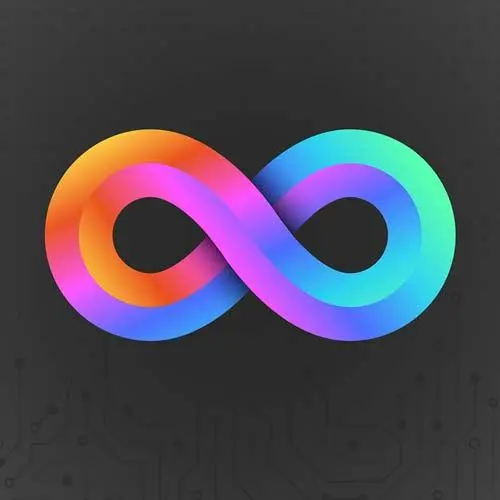
Lesson Description
The "Build Process Automation" Lesson is part of the full, Introducing DevOps for Developers course featured in this preview video. Here's what you'd learn in this lesson:
Erik live codes a CLI tool that can read a build.json file, define service names, and service types to help automate the build process.
Transcript from the "Build Process Automation" Lesson
[00:00:00]
>> And so we're basically gonna build this, we're gonna build this very, very quickly to show you guys how you never have to write a Docker file again, well, directly. You never have to write a circle CI configuration again. And if you've written programs enough, you could probably kind of see where we're going with this and how we're gonna build it.
[00:00:18]
But it's really that simple, right? So we have service directory, now we have our service templates. Now, again, we're gonna build this pretty quickly, so I will again push up the code for you guys so you have it. But what I wanna do really is, I just want to create a very simple, TypeScript project, and created index.ts with that.
[00:01:03]
Got it. One thing we need to add is what we added before, TypeScript. So now if I go here, there we go. We're good. Okay, so let's go back to our diagram. And let's make sure we check our document first actually. So we wanna make a Docker file and we wanna make a circle CI file autogeneratable.
[00:01:34]
We know that we need a DSL and a CLI tool to do that. So, why don't we do the easier one first, which is declaring our DSL, our domain-specific language. So just like we did before, I'm just gonna start with type safety. I'm just gonna start writing types.
[00:01:52]
And so in this case, we'll say build JSON, maybe, something like that. And then in this, we'll start typing our domain-specific language. So, say name, string. We'd say service type string. Now remember, this is type. So if I wanted to, I could even give service type an enum, a specific enum that is only usable within these specific things or whatever.
[00:02:19]
So that's just something else to remember is I'm just doing the simple, you can go way beyond this. So now we have this. Let's also do this really quickly. Let's create a build by JSON file so we can actually test it as well. Again, in my index.js, I have this, and in my Build.JSON, we're going to duplicate it.
[00:02:45]
So we're gonna say name and why don't we do this? Why don't we follow the same naming convention that we did before so we could say devops, this is the devops course front end. That's the name of it. And then if we wanted to, we could actually just say DevOps course there.
[00:03:09]
And because it's gonna have the service type here, front end like that. Those two variables [LAUGH] alone can tell you a lot about how a service is built and how we maintain it. Just by having a name and a service type, we can now tell it what kind of service it's built or what kind of service is built.
[00:03:41]
We can say for front ends we have a completely different Docker file than a backend per se. We have a lot of flexibility by just having those two, but I'm gonna show you just super quickly, we're not gonna do it right now. Actually I'll show it to you in a second.
[00:03:56]
Let's move forward. So as I said, these two things are very, very powerful. And again, even just having this helps us in DevOps know how to get to where we need to. So now that we have that, we're gonna have to do a little bit of coding. And we need to create a few functions to get our CLI tool to work properly.
[00:04:18]
First off, we need to make it so our program is actually a CLI tool. And so I'm just gonna use a very common tool called Yargs, to do that. If you guys have ever heard of Yargs before, it just makes it so that node CLI tool or node can be used as a CLI tool.
[00:04:37]
It's very, very simple. And the main point here is that we wanna create a command that we can use this as and generate our code with. And so I'm literally just going to copy all of that from here, and we're gonna do function main, just like I had before.
[00:04:59]
And we're gonna post all that code into it. Now, again, I'm just copying directly from their example, all right? So I'm not doing anything crazy and if you're interested, Here it is right here. All right, so here's what we're gonna do. We're gonna say yarn add yards. Now we have yards.
[00:05:33]
So we'll say import yards from yards. And then we will just get rid of this. So we have yards like this. Now it's complaining because it's saying that we don't have types. We could move forward if we wanted to and just not use types, but we're using TypeScripts, so we should probably add our types.
[00:06:00]
So I'm just gonna do yarn, add dev at types yards, In this area, that should work, cool. So I'm not gonna do anything else. I'm just gonna save this and then I'm gonna do node index.js. Cannot use import statement outside of a module. Jeez, did I do something wrong?
[00:06:32]
Let's do this really quickly. The autogenerated TypeScript file is not always exactly what you would want it to be so I'm just gonna grab one that I know is not gonna complain at me. I just gotta put that in its place. Oops, there we go. So just to go over this really quickly, this is a TSC config file.
[00:07:02]
Basically, it's a TypeScript configuration file. Because we're using TypeScript, we are using what's called a superset language. Or basically a superset to a language. And so we have to compile the code or transpile the code from TypeScript to JavaScript basically. And so this configuration helps us get there by telling us what the module resolution is, if it should pretty stuff, or in what files it's looking at.
[00:07:28]
But this just helps us set up our TypeScript stuff so that it actually just works out of the box. I do that, cannot use, why are you saying? My bad. There we go. So, property script name does not exist. So it's saying that, I guess maybe they're examples out of date.
[00:07:53]
There we go, okay, cool. So we're just gonna grab this. And we'll get rid of all this. And we'll just replace it with this little one liner. Sorry, I'm on a Mac. There we go. So then we'll go here with this like this and we'll just put this right here.
[00:08:36]
Import, you don't have to do this but I do it just because I'm weird. Process, so then, Add dev types node. Gotta add that really quickly. And then we can actually get rid of that now. There we go. So if I do console.log argv, and then we run this, see if this works.
[00:09:18]
[LAUGH] Yeah, and one more thing, ts node. So do mpx ts node. There we go. That's what we're looking for, cool. All right, so go back here. That's why it's not working. Got to tell it to execute the main. There we go. So now we can see that I have a command line of some sort and I'm getting stuff back.
[00:09:47]
So now if I was to do like, name foo, you'll see that I have name here. So now what I need to do is just start getting the args I need off of it. So iin this case if I do argv, and then just grab name, Argv. Name, Like this.
[00:10:24]
Then we run this. Look at what I get, foo. Now again, this is a very simple CLI tool [LAUGH] I'm not trying to show you guys the best way to make a CLI tool. What I am trying to show you is how to just get started with one.
[00:10:41]
And so if we do name foo we could do service type front end. And so now we can even do, Service name foo and stuff like that. Now, is this where we wanna put these parameters though? This is supposed to go in our build.json. So what is the parameter we need to give to our actual CLI tool so it can get those values?
[00:11:12]
What do you think we need to pass to our CLI tool so that it can read the build.json? We need to give it to the path to the build.json. So what we need to do is we actually need to say config. And then do something like this. Now this is gonna error because it's gonna say undefined but now if I do config, and then I rerun this, now I can find my build.json.
[00:11:45]
So now if we talk about needing a CLI tool that can read a build.json, the only thing we really need left is to be able to actually read the file. And we'll say const build equals argv config. I guess it's just readSync now. Nope, readFileSync, there she is.
[00:12:16]
So then we'll do readFileSync, Build path. And we should get back a buffer. So we'll say const configuration equals this. And then if I do console.log configuration, I believe we'll just get a buffer. We won't actually get what we need, but that's okay. As string, Implicitly, any, Shut up, Build.
[00:13:07]
I need to figure this out, just give me a second. Not entirely sure why it's giving me this. So let's do this. Let's read the docs really quickly. I could just do this too, so ignore statement. Sorry guys, just give me one second for a block. No check.
[00:13:56]
Got it. The reason why this is complaining is is because I guess Yargs isn't really comfortable with TypeScript. So we're just going to no check this part because we know it's there. But we don't want it to freak out about it. Yes, no check comment is top of the line file, disable type checking for that file.
[00:14:23]
There we go. Yeah, there, that's fine for now. Cool, so now, if I do this, We get an invalid arg type. So, let's take a look and see what we've got here. Console.log, buildPath. And we'll get rid of this. Oops, and then we'll write this. Now an arg type, let's try this.
[00:15:01]
Let's go to here. Comment that out, let's see what this says. That would be why. So why are we not, because it's config
>> It's config, not build, yeah.
>> Conventions are good to stick with. [LAUGH]
>> Should make that a rule.
>> What's that?
>> [SOUND] [LAUGH]
[00:15:27]
>> You got me! [LAUGH] So let's see if this loads now. There we go. So we should get a buffer out of this. Good call, good call. Thank you for the help on that one. [LAUGH] Bam, so what we wanna do now is is we just wanna add to this and we simply wanna make sure it knows that the encoding it needs.
[00:15:52]
And the best way to do that is just to do encoding and then I believe it's just UTF8, if I'm not mistaken. Bam, cool. So, what have we got now? Now, we've got a CLI tool that can read build.json. Not only that, it can have a service name and a service type that we can give it if we need to.
[00:16:20]
So we have successfully completed this part. That was not a green I was expecting. [LAUGH] There we go, that's a better green. So we've successfully completed this part. We now have the CLI tool being able to load in the build.json. And if we wanted to, we could even do json.parse and configuration, and then do as BuildJSON.
[00:16:53]
And then we can say const configurationData like stuff and then configurationData. If I can spell configuration properly. Bam and now we have it in a parsable format that we can easily use. So now I should just be able to do dot and there it is. There's my service name and so forth and so on.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops