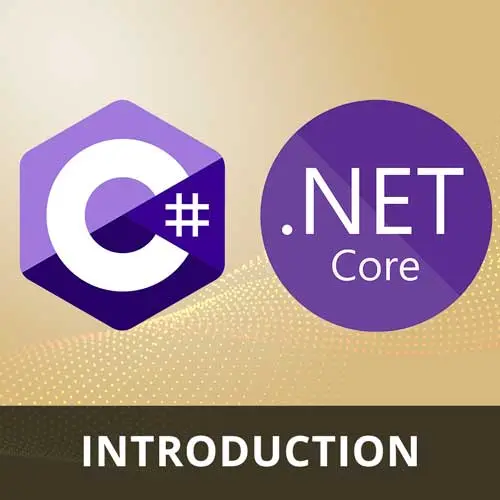
Lesson Description
The "Setup .NET CLI" Lesson is part of the full, C# and .NET Basics course featured in this preview video. Here's what you'd learn in this lesson:
Spencer demonstrates how to create a new console application using the .NET CLI and explores the generated files and folders. He also explains the purpose of the solution file, the project file, and briefly mentions the option to use a program.main style program instead of top-level statements.
Transcript from the "Setup .NET CLI" Lesson
[00:00:00]
>> Spencer Schneidenbach: Okay, using the .NET CLI, so let's create our new first console application. So I am in my repos directory, cuz I always have a repos directory. And I'm gonna say dotnet new console, and I am going to call it, I'm gonna say -n to give it a name, MyFirstApp.
[00:00:19]
Then you can see that it does a couple of things. You see that it happens all very quickly, it all happens so fast. So we're gonna go look at that. First, we're gonna see, cd into MyFirstApp. And then we're gonna hit ls. And you're gonna see that we have a thing called a csproj file, which we'll get into.
[00:00:36]
Program.cs, which is our entry point inside of the application. And an obj directory, which typically contains restored packages and whatnot.
>> Spencer Schneidenbach: All right, but let's take a look at it in Visual Studio Code, kinda take a fully integrated tour, as it were. So I'm gonna go into Repos.
[00:00:54]
I'm gonna go into MyFirstApp, and I am going to go here. And a couple of things that I wanted to point out, I'm gonna go and close this. If you're used to Visual Studio Code, some of this is gonna look pretty familiar. So you'll see that a solution file got generated, which is done by the C# Dev Kit.
[00:01:15]
So if you have multiple projects as part of your solution, you can see that the solution file will be the container for all of them. You can see that it's kind of a bunch of gobbledygook. [LAUGH] You could tell that it's just some of the vestigial stuff from .NET of old, but most of the time, you're not looking at that anyways.
[00:01:32]
You can just ignore it. We have our MyFirstApp.csproj file. So this defines the packages that are installed as part of your application. What version of .NET are you on? What version of C# are you using? In this case, we're just using the latest one. Do we wanna have nullability enabled?
[00:01:51]
Which we'll get into nullability here in a bit. And the output type of this application, it could be a library, typically, or EXE. In this case, we're just outputting an executable because that's what we're running. This is a console app. And then we're gonna look at Program.cs, which frankly looks a little lonely, I have to say.
[00:02:10]
So all we have is a Console.WriteLine("Hello, World!"), which is a very typical way to enter in an application, if I do say so myself. So I am going to hit Ctrl+Shift+tilde to open up the terminal. So I have that there, and I'm gonna hit dotnet build. And you're gonna see that the thing builds, which if it didn't, I would very much hope that it would.
[00:02:31]
And then we will go ahead and do dotnet run to run our application. And you can see it printed Hello, World here, and we change this. And you see, we run it again. Nope, I didn't save it. I don't need to turn on autosave, perfect, okay. Hello, World, there we go, boom.
[00:02:52]
You see that it's pretty simple. The project structure, if you've done any legacy .NET development, looks a lot simpler, a lot different than it did ten years ago when it was .NET Framework. They've spent a lot of time kind of simplifying things, which I think is a really good thing.
[00:03:11]
I do wanna point out a couple of things about the extension as well. You see that we have this little play button right here. We can run this and it will run our application, as you can imagine. You can also use it to debug it. So this is a little bit ahead, but if you're a person who likes to be able to run your application line by line, we have a fully integrated debugger in that.
[00:03:34]
That's part of C# Dev Kit. That's part of the licensed product. And then we have this down here, let's go back here. And I wanna just point out Solution Explorer. So if you're used to Visual Studio at all, or JetBrains, right, or anything, usually there's kind of a hierarchical Solution Explorer view, which is what we have here.
[00:04:02]
So this is basically the C# Dev Kit extension saying, hey, I see a project file, I see a solution file. Let's look at all those things. So you can see that we have the same stuff, but it hides the SLN file, and it really just kinda shows you what's there.
[00:04:15]
So if you have something in that directory, a file in that directory, say, and you've excluded that file, it wouldn't show up here. But it would show up up here in the usual Visual Studio or the Visual Studio Code view.
>> Student: What is the benefit of creating SLN before creating a .NET project?
[00:04:35]
>> Spencer Schneidenbach: What is the benefit of creating an SLN before creating a .NET project? If you know that the project that you're doing file new project on or dotnet new on is going to be multiple, is gonna encompass multiple different projects. Then it's a good idea to start with a solution file.
[00:04:52]
But, like I said, in Visual Studio and in JetBrains Rider and Visual Studio Code with the C# Dev Kit extension, one will usually be generated for you.
>> Spencer Schneidenbach: And you can use the .NET tooling to create, if you go to the previous page, you can see that there is a, well, it's not here.
[00:05:12]
But if you go to the dotnet new list, you'll see that solution file is one of the things. So you can just create a solution file on its own. I usually just let the IDE do the work for me. I'm lazy like that.
>> Student: How does the console app know to execute the code in Program.cs?
[00:05:31]
>> Spencer Schneidenbach: That's a great question. I'll go ahead and spoil it for you a little bit. One of the things that Microsoft has done is kind of tried to eliminate boilerplate as much as they can. So how does it know to do that? Implicitly under the hood, it actually creates a program class, and then it creates a main method for it to execute on.
[00:05:50]
So these are all just high level statements. And when I say spoiler alert, one of the things I could bridge into an IDE feature that I particularly like, you see this little yellow light bulb here? A little yellow light bulb is Visual Studio Code's way of saying, the C# Dev Kit's way of saying, hey, got something you can do here.
[00:06:06]
And in this case, we can actually convert this to a Program.Main style program. So what it'll do is it will actually generate for you the under the hood stuff. It will generate the stuff for you that if you're just sticking with the high level language, if you're just sticking with the top level statements, you can totally do that.
[00:06:28]
But you can also run your programs like this. And Microsoft knows. Basically what it does is the .NET tooling looks for a method called main that has a few different what we call method signatures, we'll get into that. But it looks for a method called main to actually run the program.
[00:06:45]
When you're using just top level statements like this, it's just generated for you. Great, great question.
Learn Straight from the Experts Who Shape the Modern Web
- In-depth Courses
- Industry Leading Experts
- Learning Paths
- Live Interactive Workshops