation.
Transcript from the "OpenAPI Express Validator & Orval" Lesson
[00:00:00]
>> Steve Kinney: So all of a sudden, as long as you have that contract, and if you do the work for that contract and you can figure out a way to generate it. You can have at least parts of these entire contracts handled for you, right? There are some ways to do it on the server as well.
[00:00:19]
So one really kind of easy way is there is this tool called, again, the names are everything you need. There's one called Open API express validator, right? It's like an SEO game at some point, right? You just name it the most obvious thing in the world, and well, you can install it as middleware in your express application.
[00:00:43]
I would just probably do it in development. I guess you could do it, if you have good error handling in production. And then should you break your own contract? You can get alerted to it, right? And using it is incredibly easy as well. So on the front end, generate a client automatically.
[00:01:03]
You know it's type safe based on the contract, right? You don't have to do all this odd stuff. Again, you need to have the contract, a lot of things you might not be starting there. So we've got the tools on the low level, we've got the tools on the high level.
[00:01:16]
Jumping back over to the server. So we can grab this OpenAPIValidator,
>> Steve Kinney: And all that we really that seems like too much to import.
>> Steve Kinney: Cool all we can do, all we have to do here is install it as middleware.
>> Steve Kinney: And we point it to what it should do.
[00:01:49]
Validate the requests and responses. Here we would actually want the dot Json. And now you can set things to like validate whether it should throw errors, where should log issues, right? If your express API is violating the contract, your server will know and you can fix the issue, right?
[00:02:08]
Right, or, you can theoretically take those same types that you generated and use it with the middleware. We'll talk about how to create the Zod schemas based out of that. You have lots of options, right? Which one you choose to use will work for you. This one will at least let you know that bad things are happening, right?
[00:02:26]
You can also, again, say, play the same game that we played with our middleware and the Zod schemas, right? You can create all the types, and then you will get type notifications if you are not doing the right thing. How you go about this depends, but both sides can use the same contract and have the same safety guarantees and know everything.
[00:02:47]
It just becomes an infrastructure game at this point. And just to show you how easy it is to generate these Zod schemas, let's take a look at that as well. This is a really great library and I'm gonna go to the page and visit it with you because it's totally worth it.
[00:03:05]
So this is a library, it does not have a name that explains exactly what it does. But it will actually, let's try this. Yeah, the sidebar once again tells you everything you need to know. We're gonna use it to generate the Zod types because, as you can see, it can generate a react query client for you, a used SWR client.
[00:03:28]
It takes the OpenAPI schema and can spit out lots of different things. You use Axios, great, you can get an Axios client, right? You got the fetch one, which we saw in that other library. You all know what mock service worker is? Yeah, so mock service worker is effectively service workers can hijack the network, request coming in and out.
[00:03:50]
And it's a way that, let's say, you've agreed on the API contract, but the backend team is not done yet, right? It will stub out all of the stuff according to the API contract, you can generate fake API responses, do all the stuff, right? And then when the API is ready, you remove that layer.
[00:04:10]
Or again, maybe you're just doing tests, right? You have all that in place as well. Angular, all of a sudden, it has kinda built in support for pretty much everything. We're going to look at the ability to generate the Zod types and OpenAPI schema. But as you can see, depending on what your flavor is, there are many things you can do.
[00:04:38]
Does take a little bit of configuration, but as you can see, not too much. Tell me, this is actually already in the code base, so that's why we're looking in here. You can look in my editor too. Tell me where the input file is, again, and you could clone this down.
[00:04:50]
I think even for that Typescript one that we did earlier, you can actually point it at a URL, right? So as long as it's hosted somewhere and there are a bunch of different services for just like CDNs for Git repos. You can just pull it down from whatever main is all the time.
[00:05:06]
I literally have it as like a post install for npm, right? Anytime you run npm install or something, we pull down the latest specs so I can see if something broke. But we have a backwards compatible API, so I know that nothing broke most of the time. So here we can just say, OK, I wanna output Zod.
[00:05:22]
You can either do split or one big file. Which one you choose is probably dependent on how big your code base is. Obviously, if it's big, split it, so you can do tree shaking and code splitting. If it's small, for our cases, we'll keep it single. So then, what you're able to do, I'm gonna grab this just so I can remember, is we can go in here, back into my client.
[00:05:52]
And you can say, I've got the same config right here. And then we can run that,
>> Steve Kinney: And you can see it did create a new file called schemas.ts, where import Zod, and based on that OpenAPI contract, generated all of my Zod schemas for me, right? Again, great, if you have an OpenAPI spec, obviously, a little bit harder to navigate if you don't, but like super useful.
[00:06:26]
And again, you can start in either direction, right? Sometimes you might have it. You can also, again, create an open API schema through using all the Zod Schemas that you made. But obviously, there's a little bit more ceremony and work that's gonna take someone on your team a few days, not weeks or months, but more time than anyone wants to spend right now doing it.
[00:06:45]
But yeah, you can begin to pull those things together as well, and there's validators or so on and so forth. But yeah, you can create the schemas, you can create clients. You can do all those things once you have this and then you're no longer doing that trust game, right?
[00:06:58]
You know that there is a contract. And yes, I am on call for a reason, because somebody will make a boo-boo, you'll find out ways in which your CICD process didn't catch something. The goal is to get, yeah, I would love to tell you we can get to 100%, right?
[00:07:13]
We can probably get to 99 point, some egregious number of 9s, and that is probably good enough for us in a lot of cases. But there will always be ways to slip through and mistakes are gonna be made. But again, the more tooling that you use, the less likely that's going to happen, right?
[00:07:30]
It is, we saw even when we're manually putting the Zod types in there ourselves, or Zod schemas, if we missed one, it was still any or unknown, right? Here we are, then at least. Again, we have a contract, we can run it on both the server and we can run it on the client as well.
[00:07:47]
Things will break, we were talking about this during one of the breaks, there are still the problems become more social than they become technical, right? Which is, okay, has every team invested enough to make sure that there's one end-to-end suite before anyone deploys. Well, then you've got to have maybe a test environment that is stable at all times, the ability to spin up fresh users, to get to a lot of cases.
[00:08:14]
Maybe you can't do all those things for just because, the amount of teams that would need to figure it all out together. It's just you can figure that stuff on a 30 person company, we did. I also worked at a 7,000 person company. Turns out, those things are harder.
[00:08:30]
Getting 7,000 teams or not 7,000, but 100 teams to sign on to things is a lot harder than getting 3 teams to sign on to things. But depending on where you are, you can always use those lower level ones to at least ensure your walls. Or if you can get everyone on the same page with an API spec, you can begin to generate stuff.
[00:08:48]
Which one of these approaches that you take really depends on the situation you're dealing with right? The smallest ones are probably the easiest to start with, though, and you can get some safety tomorrow. These bigger ones are obviously going to be the most stable and consistent. And also, who doesn't love getting all these schemas generated for them or entire clients, right?
[00:09:06]
With some of these, you can just generate if you use react react hooks or svelte like runes or what have you, to be able to do all these things, that's great. Do that because you're not getting paid more for doing it by hand, like there's no such thing as an artisanal Zod scheme being worth more than one that is generated.
[00:09:23]
The generated one is probably more accurate.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops