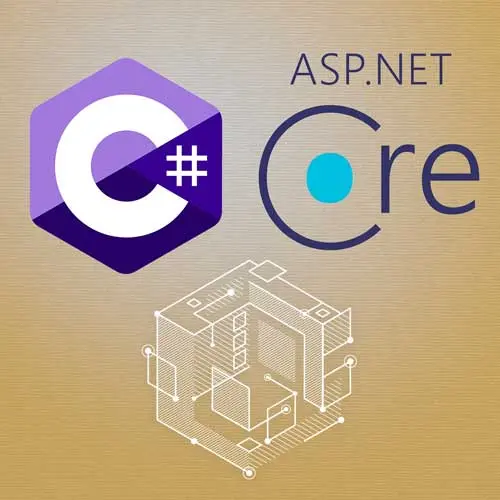
Lesson Description
The "Setup Course API Project" Lesson is part of the full, Building APIs with C# and ASP.NET Core course featured in this preview video. Here's what you'd learn in this lesson:
Spencer scaffolds the initial project files for the Employee API. The .NET CLI creates the directory and the base application files. The Employee class is created, and a route is added to the API to get a list of employees.
Transcript from the "Setup Course API Project" Lesson
[00:00:00]
>> Spencer Schneidenbach: So let me talk about what we're gonna be actually building here. So we're gonna go down and we're gonna talk about all the different components of ASP.NET Core, at least the ones that I use 99% of the time when I'm developing. We're gonna start by building a simple web API that allows us to manage a list of employees in memory.
[00:00:15]
And by the end, you'll be able to take that and we'll actually have a database, and we'll have a serious set of APIs and tests that you can use as a blueprint for creating other apps. If you're so inclined, you can go clone this repo and follow along.
[00:00:30]
Everything that you see is additive to the previous lesson. So let me give you an example. As we're going through two, writing our first tests, for example we'll be taking a base solution and we'll be adding to it as time goes on. And then at the end, the end product for that will be the next exercise.
[00:00:50]
So you can see a completed solution here by peeking ahead and looking at 2D for instance. So all of the tests will be in this one. So you'll be able to follow along, type along as I go, and you'll see exactly what I'm doing. The documentation that you have kind of takes you step by step, and I'll explain some of my thought process along the way.
[00:01:10]
The final solution here is the 4Z and it has kind of everything that I completed, plus a couple of extra things, which we'll touch on at the end. And then the link in this repo does link back to the documentation if you so choose. Let's start by building TheEmployeeAPI.
[00:01:30]
That is gonna be the API that we are going to build on, so I'm going to say dotnet new webapi, TheEmployeeAPI. All right, in the Employee API, you're gonna see pretty much exactly the same as we had before. It gives you that general blueprint, that weather forecast, but we're gonna start by deleting some of this stuff.
[00:01:54]
We don't need the weather forecast. We do need the app.Run, so we'll keep that. We don't need this record type. We don't need the summaries. So this is what you'll be left with. So let me talk about minimal APIs. So the weather forecast example is what's known as a minimal API.
[00:02:14]
It's a relatively new feature in .NET that allows you to define API endpoints right here where you define your middleware. So I wanna talk about what the actual API looks like. So we saw MapGet, you'll see that if we go here, we'll see that there are actually two types of arguments to this function.
[00:02:34]
So what we have is we've got our pattern. So we'll say employees for now, and then you'll see that we can give it a delegate, AKA any old function, or we can give it a request delegate. So a request delegate is pretty much give me the raw context and just handle it as you see fit.
[00:02:54]
So it looks a little bit like this. So there's the argument goes into get that lambda and you can see that if you want to, you can just handle the request in a very raw sense. So you can read the items, you can read the headers directly. We won't be using this one.
[00:03:11]
We won't be writing raw, we're gonna be using ASP net core framework as intended, as a high level framework. So we're not gonna be using that very much. What we will be using, however, is the delegate one where we pass in pretty much any old function. Before we get into that, though, we do need to create our employee class.
[00:03:32]
So I'm gonna go ahead and go here, and I'm gonna go to employee.cs and this is gonna be the holder. So this is the employee API, and we wanna return some employees. So we're gonna say public class employee, and we're gonna say prop. So I put prop and then I hit tab, which is just basically a shortcut for give me a property.
[00:03:49]
I'm gonna keep it as an int, and I'm gonna give it an Id, and then I'm going to tab away. An integer Id is a pretty typical thing used in database systems to indicate that this is a unique record. So we're gonna be talking to a database directly, so we know that we're gonna need an employee Id.
[00:04:05]
And then I'm gonna give it a FirstName, tab out, and then prop string LastName. So you'll notice right away that the compiler is complaining about something. You'll see these squiggly lines, and it says non-nullable property for FirstName must contain a non null value when exiting constructor. That's because we have nullability on.
[00:04:25]
So what we can do is we could handle this by adding a question mark to say that this could be a null type. But in reality, we're trying to model how employees are in the real world, we want this to be a real model at least as much as we can.
[00:04:41]
And we know that employees, at the very least, have to have a name. So we're gonna go ahead and use the required keyword here for now, and that will make that go away. By the way, I do wanna talk a little bit about nullability, so we're gonna use nullability in this project.
[00:04:57]
Lots of real world projects that already exist can't really go back and add nullability. At least not without spending a huge amount of time and money, and we're paid to ship features, so usually those win out over making refactors like that. But if you're starting with a greenfield project, I highly recommend that you use nullability, which is to say that our reference types are marked as either nullable or not nullable.
[00:05:20]
In this case, these are required so our reference type, our string, we wanna mark that as required. And the compiler will help us along the way to say, the editor will give us squiggly lines and it will tell us that, hey, you can't do this. I'm gonna do one thing here real quick.
[00:05:39]
I'm gonna take this a step further. I think if you enable nullability, it's my belief that you should also treat warnings as errors. Because right now the way it sits, the squiggly line is just gonna complain at you. It's just a warning, but your solution will still run.
[00:05:57]
But we're gonna get some extra safety here because this is a new project. So I'm gonna say true, and I'm gonna say TreatWarningsAsErrors, close that up, close that. And now you see down here that our warning, what would previously run is now showing a nice red X to say, hey, you can't do this.
[00:06:16]
Now, it's a red squiggly, it's angry, angry squiggly. So we're gonna give it that required property, get it to stop complaining.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops