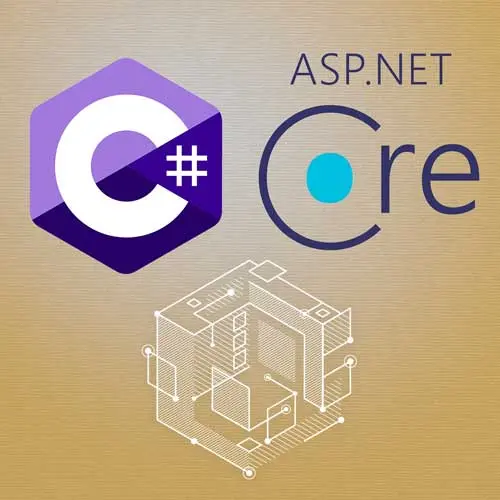
Lesson Description
The "ASP.NET Core Overview" Lesson is part of the full, Building APIs with C# and ASP.NET Core course featured in this preview video. Here's what you'd learn in this lesson:
Spencer introduces ASP.NET Core and explains that it's designed as a high-performance, modular web framework. ASP.NET Core allows developers to add middleware while putting together a robust pipeline for building web applications and APIs.
Transcript from the "ASP.NET Core Overview" Lesson
[00:00:00]
>> Spencer Schneidenbach: Now that we've talked about HTTP, let's get into how that bridges into ASP.NET Core. So ASP.NET Core is a framework built to respond to those HTTP requests, right? And it has a lot of tooling to kind of make your life as a developer easier, especially if you're programming against a database of some kind.
[00:00:18]
So as I mentioned, you're one command from creating a new ASP.NET web Core project, which we'll do here shortly. This is what the program file looks like. Let's actually do that real quick and talk about what we see. So I'm gonna actually run this, say ls, see where we are.
[00:00:36]
I will go to Repos, keep it all contained. And I will create My .NET new webapi, MyWebApp. Let's go ahead and go through the project structure real quick. So I'm gonna open this up in Visual Studio Code. I'm gonna go to Open Folder, Repos, and what is this one?
[00:00:54]
MyWebApp, perfect. Boom, there it is, okay? Wanna talk through a couple of things that we're seeing here. First of all, you get the typical folder view of Visual Studio Code. The C# dev kit extension also adds this little solution explorer, which is something that I use as well.
[00:01:16]
And that will tell you basically, here's the project structure, here's the solution, and here's all the projects in that solution. So you can see up here that when you create the application that you get a csproj file. And the csproj file is basically kind of the group of all of the files that are relevant to this web app.
[00:01:38]
It comes with a few installed like Swashbuckle, which we will talk about. That defines the target framework, which is .NET 8, which is the latest long-term support framework. It has nullable>enabled, which we'll get to here shortly, and it has ImplicitUsings, which we won't necessarily cover. These are the NuGet Packages, so NuGet being the package manager for .NET, these are the ones that are installed in the template.
[00:02:08]
Let's take a look at the Program.cs file. So there's a little bit of just general stuff that they think is useful. When you run this application, it'll actually open a browser window and it'll actually show you swagger, and will allow you to see and browse the APIs that you have defined.
[00:02:26]
Down here we see this MapGet right here, which is the give request that they give you by default. So they're telling you here's what a giver request would look like, it's a very basic API. And then this is the part where actually runs the API. Let's go ahead and run it real quick, kind of see what we get.
[00:02:49]
>> Spencer Schneidenbach: Okay, so you can see that a nice little UI comes up, nice little Swagger UI to tell you, here's all your API definitions. In this case, we only have one, which is the weatherforecast, and we can if we wanted to, execute this here. We could also execute it in Postman, which is what I'm gonna do.
[00:03:07]
So I'm gonna copy this host. I'm gonna switch over to Postman, continue without an account. Nice try Postman, not doing it. Weatherforecast. And you can see down here that our API has responded with a little bit of data.
>> Spencer Schneidenbach: It's all JSON. It's all JSON data just of random weather.
[00:03:28]
And if you keep sending it, it just basically sends you back random data. So I do wanna show a little bit of something else. There's kind of two major sections to the program file. There's the builder part where the app is being actually kind of configured and built.
[00:03:48]
So this is where you register all your services and your dependency injection container. These are all the things that are available for the application to use. And we'll get into that a little more detail as we go forward. And then from there, after your app is actually built, you'll see that you have these calls to Use.
[00:04:06]
What is Use? So Use is basically telling something inside of ASP.NET to say there's middleware, right? And so those middleware are basically just building blocks. So, think of it like a pipeline, water flows through a pipe. Our HTTP request is actually flowing through a pipe as well. And you can think of middleware as sections of that pipe.
[00:04:27]
So for instance, it says if this environment is development, which we're running it locally, so it's properly saying that this is indeed a development environment. So it says, hey, I want you to add Swagger and I want you to add the Swagger UI to the middleware pipeline so that way it can be accessible.
[00:04:46]
Order really matters in middleware, which we will demonstrate here shortly. This is a middleware that says redirect to HTTPS if you should so choose. And then this is where we actually add our get endpoint to our middleware call or middleware pipeline to say, okay, I want you to be able to handle requests that look like this.
[00:05:05]
In this case, we wanna be able to handle get requests where the resource that they're requesting is weatherforecast. And then this is actually where we actually go in and run the app. To kind of introduce a problem and to show you that middleware is always in control, we're gonna go here, and we are gonna scroll down to our,
>> Spencer Schneidenbach: Code there, and we're gonna paste this in.
[00:05:29]
So middleware has a property where it doesn't have to execute the next middleware. If it thinks that it can handle a request, it's gonna handle that request and simply return. So we're actually gonna put at the top of our middleware pipeline, we're actually gonna write something to the client, which we'll do here quickly.
[00:05:48]
And you'll see that now everything else is skipped. And what's that doing? What does that mean? Well, first of all, we're actually adding in some headers. We're appending some headers to our request. And then we are writing this Response, Welcome to Ahoy, and we're not going to the next phase of the middleware pipeline.
[00:06:07]
And the reason that we're not doing that is we've now been instructed our middleware to execute. So look at this thing right here. So this app.Use has a specific signature. It has an HTTP context, so this is where you could get your request, your response. You can write directly to the response, you can read request headers, you can understand the user context for the person who's actually asking for the request.
[00:06:30]
And then you see this thing called a RequestDelegate. So this request delegate is actually a pointer to the next phase in the middleware pipe. So if we shortcut all of this to say, await next. See, pass it the context. Cool thing about middleware, you don't even have to pass it the HttpContext.
[00:06:52]
You can fool the next middleware. Give it a completely different HttpContext if you want, not something I do, but it is possible. Now we've instructed this middleware instead of actually handling this request, actually just go to the next phase in the pipeline. And now we get what we expect.
[00:07:10]
This is telling this next call is now telling let's go down to this next middleware, in this case, we've defined it as UseSwagger. A little bit about HttpContext. HttpContext has quite a few helpful properties that are really important to understand. Request is, of course, the incoming request. It'll tell you, like I said, the request headers, the content of the request, you can read them.
[00:07:37]
A lot of times we're kind of handling it in a raw sense, but once we get further down into ASP.NET Core, we'll see how it can automatically handle some of parts of this request and make it very convenient for us. We have the response object here where we can write to the response if we so choose.
[00:07:54]
We have an items dictionary where we can say middleware might interpret a part of the request and say, hey, I think that other middleware down the pipe might need this item to continue executing. So you can add items and remove items as necessary, which we'll show a brief example of later.
[00:08:12]
We can see the user that's coming in. So if we go down here, you can see context.User.Identity to actually get the username of the person that's actually making the request. And then there's features of request which you can think of them as tiny little middleware, but they're really more like, I wanna handle a request in a particular way, or I wanna have this particular behavior that executes as part of responding to the request.
[00:08:47]
So now that we've talked about middleware, and kind of talked about the importance of middleware in the ASP.NET Core execution pipeline, continue down and see that as long as we have given the okay back to the middleware to actually execute the next thing that it will actually do so.
[00:09:11]
And it'll continue down this pipe until it finds something that it can execute. So, if we go here and put a breakpoint, I'm gonna go ahead and do that.
>> Spencer Schneidenbach: And you'll see that this middleware only gets hit, I'm refreshing, this middleware will only get hit if it's a request that it can think it can handle.
[00:09:28]
So if we have weatherforecasts, we're not gonna actually get anything. We're actually gonna get a 404. The server's gonna say no middleware was actually able to handle this request. But if we go to weatherforecasts, you'll see that once we hit it, we'll actually hit that break point, and actually you'll see that this says, yes, I can handle this request.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops