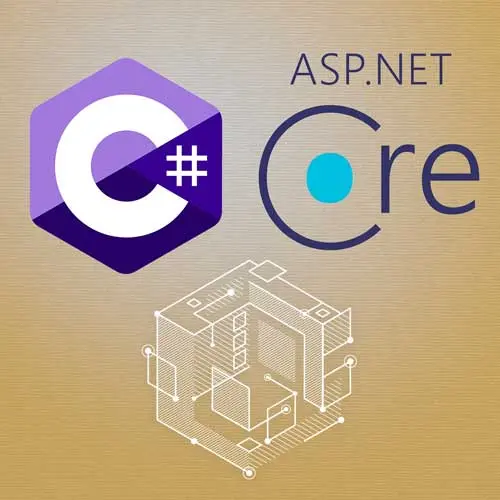
Check out a free preview of the full Building APIs with C# and ASP.NET Core course
The "Entity Framework Overview" Lesson is part of the full, Building APIs with C# and ASP.NET Core course featured in this preview video. Here's what you'd learn in this lesson:
Spencer introduces Entity Framework Core (EF Core), an object-relational mapping (ORM) framework for .NET. It allows developers to work with databases using .NET objects and provides a way to interact with databases in an object-oriented way rather than using raw SQL.
Transcript from the "Entity Framework Overview" Lesson
[00:00:00]
>> Spencer Schneidenbach: Let's talk about Entity Framework Core. And I'm all smiles because Entity Framework Core is one of my favorite parts of the .NET ecosystem. So what is Entity Framework Core? If you're familiar with ORMs in JavaScript, Prisma is an example of one. EF Core is that inside of the .NET ecosystem.
[00:00:21]
So it allows you to work with the database using .NET objects, and provide a way to interact with databases in an object-oriented way rather than using directly SQL. So developers and SQL famously do not always get along. So EF Core kind of exists to bridge that gap, but it does so much more.
[00:00:37]
It really is awesome. So why would you choose to use EF Core over something like even raw SQL, there's actually some other ORM products out there, but I don't think they're as good as EF Core. So first of all, you can do something called code Ffrst development, which allows you to define your database schema using .NET classes, and then generate the corresponding data table.
[00:00:58]
So that's called code first. You could also reverse-engineer. There is actually a way to do that, we're not gonna go through that in this course. It's called database first, and it is supported, and it is possible. So you can do it both ways if you like. So the thing that it does really well is it maps those .NET objects to database tables, making it easier to work with those databases, as I mentioned, in an object-oriented manner.
[00:01:20]
So Entity Framework Core does a lot of the plumbing under the hood. It'll write the SQL for you, and that goes for querying your database, as well as adding or removing records from your database. One of the cool things is that you're able to write link and have it talk to the database, as opposed to just writing raw SQL, as I mentioned.
[00:01:40]
So we'll go through that because that's really cool and one of the most powerful parts of using Entity Framework Core. It also includes change tracking. So it will automatically keep track of the entities inside of your project and whether or not they need to be updated or whether or not they need to be inserted into the database, or whether or not properties need to change.
[00:02:01]
So it will take care of all of that for you. And then finally, another one of its great features is that out of the box, it supports database migrations. So as your model changes, and your model and requirements will change, the EF Core will allow you to generate those migration files and make it so that you can keep your database up to date.
[00:02:22]
So how does EF Core work in code? So there's a couple of really important constructs. It's actually quite simple. DBContext is the first one. So dbContext is kind of the owner of the database tables, or it kind of represents an abstraction of the database that you're targeting. And in the dbContext, you have a series of dbSets.
[00:02:44]
DbSets would be the individual object inside of your database. So considering our application, for example, our employee object is the object that we use. So you would have a dbSet for employee. You would have a dbSet for an employee benefit. If you had a customer record, you'd have a dbSet for customers, so on and so forth.
[00:03:05]
Here's what a sample of that code looks like. So a dbContext class that you define inherits from dbContext, and then you define properties that set the dbSet for each of those employees. This is what it would look like. If you've instantiated a copy of your dbSet and you're querying your employees table, you can do link, just normal links.
[00:03:34]
So you can say where and provided a predicate to say that for the employees who department is sales. And I wanna bring those into list. And then roughly, it will return something that will roughly under the hood, go to the database and execute a query that looks very similar to this, where it does a select star from employees, where department equals sales.
[00:03:55]
So it does a lot of translation of SQL for you using LINQ, which is really cool. The way that you install EF Core is through the NuGet Package Manager. And you can do that by installing Microsoft.EntityFrameworkCore. And that's the package that includes pretty much all the core stuff that you need, which is the dbContext, dbSet.
[00:04:19]
But individual database providers are provided in separate NuGet packages. So for instance, if you're targeting SQL Server or Postgres, those are separate packages, and you would install those separately as you would need each of those in different databases. So we're gonna go with the SQLite database provider because it's super easy to get started with, and it's super easy to use.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops