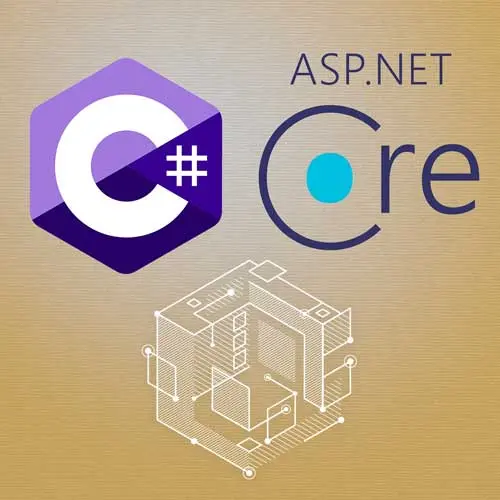
Check out a free preview of the full Building APIs with C# and ASP.NET Core course
The "HTTP Overview" Lesson is part of the full, Building APIs with C# and ASP.NET Core course featured in this preview video. Here's what you'd learn in this lesson:
Spencer spends a few minutes discussing the HTTP protocol and reviewing verbs like GET, POST, PUT, PATCH, and DELETE. The APIs built throughout the course will follow these common practices and send back the correct status codes along with the results of the requests.
Transcript from the "HTTP Overview" Lesson
[00:00:00]
>> Spencer Schneidenbach: I want to talk a little bit about HTTP in depth. I think it's important to understand kind of the low level players, so to speak, before we get to the frameworks like ASP.NET Core. It'll help us kind of understand what ASP.NET Core does under the hood. So HTTP is the protocol that pretty much the web uses.
[00:00:19]
It allows clients to communicate with servers and back. It's the protocol that powers the web. It's pretty standard. I think that if you open up a web browser and you go to a website using HTTP. I wanna take a brief look at a typical request and response. This is what it actually looks like when you're actually making a request.
[00:00:36]
Under the hood when the bytes are moving from the client to the server, this is what it looks like. If we take it line by line, we have GET/index. So GET is what's called the HTTP verb. And so we'll see a lot of those. We'll use a few of them during this course.
[00:00:54]
This will be the resource that you're actually wanting to request. In this case, it's index.html. And the version of HTTP that you're using to execute the request, most of them are HTTP/1.1. Still not a hugely amount of wide support for HTTP/2, but it's happening. Slowly, but surely. We have the host, so this is where we should actually go for www.example.com is the web host.
[00:01:18]
And then the resource, the fully qualified resource name would be example.com/index.html. And then these are what's referred to as request headers. And so these are data about the request that is being sent by the client to say hey, I want expect and I accept back a text HTML.
[00:01:36]
That gives the server the opportunity to say, well I can't serve that if you request something funky. And then you can use accept language as well to say, I want this in English, and I want it in the United States version of English. You can have as many headers as you want in your request.
[00:01:53]
It's really dependent on what the server needs. And usually these requests are a lot bigger in that there's usually lots more headers that are sent by your browser. But I think this is fine for now in terms of understanding kind of the pieces of HTTP that are relevant for this course.
[00:02:11]
So the response looks very similar. Requests and responses, they have pretty much the same similarities in that there's always a header that's defined. Yep, this is HTTP/1. This is the status code that's returned from the server to say, yes, your request is good. 200, okay, is everybody's favorite response.
[00:02:32]
Because that means, hey, everything worked, everything was good. So it says 200, okay? Gives the client the opportunity to parse that and say, request happened. And then the response headers. So, the server can respond with a huge amount of headers that define, here's the content type, here's the language, here's whether or not you should cache it.
[00:02:52]
And if you can cache it or should cache it, here's how long you can cache it, things like that. And then of course, the body of the response. You've requested data from the server, so the server has responded in kind with some type of information. Not always, sometimes the body is blank, but in this case, we expected an HTML page and sure enough, that's what we got back.
[00:03:16]
>> Spencer Schneidenbach: I want to touch on HTTP methods a little bit. They're also known as HTTP verbs. GETs are used to get resources. GETs have one interesting quality in that they are not supposed to be sent with a body in the request. You can, and some servers can respond and read that body, but recommended that you don't.
[00:03:40]
POST, PUT, and DELETE. So post and puts are usually to do some kind of mutation. In the API world, posts are usually akin to, I want to take some action or I want to create some object. We'll be using it more for the create an object. And then puts in the API world is another way to mutate a resource.
[00:03:59]
But it's often used to re-mutate, like change an existing resource like an employee, which we'll see plenty of examples of. And then the delete verb also does not get sent with the body, and it just does delete the specified resource. These are a few of additional ones that you'll see.
[00:04:18]
Patch, we won't be using this one in this course, but patch is sometimes used in place of puts to mutate a resource. And there's actually a whole patch spec that nobody seems to have adopted for actually changing just little bits of a resource, not widely adopted. A head request is just basically saying, hey, can you respond to this?
[00:04:42]
Give me the information from a get request, but don't return the body. I just wanna see if we're all good. And then options describing the communication option for the target resource, often used by cores, which we will not cover in this course, to determine whether or not a resource is accessible.
[00:04:58]
So most of the time for APIs, we're gonna be using GET, POST, PUT, and DELETE. Those are the common ones, and we'll see examples of that as we go through.
>> Spencer Schneidenbach: So HTTP status codes, the status codes define the result of the request as we mentioned. We've all seen 200, we've all seen 404 errors where we say this page or this resource is not found.
[00:05:20]
So I wanted to go through, they're generally grouped into the first digit of the response code. So we have informational, I don't see this used hardly ever. Success, probably the most common one is to say, yes, my request, was successful, and I accepted it, and I maybe responded with some relevant data.
[00:05:40]
300 level response codes indicate that there's some kind of redirection or some type of further action that needs to be handled in order to take the request. Usually, it's a redirect response code that's returned for a 300. We have 400 client errors. So this is going to be my favorite response code because I think it's the most important one, which is 400 bad requests.
[00:06:01]
And of course, there's 404 to say the resource doesn't exist. And then 500 errors are typically reserved for it. Hey, the server couldn't handle this request. Something catastrophic happened that couldn't connect to the database. I'm just gonna respond with 500, throw my hands in the air, I can't do anything.
[00:06:17]
These are the ones that we are going to use the most of. We're gonna say okay, 200 okay, 201 created to say a resource has been created. We'll also use 204 no content for seeing a resource has been deleted, which some people implement. Usually I just return a 200.
[00:06:37]
We'll see 400 bad requests. We'll see lots of those, absolutely. We'll touch on 401 and 403. I do intend to talk through some of the identity and authorization aspects of ASP network, but we're not going to get too in depth in it. So these are the kinds of requests that we're going to see a lot.
[00:06:59]
Let's take a look at a typical POST request. This is gonna be the kind of thing. We're building APIs, so we're marshaling JSON back and forth from the client to the server. So this is gonna be an example of what we're gonna send a lot. Which is to say we're gonna have a post request.
[00:07:13]
We're gonna hit the employees, create endpoint of some kind. Here's the host that we're gonna go to, and here's the content type of this request. That tells the server, hey, this is JSON, and you should interpret it as such. This is the data transfer format that is the ubiquitous format of the web for transferring data back and forth from an API.
[00:07:33]
We're gonna be using JSON, it's the most common one.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops