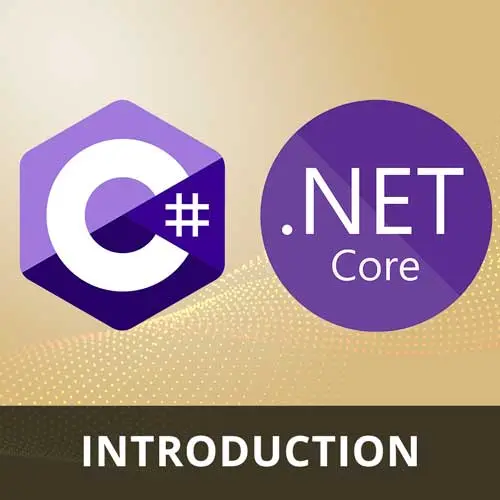
Lesson Description
The "Variables" Lesson is part of the full, C# and .NET Basics course featured in this preview video. Here's what you'd learn in this lesson:
Spencer explains that variables are a way to store data in a specific scope. Spencer covers the use of the "var" keyword for implicitly typed variables, the "const" keyword for constant values, and the difference between value types and reference types. He also touches on the scope and visibility of variables.
Transcript from the "Variables" Lesson
[00:00:00]
>> Spencer Schneidenbach: All right, so let's talk quite a bit about declaring variables. So this is gonna look familiar if you're a programmer already. But if you're new to programming this is a variable is just basically a way of kind of containing some data in a specific scope. In this case a scope is just what's denoted by these curly braces.
[00:00:23]
So there's one main way of declaring variables, but a few different ways that you can kind of declare them differently depending on what it is you're trying to do. At a minimum, the declaration for a variable looks like this, which is, we're just gonna use int as our example, which is a representative of a 32 bit integer, and we're gonna call it number of times or something.
[00:00:52]
And you can see that we've just declared a variable. It doesn't have a value in it. Implicitly, an integer has a value of zero. So we'll talk about that more when we get into value types and reference types and default values. But this, in and of itself, is a way to declare a variable.
[00:01:11]
You can set the variable to a particular value by going number of times equals five, so equals is the, what's called the assignment operator. So that's how you assign a value to a variable. But you don't need two lines to do this. You can also just do it all in the same line, which is typically what I do.
[00:01:31]
I almost always declare my variables and just put them in the same line, unless there's some kind of piece of code, I don't know what this value is going to be ahead of time, so I'm just gonna declare because I know I'm going to need it very soon.
[00:01:44]
One thing to mention that for variable declaration, strictly speaking, you don't need to declare the type so you can write what's called var. So var, if you're coming from a language like JavaScript, var kind of has a bad reputation. It is not at all the same. I mean, it is then that you declare a variable with it, but it's very different in that.
[00:02:03]
It's just basically saying, hey, just interpret the type for me, right? And type is interpreted at compile time, not run time. So you can see that when we hover over it, because we know that we've assigned it to five, the compiler and .NET has already figured out this is an integer, okay?
[00:02:19]
And it won't let you do a var declaration without something assigned to it. So it says, implicitly typed variables must be initialized.
>> Student 1: Can the type of var of implicitly typed variables change? Yeah, if you start out with number of times equals five, can you reassign it as a string?
[00:02:41]
>> Spencer Schneidenbach: No. So no, the type will not change. So if you try to type it as equals five and then you say number of times equal to some string value, it won't let you do it. It will say you can't implicitly convert a string to int. You can do that sometimes not on vastly different types.
[00:03:01]
If they're in the inheritance hierarchy, if the type that you're assigning it to was that type, then yes, you could do it. For example, you could declare this as object, all types inherit from object, which we'll get into types here more in a bit. But because everything Including int and string have a common shared inheritance of object, you can assign this number of times variable to any value you want.
[00:03:32]
Good question.
>> Student 2: Is C-Sharp strictly typed?
>> Spencer Schneidenbach: C-Sharp strictly typed ,yes, it is, yeah. There is a dynamic keyword for dynamic typing. I don't cover it in this course, because I don't use it. I use it. I don't use it [LAUGH] very, very, very, very sparingly, but it is strictly typed.
[00:03:54]
Yes, good question.
>> Spencer Schneidenbach: Something you can do and something that I don't do is you can, if you want, declare multiple variables in comma separated. I choose not to do this, mostly because most of the time I'm assigning to values anyways, and I like the multiple lines to, I don't think less lines of code is necessarily a better thing.
[00:04:18]
I think in general, that's a good rule but not necessarily for variables. I like to just put all my variables at the top of a statement and just assign them all separately to kind of just show exactly what they are. So you can do this. In practice, I don't.
[00:04:31]
I don't really think it adds much to the clarity of the language. So let's see. I'm kind of going off the back of, well, talking about how I declare variables. Almost all of the time you could call it laziness, or force of habit. I'm always using var. Var is probably my favorite way of declaring variables.
[00:04:56]
I don't think not using var adds. I know some developers are strictly speaking, they really want to declare the type of the thing ahead of time. And that's fine if you want to do that. Me, personally, I'm fine with var. It also has the benefit of putting the variable names, kind of starting on the same fifth, fourth character, fifth character, which is a small thing, but.
[00:05:20]
But maybe it enhances readability, kind of reaching on that one. But I almost always exclusively use var. I do for certain instances, which we'll get into, I will use, especially in the next course, I will use type declaration. Especially, of course it's required if I can't initialize that variable at the same time, I will use a type declaration to make it super clear what it is I'm trying to do.
[00:05:48]
>> Spencer Schneidenbach: Let's talk about the const keyword for a second. Const, again, is similar, but different than if you're coming from JavaScript, const is my var when I'm writing JavaScript, I use const and let because var in JavaScript has problems and C-Sharp it does not have any of the problems that it has, but there is a const keyword.
[00:06:10]
And this is a little bit confusing because const is really a compiler time, kind of like static variable, and it really is only good for using value types. You cannot set a reference type to a const, you would use a static instead, which we'll get into.
[00:06:24]
But this is what it looks like.
>> Spencer Schneidenbach: So this is something that you can declare in a specific scope. So in this case, I've declared it in the mains method scope. You don't have to put it there. You can also put it at class scope for something that would be used among multiple different things that might access this variable.
[00:06:49]
So const is different in JavaScript, in that, like I said, you don't assign anything but integers and strings or floats, Boolean. Those are the kinds of things that you would assign to a const. It wouldn't be used in most of your programming. Most of the time you're gonna be using var or declaring the variable with the with the type, like that,
>> Spencer Schneidenbach: Variables fall out of scope.
[00:07:19]
So that's a really important thing to say. So let's show a quick example of an if statement. So if this value is true then we wanna execute this particular piece of code. So I'm gonna move my number of times variable declaration there to show that if I try to access it outside of scope.
[00:07:44]
>> Spencer Schneidenbach: I can't do it, because variables are invariably scoped inside of the curly braces in which they live. There's also, when we talk about visibility more, we'll talk about variables at the class level declared with different types of visibility in order for this to be accessible outside of 'Program' for instance this would need to be 'public' but we'll get into that more as we talk about accessibility modifiers.
[00:08:09]
>> Student 1: Is var inherently a broader scope or I forget what it's called, in the way that it is in JavaScript?
>> Spencer Schneidenbach: No, that is a great question. So believe what you're kind of asking about is the thing called hoisting or right where the the var statement in JavaScript gets moved.
[00:08:35]
Essentially, if you declare this as a var, you'd be able to access this in JavaScript outside of its scope. C-Sharp has none of that. All variables fall out of scope when you go out a block. And it could be a block like this, it could be a block inside of a method, it could be a block inside of a class, but it doesn't have the same kind of assignments, semantics and memory implications that JavaScript does.
[00:08:59]
Really great question. That's a really great question, a really important point because, like I said, var is totally different. It's obviously very similar because you're declaring variables with it, but it's totally different in practice. Great question.
>> Student 1: Is then an analogous way of hoisting it to use the public word?
[00:09:18]
>> Spencer Schneidenbach: Yeah, kind of. Yeah, so if you wanted to expose it to a broader scope. This would be kind of the way to do that, but it wouldn't put it in if you had a separate class declaration, you couldn't just implicitly access this max score unless you used its, what we call the fully qualified name.
[00:09:40]
So you'd be able to void TestMethod. You can't just go up here and because this is declared as const in a high level scope, you can't just say MaxScore and expect to be able to access it. It doesn't have any of that, but you can access it because it's declared as public.
[00:10:00]
You could go public, sorry, Program.MaxScore and access the variable that way. So it doesn't have nearly the same poor implications as it does in JavaScript. Last thing we'll talk about is value types versus reference types, which we will talk about more as we get into the type conversation.
[00:10:19]
But C-Sharp variables can Invariably be categorized into one of two types, which is that there are value types, things like int and bool, that are built into the language and have default values, for instance, that are essentially a value, like an int is always its default value is zero.
[00:10:38]
Bools is false. Floats is zero, when you initialize them, they are initialized in memory.
>> Student 2: Are there class wrappers of int float, etc?
>> Spencer Schneidenbach: That's a great question. The answer is kind of, and we'll talk about that when we get into talking about value types and reference types a little further in, but essentially you can declare a value type as a reference type, and you would do that by calling this object.
[00:11:07]
This converts this five into a reference type. And I'll go through the implications of that. It's not something I do super on a very common. It's not common at all, but it is something that is important to know as you get into the language. Good question. So I do want to touch on variables when you declare them, they're either going to be, like I said, a value type or a reference type.
[00:11:28]
So this is a value type because it's declared, even if you declare it as an int or a var, it doesn't matter. It's exactly the same thing to C-Sharp. This is what's called a value type. Reference types, value types, as they're passed to things are passed by copy, so they're copied over, as opposed to referred to directly.
[00:11:45]
Reference types for things like object, let me just put this here, delete that object referenceType. Reference types are typically complex types. Things that are declared as class are going to be reference type. String is a reference type that's kind of treated like a value type. Again, we'll get to that in a little bit.
[00:12:07]
We'll talk a little bit more about the implications of this as we get further into the course. But it's important to know for now that there are value types and reference types when you do declare variables, and there are implications to both.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops